Matrix inversion in C++ involves calculating the inverse of a given square matrix using techniques such as Gaussian elimination or the adjugate method; here’s a simple implementation using the Eigen library.
#include <Eigen/Dense>
#include <iostream>
int main() {
Eigen::Matrix2d A;
A << 4, 7, 2, 6; // Example 2x2 matrix
Eigen::Matrix2d A_inv = A.inverse(); // Inverse of matrix A
std::cout << "The inverse of the matrix is:\n" << A_inv << std::endl;
return 0;
}
Understanding Matrices
What is a Matrix?
A matrix is a rectangular array of numbers arranged in rows and columns. The values contained in these cells are called elements. Matrices are fundamental tools in both mathematics and computer science, serving a multitude of functions, from handling systems of linear equations to representing transformations in graphics.
Types of Matrices
Matrices can take various forms, each with unique characteristics and uses:
- Square Matrix: A matrix with an equal number of rows and columns. These matrices are crucial for defining determinants and inverses.
- Identity Matrix: A special type of square matrix where all the diagonal elements equal one, and all other elements are zero. It acts as the multiplicative identity in matrix multiplication.
- Diagonal Matrix: A matrix where all non-diagonal elements are zero. They simplify various computations, particularly in linear transformations.
Understanding these types will help in grasping matrix operations, especially when discussing C++ matrix inversion.
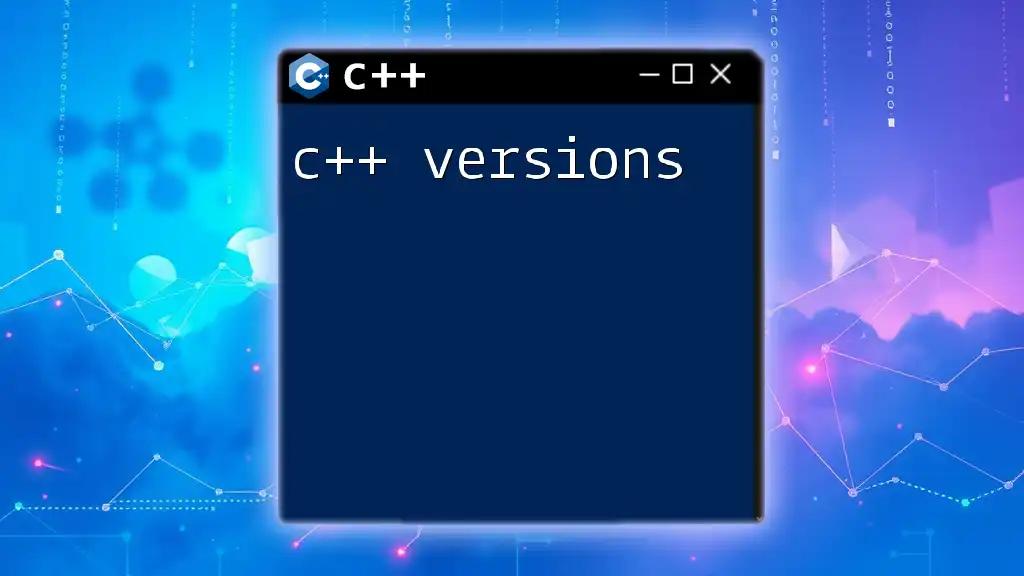
Matrix Inversion Fundamentals
What is Matrix Inversion?
Matrix inversion refers to the process of finding an inverse matrix \( A^{-1} \), which satisfies the equation \( A \cdot A^{-1} = I \), where \( I \) is the identity matrix. The existence of an inverse is vital for solving linear equations efficiently; when a matrix is invertible, we can directly obtain solutions using the inverse matrix.
Conditions for Inversion
Not every matrix possesses an inverse. A matrix is invertible (or non-singular) if its determinant is non-zero. The determinant is a scalar value that reflects certain properties of the matrix, such as its volume scaling factor and whether it can be converted into its reduced row echelon form. If the determinant equals zero, the matrix is singular, and inversion is impossible.
The Inverse of a Matrix
The notation \( A^{-1} \) denotes the inverse of a matrix \( A \). Mathematically, if \( A \) is invertible, the operation of multiplying \( A \) by its inverse yields the identity matrix.
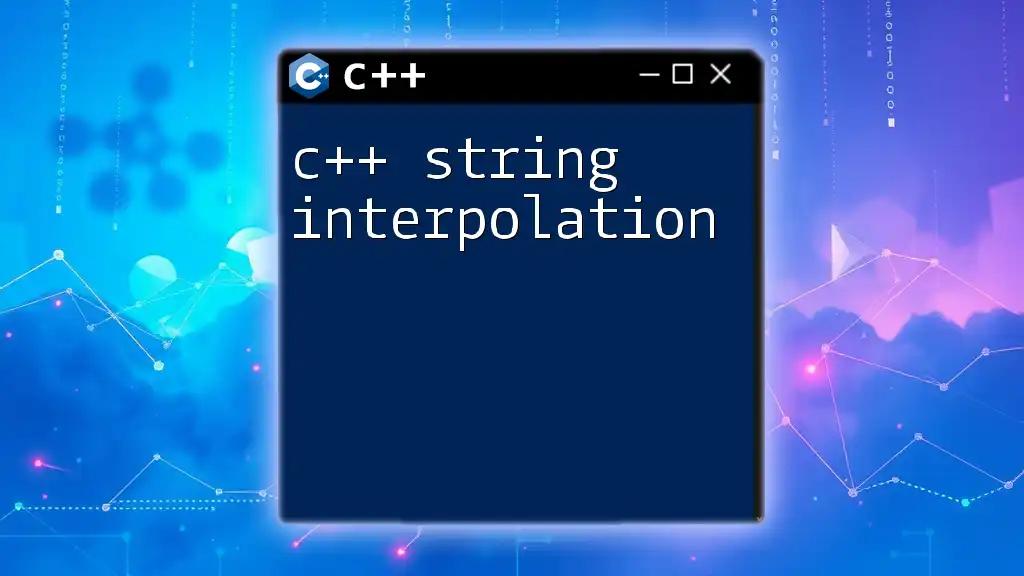
Methods for Inverting a Matrix
Using Gaussian Elimination
Gaussian elimination is a systematic method for performing row operations on a matrix to reach its row echelon form, which can be transformed into an identity matrix, thus revealing the inverse.
Step-by-step Example
Consider a \( 2 \times 2 \) matrix:
\[ A = \begin{pmatrix} 4 & 7 \\ 2 & 6 \end{pmatrix} \]
To find its inverse using Gaussian elimination, we augment it with the identity matrix:
\[ \begin{pmatrix} 4 & 7 & | & 1 & 0 \\ 2 & 6 & | & 0 & 1 \end{pmatrix} \]
Performing row operations, we manipulate the left side to form the identity matrix, leading us to discover \( A^{-1} \).
C++ Implementation
Here is a basic C++ function demonstrating Gaussian elimination for matrix inversion:
void gaussianElimination(double** matrix, int n) {
double** augmented = new double*[n];
for (int i = 0; i < n; ++i) {
augmented[i] = new double[2*n];
for (int j = 0; j < n; ++j) {
augmented[i][j] = matrix[i][j];
augmented[i][j + n] = (i == j) ? 1 : 0; // Identity matrix
}
}
for (int i = 0; i < n; ++i) {
// Normalize row i.
double diag = augmented[i][i];
for (int j = 0; j < 2*n; ++j) {
augmented[i][j] /= diag;
}
// Reduce other rows
for (int k = 0; k < n; ++k) {
if (k != i) {
double factor = augmented[k][i];
for (int j = 0; j < 2*n; ++j) {
augmented[k][j] -= factor * augmented[i][j];
}
}
}
}
// Extract the inverse from the augmented matrix
for (int i = 0; i < n; ++i) {
for (int j = 0; j < n; ++j) {
matrix[i][j] = augmented[i][j + n];
}
}
// Clean up
for (int i = 0; i < n; ++i) {
delete[] augmented[i];
}
delete[] augmented;
}
Using Adjoint Method
The adjoint method involves calculating the adjoint of a matrix and then dividing it by the determinant to find the inverse. The adjoint is the transpose of the cofactor matrix, and it's useful for smaller matrices.
C++ Implementation
Here is an example function to compute the inverse using the adjoint method:
void adjoint(double** matrix, double** adjointMatrix, int n) {
if (n == 1) {
adjointMatrix[0][0] = 1;
return;
}
int sign = 1; // sign multiplier for cofactors
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
// Getting the cofactor for each element
double** temp = new double*[n - 1];
for (int k = 0; k < n - 1; k++) {
temp[k] = new double[n - 1];
}
// Populate temp matrix with minor
for (int x = 0; x < n; x++) {
for (int y = 0; y < n; y++) {
if (x != i && y != j) {
temp[x < i ? x : x - 1][y < j ? y : y - 1] = matrix[x][y];
}
}
}
// Assign cofactor to adjoint matrix
adjointMatrix[j][i] = sign * determinant(temp, n - 1); // Transpose
sign = -sign; // Alternate signs
for (int k = 0; k < n - 1; k++) {
delete[] temp[k];
}
delete[] temp;
}
}
}
double determinant(double** matrix, int n) {
// Determinant calculation using recursion for cofactor expansion
}
Using LU Decomposition
LU decomposition involves breaking down a matrix into the product of a lower triangular matrix \( L \) and an upper triangular matrix \( U \). This method is efficient for larger matrices, especially when multiple inversions may be required on the same matrix.
C++ Implementation
A basic C++ function for LU decomposition could look like this:
void luDecomposition(double** matrix, double** L, double** U, int n) {
for (int i = 0; i < n; i++) {
// Upper Triangular
for (int j = i; j < n; j++) {
U[i][j] = matrix[i][j];
for (int k = 0; k < i; k++)
U[i][j] -= L[i][k] * U[k][j];
}
// Lower Triangular
for (int j = i; j < n; j++) {
if (i == j)
L[i][i] = 1; // Diagonal as 1
else {
L[j][i] = matrix[j][i];
for (int k = 0; k < i; k++)
L[j][i] -= L[j][k] * U[k][i];
L[j][i] /= U[i][i];
}
}
}
}
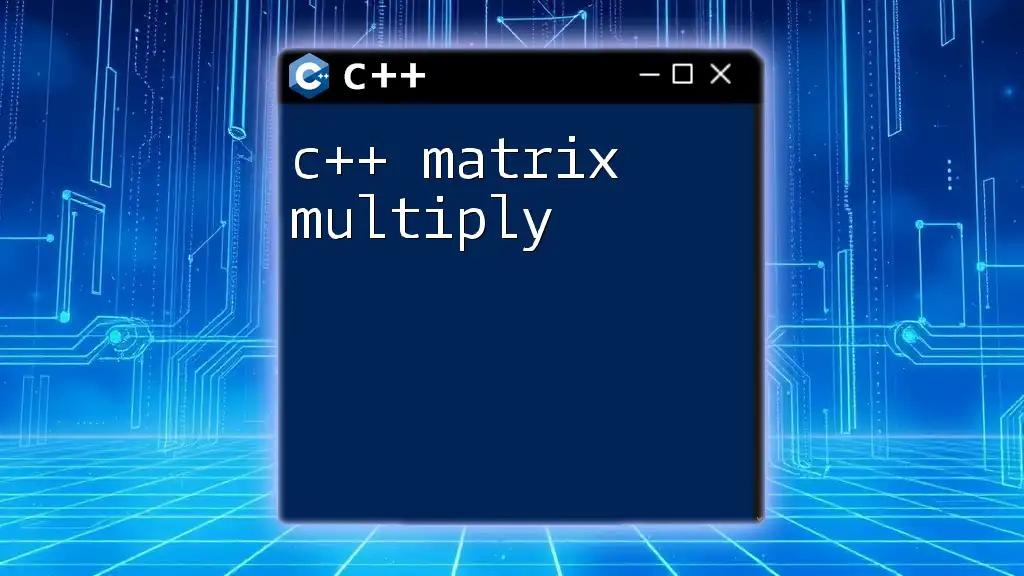
Evaluating Matrix Inversion Implementations
Efficiency and Complexity
The time complexity varies across methods, with Gaussian elimination generally taking \( O(n^3) \), the adjoint method being less efficient at \( O(n!) \), and LU decomposition also about \( O(n^3) \). Understanding the complexity helps in deciding the appropriate method based on your context.
When to Use Each Method
- Gaussian elimination is often the go-to for its versatility and straightforward implementation.
- The adjoint method is best suited for small matrices due to its complexity.
- Use LU decomposition when working with larger matrices and especially when dealing with systems of equations multiple times.
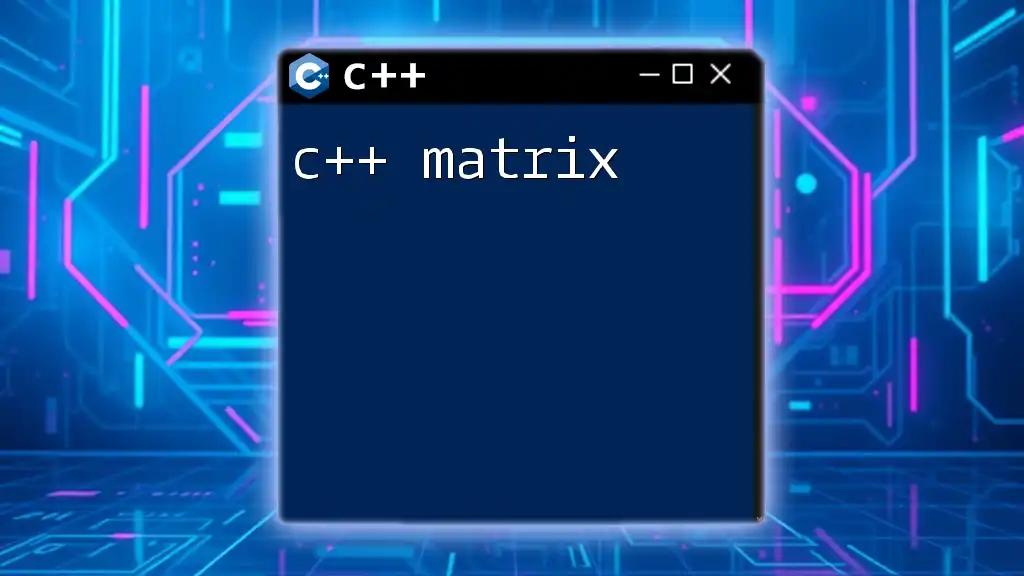
Practical Applications of Matrix Inversion
Role in Solving Linear Equations
Matrix inversion can conveniently solve systems of linear equations. For example, if you have equations represented in matrix form \( Ax = b \), finding the inverse \( A^{-1} \) allows you to directly compute \( x \) by evaluating \( x = A^{-1}b \).
Applications in Computer Graphics
In computer graphics, transformations such as translations, rotations, and scaling are represented by matrices. The ability to invert these matrices is crucial for reversing transformations, allowing for advanced graphical operations like camera movement and perspective adjustments.
Applications in Machine Learning
Many machine learning algorithms, such as linear regression, rely on matrix inversion for optimization. Here, matrices represent data points and parameters; thus, finding the inverse helps in computations related to predictions and model fitting.
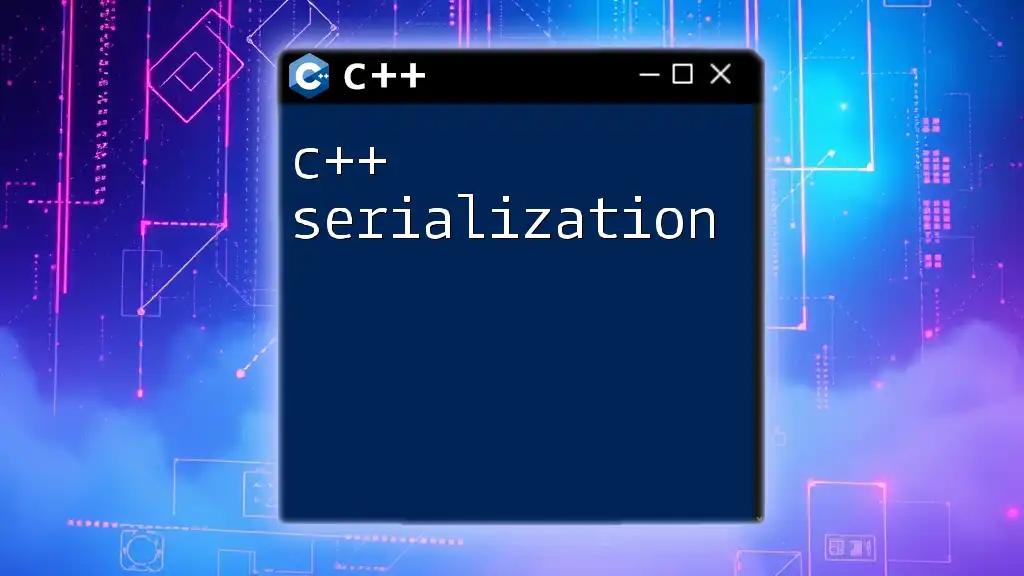
Common Mistakes and Troubleshooting
Common Errors in Implementation
When implementing C++ matrix inversion, developers often run into issues like neglecting to check for the singularity of a matrix or mishandling floating-point precision. Such oversights can lead to runtime errors or incorrect results.
Debugging Tips
- Check Determinants: Always compute the determinant before attempting to find an inverse.
- Use Validation: Verify the inverse by multiplying it with the original matrix to see if you obtain the identity matrix.
- Test with Small Matrices: Start with smaller matrices to isolate problems in your implementation before applying your code to larger datasets.
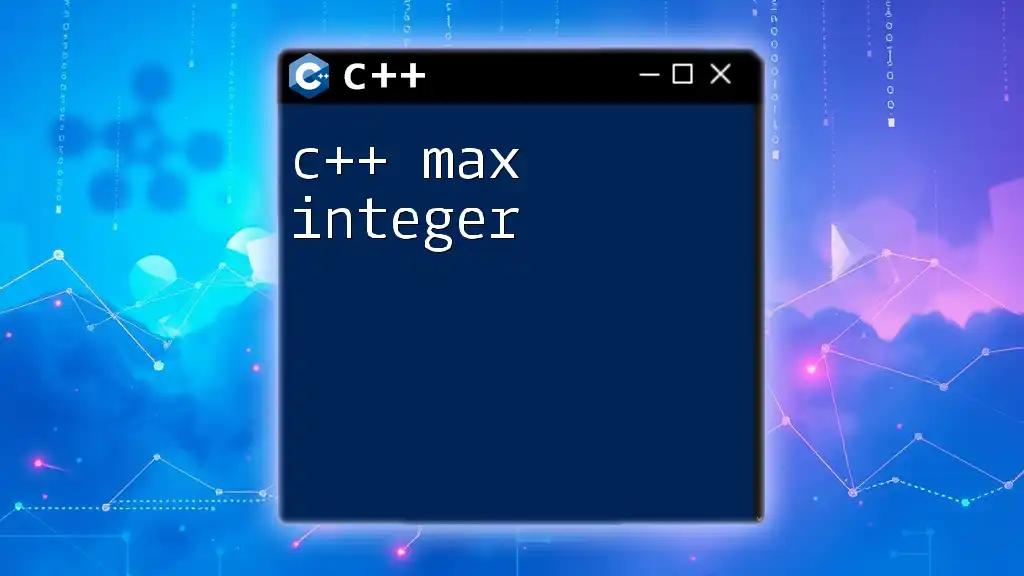
Conclusion
Understanding C++ matrix inversion is not just vital for solving mathematical problems but also plays a key role in various applications in computer science, physics, and engineering. By practicing the techniques outlined in this guide and exploring their numerous applications, you can empower yourself in both coding and theoretical scenarios.
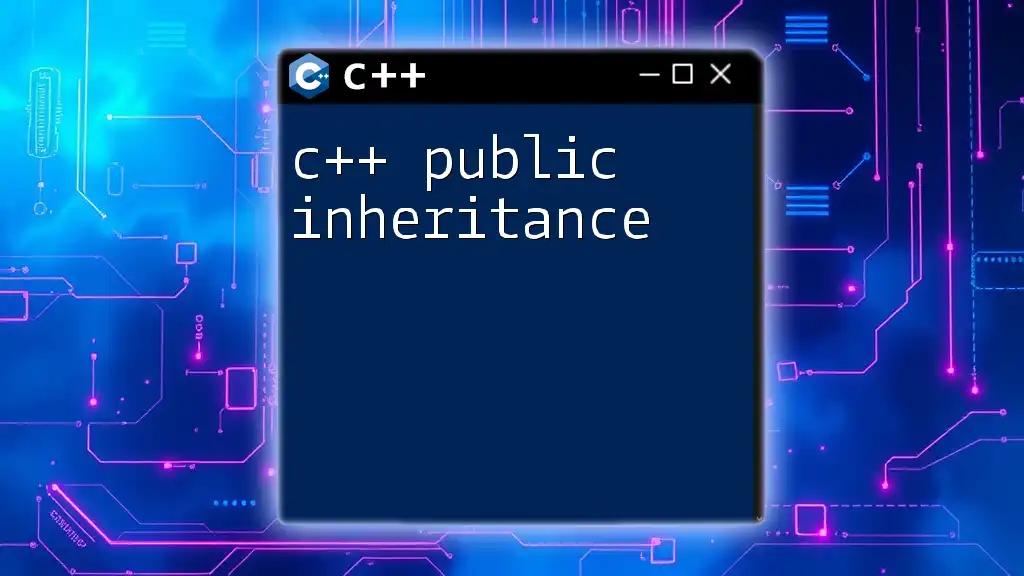
Additional Resources
Recommended Readings
Consider diving deeper into matrix algebra through essential textbooks and academic articles. Exploring these materials will enhance your foundational knowledge and expertise in C++ matrix inversion.
Online Resources and Tutorials
Many online platforms offer free tutorials and courses on both C++ and matrix operations. These resources can provide interactive learning experiences and help solidify your understanding of the subject.