To retrieve the last element of a vector in C++, you can use the `back()` method or access the last element via its index using `vector.size() - 1`.
Here's a code snippet demonstrating both methods:
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
// Method 1: Using back()
int lastElement1 = nums.back();
// Method 2: Using index
int lastElement2 = nums[nums.size() - 1];
std::cout << "Last element using back(): " << lastElement1 << std::endl;
std::cout << "Last element using index: " << lastElement2 << std::endl;
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
In C++, a vector is a dynamic array that can resize itself automatically when an element is added or removed. This makes vectors one of the most frequently used container types in C++ due to their flexibility and ease of use. Unlike regular arrays, vectors can grow and shrink as needed, making them ideal for situations where the number of elements may change unpredictably.
Basic Operations on Vectors
Vectors allow several essential operations that make them invaluable for C++ programmers:
-
Adding Elements: You can easily add elements to a vector using the `push_back()` method.
-
Removing Elements: Elements can be removed using the `pop_back()` method, which removes the last element, or `erase()` for specific elements.
-
Accessing Elements: You can access elements through indexing, similar to arrays.

Accessing the Last Element of a Vector in C++
One of the most common tasks in programming with vectors is accessing the last element. There are two primary methods for achieving this: using the `size()` method and using the `back()` method.
Using Size Method
The `size()` function returns the number of elements in the vector. This method allows you to access the last element by calculating the index, which is `size() - 1`. Here's a quick code example demonstrating this approach:
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
int lastElement = nums.size() > 0 ? nums[nums.size() - 1] : -1; // Check for empty vector
std::cout << "Last Element: " << lastElement << std::endl;
return 0;
}
In this snippet, we check whether the vector is empty before attempting to access the last element. This precaution prevents undefined behavior, which can occur when you try to access elements in an empty vector.
Using `back()` Method
The `back()` method is a more straightforward and efficient way to access the last element. This method does not require you to manually check the size beforehand since it handles this internally. Here’s a code example using the `back()` method:
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
int lastElement = nums.back(); // No need to check size
std::cout << "Last Element: " << lastElement << std::endl;
return 0;
}
In this case, calling `nums.back()` returns the last element directly, making the code more concise and easier to read.
Safety Considerations
Checking for Empty Vectors
When working with vectors, it's critical to consider the possibility that a vector may be empty. Accessing the last element of an empty vector can lead to undefined behavior, which often manifests as a program crash or erroneous outputs. Always ensure that the vector has elements before accessing the last element.

Comparison of Methods to Get Last Element of Vector in C++
Performance Analysis
Both the `size()` method and the `back()` method provide efficient ways to access the last element, but there are distinctions:
-
Time Complexity: Accessing the last element through either method operates in constant time O(1). However, `back()` is often preferred due to its simplicity and directness.
-
Use Cases: If you already need to use the size for other operations, then using `size()` might make sense, but for purely accessing the last element, `back()` is the cleaner choice.
Best Practices
When writing production code, adhere to best practices:
-
Use `back()` when you need to access the last element, as it avoids unnecessary calculations and improves code readability.
-
Always validate that the vector is not empty before accessing elements, particularly when using the `size()` method.
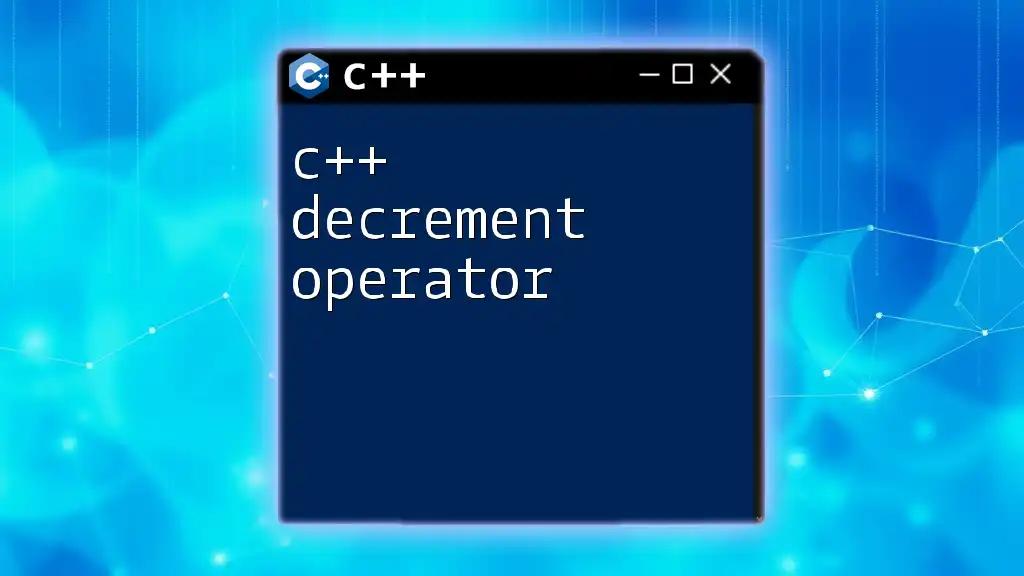
Conclusion
Accessing the last element of a vector in C++ is a fundamental operation that can be accomplished using methods like `size()` and `back()`. Each method has its benefits, but for simplicity and clarity, `back()` is often the best option. Make sure to handle empty vectors appropriately to avoid potential pitfalls.
As you continue to dive deeper into C++, practicing these techniques will significantly strengthen your programming skills. Consider exploring additional resources and tutorials to expand your understanding of vectors and their applications in various scenarios.