To retrieve a random element from a vector in C++, you can use the `<random>` library along with the `std::uniform_int_distribution` to generate a random index.
Here’s a code snippet demonstrating this:
#include <iostream>
#include <vector>
#include <random>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
std::random_device rd; // Obtain a random number from hardware
std::mt19937 eng(rd()); // Seed the generator
std::uniform_int_distribution<> distr(0, vec.size() - 1); // Define the range
int randomIndex = distr(eng);
std::cout << "Random element: " << vec[randomIndex] << std::endl;
return 0;
}
Understanding the Basics of Vectors
What is a Vector?
In C++, a vector is a dynamic array that can change size during program execution. Unlike traditional arrays, vectors can grow and shrink, which makes them extremely flexible and efficient for handling collections of data. They provide easy access to their elements and support various operations such as adding or removing items.
How to Create and Initialize a Vector
Creating and initializing a vector involves using the `std::vector` template. Here’s a basic example of creating a vector:
#include <vector>
std::vector<int> myVector; // An empty vector of integers
You can initialize a vector with specific elements as follows:
std::vector<int> myVector = {1, 2, 3, 4, 5}; // Vector initialized with five integers
Vectors can also be initialized to a specific size with a given value:
std::vector<int> myVector(5, 0); // A vector of size 5, initialized with zeros
This flexibility in initialization makes vectors an ideal choice for dynamic data storage.

Random Number Generation in C++
Using the `<random>` Library
The `<random>` library in C++ provides various functionalities for generating random numbers, and it is the preferred method for producing randomness in modern C++ programming. The random number generator in this library is designed to be more powerful and offer better statistical properties than the traditional `rand()` function.
Creating a Random Number Generator
To utilize the `<random>` library, you need to include it and set up a random number generator. Here's how you can do this:
#include <random>
std::random_device rd; // Obtain a random number from hardware
std::mt19937 gen(rd()); // Seed the generator
In this code snippet, `std::random_device` is used to obtain a random seed from the hardware, and `std::mt19937` is a Mersenne Twister generator that produces high-quality pseudo-random numbers.
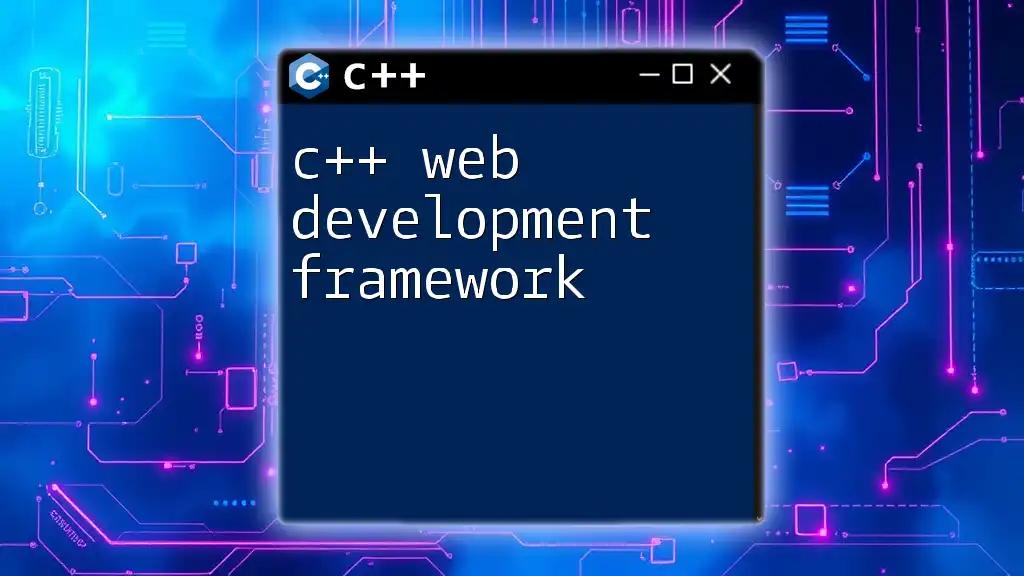
Selecting a Random Element from a Vector
Accessing Elements in a Vector
Accessing elements in a vector can be done using indexing. For instance, to access the first element of a vector:
std::vector<int> myVector = {1, 2, 3, 4, 5};
int element = myVector[0]; // Accessing the first element
It's essential to ensure that your index is in bounds to avoid runtime errors.
The Process of Getting a Random Index
To randomly select an element from a vector, you first need to generate a random index that falls within the bounds of the vector size. Here's how to do it safely:
std::uniform_int_distribution<> dis(0, myVector.size() - 1);
int randomIndex = dis(gen);
This code snippet uses `std::uniform_int_distribution` to produce a random index uniformly distributed between 0 and the last index of the vector. Remember, the last index in a vector is `size() - 1`.
Putting It All Together: Getting a Random Element
Now that you have a method for generating a random index, you can combine all of these steps into a single program that retrieves a random element:
#include <iostream>
#include <vector>
#include <random>
int main() {
std::vector<int> myVector = {10, 20, 30, 40, 50};
std::random_device rd; // Get random number from hardware
std::mt19937 gen(rd()); // Seed generator
std::uniform_int_distribution<> dis(0, myVector.size() - 1); // Distribution range
int randomElement = myVector[dis(gen)]; // Get random element
std::cout << "Random Element: " << randomElement << std::endl; // Output result
return 0;
}
In this program, we initialize a vector, create a random number generator, generate a random index, and then output the random element selected.

Common Challenges When Working with Randomness
Ensuring Unique Randomness
When retrieving random elements, you may want to ensure that each element is selected only once during a single run. One approach to achieve this is to store the indices in another data structure and remove them after selection. This prevents repetition and keeps your output varied.
Debugging Common Errors
Errors can occur during implementation, particularly related to accessing out-of-bounds elements or failing to seed the random number generator. Always check that your indices are valid and that your generator is adequately seeded to avoid predictable patterns in randomness.
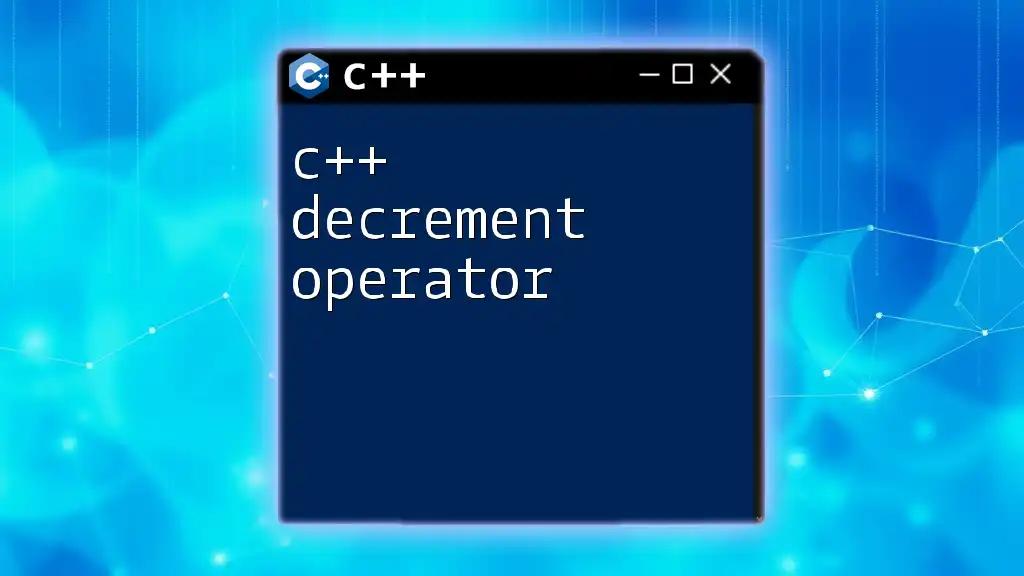
Best Practices in Random Number Generation
Seeding the Random Number Generator
Properly seeding your random number generator is crucial for achieving true randomness. Using `std::random_device` is recommended as it often provides higher-quality randomness than hardcoding a seed value.
Performance Considerations
While accessing a random element from a vector seems trivial, performance can be a concern as vector size increases. Keep in mind that both the random number generation and the access to the vector are O(1) operations, making them efficient. However, if you find the need to select random elements frequently from large datasets, consider evaluating different data structures or algorithms that can improve efficiency.
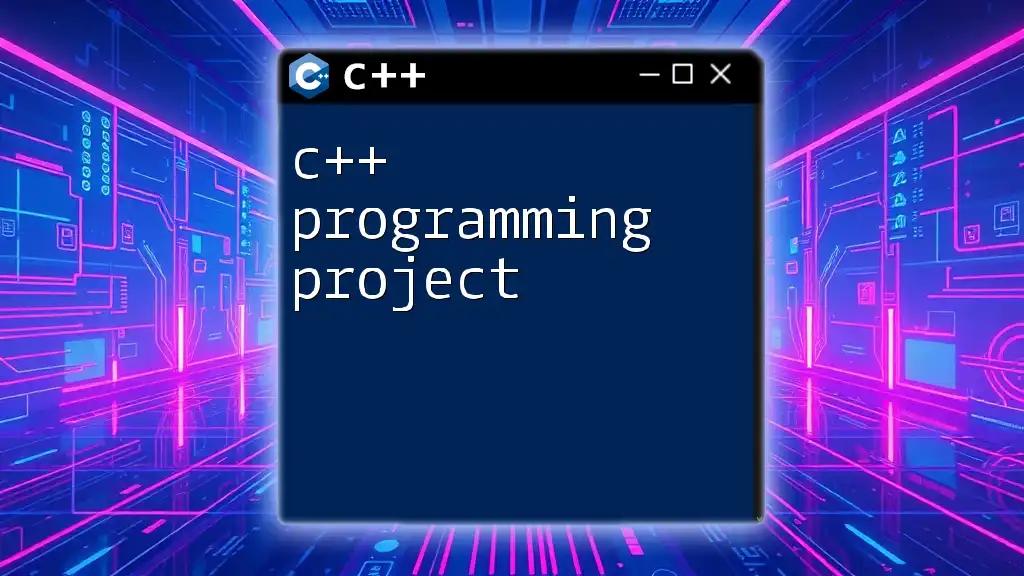
Conclusion
Retrieving a random element from a vector in C++ combines knowledge of vectors, random number generation, and careful programming practices. By understanding how to easily access elements and generate random indices, developers can leverage randomness effectively in their applications. Don't hesitate to experiment further with the techniques discussed; they can greatly enhance the functionality and user experience of your software.
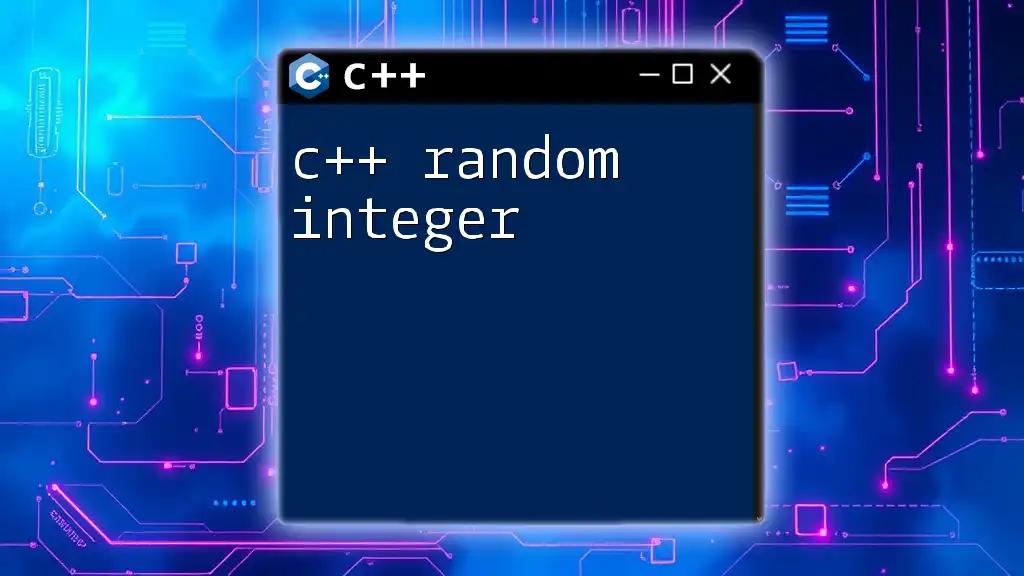
Additional Resources
For those ready to dive deeper, consult the official C++ documentation for more about `std::vector` and the `<random>` library. Exploring these resources can provide greater insight into the intricacies of C++ programming and random number generation.