To exit a `for` loop prematurely in C++, you can use the `break` statement, which immediately terminates the loop when executed.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
std::cout << i << " ";
}
return 0;
}
Understanding the For Loop in C++
Definition and Syntax
A `for` loop in C++ is a control flow statement used to repeat a block of code a certain number of times. It is ideal for situations where the number of iterations is known before the loop starts. The syntax for a `for` loop is:
for (initialization; condition; increment) {
// code block
}
In this structure:
- Initialization: Typically used to declare and initialize the loop variable.
- Condition: A boolean expression that is evaluated before each iteration.
- Increment: Code that updates the loop variable.
Example of a Basic For Loop
Here is a simple example of a for loop iterating through an array:
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
This code will output the elements of the array in order. Each element is accessed using the loop index `i`, starting from 0 and ending at 4.
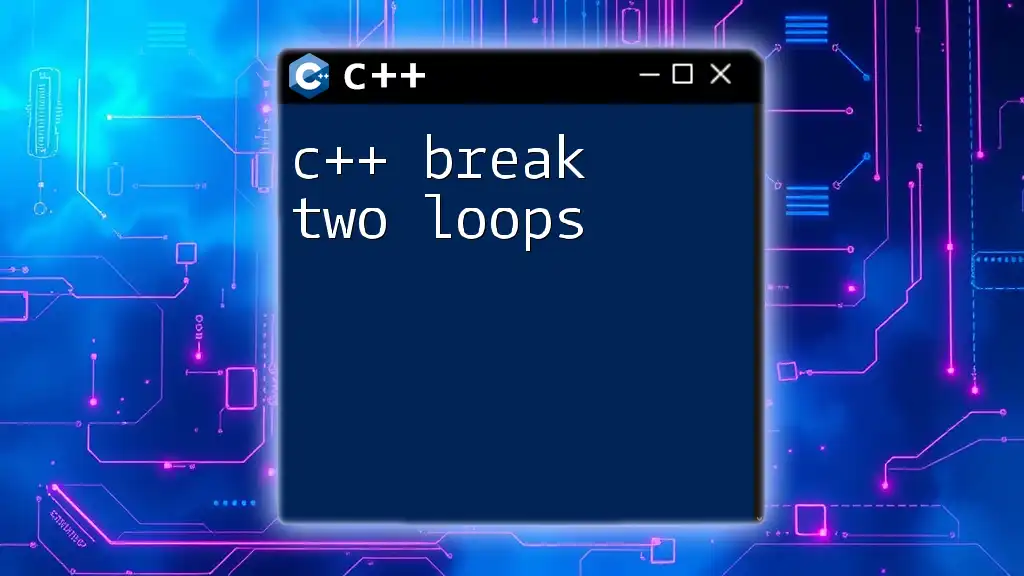
The Break Statement in C++
What is the Break Statement?
The `break` statement is a powerful feature in C++ that allows you to exit a loop prematurely, without having to wait for the loop's termination condition to be met. This is particularly useful when you want to end the loop based on a specific condition that may not be strictly tied to the loop's normal iteration.
Syntax of the Break Statement
The `break` statement is expressed simply as:
break;
When executed within a loop, this statement immediately terminates that loop.
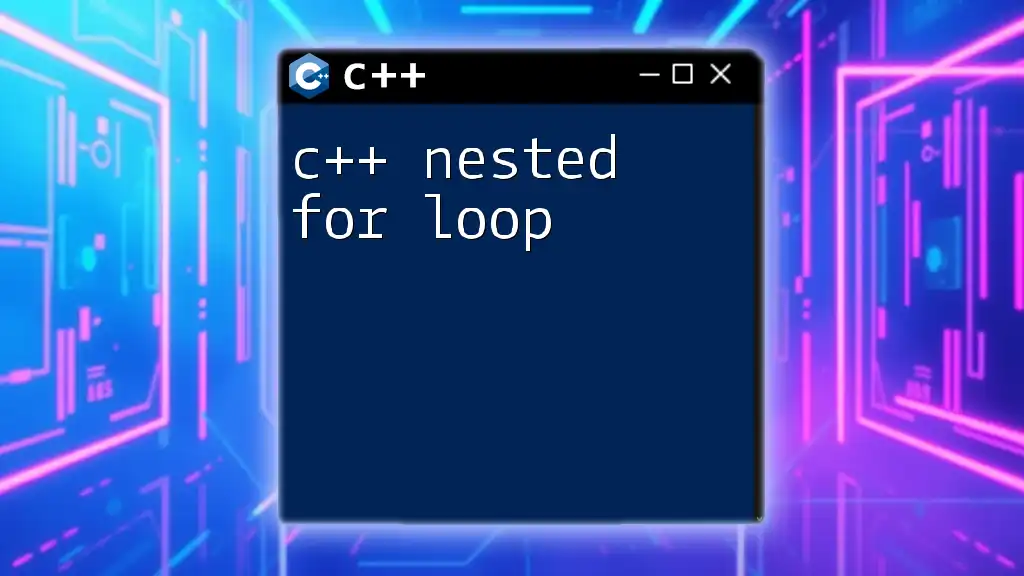
Using Break to Exit a For Loop
How to Break Out of a For Loop
The need to exit a loop prematurely arises in various scenarios, such as when a specific condition is met or when you have found the required result. By using `break`, you ensure that your program can continue executing the subsequent code rather than going through all iterations of the loop.
Here’s an example demonstrating the use of `break` within a for loop:
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // exits the loop when i equals 5
}
std::cout << i << " ";
}
In this code snippet, the loop will print numbers from 0 to 4, and when `i` equals 5, it will exit the loop because of the `break` statement.
Example Scenario: Searching for a Value
A common and practical example of using `break` is during the search for a particular value within an array. Here's how that might look:
int arr[] = {1, 3, 5, 7, 9};
int searchValue = 5;
for (int i = 0; i < 5; i++) {
if (arr[i] == searchValue) {
std::cout << "Found " << searchValue << " at index " << i << std::endl;
break; // Exit once the value is found
}
}
In this example, the for loop iterates through the array, and as soon as it finds the specified `searchValue`, it prints out the index and uses `break` to exit the loop immediately. This prevents unnecessary further iterations.
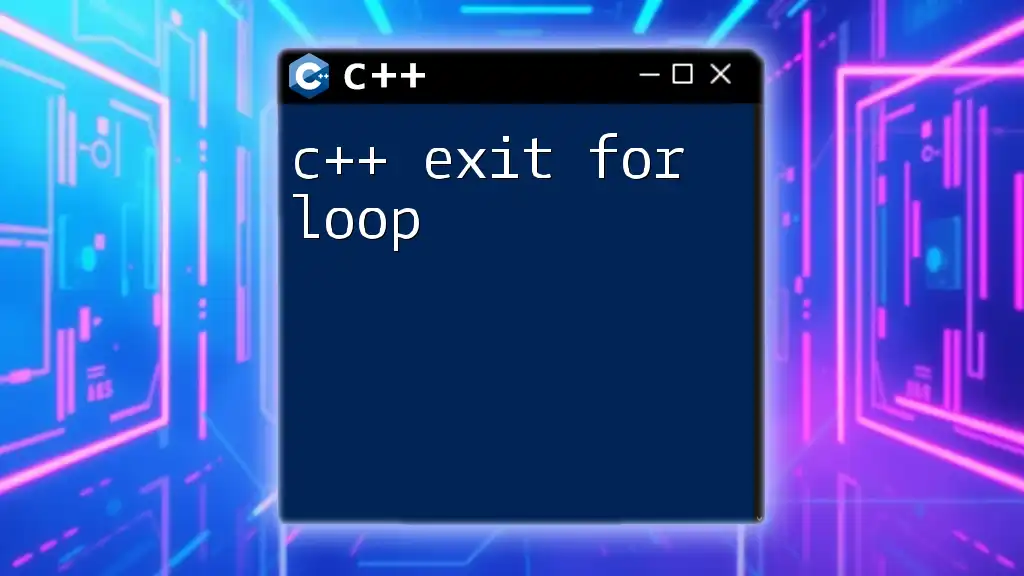
Avoiding Infinite Loops with Break
Understanding Infinite Loops
An infinite loop occurs when a loop continues to execute indefinitely due to a condition that never becomes false. This can lead to program unresponsiveness or crashes. It's essential to manage loop execution effectively to avoid such scenarios.
Example of an Infinite Loop and Using Break
Consider the following example of an infinite loop where we ask the user for input:
while (true) {
// Simulating some condition
int input;
std::cout << "Enter 0 to exit: ";
std::cin >> input;
if (input == 0) {
break; // Exit the loop
}
std::cout << "You entered: " << input << std::endl;
}
In this code, the loop continues to prompt the user until they enter 0. The `break` statement effectively terminates the loop, thus preventing an infinite execution.
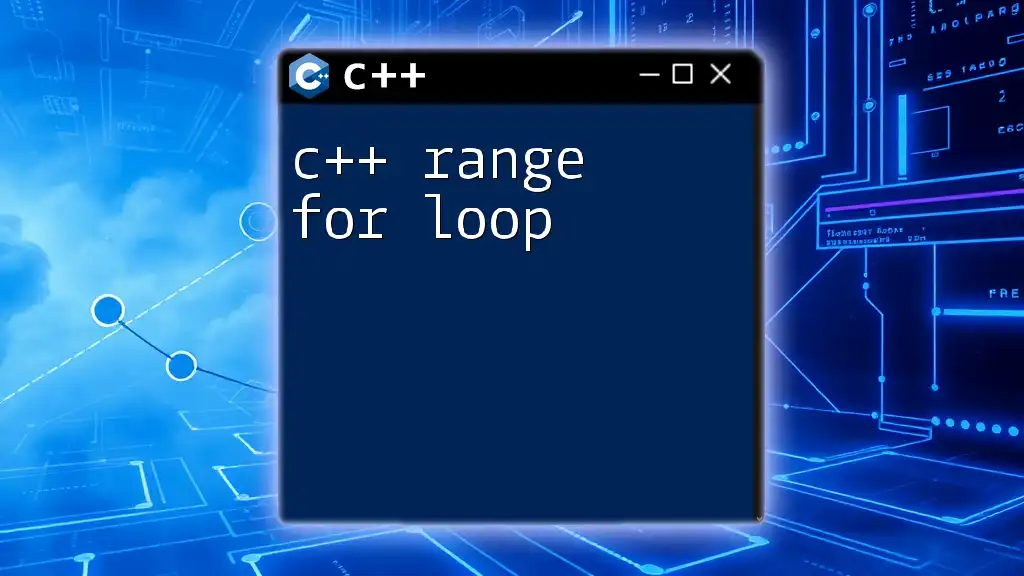
Best Practices for Using Break in For Loops
When to Use Break
Using `break` can make your code more readable and efficient, especially when you are searching through data or waiting for a specific condition that doesn't align directly with the loop's iteration criteria. It provides clarity to your logic by indicating that an exit from the loop was intentional.
When to Avoid Break
While `break` is useful, relying on it too heavily can lead to confusion and complex code structure. It's advisable to use it sparingly and only in cases where it enhances clarity. Instead of using `break`, consider structuring your loops to complete naturally whenever possible.
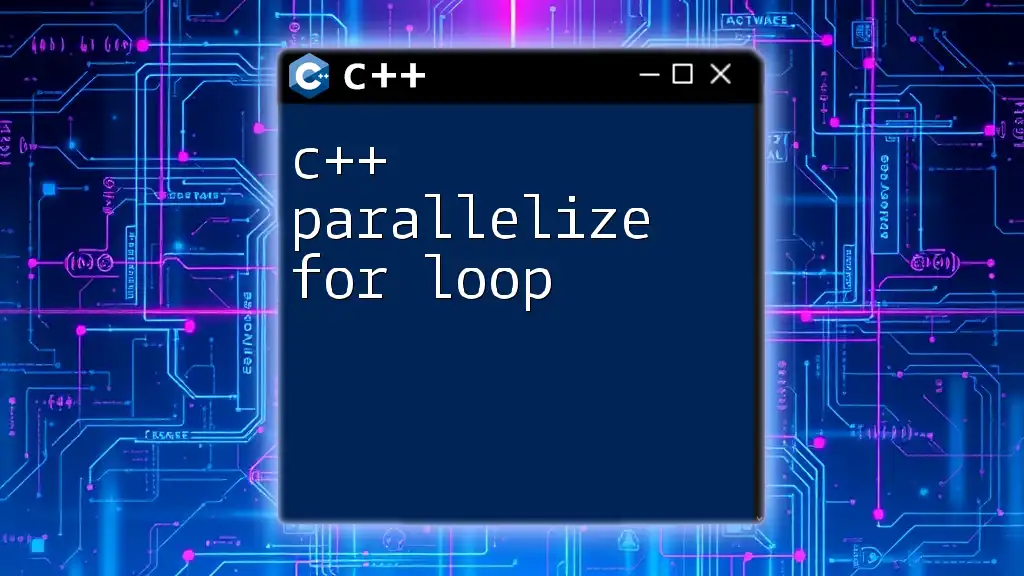
Alternative to Break: Using Return Statements
How to Exit a Loop with Return
Another way to exit from loops, especially in functions, is by using the `return` statement. Unlike `break`, which only exits the loop, `return` will exit the current function entirely.
Example of Return to Exit Loop
Here's a brief example to illustrate this:
void searchAndExit(int* arr, int length, int searchValue) {
for (int i = 0; i < length; i++) {
if (arr[i] == searchValue) {
std::cout << "Found at index: " << i << std::endl;
return; // Exit the function, hence the loop
}
}
std::cout << "Not found." << std::endl;
}
In this case, if the value is found, the function will return, terminating not only the loop but also the function's execution.
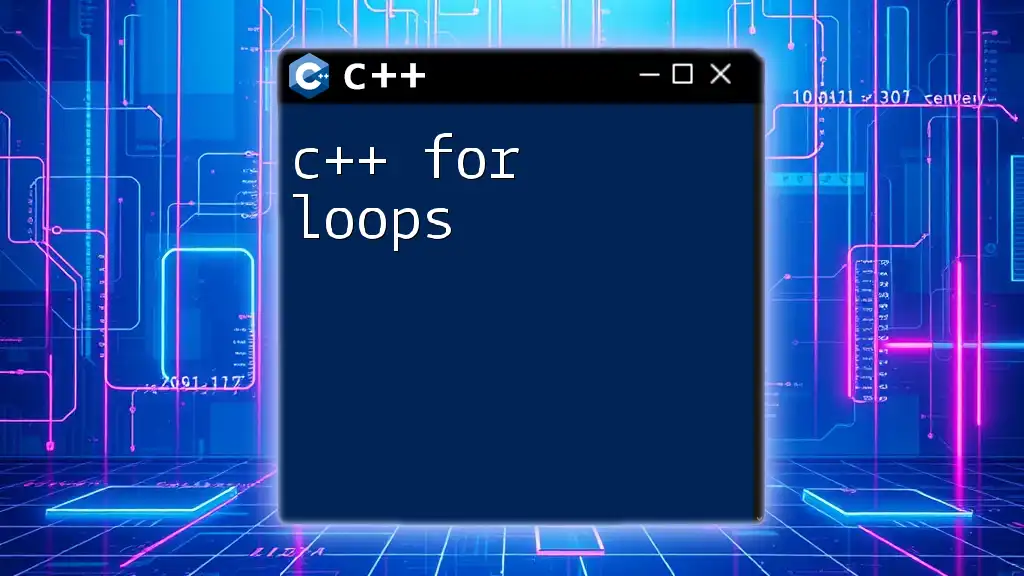
Conclusion
Understanding how to use the `c++ break out of for loop function is essential for effective programming in C++. It provides you with critical control over loop execution, allowing you to escape from iterations when conditions dictate. By practicing the examples and carefully applying these techniques, you can produce cleaner, more efficient code. Don’t hesitate to explore more complex C++ features as you become more comfortable with the basics of control flow!