In C++, you can remove the first element from a vector using the `erase` method combined with the `begin` iterator, as shown in the following code snippet:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin()); // Removes the first element
for (int n : vec) {
std::cout << n << ' '; // Outputs: 2 3 4 5
}
return 0;
}
What is a C++ Vector?
In C++, a vector is a dynamic array provided by the Standard Template Library (STL) that can automatically resize itself when necessary. This feature makes vectors highly versatile compared to standard arrays that have a fixed size.
Vectors come with several advantages:
- Dynamic Sizing: Vectors adjust their size automatically, allowing you to add or remove elements easily without worrying about memory allocation.
- Ordered Storage: Elements are stored in the order they are inserted, preserving the sequence of data.
- Performance: Accessing elements is O(1), which means it is very fast to access elements by their index.
Need for Removing Elements
Removing elements from a vector, including the first element, may be necessary in various programming scenarios. Some common situations include:
- When processing user inputs where the initial data is no longer relevant.
- In simulations or games where certain elements (like bullets in a game) must be removed after they have served their purpose.
- Data processing tasks where outdated records need to be cleaned up.
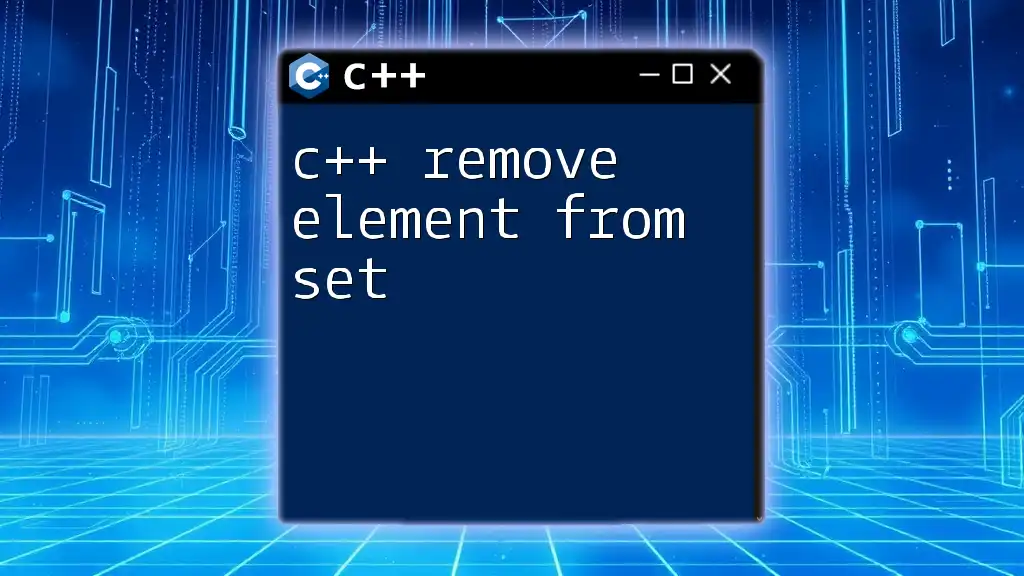
Understanding How to Remove the First Element from a Vector
Methods to Remove the First Element
Using `erase()` Function
The most straightforward method for removing the first element from a vector is by using the `erase()` function. This method modifies the size of the vector and can be easily executed as shown below.
Syntax:
vector.erase(position);
Here, the `position` is an iterator pointing to the element you wish to remove.
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin()); // Removes the first element
std::cout << "After removing the first element: ";
for (auto v : vec) std::cout << v << " "; // Output: 2 3 4 5
return 0;
}
In this example, the `erase()` function successfully removes the first element from the vector, leaving the subsequent elements intact.
Using `pop_front()` (Custom Implementation)
While the C++ standard library's `std::vector` does not have a built-in `pop_front()` function like some other STL containers (e.g., `std::deque`), we can create a custom function to achieve this.
Example of a Custom `pop_front()` Implementation:
#include <iostream>
#include <vector>
void pop_front(std::vector<int>& vec) {
if (!vec.empty()) {
vec.erase(vec.begin());
}
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
pop_front(vec); // Removes the first element
std::cout << "After custom pop_front: ";
for (auto v : vec) std::cout << v << " "; // Output: 2 3 4 5
return 0;
}
In this example, the `pop_front()` function checks if the vector is not empty before calling `erase()` to remove the first element safely.
Considerations When Removing Elements
Impact on Performance
Removing the first element using `erase()` has a time complexity of O(n), as it needs to shift all subsequent elements to fill the gap left by the removed element. If performance is a concern due to frequent removals at the front of the vector, consider using `std::deque` instead, which is optimized for quick inserts and deletes at both ends.
Memory Management
When you remove an element from a vector, the vector may need to reallocate its memory depending on the new size. This process will handle automatically, but it's worth recognizing that repeated removals can lead to additional overhead if the vector frequently resizes itself.
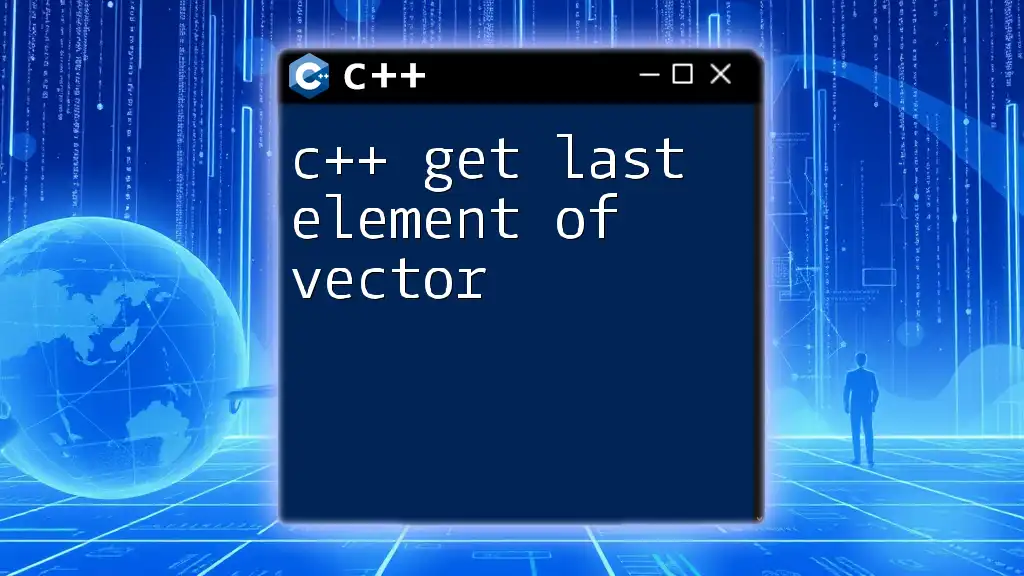
Alternative Methods for Removing Elements
Removing Elements from a Vector (Not Just the First)
While this article focuses on removing the first element, you may also wish to remove elements at specific positions or based on certain conditions. You can leverage the `remove_if` algorithm in combination with `erase()` to accomplish this task.
Example: Removing Specific Elements:
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 2, 4, 2};
vec.erase(std::remove(vec.begin(), vec.end(), 2), vec.end()); // Removes all instances of 2
std::cout << "After removing all instances of 2: ";
for (auto v : vec) std::cout << v << " "; // Output: 1 3 4
return 0;
}
Clearing the Vector Completely
If you need to remove all elements from a vector, you can use the `clear()` method, which effectively empties the vector without deallocating the memory allocated for the vector.
Example:
std::vector<int> vec = {1, 2, 3};
vec.clear(); // All elements removed, size becomes 0
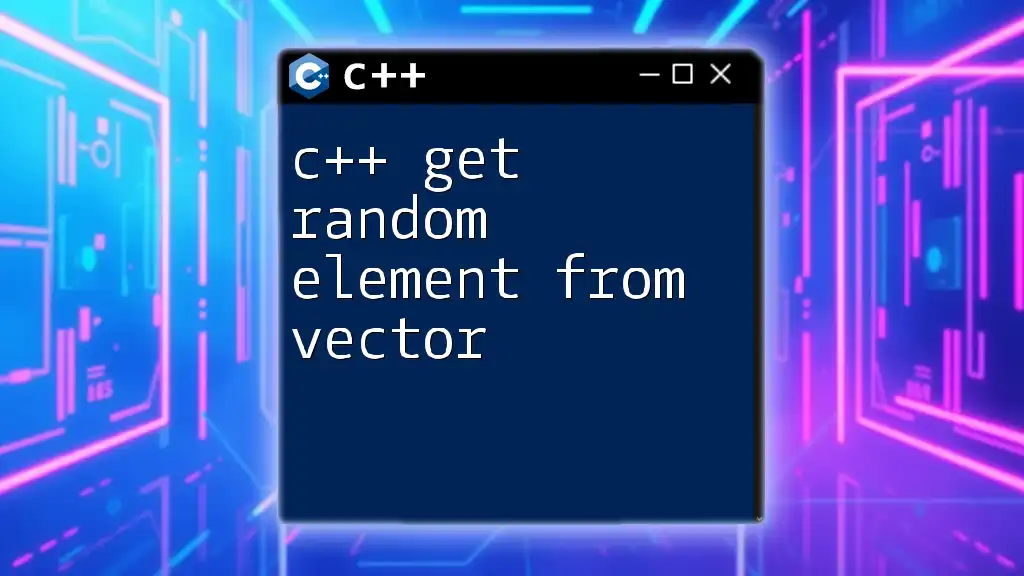
Best Practices for Managing Vectors
Efficient Use of Vectors
To maintain both efficiency and performance when working with vectors, consider these best practices:
- Pre-allocate space using `reserve()` when you know the number of elements in advance to minimize reallocations.
- Use the `emplace_back()` function instead of `push_back()` when dealing with complex objects to avoid unnecessary copies.
Error Handling
When manipulating vectors, it's crucial to handle potential errors, such as attempting to remove an element from an empty vector. Implement checks to ensure your program remains robust:
if (vec.empty()) {
std::cerr << "Vector is empty, cannot remove elements." << std::endl;
}
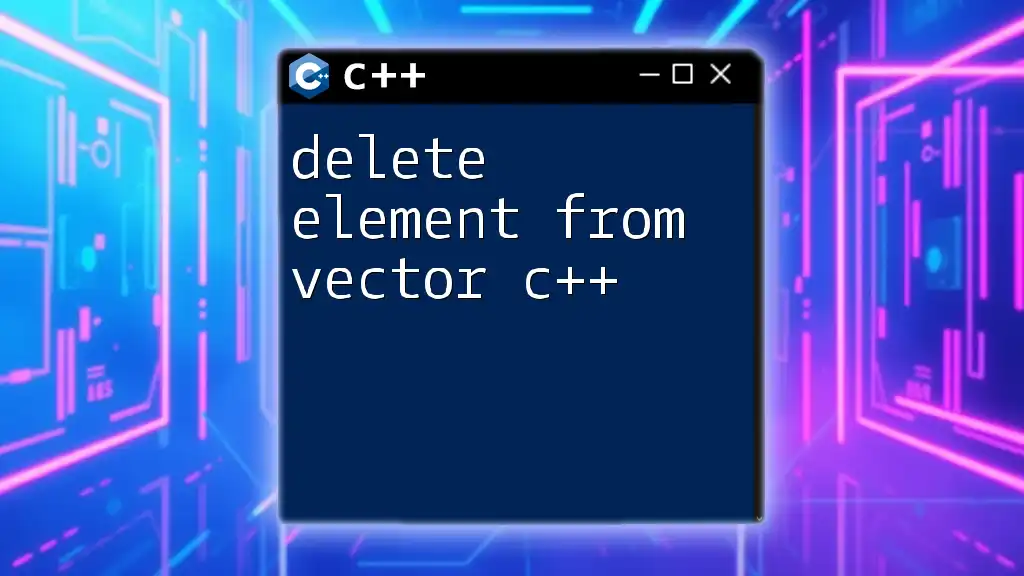
Frequently Asked Questions
How to Remove an Element from Vector in C++?
You can use the `erase()` function to remove any element from a vector by passing the iterator pointing to that element. To remove the first element, simply use `vec.erase(vec.begin());`.
Can I Remove an Element from a Specific Position?
Yes, to remove an element from a specific position, provide the appropriate iterator to the `erase()` function. For example, `vec.erase(vec.begin() + 2);` would remove the third element from the vector.
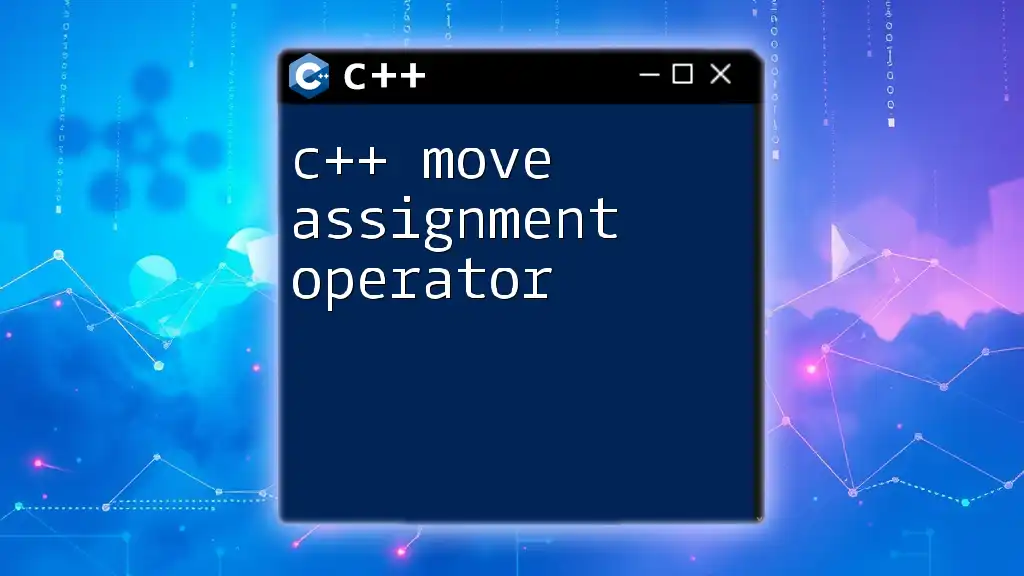
Conclusion
In this article, we explored how to c++ remove first element from vector through different methods, including the usage of the `erase()` function and a custom implementation with `pop_front()`. We discussed related topics, like performance considerations and best practices, to encourage efficient vector management.
By understanding these techniques and practices, you can enhance your proficiency in C++ and manipulate vectors effectively. Consider applying these concepts in your programming projects and experimenting with additional vector manipulations to solidify your understanding.