To break out of two nested loops in C++, you can use a boolean flag or the `goto` statement for a concise exit point, as shown in the following code snippet:
#include <iostream>
int main() {
bool breakAll = false;
for (int i = 0; i < 5 && !breakAll; ++i) {
for (int j = 0; j < 5; ++j) {
if (i == 2 && j == 2) {
breakAll = true; // set the flag to break both loops
break;
}
std::cout << "i: " << i << ", j: " << j << std::endl;
}
}
return 0;
}
Understanding Loops in C++
What are Loops?
Loops are a fundamental construct in programming that allow for the repeated execution of a block of code as long as a specified condition remains true. In C++, there are several types of loops: for, while, and do-while. Each type has its specific use cases and advantages.
Nested Loops
Nested loops are loops that are placed inside of other loops. They are useful in scenarios where you need to perform more complex iterations, such as traversing multidimensional arrays or implementing backtracking algorithms. Here's a basic structure of nested loops in C++:
for (initialization; condition; increment) {
for (initialization; condition; increment) {
// Inner loop statements
}
// Outer loop statements
}
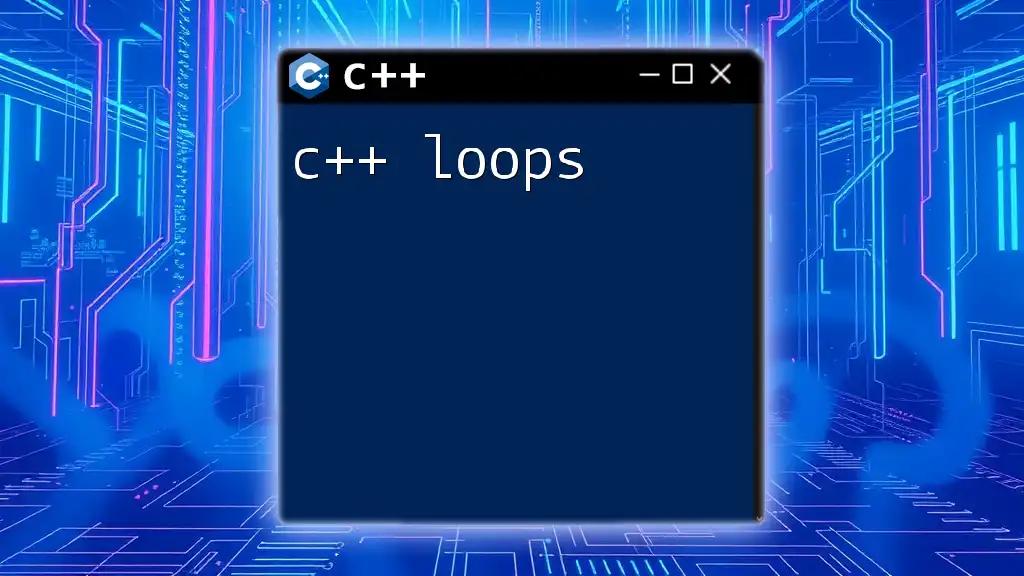
The Break Statement
What is the Break Statement?
The break statement in C++ is used to terminate a loop or switch statement block prematurely. When the break statement is executed, control jumps out of the loop, meaning any subsequent iterations are skipped. This is especially useful when a certain condition is met, and there is no need to continue further execution of the loop.
Syntax of the Break Statement
The basic syntax for using the break statement is simply as follows:
break;
This statement can be placed anywhere within the loop but is most commonly used within conditional statements to effectively terminate the loop based on logical conditions.
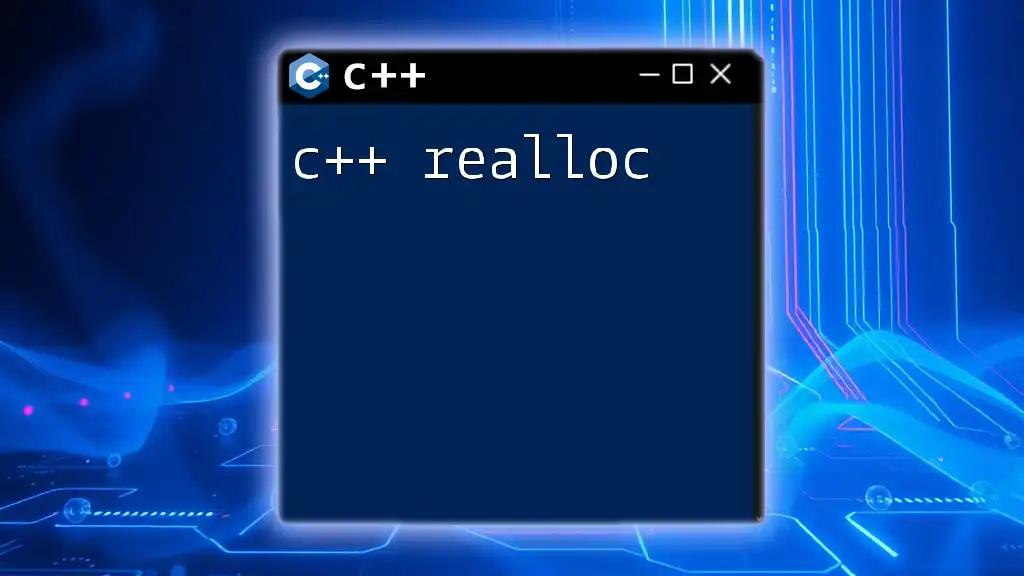
Breaking Out of Two Loops: Techniques and Examples
The Importance of Breaking Two Loops
In programming, there may be scenarios where we need to exit from multiple nested loops based on a particular condition. For example, if processing data in a nested format, we might want to stop processing altogether once a certain condition is met. Understanding how to achieve this is crucial for writing efficient and logical code.
Using a Flag Variable
Explanation of Flag Variables
A flag variable is a simple yet effective method of controlling loop flow. By defining a boolean variable, you can use it to signal when both loops should terminate. Once this flag is set, the outer loop can also check this state and exit accordingly.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
bool breakOuter = false;
for (int i = 0; i < 5 && !breakOuter; ++i) {
for (int j = 0; j < 5; ++j) {
if (/* conditionToBreak */) {
breakOuter = true;
break; // Breaks inner loop
}
}
}
}
In this example, the flag variable `breakOuter` is checked both in the outer loop's condition and is set to `true` when the inner condition is met. This way, the outer loop can be exited without needing to specify any additional logic.
Using Goto Statement (Caution)
What is the Goto Statement?
The goto statement provides a way to jump to another location in the code, which can be useful for breaking out of nested loops. However, its use is generally discouraged due to the potential for creating hard-to-understand code flows.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 5; ++i) {
for (int j = 0; j < 5; ++j) {
if (/* conditionToBreak */) {
goto endLoops; // Jumps out of both loops
}
}
}
endLoops:
cout << "Exited from both loops." << endl;
}
In this code, the goto statement provides a direct way to exit both loops when the specified condition is met. However, bear in mind that while this method works, it can make the code less readable and maintainable, creating what’s known as "spaghetti code."
Using Exception Handling
How Exception Handling Works
Exception handling in C++ is another advanced technique that can be leveraged to break out of multiple loops. By throwing an exception when a certain condition is met, the program can jump out of the nested loops and into a catch block designed to handle the exception.
Example Code Snippet
#include <iostream>
#include <stdexcept>
using namespace std;
int main() {
try {
for (int i = 0; i < 5; ++i) {
for (int j = 0; j < 5; ++j) {
if (/* conditionToBreak */) {
throw runtime_error("Breaking out of both loops");
}
}
}
} catch (const runtime_error& e) {
cout << e.what() << endl; // Handle the exception
}
}
In this example, when the specific condition is reached, a runtime error is thrown, causing the program flow to jump directly to the catch block. This method effectively terminates both loops, demonstrating a clean way to manage complex control flows.
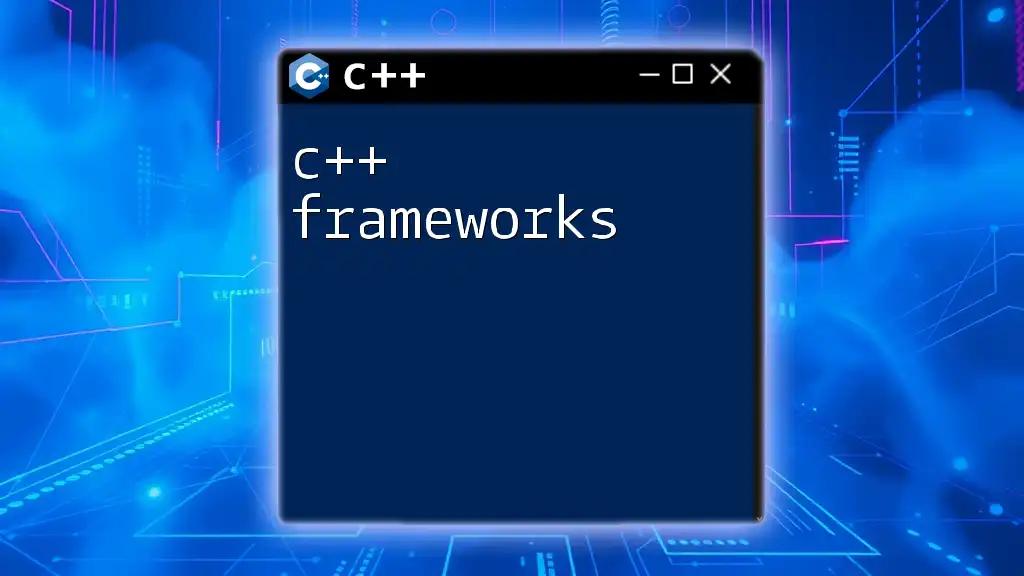
Best Practices for Breaking Loops
Understand the Flow of Control
When implementing any of the methods to break two loops, it is crucial to maintain a clear understanding of the flow of control in your program. This clarity ensures that other developers can read and understand your code without confusion.
Avoid Overusing Breaks
While it might be tempting to frequently use break statements liberally, this can lead to confusing code. Prioritize methods that allow for clear flow, and consider alternatives like returning from functions or restructuring your logic to avoid excessive use of breaks.
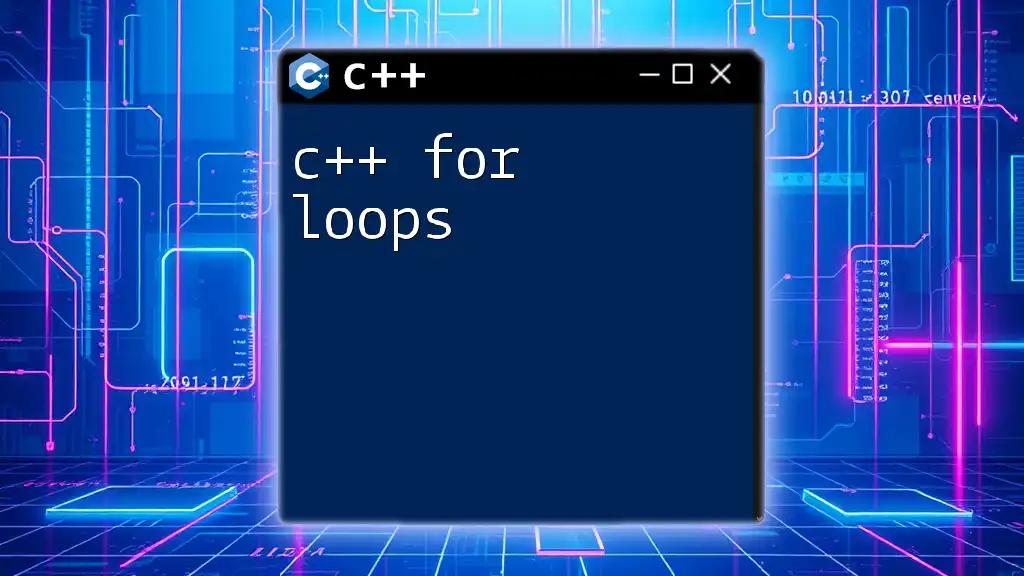
Conclusion
In conclusion, mastering how to use the c++ break two loops technique is an essential skill for any C++ programmer. From using flag variables to the more controversial goto statement and exception handling, programmers have several options to control loop execution effectively. Each method has its use case, strengths, and limitations. By practicing these techniques, you can enhance your coding efficiency, making your programs robust and efficient.
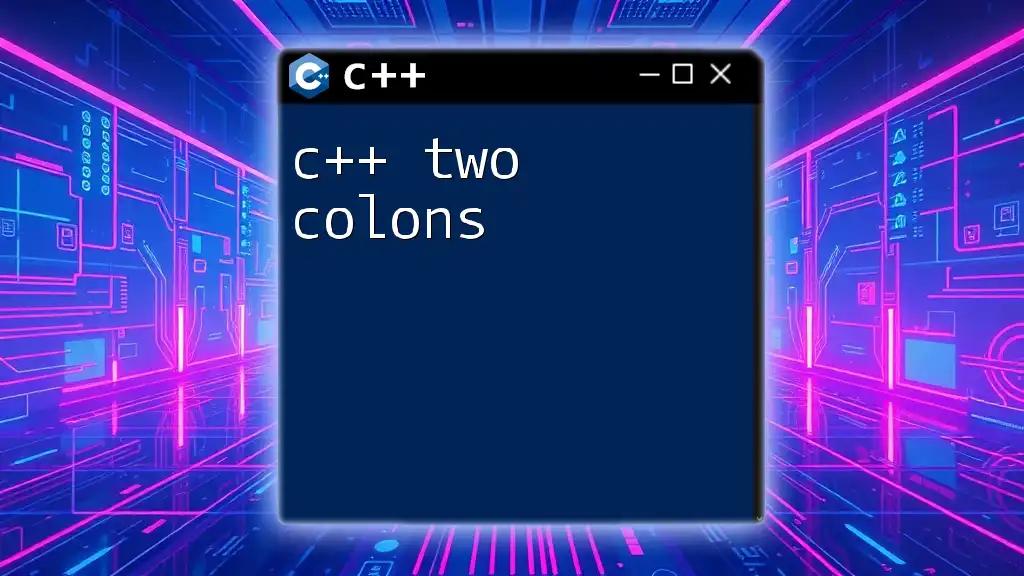
Additional Resources
To deepen your understanding of C++ loops and control flow, consider exploring recommended tutorials, documentation, and online courses that offer comprehensive coverage of these topics. Happy coding!