C++ can be compiled into assembly language, allowing developers to optimize code at a lower level for performance; below is a simple C++ code snippet demonstrating this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this C++ code to assembly, you could use a command like `g++ -S hello.cpp` which generates an assembly file named `hello.s`.
Understanding Assembly Language
What is Assembly Language?
Assembly language is a low-level programming language that has a close relationship with machine code. Unlike high-level languages like C++, which abstract away the details of the hardware, assembly allows programmers to write instructions that directly manipulate the hardware of a computer. Each assembly language corresponds to a specific architecture, which means that assembly code is generally not portable across different computer architectures.
Key characteristics of assembly language include:
- The use of mnemonics for instructions (such as MOV, ADD, SUB) instead of numeric opcodes.
- A detailed control over hardware operations.
- The necessity of managing memory addresses and registers directly.
Importance of Learning Assembly
Understanding assembly language offers several advantages, particularly in optimizing performance and debugging C++ applications.
Performance Optimization
When you write C++ code, a compiler translates it into machine code, often optimizing as it goes. However, understanding assembly allows you to see how your high-level code relates to the CPU’s instruction set. By directly manipulating assembly code, you can write more efficient algorithms that can significantly enhance execution times—a critical asset for performance-sensitive applications.
Debugging
Knowledge of assembly improves your debugging capabilities. When debugging complex issues, examining the assembly code generated by the compiler can provide insights into the behavior of your C++ program. You can better understand how variables are stored and manipulated in memory, uncovering bugs that high-level language debugging might miss.
Resource Management
Learning assembly gives you better control over system resources. By using assembly, you can carefully manage memory allocation, improve response times, and reduce latency in applications, which is essential in environments where resources are limited, such as embedded systems and real-time applications.
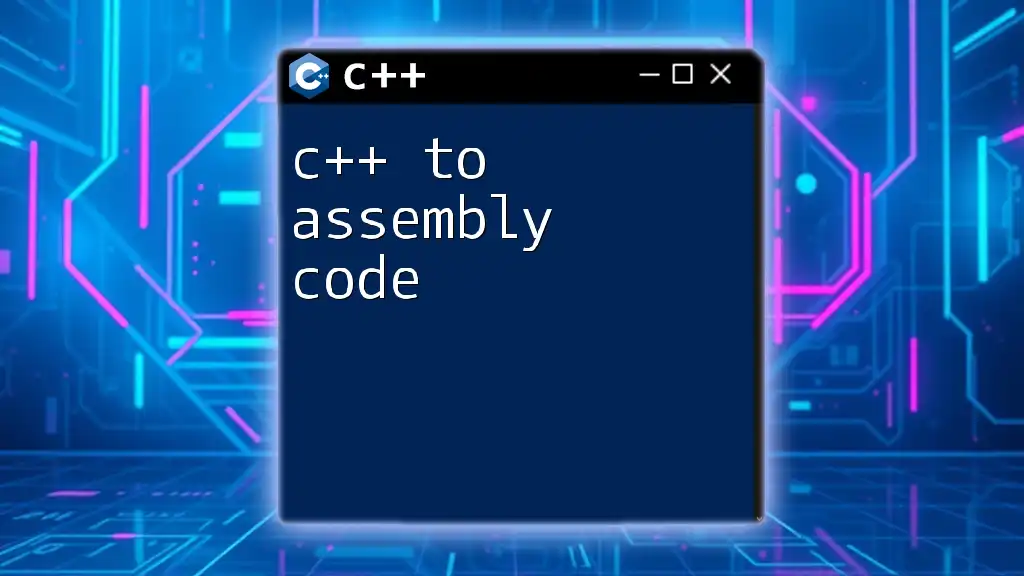
The Basics of C++ and Assembly Interoperability
How C++ Relates to Assembly
To grasp the relationship between C++ and assembly, one must understand the compilation process. This process involves multiple stages:
- Preprocessing: The compiler processes headers and macros.
- Compilation: The C++ code is transformed into an intermediate format.
- Assembly: The intermediate code is translated into assembly language.
- Linking: The assembly code is linked with libraries and other modules into an executable.
Each stage modifies the code in ways that affect its execution, leading to the assembly language’s critical role in the overall process.
C++ Constructs Translated to Assembly
Some common C++ statements and their corresponding assembly code can help elucidate this relationship.
Variable Declaration
When you declare a variable in C++, such as:
int x = 5;
The equivalent assembly code might look like:
mov eax, 5
mov [x], eax
This snippet shows how the integer value `5` is loaded into the `eax` register before being stored in the memory location representing `x`.
Control Structures
Control structures in C++ — such as loops and conditionals — are also translated to assembly. For example, an `if` statement in C++:
if (x > 10) { /* do something */ }
Could translate to:
cmp [x], 10
jg Label
Here, `cmp` compares the stored variable with `10`, and if the condition is met, the program jumps to a specified label for further instructions.
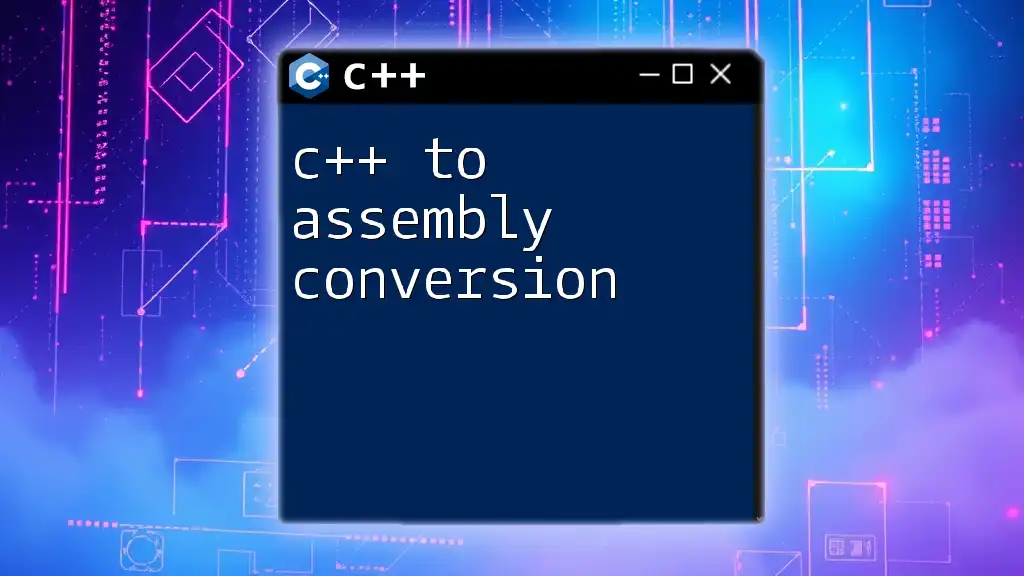
Compiling C++ to Assembly
Tools and Environment Setup
To compile C++ code into assembly, you need a reliable compiler. Some of the most commonly used compilers include GCC and Clang. Setting up these compilers on your system is usually straightforward, but make sure to follow the appropriate installation guides according to your operating system.
Generating Assembly Code from C++
Once your environment is set up, you can generate assembly code from your C++ files using specific compiler flags. For instance, with GCC, you can execute:
g++ -S your_program.cpp -o your_program.s
This command instructs the GCC compiler to generate an assembly file from your C++ source code. The `-S` flag tells the compiler to compile only to assembly language.
Analyzing the Generated Assembly Code
When you examine the produced assembly code, you will encounter several segments:
- .text: This section contains the executable code, where the actual instructions reside.
- .data: This section holds global and static variables that are initialized before use.
- .bss: This portion reserves space for uninitialized variables.
Understanding these segments helps you gain insight into how your high-level constructs are represented at a lower level.
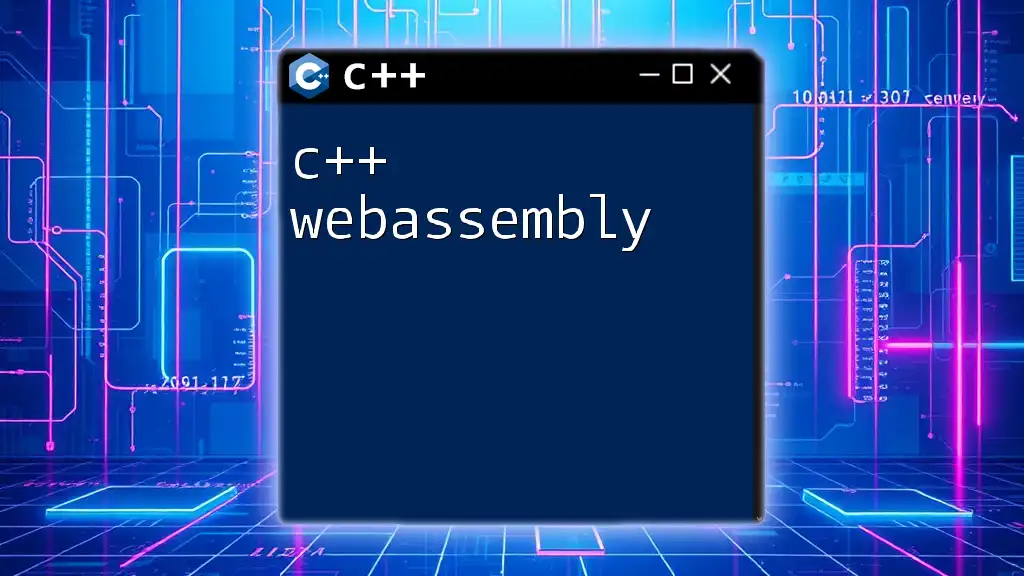
Advanced Topics in C++ to Assembly
Inline Assembly in C++
Inline assembly allows you to include assembly language instructions directly within your C++ code. This approach is useful for optimizing specific operations without having to manage a separate assembly file. For example:
int x = 5;
asm ("movl $5, %eax");
In this code snippet, the inline assembly instruction `movl` loads the value `5` directly into the `eax` register during the execution of the program.
Calling Conventions
Called conventions dictate how functions receive parameters and return values. Different systems adopt various calling conventions, such as cdecl, stdcall, and others. Understanding these conventions is essential for ensuring that your C++ functions interface correctly with assembly code, especially in mixed-language programming situations.
Optimization Techniques
Compiler optimizations are crucial for improving performance. Common flags like `-O2` enable various optimization strategies that can lead to faster execution of your C++ code. However, there are times when you might want to perform hand-tuning of assembly code to achieve even greater performance gains. Recognizing when manual optimization is necessary versus relying on the compiler is a crucial skill in performance-critical applications.
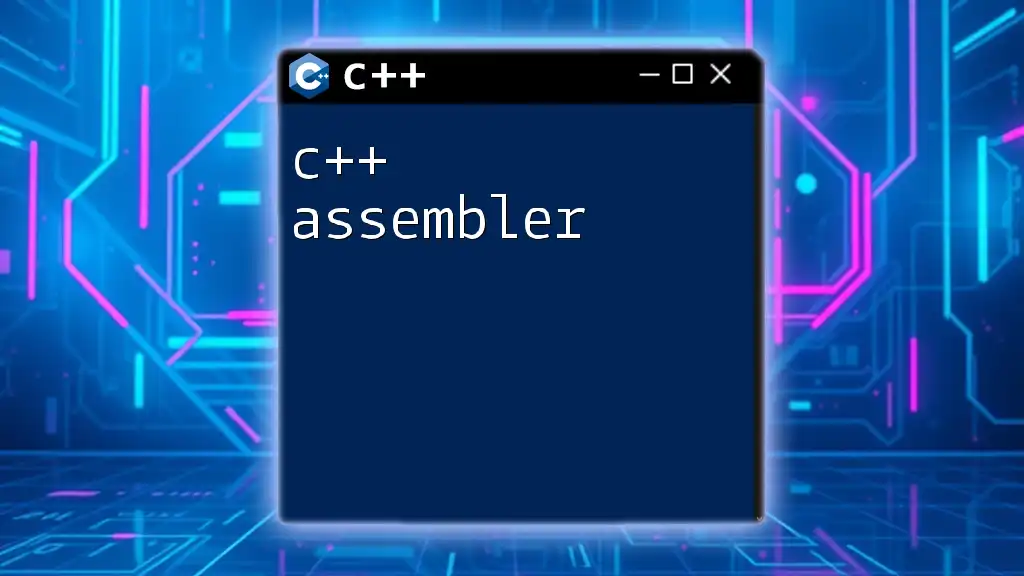
Real-world Applications
Performance Critical Applications
Assembly language is often used in performance-critical applications. Systems programming, embedded systems, and game development frequently require optimized code that runs efficiently and responsively. In such areas, using assembly can lead to marked improvements in speed and resource management.
Debugging and Reverse Engineering
An understanding of assembly is also invaluable in debugging applications and conducting reverse engineering tasks. When faced with obscure bugs or needing to understand the inner workings of existing code, the ability to read and interpret assembly language can be a game changer.
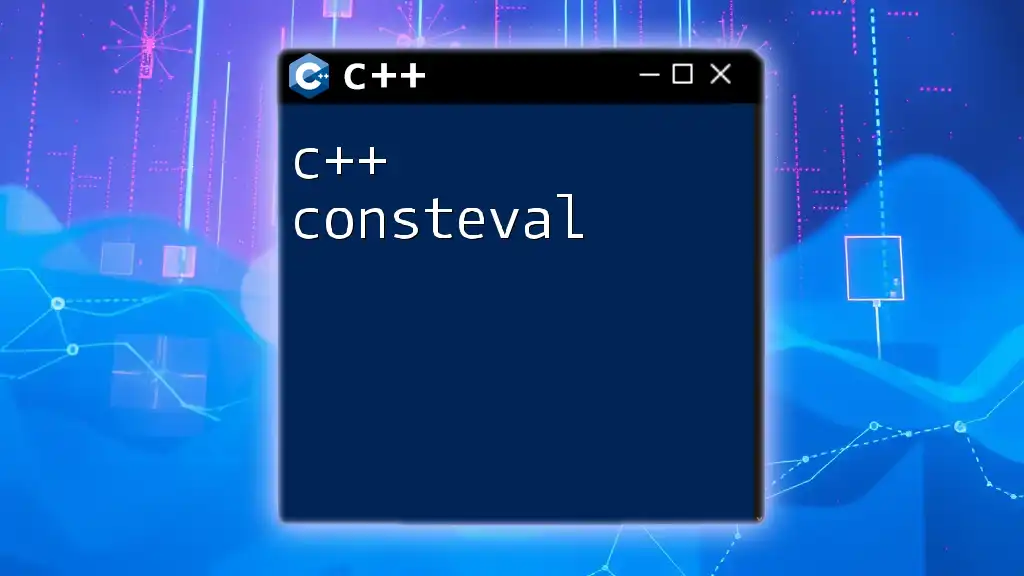
Conclusion
The relationship between C++ and assembly is pivotal for programmers looking to optimize performance and understand the underlying mechanics of their applications. Bridging the gap between high-level code and low-level assembly empowers developers to write robust, efficient programs. Your journey into the intricacies of assembly language can greatly enhance your programming toolkit—encouraging you to explore further and master the craft.
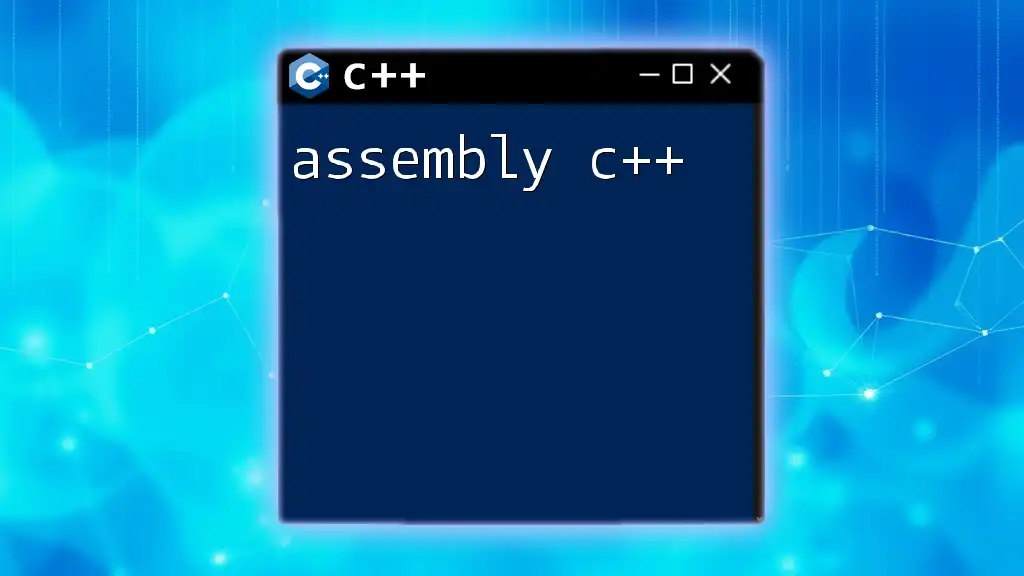
Additional Resources
To deepen your understanding of C++ and assembly programming, consider exploring advanced tutorials, online courses, and relevant literature that delve into these subjects.
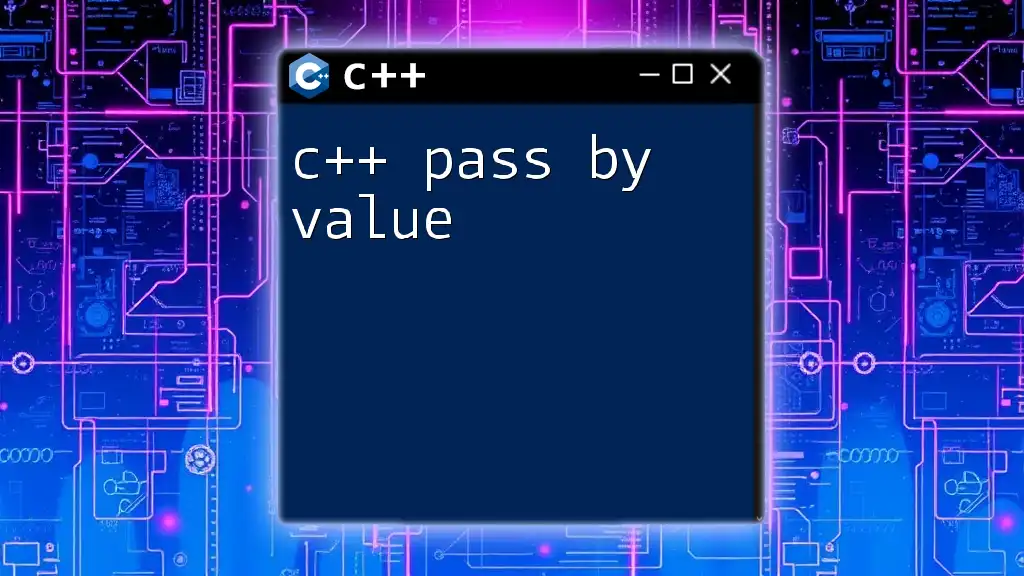
Call to Action
If you're eager to enhance your skills in C++ and assembly language, contact us for tailored courses and personalized assistance to help you master these powerful programming tools.