Depth First Search (DFS) in C++ is an algorithm used to traverse or search through graph data structures by exploring as far as possible along each branch before backtracking.
Here's a simple example of Depth First Search implemented in C++:
#include <iostream>
#include <vector>
using namespace std;
void DFS(int vertex, vector<bool> &visited, const vector<vector<int>> &graph) {
visited[vertex] = true;
cout << vertex << " ";
for (int i : graph[vertex]) {
if (!visited[i]) {
DFS(i, visited, graph);
}
}
}
int main() {
vector<vector<int>> graph = {
{1, 2}, // connections for vertex 0
{0, 3, 4}, // connections for vertex 1
{0}, // connections for vertex 2
{1}, // connections for vertex 3
{1} // connections for vertex 4
};
vector<bool> visited(graph.size(), false);
cout << "Depth First Search starting from vertex 0:" << endl;
DFS(0, visited, graph);
return 0;
}
What is Depth First Search?
Depth First Search (DFS) is a widely-used algorithm for traversing or searching tree or graph data structures. The algorithm starts at a selected node (often referred to as the "root") and explores as far as possible along each branch before backtracking. This approach ensures that every node is visited, making it valuable for various applications.
One key distinction to understand about DFS is how it differs from other search algorithms like Breadth First Search (BFS). While BFS explores nodes layer by layer, DFS dives deep into a graph's branches before exploring sibling nodes at the same depth. This fundamental difference defines the algorithm's performance, memory requirements, and typical use cases.
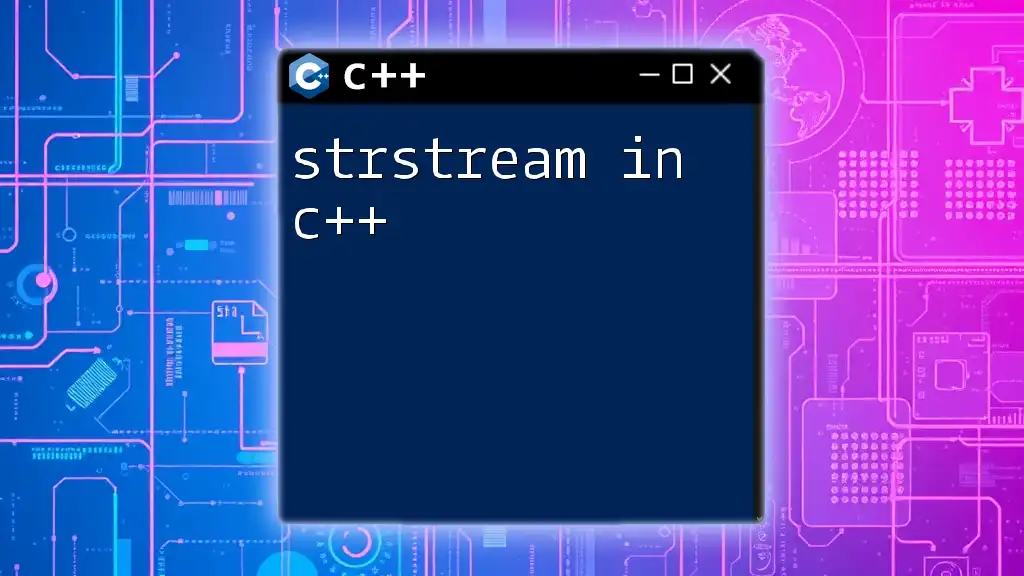
Applications of Depth First Search
DFS has a myriad of applications:
- Graph Traversal: To visit nodes in a deep, exhaustive manner, which is crucial in many graph problems.
- Solving Puzzles: It can be applied to games and puzzles, such as mazes or Sudoku, leveraging its ability to explore all possibilities.
- Pathfinding Algorithms: Useful for scenarios where you want to find paths or connections in complex network structures.
- Topological Sorting: Organizing tasks or events based on dependencies — DFS can help determine the proper sequence of execution.
- Web Crawling: Used in search engines to build a comprehensive index of web pages through deep exploration of site links.
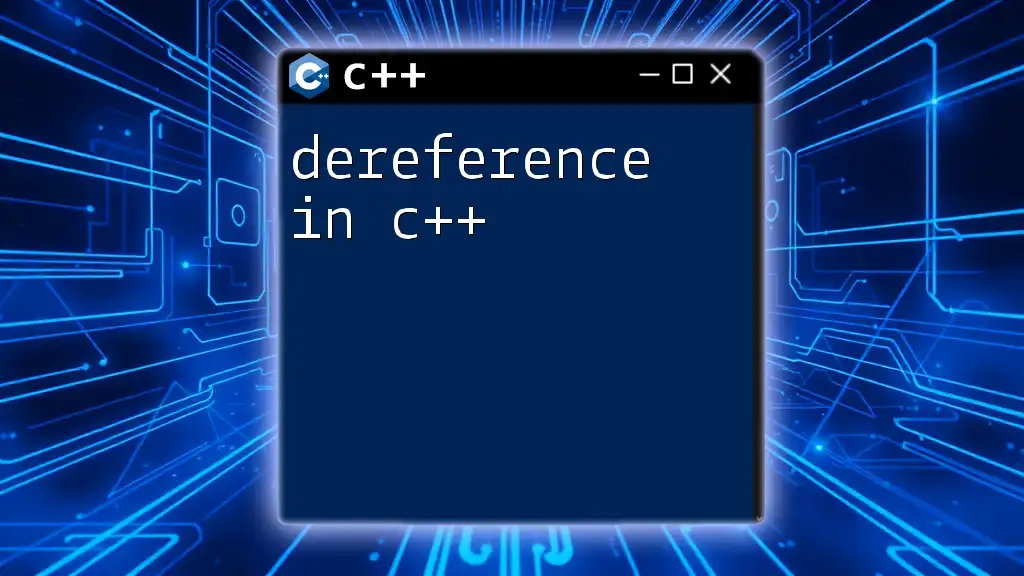
Understanding Graphs
Types of Graphs
Understanding the types of graphs is essential for implementing DFS effectively. The two main categories are:
- Directed Graphs: Edges have a direction, indicating a one-way relationship between two vertices (nodes).
- Undirected Graphs: Edges signify a two-way relationship between nodes, allowing traversal in either direction.
Another critical distinction is between:
- Weighted Graphs: Each edge carries a weight, representing cost, distance, or time, which can be significant in routing problems.
- Unweighted Graphs: All edges are treated equally, which simplifies computations.
Graph Representation in C++
Graph algorithms often hinge on how graphs are represented. The two common representations are:
-
Adjacency List Representation: In this method, each node maintains a list of its adjacent (neighboring) nodes. This is memory-efficient for sparse graphs.
Example:
std::vector<std::vector<int>> graph(numNodes); graph[0] = {1, 2}; // Node 0 -> Node 1 and Node 2 graph[1] = {0, 3}; // Node 1 -> Node 0 and Node 3
-
Adjacency Matrix Representation: Here, a 2D array is used to represent edges. If there’s an edge between nodes i and j, the cell at (i, j) is marked.
Example:
std::vector<std::vector<int>> graph(numNodes, std::vector<int>(numNodes, 0)); graph[0][1] = 1; // Edge exists between Node 0 and Node 1 graph[1][3] = 1; // Edge exists between Node 1 and Node 3
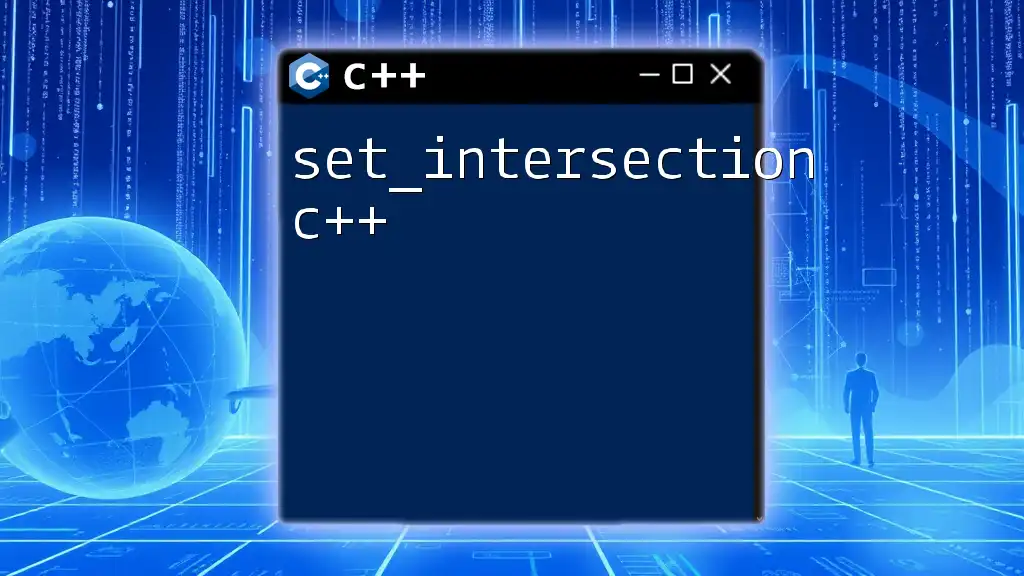
Implementing Depth First Search in C++
Iterative DFS
Understanding the Iterative Approach
The iterative version of DFS utilizes a stack to keep track of the nodes to be explored. This method avoids the pitfalls of deep recursion, especially in scenarios where a high tree depth might cause stack overflow errors.
Code Snippet for Iterative DFS
Here’s how a simple iterative DFS is implemented:
#include <iostream>
#include <vector>
#include <stack>
void iterativeDFS(int startNode, const std::vector<std::vector<int>>& graph) {
std::stack<int> stack;
std::vector<bool> visited(graph.size(), false);
stack.push(startNode);
while (!stack.empty()) {
int node = stack.top();
stack.pop();
if (!visited[node]) {
std::cout << "Visited: " << node << std::endl;
visited[node] = true;
}
for (const int &neighbor : graph[node]) {
if (!visited[neighbor]) {
stack.push(neighbor);
}
}
}
}
Each element of the algorithm indicates which node is currently being explored and which nodes remain to be visited. The function maintains a list of visited nodes to prevent cycles or redundant visits.
Recursive DFS
Understanding the Recursive Approach
The recursive version of DFS is conceptually simpler, relying on function call stacks to remember paths. Recursive DFS is elegant but can encounter limitations based on system stack size and deep recursion limits.
Code Snippet for Recursive DFS
The recursive implementation looks like this:
#include <iostream>
#include <vector>
void recursiveDFS(int node, const std::vector<std::vector<int>>& graph, std::vector<bool>& visited) {
visited[node] = true;
std::cout << "Visited: " << node << std::endl;
for (const int &neighbor : graph[node]) {
if (!visited[neighbor]) {
recursiveDFS(neighbor, graph, visited);
}
}
}
void startDFS(int startNode, const std::vector<std::vector<int>>& graph) {
std::vector<bool> visited(graph.size(), false);
recursiveDFS(startNode, graph, visited);
}
This implementation recursively visits every unvisited neighboring node, thereby exploring the entire depth of the graph.
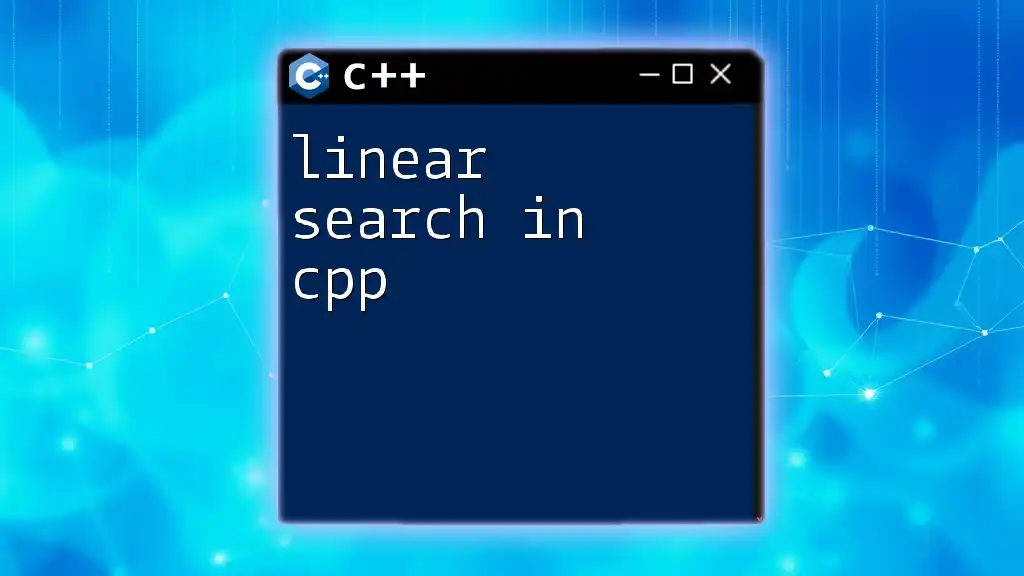
Comparing Iterative and Recursive DFS
Performance Considerations
Both iterative and recursive DFS share the same time complexity of O(V + E), where V is the number of vertices and E is the number of edges. However, their space complexity varies:
- Iterative DFS leverages a stack data structure, which can often be more memory-efficient than the call stack used in recursion.
- Recursive DFS can lead to stack overflow for deep trees, although it's generally easier to implement and understand.
Common Use-Cases that Favor One Approach Over the Other
In situations where the graph is wide and shallow, iterative DFS can handle broader searches without hitting memory limits. Conversely, for problems that naturally fit a recursive structure, such as tree traversals or problems involving backtracking, recursive DFS is often more intuitive.
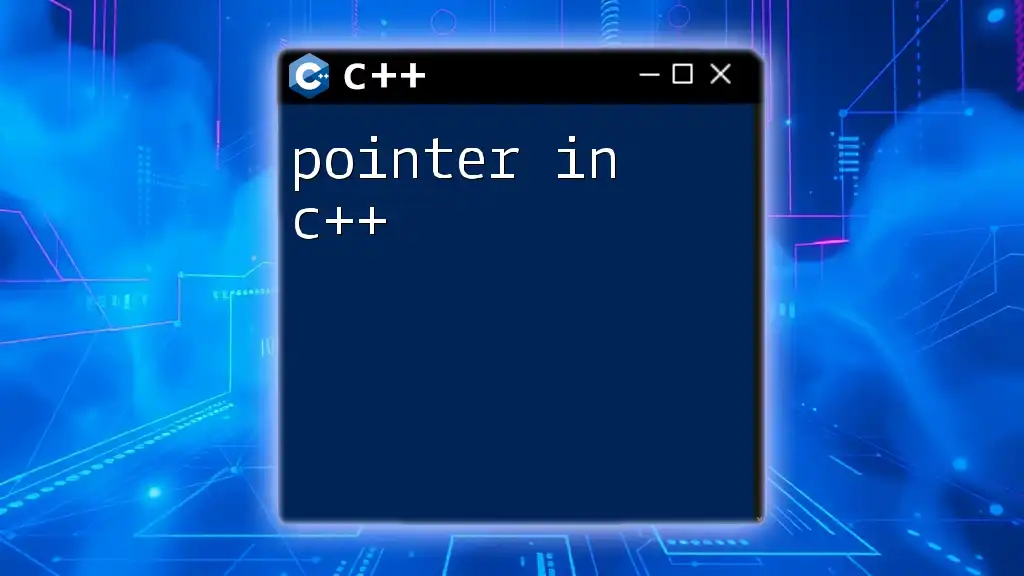
Advanced Topics in Depth First Search
Depth Limit Search
In certain scenarios, DFS may require a limit on how deep it dives into a graph structure. This is useful to prevent infinite recursion in cyclic graphs. A depth limit can be integrated easily into either an iterative or recursive implementation by checking against a predefined limit before making further recursive calls or pushing onto the stack.
Finding Connected Components
DFS is excellent for discovering connected components in a graph, which are subsets of nodes that are interconnected. Here's a brief example of how this can be accomplished:
void findConnectedComponents(const std::vector<std::vector<int>>& graph) {
std::vector<bool> visited(graph.size(), false);
for (int i = 0; i < graph.size(); ++i) {
if (!visited[i]) {
startDFS(i, graph); // Call DFS to visit this component
std::cout << "Finished component at node: " << i << std::endl;
}
}
}
Each call to `startDFS` marks a new connected component.
Cycle Detection in Graphs
Another application of DFS is detecting cycles in graphs. This is crucial for applications where cyclic paths can create infinite loops. Below is how you can implement cycle detection using DFS:
bool isCyclicUtil(int node, const std::vector<std::vector<int>>& graph, std::vector<bool>& visited, int parent) {
visited[node] = true;
for (const int &neighbor : graph[node]) {
if (!visited[neighbor]) {
if (isCyclicUtil(neighbor, graph, visited, node)) {
return true;
}
} else if (neighbor != parent) {
return true;
}
}
return false;
}
bool isCyclic(const std::vector<std::vector<int>>& graph) {
std::vector<bool> visited(graph.size(), false);
for (int i = 0; i < graph.size(); ++i) {
if (!visited[i]) {
if (isCyclicUtil(i, graph, visited, -1)) {
return true;
}
}
}
return false;
}
This function checks each node's neighbors while ensuring that if a neighbor is visited, it's not the immediate parent, which would indicate a cycle.
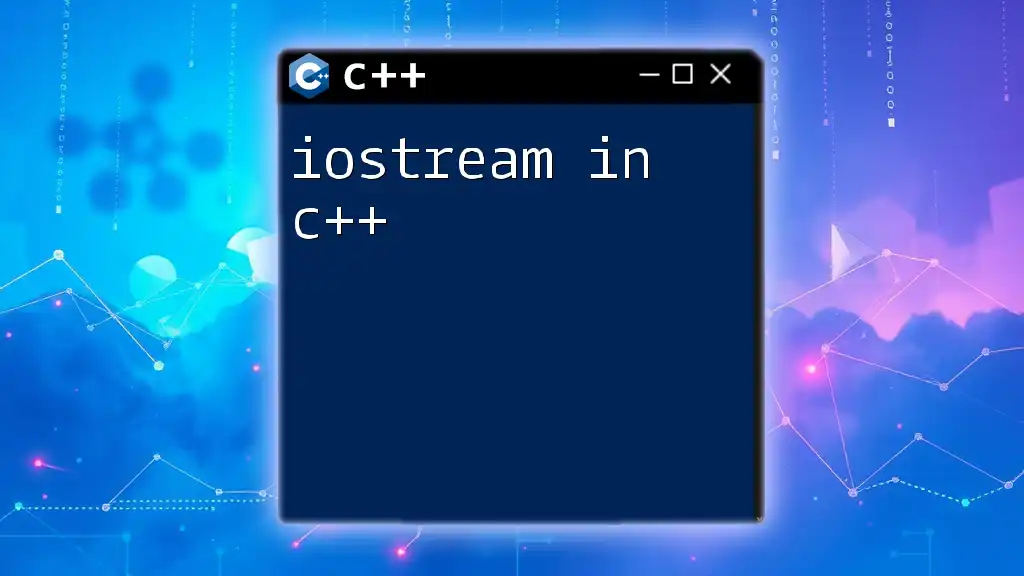
Best Practices and Tips for DFS Implementation
Code Optimization Techniques
Avoid Redundant Operations: Ensure that the adjacent nodes are explored only once, as demonstrated in the code examples above. Maintaining a visited list effectively prevents revisiting nodes.
Memory Usage: Consider the size of the stack or recursion depths, especially in large or complicated graphs. Limit recursion or use iterative solutions when appropriate to avoid stack overflow.
Debugging Common Pitfalls
Common pitfalls include:
- Failing to mark nodes as visited properly, leading to infinite loops.
- Incorrectly setting up the graph structure, which can lead to null references or unexpected behaviors.
- Not considering edge cases such as graphs with only one node or completely disconnected graphs.
Adhering to clear debugging practices, such as adding print statements or assertions, can significantly ease the debugging process.
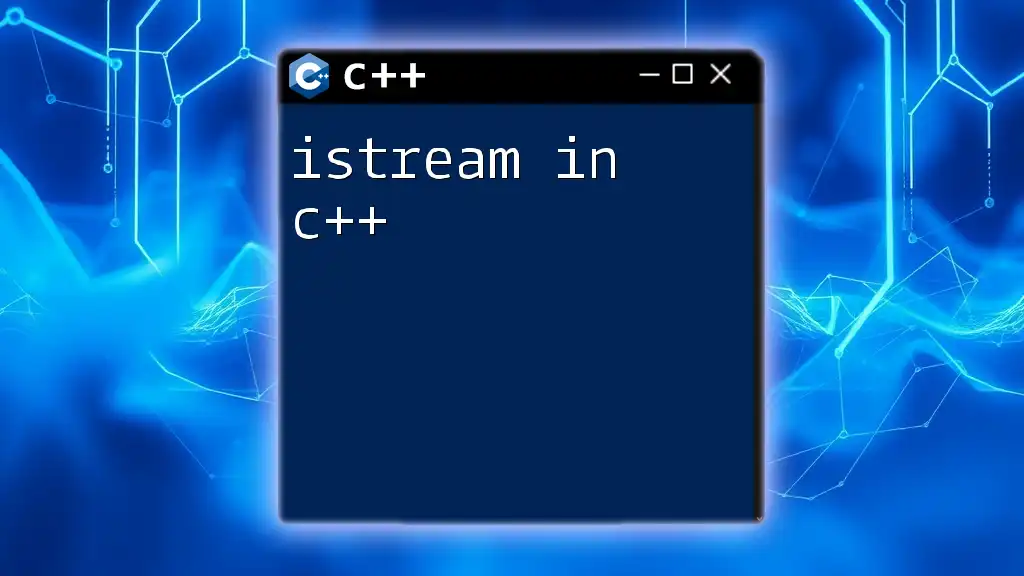
Conclusion
In summary, Depth First Search in C++ is a versatile and powerful technique for navigating graphs and trees, with applications spanning from simple traversals to complex problem-solving scenarios. Understanding both iterative and recursive implementations equips developers with the tools necessary to tackle a wide range of algorithmic challenges.
For those eager to deepen their understanding of DFS, continuous practice through varied problems is essential. Resources such as textbooks, online courses, and coding forums provide excellent avenues for further learning. Engaging with the community can also enhance your grasp of DFS and its applications.