A C++ matrix class is a user-defined class that encapsulates matrix operations such as addition, multiplication, and element access for convenient manipulation of 2D arrays.
Here’s a simple implementation of a C++ matrix class:
#include <iostream>
#include <vector>
class Matrix {
public:
Matrix(int rows, int cols) : data(rows, std::vector<int>(cols)) {}
void set(int row, int col, int value) { data[row][col] = value; }
int get(int row, int col) const { return data[row][col]; }
void display() const {
for (const auto& row : data) {
for (int val : row) {
std::cout << val << " ";
}
std::cout << std::endl;
}
}
private:
std::vector<std::vector<int>> data;
};
int main() {
Matrix mat(2, 2);
mat.set(0, 0, 1);
mat.set(0, 1, 2);
mat.set(1, 0, 3);
mat.set(1, 1, 4);
mat.display();
return 0;
}
Understanding Matrices
What is a Matrix?
A matrix is a rectangular array of numbers arranged in rows and columns. In programming, matrices serve various purposes, including representing data structures for images, graphs, and mathematical computations. Matrices are fundamental tools in linear algebra, computer graphics, and machine learning, making a C++ matrix class invaluable for developers.
Types of Matrices
Matrices can come in different forms, each with specific characteristics:
Row Matrix: A matrix with a single row, e.g., \([a_1, a_2, a_3]\).
Column Matrix: A matrix with a single column, e.g., \(\begin{pmatrix}a_1 \\ a_2 \\ a_3\end{pmatrix}\).
Square Matrix: A matrix with an equal number of rows and columns, such as:
\begin{pmatrix}
a_{11} & a_{12} \\
a_{21} & a_{22}
\end{pmatrix}
Rectangular Matrix: A matrix where the number of rows and columns differ.
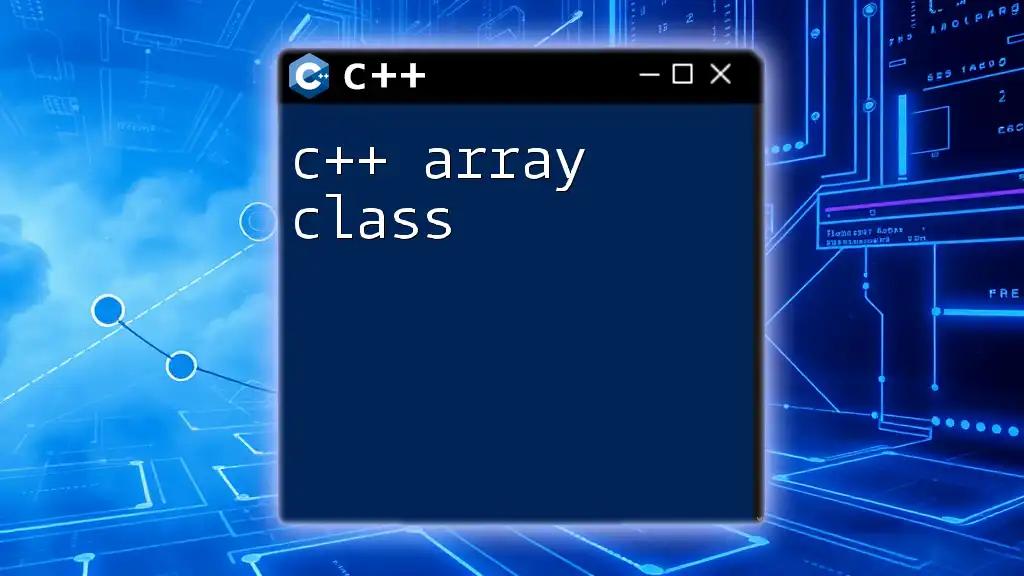
Designing a Matrix Class in C++
Class Structure
Creating a C++ matrix class involves defining its structure to facilitate matrix operations. The class primarily contains the number of rows and columns, along with a pointer to a 2D array, which holds the matrix data.
Consider the following basic structure:
class Matrix {
private:
int rows; // number of rows
int cols; // number of columns
double** data; // Pointer to a 2D array for matrix data
public:
Matrix(int r, int c); // Constructor
~Matrix(); // Destructor
// Other member functions to be defined...
};
Constructor and Destructor
The constructor is fundamental for initializing a new matrix object, allocating memory for the matrix data, and setting the number of rows and columns. Here's how the constructor can be implemented:
Matrix::Matrix(int r, int c) : rows(r), cols(c) {
data = new double*[rows];
for (int i = 0; i < rows; ++i) {
data[i] = new double[cols]; // Allocating memory for each row
}
}
The destructor ensures that allocated memory is properly released when the matrix object is no longer needed, preventing memory leaks:
Matrix::~Matrix() {
for (int i = 0; i < rows; ++i) {
delete[] data[i]; // Deallocating memory for each row
}
delete[] data; // Deallocating memory for the array of rows
}
Additional Member Functions
Set and Get Functions
To access and manipulate matrix elements, setter and getter functions are essential. Setters configure specific elements, while getters retrieve their values:
void Matrix::set(int r, int c, double value) {
if (r >= 0 && r < rows && c >= 0 && c < cols) {
data[r][c] = value; // Setting the value at specified location
}
}
double Matrix::get(int r, int c) const {
if (r >= 0 && r < rows && c >= 0 && c < cols) {
return data[r][c]; // Returning the value from specified location
}
return 0.0; // Return 0.0 for invalid indices
}
Display Function
A display function simplifies visual verification of the matrix contents. By iterating through rows and columns, this implementation visually presents the matrix:
void Matrix::display() const {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
std::cout << data[i][j] << " "; // Printing each element
}
std::cout << std::endl; // New line after each row
}
}
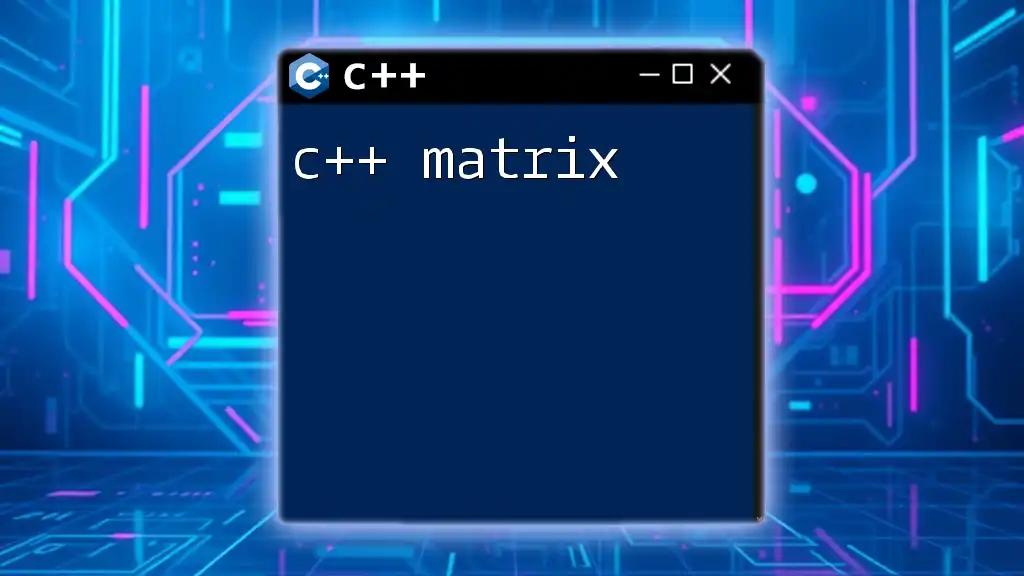
Matrix Operations
Addition of Matrices
Matrix addition occurs when two matrices of identical dimensions are combined. The resulting matrix contains the sum of corresponding elements. The operator overloading approach simplifies this operation:
Matrix Matrix::operator+(const Matrix& other) {
assert(rows == other.rows && cols == other.cols); // Ensuring size compatibility
Matrix result(rows, cols); // Create a new matrix for the result
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.set(i, j, this->get(i, j) + other.get(i, j)); // Element-wise addition
}
}
return result; // Returning the resulting matrix
}
Subtraction of Matrices
Similar to addition, subtraction requires matrices of matching sizes. Here's how you might implement subtraction:
Matrix Matrix::operator-(const Matrix& other) {
assert(rows == other.rows && cols == other.cols);
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.set(i, j, this->get(i, j) - other.get(i, j)); // Element-wise subtraction
}
}
return result; // Returning the resulting matrix
}
Multiplication of Matrices
Matrix multiplication follows specific rules; namely, the number of columns in the first matrix must match the number of rows in the second. Here’s an efficient way to implement it:
Matrix Matrix::operator*(const Matrix& other) {
assert(cols == other.rows);
Matrix result(rows, other.cols); // Resultant matrix
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < other.cols; ++j) {
double sum = 0.0;
for (int k = 0; k < cols; ++k) {
sum += this->get(i, k) * other.get(k, j); // Multiplying and summing
}
result.set(i, j, sum); // Storing result
}
}
return result; // Returning the resulting matrix
}
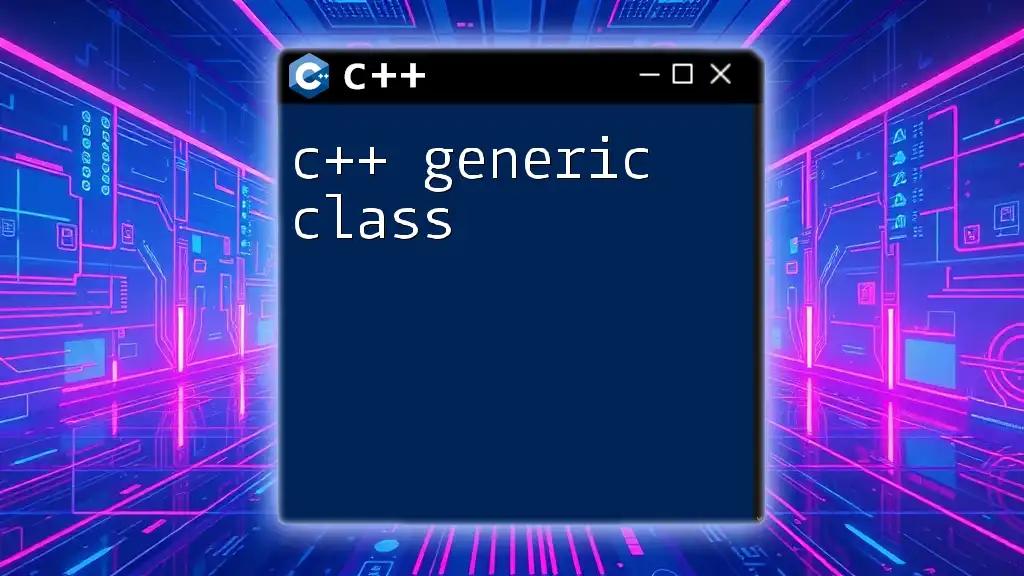
Advanced Matrix Operations
Transpose of a Matrix
Transposing a matrix involves flipping it over its diagonal; this means rows become columns and vice versa. Here’s how to implement it:
Matrix Matrix::transpose() const {
Matrix transposed(cols, rows); // New dimensions
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
transposed.set(j, i, this->get(i, j)); // Switching row and column indices
}
}
return transposed; // Returning the transposed matrix
}
Determinant and Inverse of a Matrix
The determinant expresses certain numerical properties of a matrix, crucial for solving linear equations and understanding matrix behavior. Here's a basic implementation for 2x2 matrices:
double Matrix::determinant() const {
assert(rows == 2 && cols == 2); // Assuming 2x2 matrix
return (data[0][0] * data[1][1]) - (data[0][1] * data[1][0]); // Cross-multiplication
}
The inverse exists only for square matrices and reveals a different set of properties—implementing it generally requires advanced techniques and considerations regarding singularity.

Example Usage
Creating and Using a Matrix
Let’s put everything together and showcase how to create and manipulate a matrix object. The following code snippet demonstrates initializing a matrix and setting values:
int main() {
Matrix m1(2, 2); // Creating a 2x2 matrix
m1.set(0, 0, 1.0);
m1.set(0, 1, 2.0);
m1.set(1, 0, 3.0);
m1.set(1, 1, 4.0);
m1.display(); // Display the matrix
}
Performing Matrix Operations
You can perform operations seamlessly using the overloaded operators. Here’s an example of adding two matrices:
Matrix m2(2, 2);
m2.set(0, 0, 5.0);
m2.set(0, 1, 6.0);
m2.set(1, 0, 7.0);
m2.set(1, 1, 8.0);
Matrix sum = m1 + m2; // Using the overloaded addition operator
sum.display(); // Display the result of the addition
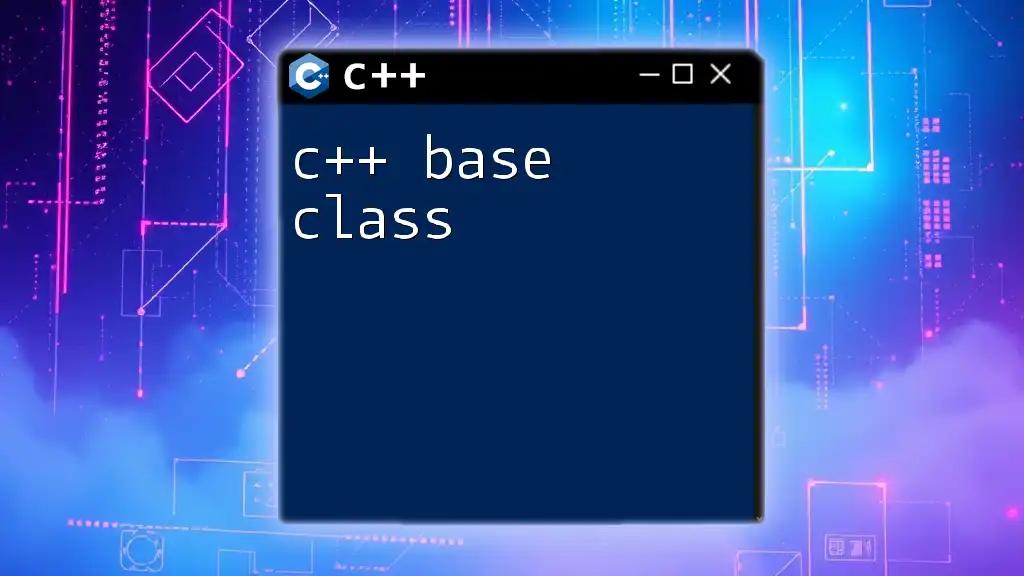
Conclusion
By implementing a C++ matrix class, programmers can effortlessly manage and manipulate matrix data structures. This knowledge not only facilitates numerical computing but also opens up pathways to more complex algorithms involving linear algebra, computer graphics, and data analysis. Experimenting further with the matrix class leads to enhanced understanding and proficiency in C++ programming. Dive deeper into developing this class and explore adding functionalities like eigenvalues, eigenvectors, and various decomposition techniques for advanced applications.
References and Further Reading
For more insights on linear algebra and its applications in programming, consider exploring introductory books, interactive courses on C++, and online resources that reinforce matrix operations and advanced concepts.