C++ to assembly conversion involves translating high-level C++ code into low-level assembly language, enabling greater control over hardware and optimization for performance.
Here’s an example of a simple C++ function and its corresponding assembly code snippet:
// C++ code
int add(int a, int b) {
return a + b;
}
The equivalent assembly code (for x86 architecture) might look like this:
; Assembly code
add:
mov eax, edi ; Move first argument into eax
add eax, esi ; Add second argument to eax
ret ; Return the result in eax
Understanding the C++ to Assembly Conversion Process
How C++ is Translated to Assembly
The C++ to assembly conversion begins with the compilation process, which is crucial for turning high-level code into machine-readable instructions. This process involves several steps:
-
Preprocessor: The C++ preprocessor handles directives (such as `#include` and `#define`) and prepares the code for the compiler. It expands macros and includes header files.
-
Compiler: The compiler translates the expanded source code into assembly language specific to the target architecture. Here is where the actual conversion takes place.
-
Assembler: The assembler translates the assembly code into machine code or object code, generating a binary file that the computer can execute.
-
Linker: Finally, the linker takes one or more object files generated by the assembler and combines them into a single executable.
C++ to Assembly Language Converter Tools
Several tools can facilitate the C++ to assembly conversion process, enabling developers to analyze how their C++ code translates into lower-level instructions.
-
GCC (GNU Compiler Collection): A widely used toolchain that supports various programming languages, including C++. It provides options to output assembly code directly.
-
Clang: Another powerful compiler that focuses on providing fast compilation and excellent diagnostics. It can also generate assembly output alongside its standard compilation capabilities.
Step-by-Step Guide to Convert C++ Code to Assembly
Preparing Your Environment
To start converting C++ code to assembly, you need to have a suitable environment set up. Here are instructions for installing GCC and Clang on various operating systems:
-
Windows: You can download a distribution like MinGW, which includes GCC. Alternatively, the Windows Subsystem for Linux (WSL) allows you to install a more Unix-like environment where GCC can be used directly.
-
Linux: Most distributions offer GCC through their package managers. You can install it via commands like `sudo apt install build-essential` for Ubuntu or `sudo dnf groupinstall "Development Tools"` for Fedora.
-
macOS: You can use the Xcode Command Line Tools. Simply run `xcode-select --install` in the terminal.
Basic C++ to Assembly Conversion Examples
Now, let's look at a practical example of converting a simple C++ program into assembly language.
Simple C++ Program Example
#include <iostream>
int main() {
std::cout << "Hello World!" << std::endl;
return 0;
}
Using GCC to Generate Assembly Code
To convert the above code to assembly using GCC, we can run the following command:
g++ -S hello.cpp
This command produces an output file named `hello.s` that contains the corresponding assembly code. You can explore the generated `.s` file to see how the compiler represented the C++ constructs in assembly.
Using Clang to Generate Assembly Code
If you prefer using Clang, use this command:
clang++ -S hello.cpp
Like GCC, Clang will create an assembly file (e.g., `hello.s`) where you can observe the assembly language representation of your C++ code.
Analyzing the Assembly Output
Understanding the assembly generated from your C++ code is vital for performance optimization and debugging. Assembly language consists of a set of mnemonic codes corresponding to machine instructions.
Understanding the Generated Assembly
Assembly output typically includes various instructions such as `mov`, `add`, `sub`, and more. These instructions interact directly with CPU registers and memory. In the generated assembly code, you will commonly see sections labeled `.data` (for initialized global and static variables) and `.text` (for the code itself).
Interpreting Symbols and Directives
The assembly file will use symbols to refer to functions and variables. Additionally, it may have directives such as `.global` or `.section` that tell the assembler how to handle code and data segments. Familiarizing yourself with these syntax components will enhance your ability to read and understand assembly language effectively.
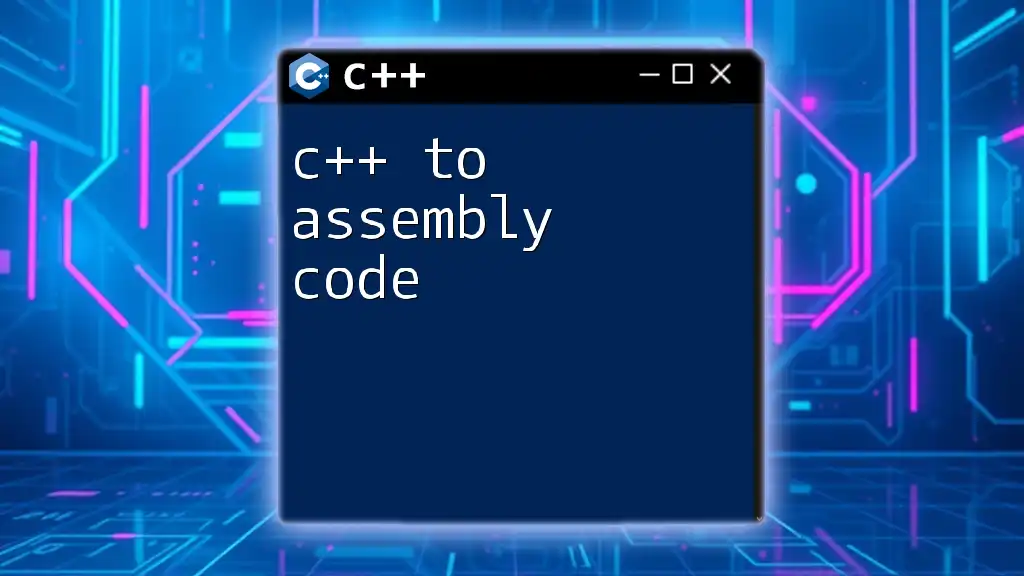
Advanced C++ Constructs and Their Assembly Equivalents
Converting Functions and Classes
Certain C++ constructs may require more complex translations into assembly.
C++ Functions to Assembly
Consider the following simple function in C++:
int add(int a, int b) {
return a + b;
}
When this code is compiled into assembly, you would see instructions representing the function parameters stored in registers, an addition operation, and the return value managed accordingly.
C++ Classes and Objects in Assembly
A more complex scenario involves classes. For instance:
class Example {
public:
int value;
void setValue(int v) { value = v; }
};
When compiled, the class members and methods translate into assembly instructions that handle object instantiation, member access, and method invocation.
Handling Control Structures
If Statements and Loops
Control structures like if statements and loops are also translated into assembly language. For instance, a simple C++ if statement:
if (x > y) {
// ... do something
}
This will generate conditional jumps in assembly, allowing the CPU to branch based on the result of a comparison.
Switch Statements in Assembly
Switch-case structures are usually transformed into jump tables or sequences of comparisons in assembly, providing efficient multi-way branching capabilities.
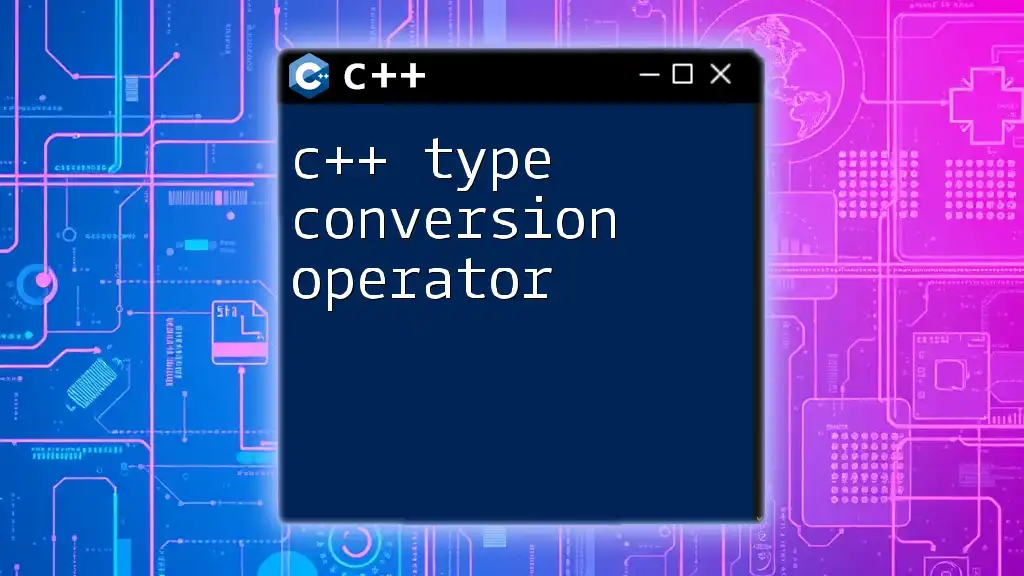
Tips for Optimizing C++ Code for Assembly
Best Practices for Efficient C++ Code
Making educated decisions in crafting C++ code can result in more efficient assembly. Here are some best practices:
- Prefer primitive data types over complex structures when performance is critical.
- Minimize function calls within tight loops to reduce overhead.
- Use inlining for small functions to eliminate call overhead.
Utilizing Compiler Optimization Flags
Compilers provide optimization flags that significantly affect the assembly output. For instance, using:
g++ -O2 hello.cpp -o hello
The `-O2` flag instructs the compiler to apply various optimizations without compromising compilation time drastically.
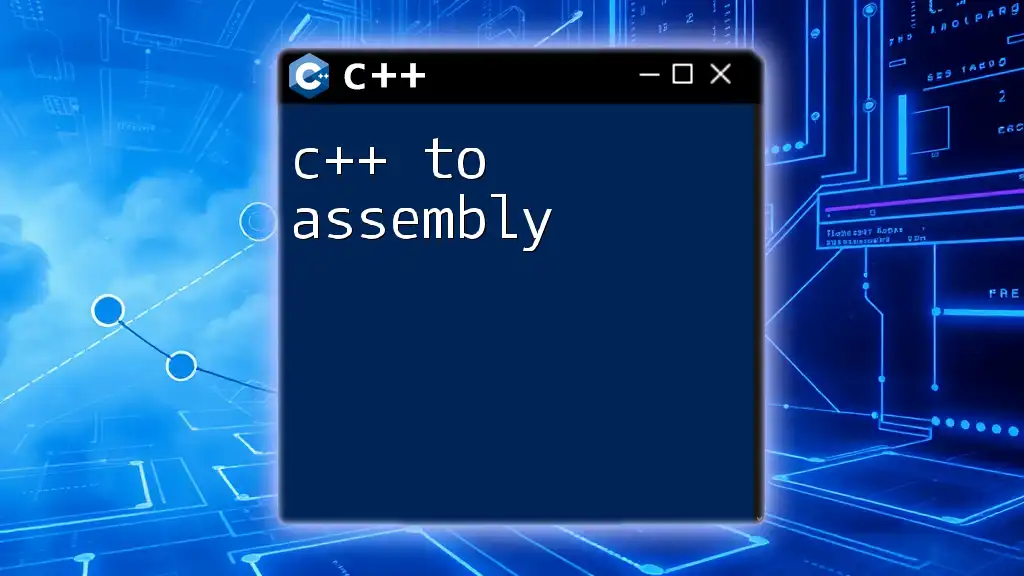
Conclusion
C++ to assembly conversion plays a pivotal role in understanding how high-level constructs translate into machine-readable code, allowing for optimizations and insights into performance bottlenecks. This guide equips you with the knowledge and tools necessary to explore this important aspect of programming deeply. Continuous learning and practice will further enhance your skills in navigating from C++ to assembly language, improving your coding efficiency and capabilities.
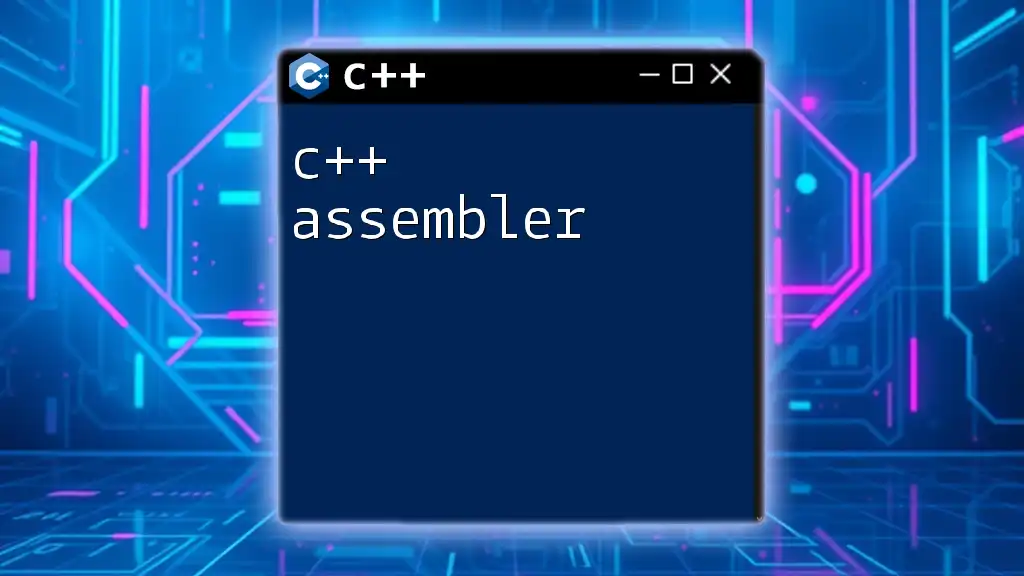
Additional Resources
Explore further learning resources such as practical code examples on GitHub, online courses focused on systems programming, and community forums where you can engage with other enthusiasts in C++ and assembly languages.