C++ offers powerful performance and fine control over system resources, but its complexity can lead to steep learning curves for beginners.
Here's a simple example illustrating the syntax of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
C++ is a powerful programming language that combines features of both high-level and low-level programming. It was developed by Bjarne Stroustrup at Bell Labs in the early 1980s as an extension of the C programming language, introducing the concepts of object-oriented programming (OOP). Since then, it has evolved into a versatile tool widely used in diverse applications, from system and software development to game creation.

The Pros of C++
Versatility and Flexibility
One of the most significant pros of C++ is its versatility as a multi-paradigm language. C++ supports various programming styles, including procedural, object-oriented, and generic programming. This flexibility makes it suitable for a wide array of projects, from system software to video games.
For instance, most modern game engines, like Unreal Engine, leverage C++ for its performance capabilities. Below is a simple C++ program that demonstrates its versatility:
#include <iostream>
int main() {
std::cout << "Hello, C++ World!";
return 0;
}
The ability to handle various programming paradigms allows developers to choose the best approach based on their specific requirements.
Performance and Efficiency
C++ is often lauded for its speed and efficiency, as it is a compiled language. Unlike interpreted languages, C++ code is translated into machine code, resulting in faster execution. This is particularly critical in performance-sensitive applications, such as game development or systems programming.
Additionally, C++ provides developers with manual memory management, allowing for fine-tuned control over resource allocation. This can lead to optimized performance, especially in systems with limited resources. Here's an example of optimizing a loop for performance:
#include <iostream>
#include <vector>
#include <chrono>
int main() {
std::vector<int> numbers(1000000, 1);
auto start = std::chrono::high_resolution_clock::now();
for (int i = 0; i < numbers.size(); ++i) {
numbers[i] += 1;
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Time taken: "
<< std::chrono::duration_cast<std::chrono::microseconds>(end - start).count()
<< " microseconds" << std::endl;
return 0;
}
Rich Standard Library (STL)
The Standard Template Library (STL) is another compelling advantage of C++. It provides a rich set of templates and algorithms that streamline development, saving time and effort.
With STL, developers can easily manage data structures such as vectors, lists, and maps, all optimized for performance. Here’s an example of using STL to sort a collection of numbers:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 1, 4, 2};
std::sort(numbers.begin(), numbers.end());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
By harnessing the power of STL, developers can focus more on the algorithm design rather than low-level implementation details.
Object-Oriented Programming (OOP) Support
C++ implements object-oriented programming, which promotes encapsulation, inheritance, and polymorphism. These core principles lead to cleaner code and better organization, particularly in larger projects.
An example of a simple class in C++ demonstrates how OOP is utilized:
class Animal {
public:
virtual void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
class Dog : public Animal {
public:
void speak() override {
std::cout << "Dog barks" << std::endl;
}
};
// Usage
int main() {
Animal* myDog = new Dog();
myDog->speak(); // Output: Dog barks
delete myDog;
return 0;
}
This structure helps in building robust applications where related functionalities are grouped, enhancing maintainability and scalability.
Compatibility with C
C++ maintains backward compatibility with C, which is a critical advantage for developers who want to leverage existing C libraries. C++ code can directly call C functions and utilize C libraries, expanding its functionality without needing substantial rewriting of existing codebases.
This compatibility enables developers to gradually transition from C to C++ while still leveraging the strengths of both languages.
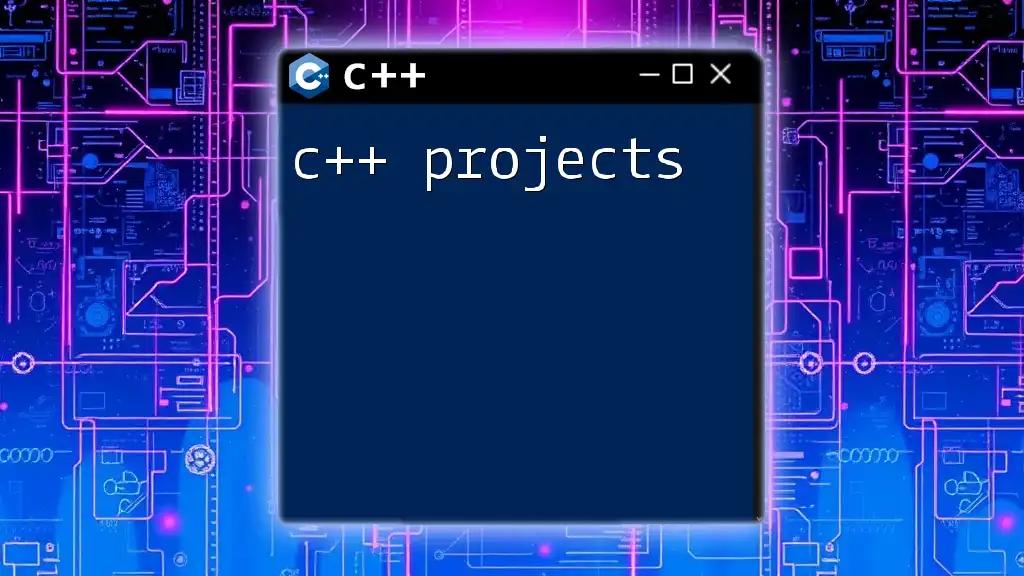
The Cons of C++
Complexity and Learning Curve
Despite its strengths, one of the significant cons of C++ is its complexity. The language has a steep learning curve, particularly for beginners. Concepts such as pointers, memory management, and advanced features like templates can be daunting for newcomers.
New developers often encounter challenges grasping the intricacies of the language, leading to frustration when trying to write error-free code.
Lack of Garbage Collection
C++ does not feature automatic garbage collection, which means developers are responsible for managing memory themselves. This manual memory management can lead to memory leaks and undefined behavior if not handled correctly.
For instance, if memory is allocated but not freed properly, it results in wasted resources:
int* ptr = new int(5); // Memory allocated
// Some operations...
delete ptr; // Proper memory management
Failure to use `delete` can lead to memory leaks, making careful memory management a necessity when developing in C++.
Potential for Undefined Behavior
C++ gives developers significant control, but this also opens the door to errors like undefined behavior. Accessing out-of-bounds array elements, dereferencing null pointers, or using uninitialized variables can have unpredictable consequences, making debugging difficult.
For example, accessing an array out of its bounds may lead to a program crash or corrupted memory:
int arr[5] = {0, 1, 2, 3, 4};
// Undefined Behavior (Out of bounds access)
std::cout << arr[6]; // This could lead to a crash
Compilation Speed
C++ programs often take longer to compile due to their complexity and the extensive use of templates. This could slow down the development process, particularly in large projects with many dependencies. Longer compile times can frustrate developers, especially in iterative development cycles.
Standardization Issues
While C++ is standardized, inconsistencies between compilers can lead to compatibility issues. Variations in how different compilers implement certain aspects of the language can create challenges in cross-platform development.
Furthermore, differences in language standards (C++11, C++14, C++17, etc.) can also introduce additional complications. Developers may face difficulties ensuring their code is compatible across various environments.
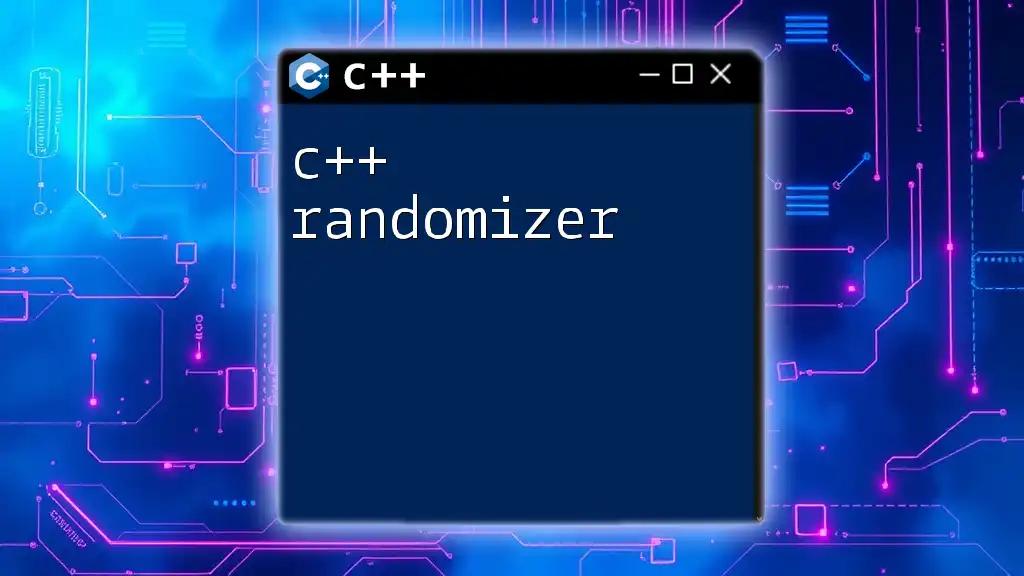
Conclusion
Understanding the c++ pros and cons is essential for anyone looking to learn or work with this powerful language. C++ offers remarkable versatility, performance, and an extensive library, making it a key player in software development. However, its complexity, manual memory management, and potential for undefined behavior present challenges that developers must navigate carefully.
Balancing its benefits against its challenges is crucial for making informed decisions about when and how to use C++ effectively.

Frequently Asked Questions
Is C++ suitable for beginners?
While C++ is a powerful and versatile language, its steep learning curve can be a barrier for beginners. However, mastering C++ facilities essential programming skills that carry over to other languages.
What types of projects are best suited for C++?
C++ excels in performance-critical applications, such as game development, system programming, and real-time simulations. Its versatility makes it suitable for a vast scope of projects across industries.
How does C++ compare to other programming languages?
C++ provides more control and efficiency than many interpreted languages, such as Python. However, it also requires a more in-depth understanding of low-level programming concepts, making it less beginner-friendly compared to languages like Java or Python.