Breadth First Search (BFS) is a graph traversal algorithm that explores all neighboring nodes at the present depth before moving on to nodes at the next depth level.
Here’s a simple implementation of BFS in C++:
#include <iostream>
#include <vector>
#include <queue>
void bfs(int start, const std::vector<std::vector<int>>& adj) {
std::vector<bool> visited(adj.size(), false);
std::queue<int> q;
visited[start] = true;
q.push(start);
while (!q.empty()) {
int node = q.front();
q.pop();
std::cout << node << " ";
for (int neighbor : adj[node]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
q.push(neighbor);
}
}
}
}
int main() {
std::vector<std::vector<int>> adj = {
{1, 2}, // 0
{0, 3, 4}, // 1
{0, 4}, // 2
{1}, // 3
{1, 2} // 4
};
bfs(0, adj);
return 0;
}
What is BFS?
Breadth-First Search (BFS) is a fundamental algorithm used for traversing or searching tree or graph data structures. It explores vertices in layers, starting from a given source node and visiting all of its neighbors before moving on to their neighbors. This "layer-by-layer" approach makes BFS particularly effective for finding the shortest path in unweighted graphs—a common scenario in various applications like networking, pathfinding, and solving puzzles.
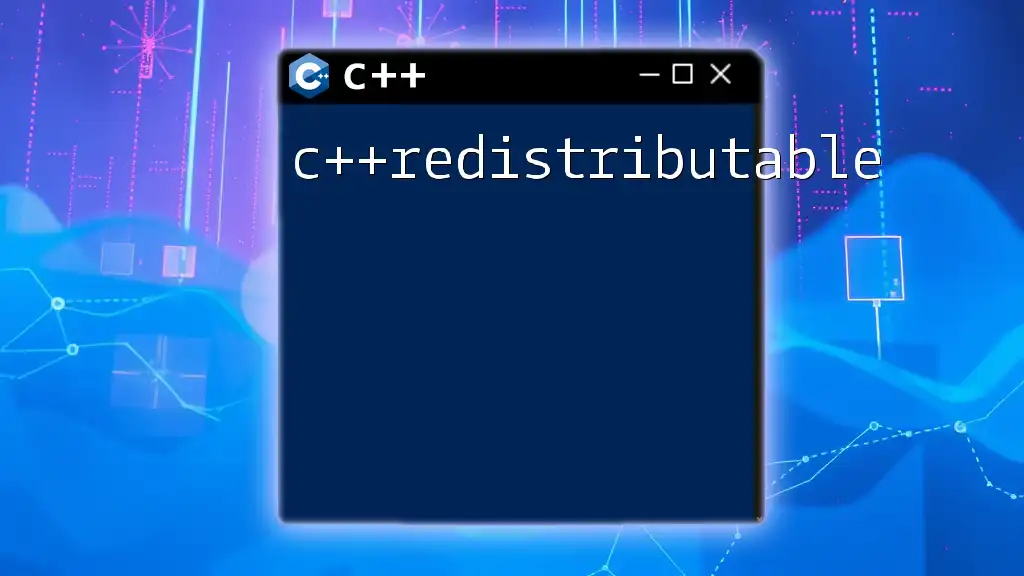
Why Use BFS?
When comparing BFS to other search algorithms, such as Depth-First Search (DFS), it’s essential to understand when and why BFS excels. BFS is particularly beneficial in:
- Finding the shortest path in an unweighted graph.
- Exploring all possibilities in a systematic manner (e.g., solving mazes).
- Level-order traversal in trees, where nodes are processed level by level.
In cases where the shortest path is a priority and the graph is not weighted, BFS shines and offers a more optimal solution.
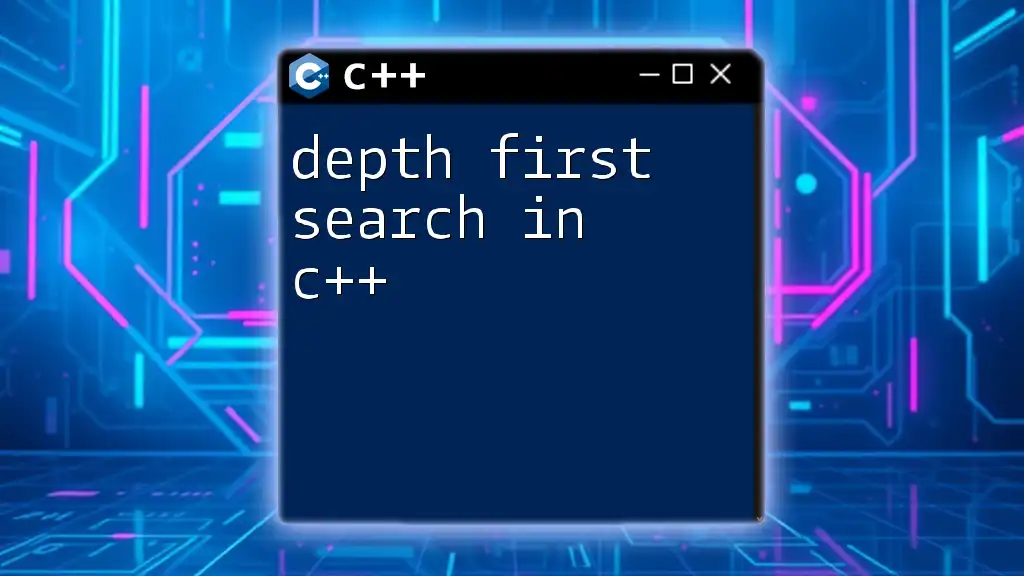
Understanding the BFS Algorithm in C++
Basic Concept of BFS Algorithm
The BFS algorithm works by exploring all nodes at the present depth level before moving on to nodes at the next depth level. This means that each vertex is processed, and its unvisited adjacent vertices are added to a queue. The queue allows BFS to manage which vertex to process next effectively.
Key Components of BFS
Graph Representation
To implement BFS, a clear representation of the graph is necessary. In C++, graphs are commonly represented using either adjacency lists or adjacency matrices.
An adjacency list is generally more efficient in terms of space when dealing with sparse graphs. Here’s a simple representation of a graph as an adjacency list:
#include <vector>
std::vector<std::vector<int>> graph = {
{1, 2}, // Neighbors of vertex 0
{0, 3, 4}, // Neighbors of vertex 1
{0}, // Neighbors of vertex 2
{1, 4}, // Neighbors of vertex 3
{1, 3} // Neighbors of vertex 4
};
Queue Data Structure
The queue is a vital component in BFS that adheres to the First-In-First-Out (FIFO) principle. It holds all the nodes that need to be processed while ensuring the exploration happens layer by layer. Implementing BFS without a queue may lead to incorrect or inefficient traversal.
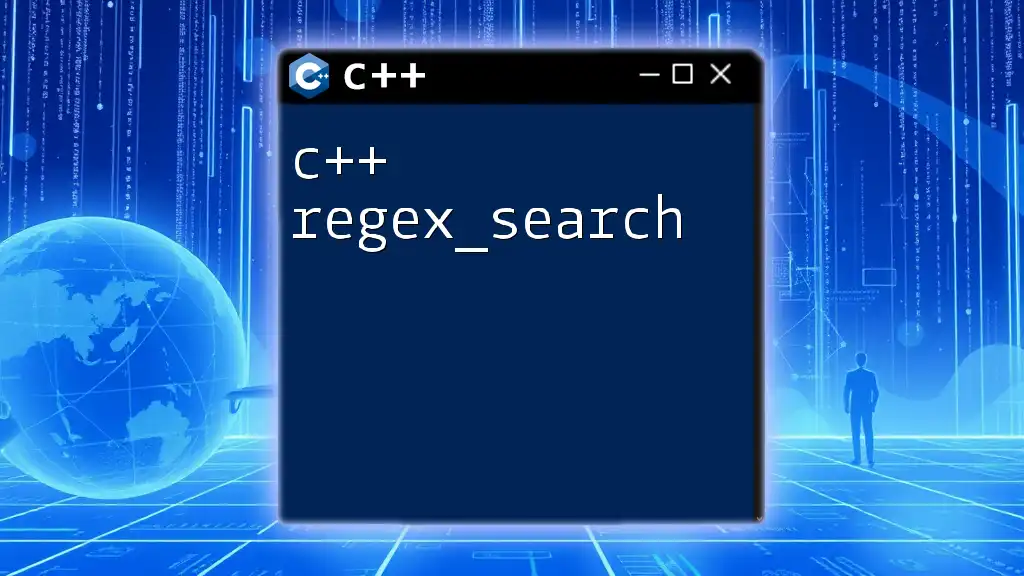
Implementing BFS Algorithm in C++
Setting Up Your C++ Environment
To begin implementing BFS, you need a proper setup. Typically, this involves using STL libraries such as `<vector>`, `<queue>`, and `<iostream>`. Ensure your environment allows you to compile standard C++ code.
Implementing BFS: Step by Step
Initialization of Variables
When implementing BFS, the first step is to initialize a queue and a vector to keep track of visited nodes. This ensures that each node is processed only once.
Here’s a clean example of setting up the BFS function:
#include <iostream>
#include <vector>
#include <queue>
void bfs(int start, const std::vector<std::vector<int>>& graph) {
std::vector<bool> visited(graph.size(), false);
std::queue<int> q;
visited[start] = true; // Mark the start node as visited
q.push(start); // Add the start node to the queue
Traversing the Graph
The heart of the BFS algorithm lies in the traversal loop. This loop continues until there are no more nodes in the queue. During this process, the algorithm will dequeue a node, process it (print or store the value), and enqueue all its unvisited neighbors.
Here’s how you process and explore nodes in the BFS loop:
while (!q.empty()) {
int node = q.front();
q.pop();
std::cout << node << " "; // Process the node
for (int neighbor : graph[node]) {
if (!visited[neighbor]) {
visited[neighbor] = true; // Mark the neighbor as visited
q.push(neighbor); // Add neighbor to queue
}
}
}
}
In this implementation, as nodes are visited, they are marked as visited to prevent reprocessing them.
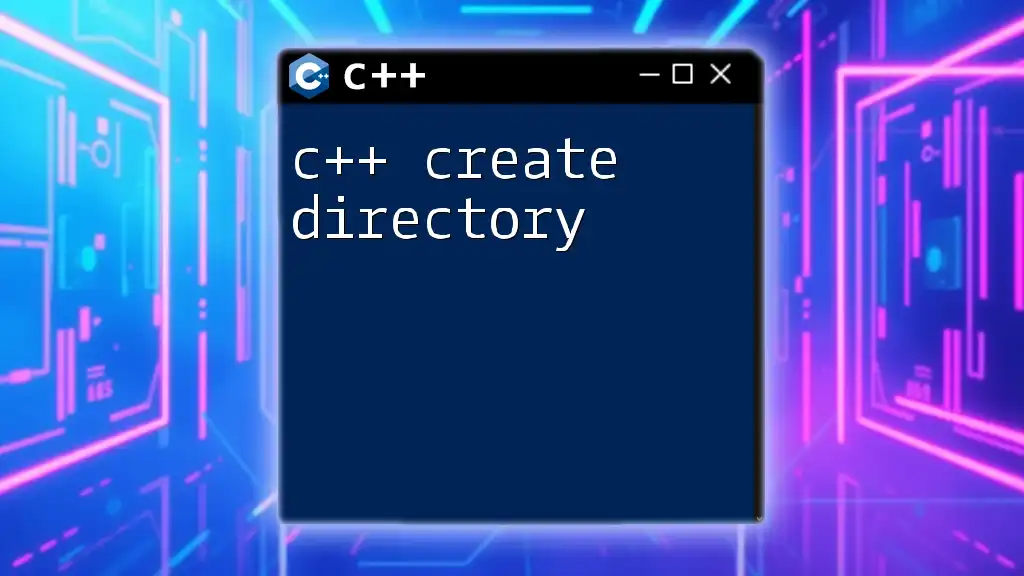
Real-World Applications of BFS
Finding Shortest Paths in Unweighted Graphs
One of the most compelling uses of BFS is finding the shortest path in unweighted graphs. A practical example could be navigating a maze where each decision point is represented as a node and potential moves as edges. BFS effectively explores all routes before determining the shortest.
Here’s a brief illustration of how BFS handles this within the structure outlined above.
Web Crawlers and Network Broadcasting
Another fascinating application of BFS is in web crawlers, where a crawler starts with a list of URLs (nodes) and explores each linked page systematically, broadening its search outward until every page within the specified depth has been visited.
Broadcasting in networks also utilizes BFS, where messages are propagated from one node to all other nodes in a systematic manner, ensuring every node receives the message without redundancy.
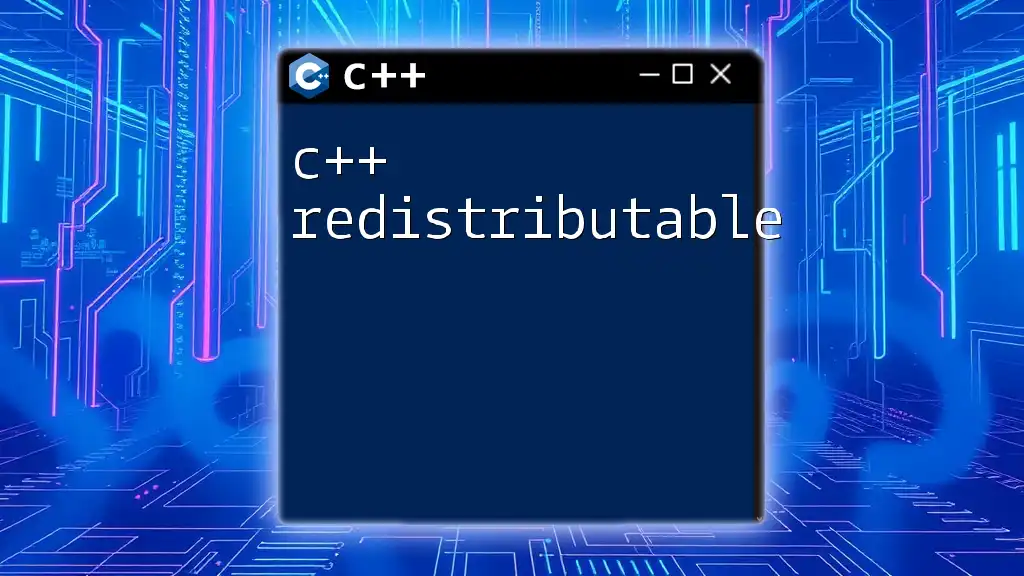
Optimizing BFS in C++
Handling Large Graphs
For large graphs, memory management and execution time become critical. Using data structures like hash maps can optimize the visited array by storing only necessary nodes instead of a vector that scales with the graph size. This is beneficial when working with sparse graphs where many entries may never get visited.
Multi-threading BFS
In specific scenarios, a multi-threaded approach can be applied to BFS to speed up processing. While BFS is fundamentally sequential, dividing the graph into smaller, manageable sections and processing them in parallel can lead to significant performance improvements.
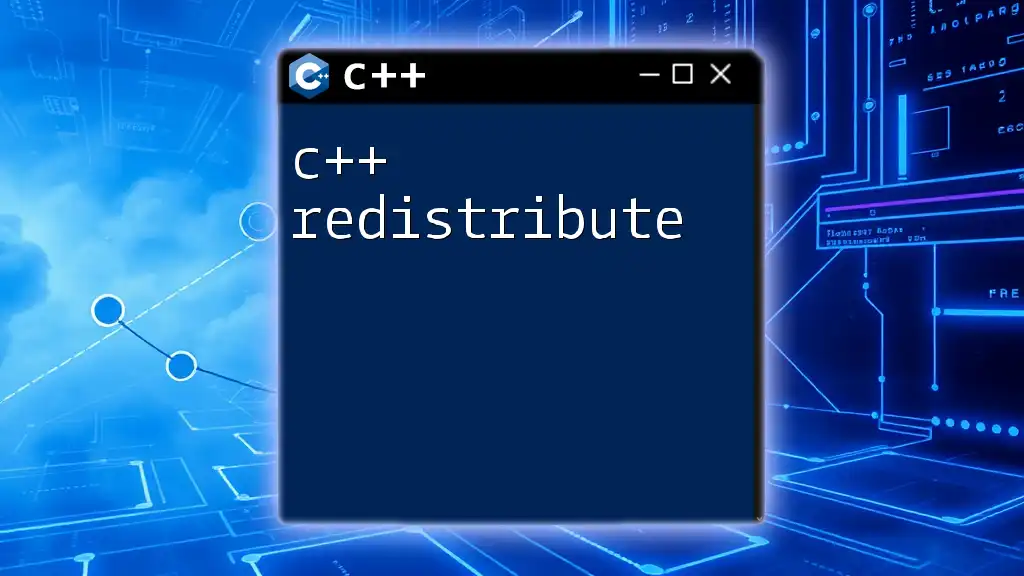
Common Pitfalls in BFS Implementation
Infinite Loops in Cyclic Graphs
Sources of infinite loops typically arise in cyclic graphs. Effective cycle management involves marking nodes as visited before they are enqueued. Failing to do so could result in processing the same node repeatedly.
Improper Queue Management
Common mistakes in using queues include forgetting to dequeue nodes or failing to mark them as visited. Proper queue management and following the BFS structure ensure the algorithm runs efficiently without errors.
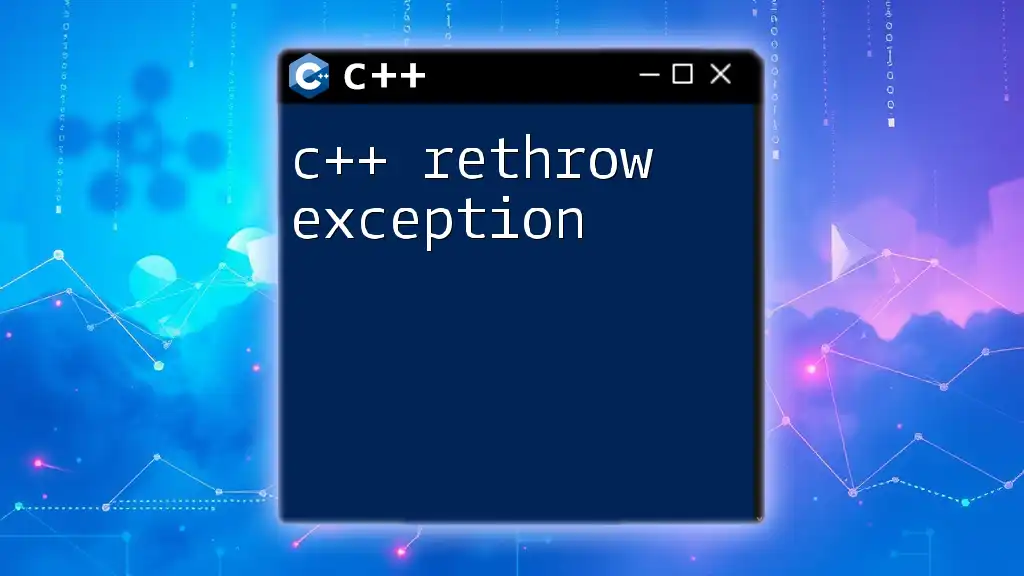
Summary of Key Takeaways
The BFS algorithm is an essential tool in the C++ developer’s arsenal. It provides a systematic way to explore and navigate graph-like structures effectively, making it invaluable for pathfinding and similar applications.
Encouragement to Practice
To reinforce your grasp of BFS concepts, practice with various graph structures. Implement BFS in different scenarios, such as shortest path discovery and exploring new ways to enhance its efficiency.
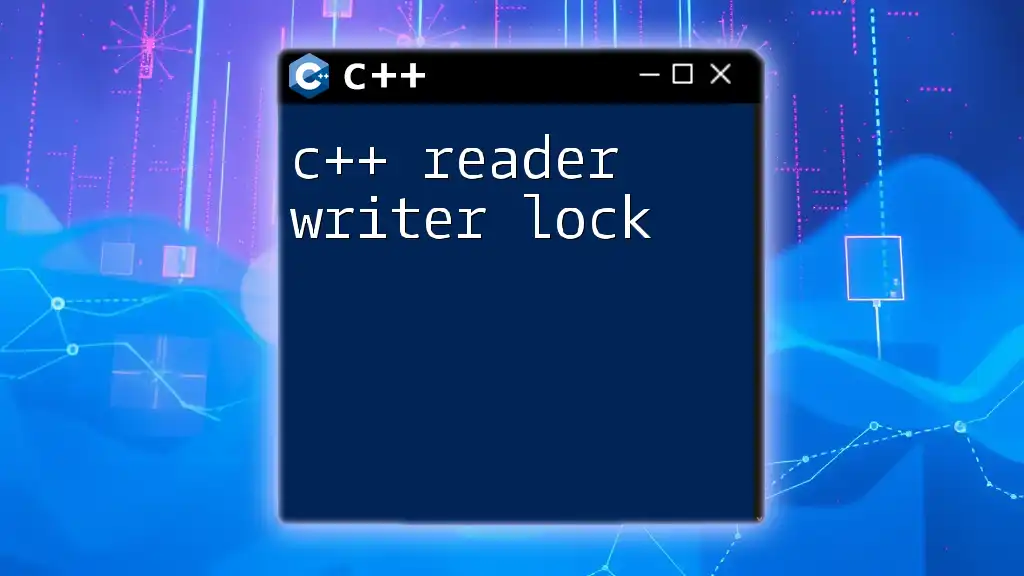
Additional Resources
Useful Libraries for C++ Graph Algorithms
Several C++ libraries can assist in more complex graph traversal scenarios. Libraries like Boost Graph Library can enrich your BFS implementations, offering an array of tools for advanced graph operations.
Recommendations for Further Learning
For further comprehension, delve into books and online courses that delve deeper into graph algorithms and data structures. Websites like Coursera and Udacity provide excellent resources for learning about graph theory and its practical applications.
By engaging with these concepts and tools, you will equip yourself with the knowledge to apply C++ breadth-first search proficiently in various programming scenarios.