A `weak_ptr` in C++ is a smart pointer that holds a non-owning reference to an object managed by a `shared_ptr`, preventing circular references and allowing the object to be deleted even if there are still `weak_ptr` instances referencing it.
Here’s a simple example:
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> sharedPtr = std::make_shared<int>(42);
std::weak_ptr<int> weakPtr = sharedPtr;
std::cout << "Shared pointer value: " << *sharedPtr << std::endl;
std::cout << "Weak pointer expired: " << (weakPtr.expired() ? "Yes" : "No") << std::endl;
sharedPtr.reset(); // Resetting the shared pointer
std::cout << "Weak pointer expired: " << (weakPtr.expired() ? "Yes" : "No") << std::endl;
return 0;
}
What is `weak_ptr`?
In C++, `weak_ptr` is a smart pointer that provides a non-owning reference to an object that is managed by a `shared_ptr`. Its main purpose is to prevent memory leaks and dangling pointers, especially in situations where objects reference each other, creating cyclic dependencies.
Unlike `shared_ptr`, which maintains ownership of the allocated memory and contributes to its reference count, `weak_ptr` does not affect the reference count. This makes it an ideal choice when you want to observe an object managed by a `shared_ptr` without extending its lifecycle.

How `weak_ptr` Works
The Concept of Shared Ownership
To understand `weak_ptr`, it’s essential to grasp how `shared_ptr` works. A `shared_ptr` maintains a reference count that keeps track of how many pointers are referring to the same object. When the last `shared_ptr` referencing an object is destroyed or reset, the object's memory is freed.
std::shared_ptr<MyClass> sp1 = std::make_shared<MyClass>();
std::shared_ptr<MyClass> sp2 = sp1; // Both sp1 and sp2 share ownership
However, if both `sp1` and `sp2` were `shared_ptr`, and they were pointing to each other, it would create a cyclic reference, which results in a memory leak because neither can ever reach a count of zero.
Preventing Cyclic References
Cyclic references occur when two or more `shared_ptr` instances reference each other. This situation leads to a scenario where the reference counts never reach zero, and as a result, the memory allocated for both objects is never released.
By using `weak_ptr`, you can create non-owning references to objects within the cycle, thus breaking the cycle and allowing proper cleanup.
class A;
class B {
public:
std::shared_ptr<A> a;
};
class A {
public:
std::weak_ptr<B> b; // Use weak_ptr to prevent circular reference
};
In this example, class A holds a `weak_ptr` to class B, allowing B to be deleted once it is no longer needed without preventing A from also being deleted.
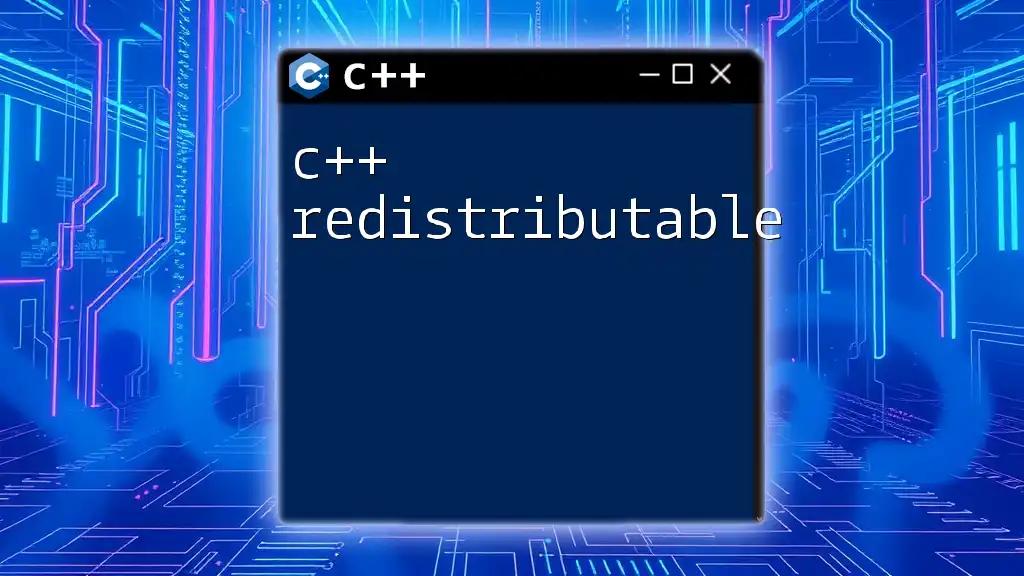
Creating and Using `weak_ptr`
Declaring a `weak_ptr`
To declare a `weak_ptr`, the syntax is straightforward. You create a `weak_ptr` linked to a `shared_ptr` instance:
std::shared_ptr<MyClass> sp = std::make_shared<MyClass>();
std::weak_ptr<MyClass> wp = sp; // Creating a weak_ptr from shared_ptr
Here, the `weak_ptr` `wp` is a non-owning reference, which does not increase the reference count of the `shared_ptr` `sp`.
Locking a `weak_ptr`
To safely access the object managed by a `weak_ptr`, you can use the `lock()` method. This method attempts to obtain a `shared_ptr` from the `weak_ptr`. If the managed object has been destroyed, `lock()` returns an empty `shared_ptr`.
if (auto locked_sp = wp.lock()) {
// Use locked_sp safely
} else {
// The object has been destroyed
}
This mechanism allows you to work safely with the object in a way that respects its lifecycle, avoiding crashes related to dangling pointers.

Common Use Cases for `weak_ptr`
Managing Resource Lifetimes
`weak_ptr` is especially useful when implementing caching mechanisms, where you want to have a non-owning reference to managed objects. If resources are scarce, a `weak_ptr` ensures that data can be released as soon as it is out of scope but gives you a way to access it if it still exists.
Implementing Observer Patterns
One of the classic use cases for `weak_ptr` is in the observer design pattern. When observers (listeners) need to reference subjects, having them hold a `shared_ptr` can lead to cyclic dependencies. By instead having observers hold a `weak_ptr`, they can observe the subject without extending its lifespan.
class Observer {
public:
virtual void update() = 0;
};
class Subject {
private:
std::vector<std::weak_ptr<Observer>> observers;
public:
void addObserver(std::shared_ptr<Observer> observer) {
observers.push_back(observer);
}
void notify() {
for (auto& weak_observer : observers) {
if (auto observer = weak_observer.lock()) { // lock to check ownership
observer->update();
}
}
}
};
In this example, each observer is added as a `weak_ptr`, ensuring that the Subject does not hold onto them longer than necessary.
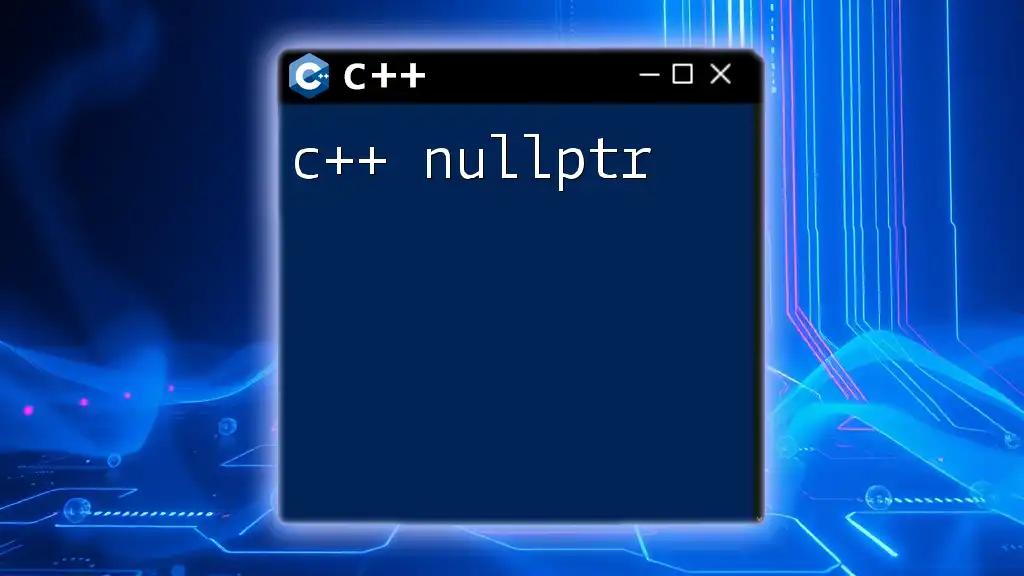
Advantages of Using `weak_ptr`
Using `weak_ptr` comes with significant benefits:
- Memory Management: By avoiding shared ownership, `weak_ptr` helps manage memory more efficiently, particularly in complex systems with multiple interdependencies.
- Avoiding Dangling Pointers: It prevents access to destroyed objects, eliminating the risk of undefined behavior from accessing memory that has already been released.
- Performance Considerations: Since `weak_ptr` does not contribute to the reference count, it slightly reduces the overhead associated with managing `shared_ptr` instances.

Disadvantages and Limitations of `weak_ptr`
Despite the advantages, there are certain disadvantages to consider.
- Complexity in Management: While `weak_ptr` is beneficial, introducing it into code can add complexity. Developers need to ensure that they properly understand when and how to use `weak_ptr` without mismanaging object lifetimes.
- Overheads: Even though `weak_ptr` itself does not maintain ownership, there is still some overhead associated with its reference counting and management features, which might be more than simply using raw pointers in low-level performance scenarios.
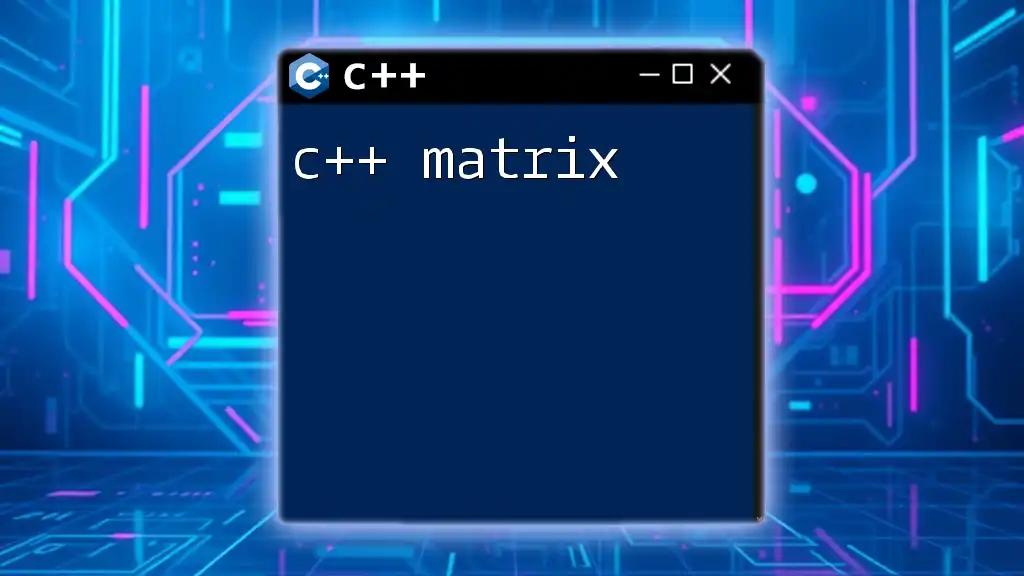
Conclusion
`weak_ptr` plays a crucial role in effective memory management in C++. It provides a mechanism for observing objects without extending their lifetimes unnecessarily, especially in scenarios with multiple interconnected objects. By understanding how to create, lock, and utilize `weak_ptr`, you can significantly improve your code’s robustness and performance while preventing common pitfalls associated with pointer management.
Continue exploring more advanced topics in C++ and embrace the powerful features offered by smart pointers!