In C++, the `break` statement terminates the nearest enclosing loop or switch statement, while the `continue` statement skips the rest of the current loop iteration and proceeds to the next iteration.
Here’s an example demonstrating both `break` and `continue` in a loop:
#include <iostream>
int main() {
for (int i = 0; i < 10; ++i) {
if (i == 5) {
break; // terminates the loop when i equals 5
}
if (i % 2 == 0) {
continue; // skips the rest of the loop for even numbers
}
std::cout << i << " "; // only prints odd numbers less than 5
}
return 0;
}
Understanding Control Flow
Control flow is a fundamental concept in programming that dictates the order in which individual statements, instructions, or function calls are executed or evaluated in a program. In C++, control flow is generally directed through various structures, such as loops and conditional statements.
The significance of implementing control flow effectively cannot be overstated, especially when it comes to optimizing your code for better clarity and performance. Among the control flow constructs in C++, the `break` and `continue` statements play essential roles in managing loop execution.
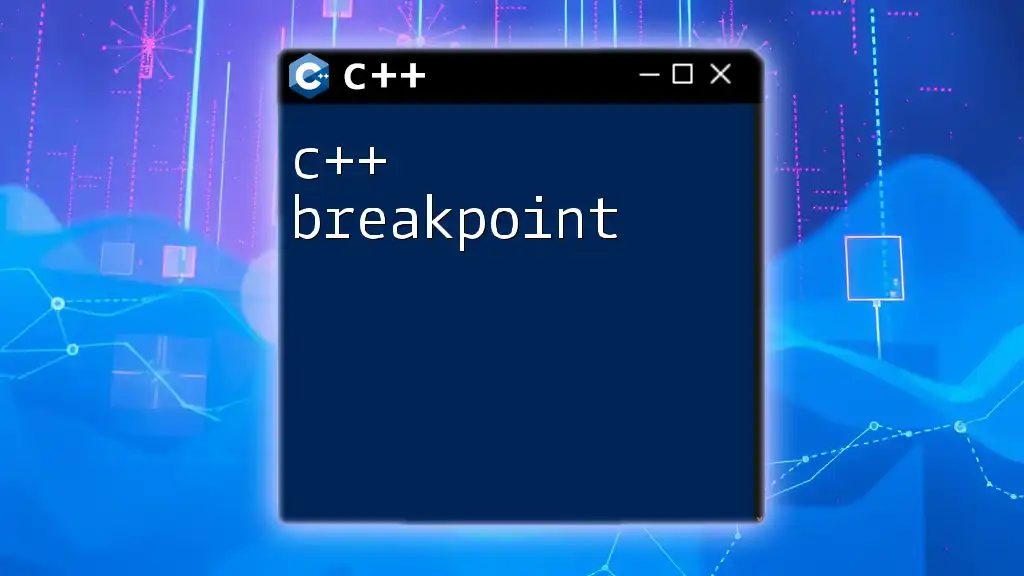
The `break` Statement in C++
What is the `break` Statement?
The `break` statement in C++ serves to exit a loop prematurely. When a `break` statement is encountered, the current loop terminates, and control transfers to the statement immediately following the loop.
Syntax:
break;
How `break` Works in Loops
The `break` statement can be utilized within `for`, `while`, and `do-while` loops. When applied, it can quickly terminate the loop, making it an efficient way to control execution flow.
Example of `break` in Action
Consider the following C++ code:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
cout << i << " ";
}
return 0;
}
In this example, the loop iterates from 0 to 9, but it stops execution once `i` equals 5. The expected output will be:
0 1 2 3 4
Common Use Cases for `break`
- Exiting Loops Based on Conditions: The `break` statement is particularly useful in scenarios where a specific condition is met, and continuing the loop is unnecessary.
- Optimizing Algorithms: Using `break` can enhance performance in searching algorithms where the search can terminate once the desired result is found.
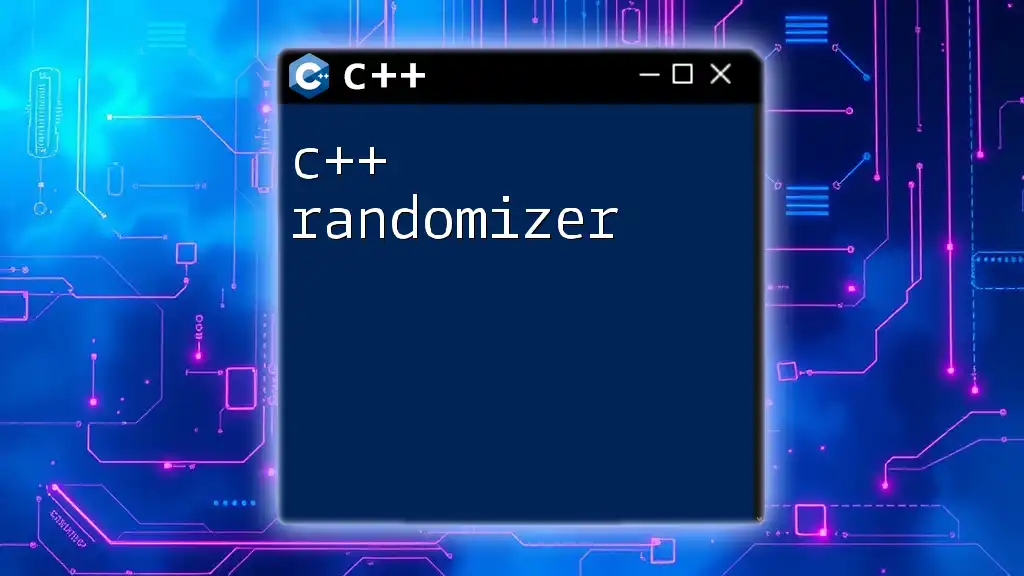
The `continue` Statement in C++
What is the `continue` Statement?
The `continue` statement is used within loops to skip the current iteration and continue to the next iteration immediately. Unlike `break`, which terminates the loop entirely, `continue` merely moves the control to the next cycle of the loop.
Syntax:
continue;
How `continue` Works in Loops
The `continue` statement can also be employed in `for`, `while`, and `do-while` loops. When reached, it skips the remainder of the loop body for the current iteration and proceeds directly to the next iteration.
Example of `continue` in Action
Here’s an example demonstrating the `continue` statement:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
continue;
}
cout << i << " ";
}
return 0;
}
In this code, the loop prints only odd numbers from 0 to 9 by skipping every even number using the `continue` statement. The expected output is:
1 3 5 7 9
Common Use Cases for `continue`
- Skipping Unnecessary Iterations: The `continue` statement is perfectly suited for scenarios where specific conditions are met that indicate the remainder of the loop's logic should be bypassed.
- Handling Specific Conditions: When working through datasets with specific conditions, `continue` allows for focused processing without terminating the entire loop.
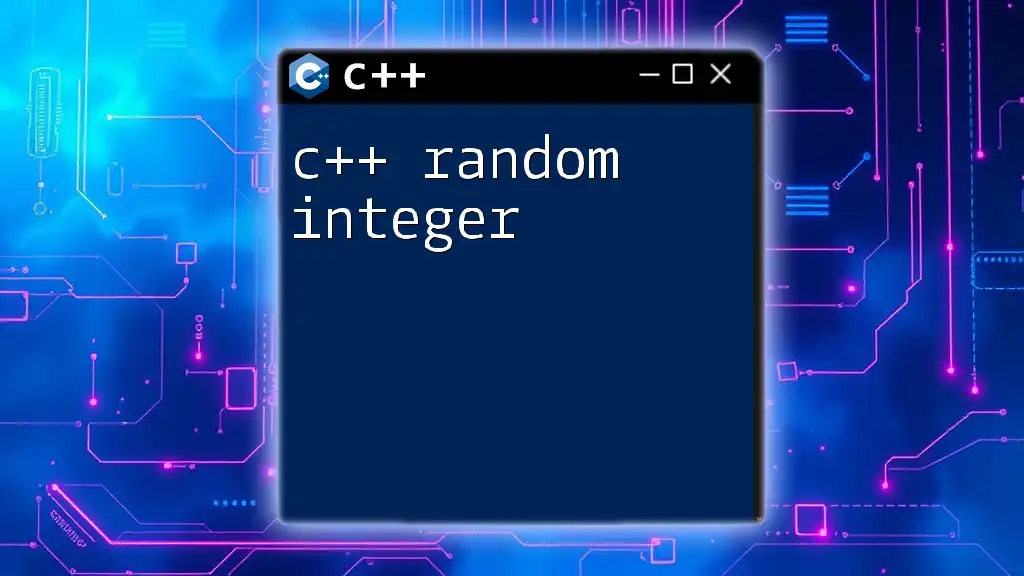
Break and Continue in Nested Loops
How to Use `break` and `continue` in Nested Loops
Nested loops are a common structure in programming where one loop exists inside another. Utilizing `break` and `continue` in nested loops requires understanding that they affect only the closest enclosing loop.
Examples of Nested Loops with `break` and `continue`
Using `break` in Nested Loops
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (i == j) {
break;
}
cout << "(" << i << ", " << j << ")" << endl;
}
}
return 0;
}
In this example, the outer loop iterates three times, and whenever `i` equals `j`, the `break` statement exits only the inner loop. The output will be:
(0, 0)
(1, 0)
(1, 1)
(2, 0)
(2, 1)
Using `continue` in Nested Loops
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (j == 1) {
continue;
}
cout << "(" << i << ", " << j << ")" << endl;
}
}
return 0;
}
In this snippet, the `continue` statement skips the iteration when `j` equals 1, allowing the loop to print pairs while avoiding the column where `j` is 1. The expected output is:
(0, 0)
(0, 2)
(1, 0)
(1, 2)
(2, 0)
(2, 2)
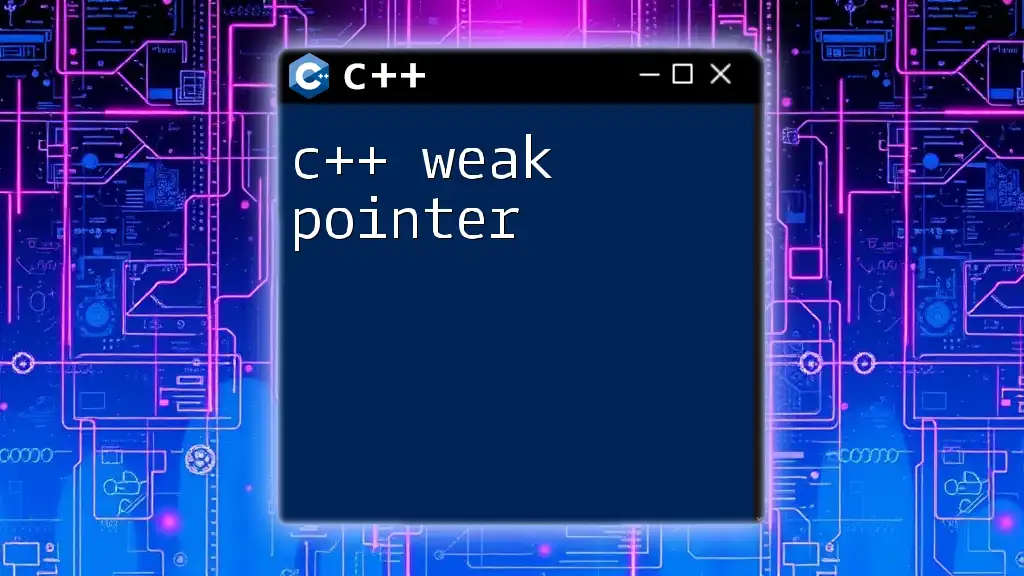
Best Practices for Using `break` and `continue`
When to Use `break` and `continue`
While `break` and `continue` can simplify your code by controlling loop flow, it's crucial to use them wisely. They should be used when they genuinely contribute to the clarity and efficiency of the code.
Maintaining Readability in Code
To ensure your code remains readable while using `break` and `continue`:
- Always aim for clear and concise conditions that dictate loop behavior.
- Employ comments judiciously to elucidate how `break` and `continue` affect your loop logic.
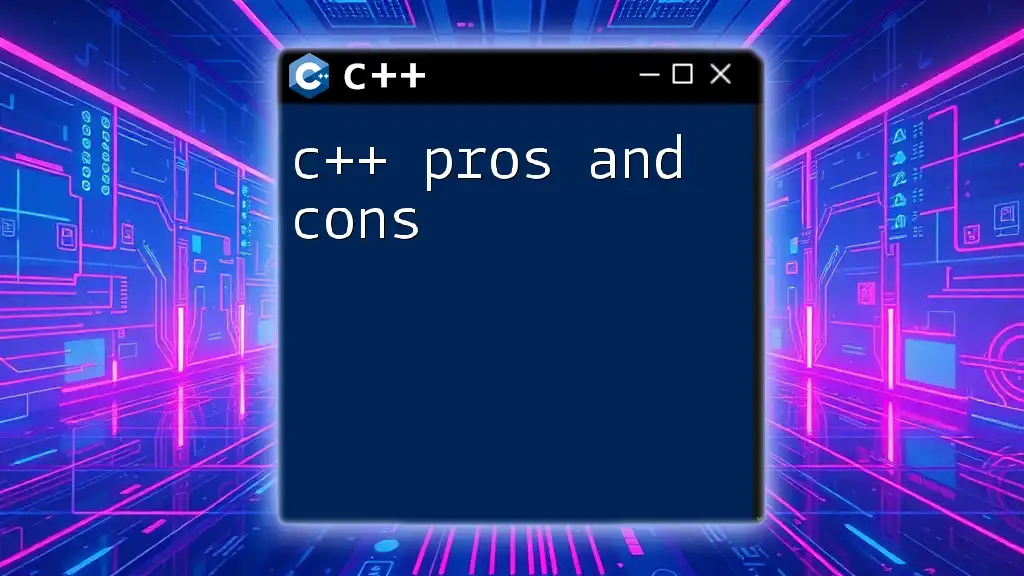
Conclusion
Understanding how to effectively use the `break` and `continue` statements can empower you to write more efficient and clear C++ code. These control flow constructs help you manage loop execution, creating opportunities for optimization and enhancing code comprehensibility. As you advance in your C++ journey, remember that mastering these concepts will greatly aid your programming proficiency. Experiment with implementing `break` and `continue` in your projects to enhance your coding skills!