A C++ breakpoint is a debugging tool that allows you to pause the execution of your program at a specific line of code, enabling you to inspect variables and control the flow of execution.
Here’s a simple code snippet demonstrating how to set a breakpoint in a C++ program:
#include <iostream>
int main() {
int x = 10; // Set a breakpoint here
int y = 20;
std::cout << "Sum: " << x + y << std::endl;
return 0;
}
What is a Breakpoint?
Definition of Breakpoints
A C++ breakpoint is a crucial debugging feature that allows developers to pause the execution of a program at a specific line of code. This pause enables the examination of the state of the program, including variable values and call stack information at that particular moment. By using breakpoints strategically, developers can isolate issues within their code, understand program flow, and troubleshoot effectively.
Why Use Breakpoints?
Utilizing breakpoints significantly aids in the debugging process:
- Investigate Program Flow: Breakpoints provide insight into how the program executes, enabling developers to verify whether the code behaves as expected.
- Analyze Variable States: By pausing execution, developers can inspect the values of variables at critical junctions in the code.
- Simplify Debugging: Breakpoints reduce the need for scattered print statements throughout the code, creating a cleaner debugging experience.
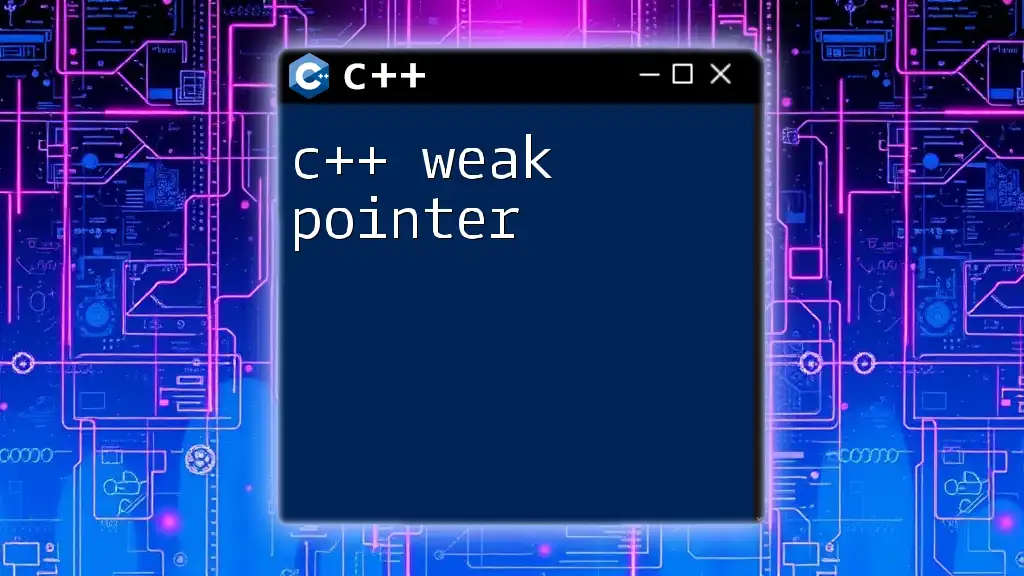
Types of Breakpoints
Standard Breakpoints
Standard breakpoints are the most common type. They halt the execution of the code at a specified line. For instance, consider the following C++ code:
#include <iostream>
void exampleFunction() {
int sum = 0;
for (int i = 0; i < 5; i++) {
sum += i; // Set a breakpoint here
}
std::cout << "Sum is: " << sum << std::endl;
}
int main() {
exampleFunction();
return 0;
}
When a standard breakpoint is set at the line `sum += i;`, execution will pause every time it reaches that line, allowing developers to observe changes in the variable `sum` as the loop iterates.
Conditional Breakpoints
Conditional breakpoints are advanced breakpoints that only trigger when certain conditions are met. This is particularly useful for focusing on specific scenarios without interrupting every execution instance.
For example, to only pause execution when `i` reaches 3 in the previous example, the conditional expression may look like this:
sum += i; // Breakpoint: pause when i == 3
Temporary Breakpoints
Temporary breakpoints act similarly to standard breakpoints but automatically disable themselves after being triggered for the first time. This is useful when you're interested in examining a particular instance without cluttering the breakpoint management.
For example, if a loop runs five times, and you want to inspect the behavior only for the third iteration, you can set a temporary breakpoint for that condition.
Function Breakpoints
Function breakpoints pause execution when entering or exiting specified functions. This can be particularly powerful for monitoring function behavior without forcing breaks within the function body.
Consider the following function:
void sampleFunction(int value) {
std::cout << "Value received: " << value << std::endl;
}
Setting a breakpoint on `sampleFunction` will halt execution every time this function is called, offering visibility into input values and function flow.
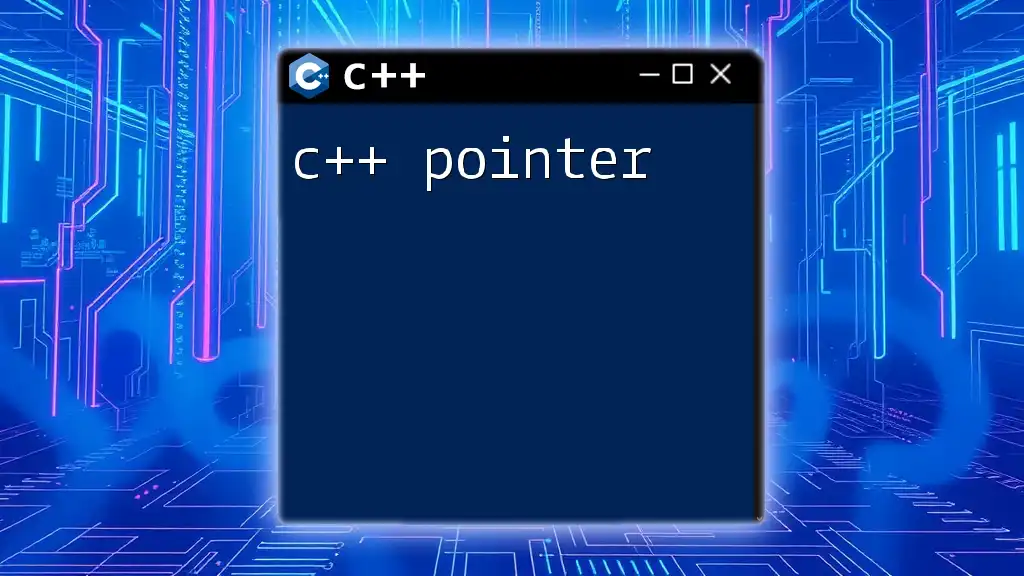
Using Breakpoints in Different Environments
C++ IDEs
Many C++ IDEs come equipped with robust debugging tools that support breakpoints. Popular IDEs include Visual Studio and CLion.
Visual Studio: To set breakpoints in Visual Studio, one can simply click in the margin next to the line of code or press `F9`. Managing breakpoints is also straightforward – the Breakpoints window allows developers to enable, disable, or remove breakpoints easily.
CLion: In CLion, setting breakpoints is similar. Clicking on the left gutter next to the line of code will place a breakpoint. The debugger pane displays all active breakpoints, making it easy to manage them during the debugging session.
Command-line Debuggers
When working with command-line tools such as `gdb` (GNU Debugger), breakpoints can be set using commands.
Setting up a breakpoint in gdb:
gdb ./your_program
(gdb) break exampleFunction
(gdb) run
Conditional breakpoints in gdb:
You can also set conditional breakpoints with a command like:
(gdb) break exampleFunction if value == 3
This command pauses execution only when `value` equals 3.
Cross-Platform Debugging
Debugging can be challenging when transitioning between different platforms. Using tools such as CMake can help manage project configurations and facilitate breakpoint handling across various environments. This ensures a smoother debugging experience regardless of the platform.
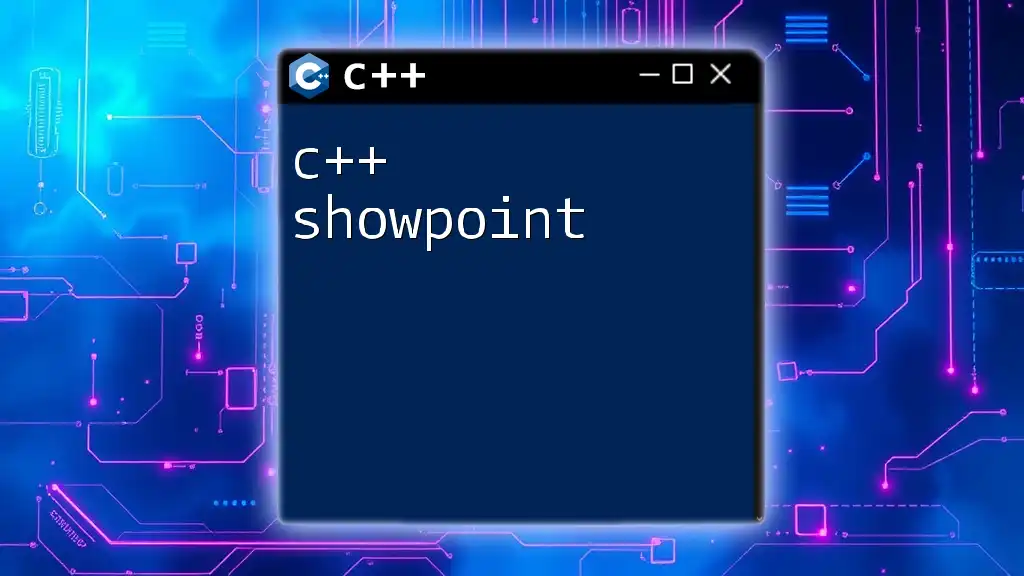
Advanced Breakpoint Techniques
Monitoring Variable Values
Setting breakpoints allows for the tracking of variable states as the program executes. By placing a breakpoint on a specific line, developers can monitor how a variable—such as a loop counter—changes with each iteration:
for (int j = 0; j < 10; j++) {
// Set a breakpoint here to inspect 'j'
}
Using Watchpoints
Watchpoints are specialized breakpoints that trigger halting whenever a specified memory location changes. This is particularly useful for monitoring unintended side effects in code.
To set a watchpoint in `gdb`, you could use the following command:
(gdb) watch variableName
This initiates a watchpoint on `variableName`, stopping execution whenever its value changes, thus providing valuable insights into variable changes under specific conditions.
Incorporating Breakpoints in Unit Testing
Using breakpoints within an automated unit testing framework—like Google Test—can enhance test coverage and quality assurance. By strategically placing breakpoints in test functions, developers can analyze test failures directly, adjusting test parameters or tracked values accordingly.
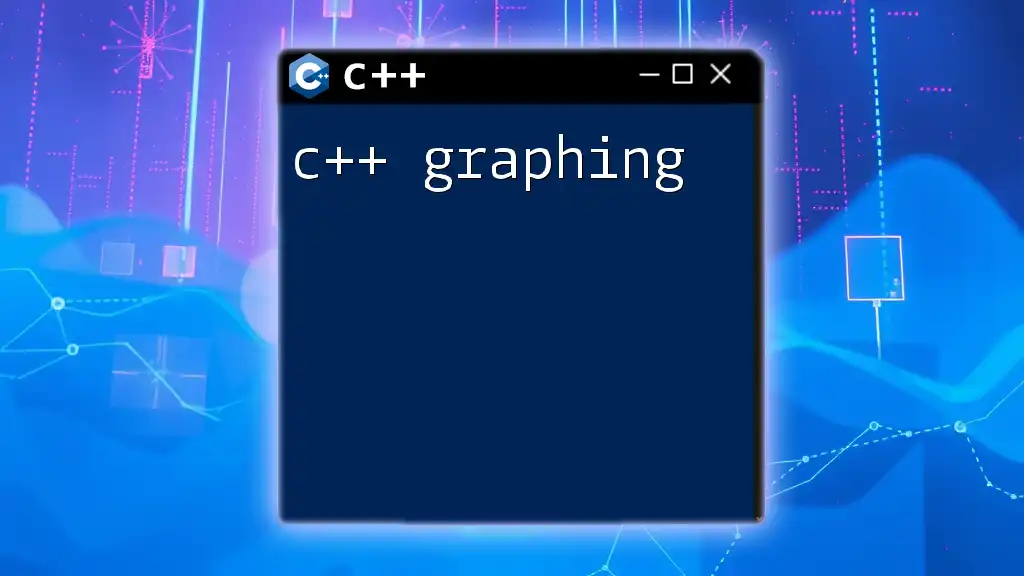
Common Issues and Troubleshooting
Broken Breakpoint Issues
At times, breakpoints may not activate as anticipated. Common culprits include compiler optimizations that strip away debugging information. Sometimes, certain lines of code may never be executed or might be optimized out completely. To resolve this, developers can switch off optimization flags or ensure proper compilation settings, such as using `-O0` for debugging purposes in `g++`.
Understanding Program State
A critical skill in debugging is understanding the program state. Utilizing breakpoints helps developers capture a snapshot of the stack and variables. Understanding how to traverse the call stack or access variable states through the debugger interface is vital for troubleshooting effectively.
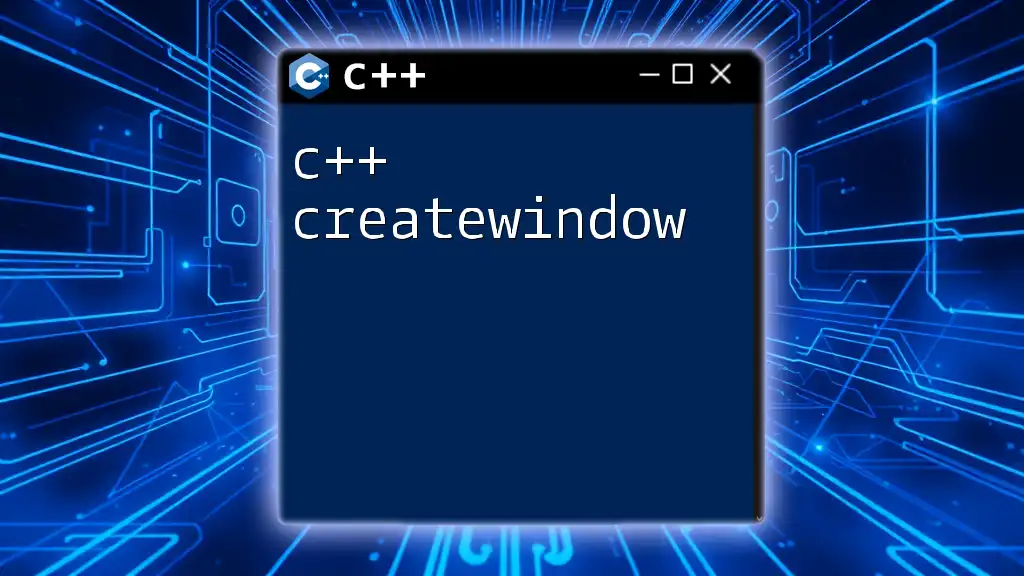
Best Practices for Using Breakpoints
Maintain Readable Code
Writing clean code makes debugging easier and enhances the overall quality of the software. Well-structured code aids in the identification of potential issues, allowing breakpoints to effectively target problem areas.
Limit Overuse of Breakpoints
While breakpoints are powerful debugging tools, excessive use can lead to confusion. Developers should think critically about where and when to set breakpoints, using them judiciously to streamline their debugging process.
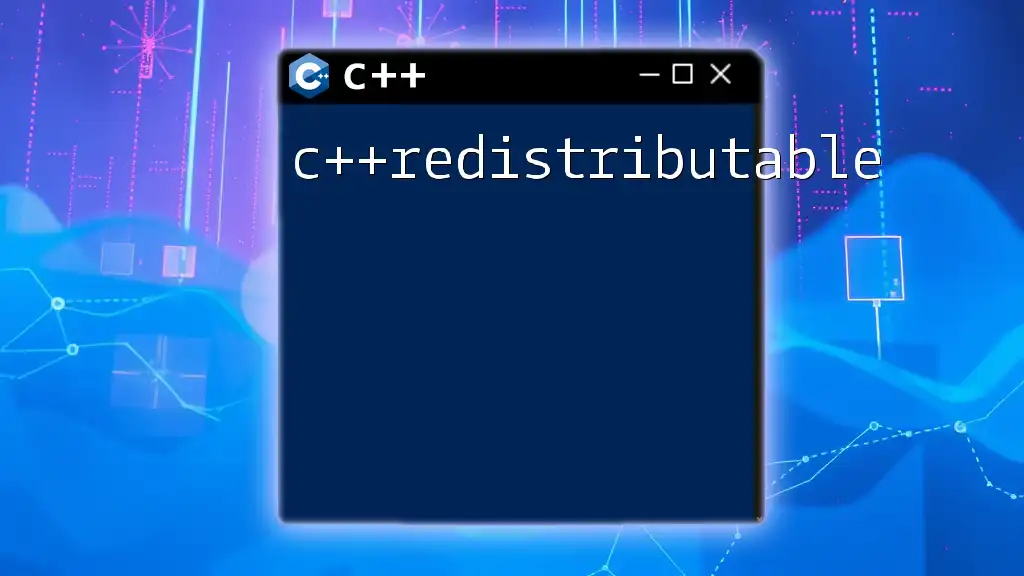
Summary
In this guide, we covered the essential aspects of C++ breakpoints, including their definition, types, and how to utilize them across various environments. Mastering breakpoints is a valuable skill that can significantly enhance your debugging proficiency and overall software development process.
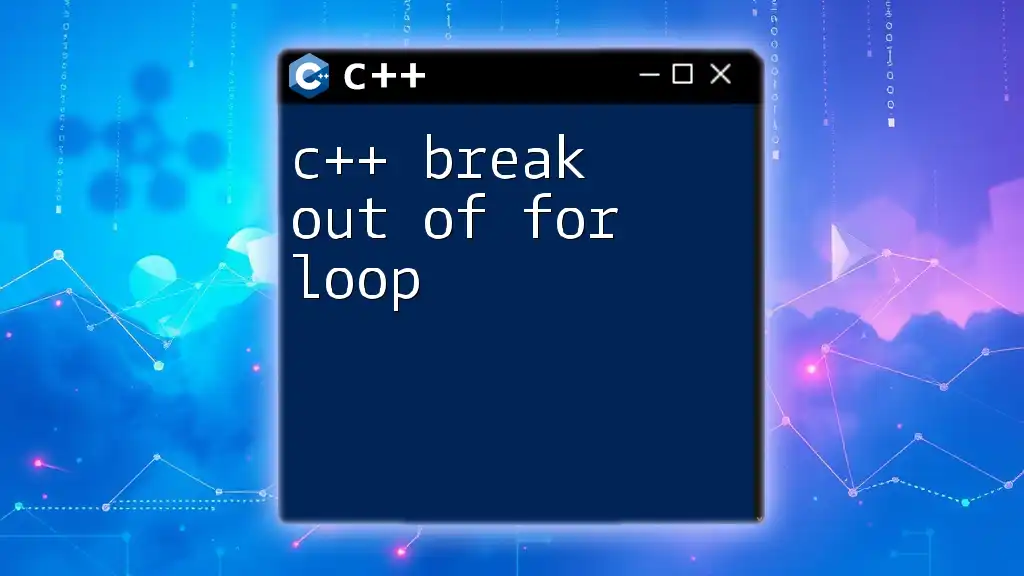
Additional Resources
For further exploration, consider investigating recommended debugging tools that enhance breakpoint capabilities. Numerous online resources and community forums exist to offer support and discuss advanced debugging techniques, providing a collaborative environment for continuous learning.
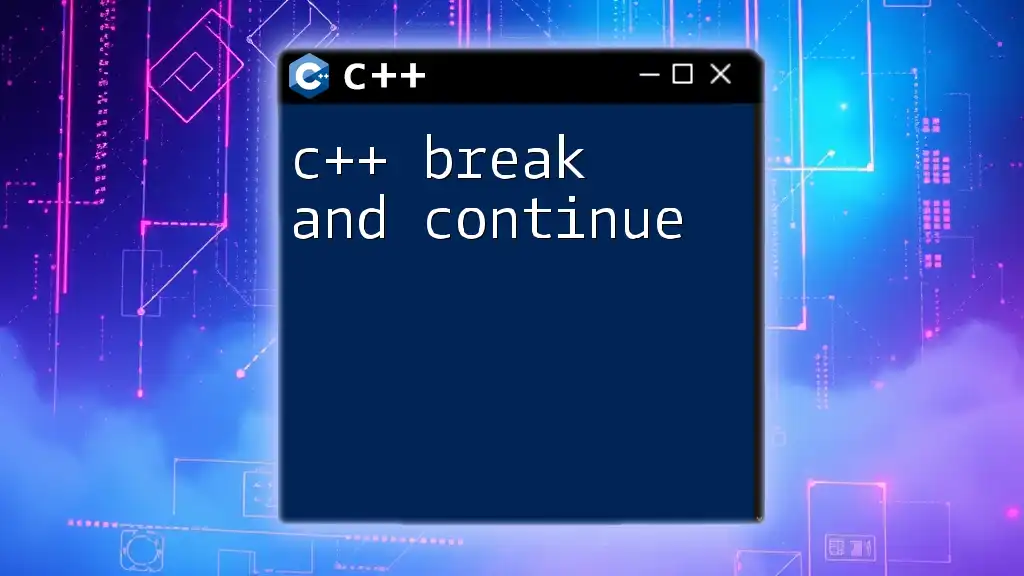
Conclusion
By mastering the use of breakpoints in C++, you equip yourself with an invaluable tool that can enhance the debugging process, improve code quality, and ultimately lead to more efficient software development. Engage with the community and share your breakpoint tips and experiences to enrich the collective knowledge!