In C++, you can remove an element from a `set` using the `erase()` method, which takes the value of the element you want to remove as its argument.
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
mySet.erase(3); // Removes the element with value 3
for (int element : mySet) {
std::cout << element << " ";
}
return 0;
}
Understanding C++ Sets
What is a Set in C++?
A set in C++ is a collection of unique elements that are stored in a specific order, usually sorted. The primary characteristics of a set include:
- Uniqueness: Each element can only appear once; duplicates are not allowed.
- Automatic sorting: Elements are kept in a sorted order, which facilitates efficient searching and access.
- Dynamic resizing: You can add or remove elements at runtime, making sets flexible to work with.
Sets are particularly useful in scenarios where you need to maintain a collection of unique items, such as managing user IDs or a collection of distinct objects.
The Set Data Structure
C++ sets are typically implemented as balanced binary search trees (most commonly Red-Black Trees). This allows for operations like insertions, deletions, and lookups to be performed in O(log n) time complexity.
Pros of Using Sets:
- Ensures elements are unique, which is beneficial for data integrity.
- Automatic sorting for faster access to the smallest or largest element.
Cons of Using Sets:
- Slightly more overhead in terms of memory usage compared to other containers like vectors.
- Random access is not possible, as sets are not indexed like arrays or vectors.
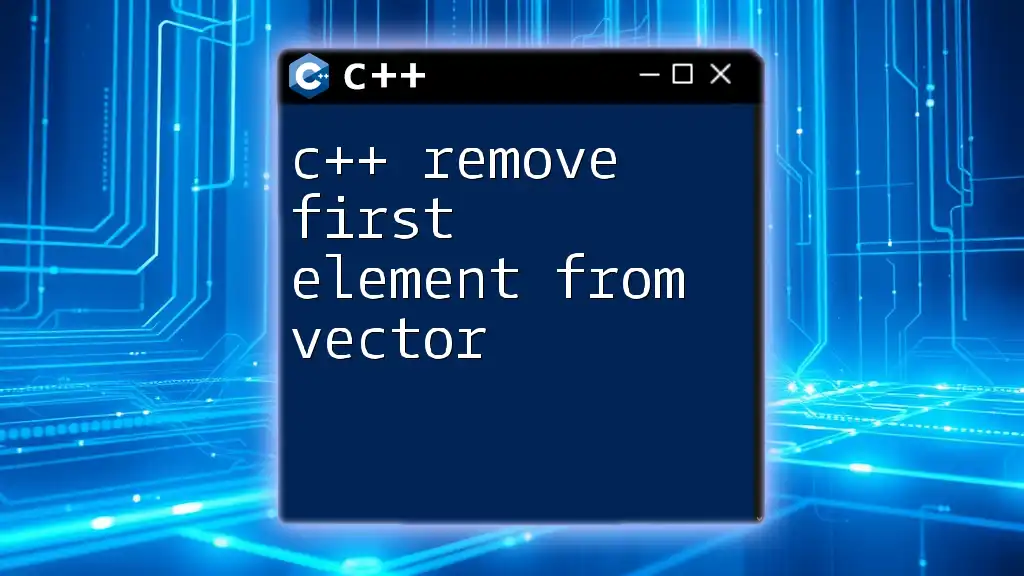
The `remove` Operation
What Does "Remove" Mean?
In the context of a C++ set, to remove an element means to delete it from the collection. When an element is removed, the internal structure of the set adjusts to ensure the property of uniqueness and order is maintained.
Syntax of the `erase()` Function
To remove an element from a set, you utilize the `erase()` function. The syntax is as follows:
set_name.erase(element);
This function directly removes the specified element from the set if it exists.
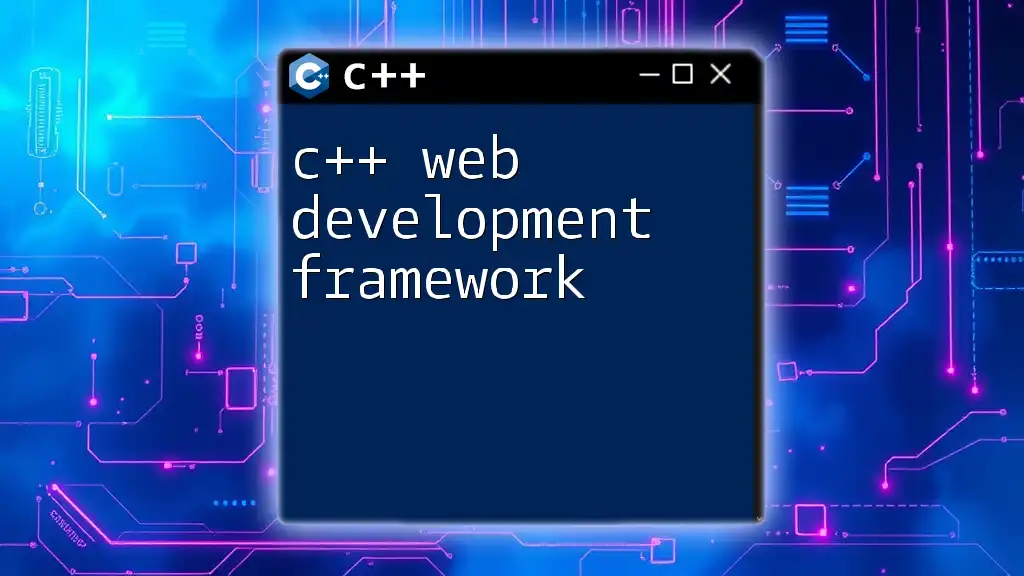
Removing Elements from a Set
Removing a Single Element
To remove a single element from a set, simply call the `erase()` function with the element you wish to remove as an argument. Here’s a code snippet demonstrating this:
#include <iostream>
#include <set>
using namespace std;
int main() {
set<int> mySet = {1, 2, 3, 4, 5};
mySet.erase(3); // Removes the element '3'
cout << "Set after removal: ";
for (const auto &elem : mySet) {
cout << elem << " ";
}
return 0;
}
In this example, you see that after calling `mySet.erase(3)`, the element `3` is removed. The output will demonstrate that the set contains `{1, 2, 4, 5}` after the removal.
Attempting to Remove Non-Existent Elements
When you try to remove an element that doesn't exist in the set, the `erase()` function does not throw an error. Instead, it simply has no effect:
mySet.erase(6); // No effect, as '6' is not in the set
This behavior makes it safe to attempt to remove elements without needing to check first whether they are present in the set.
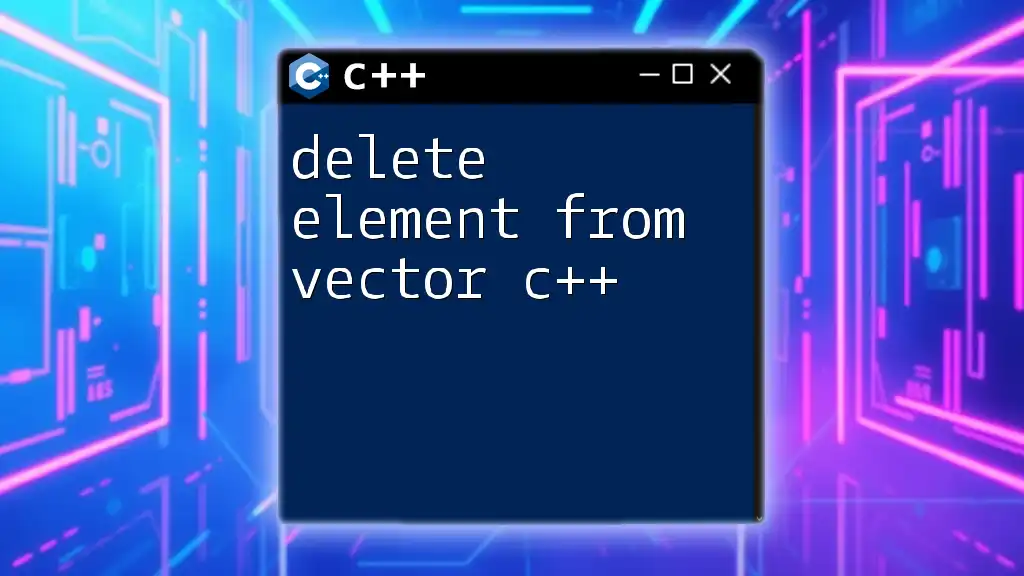
Remove Multiple Elements
Removing All Elements
If you wish to clear all the elements from a set, you can use the `clear()` function. For instance:
mySet.clear(); // Removes all elements from the set
After this operation, the set will be empty, and you can confirm it by checking its size using `mySet.size()`.
Removing Elements with a Range
You can also remove a range of elements from a set using iterators. Consider this code:
auto it = mySet.find(1); // Find the position of '1'
mySet.erase(it, mySet.end()); // Removes elements from '1' to end
Here, `mySet.erase(it, mySet.end())` will remove all elements starting from the position of `1` up to the end of the set. Keep in mind that the iterators must point to valid elements within the set.
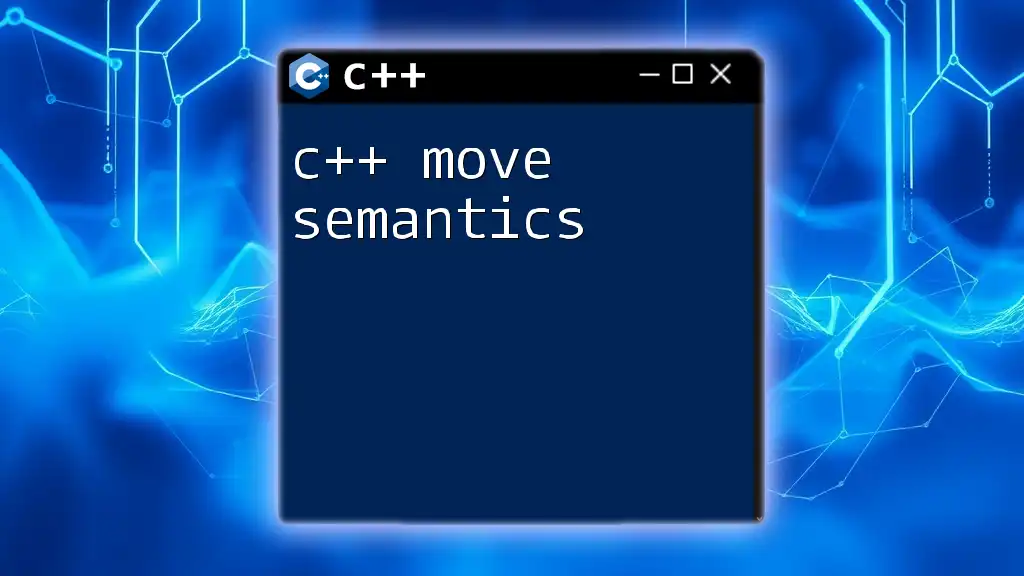
Checking if an Element Exists Before Removal
Using the `find()` Function
Before removing an element, you might want to check whether it exists. You can achieve this using the `find()` function:
if (mySet.find(2) != mySet.end()) {
mySet.erase(2); // Remove only if found
}
This snippet will ensure that you only attempt to erase `2` if it exists in the set. This practice helps in maintaining code robustness, particularly when interacting with large datasets.
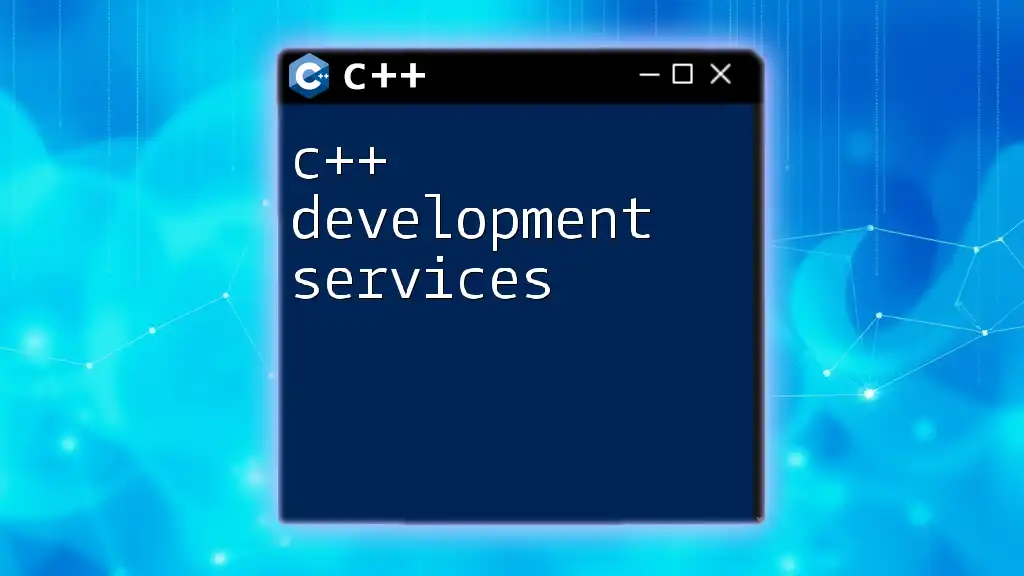
Performance Considerations
Amortized Time Complexity
The time complexity for the `erase()` operation in a set is O(log n). This means that even as the size of the set grows, the time taken to remove an element will not increase dramatically. In contrast, removing an element from a vector could be O(n) since it may require shifting elements.
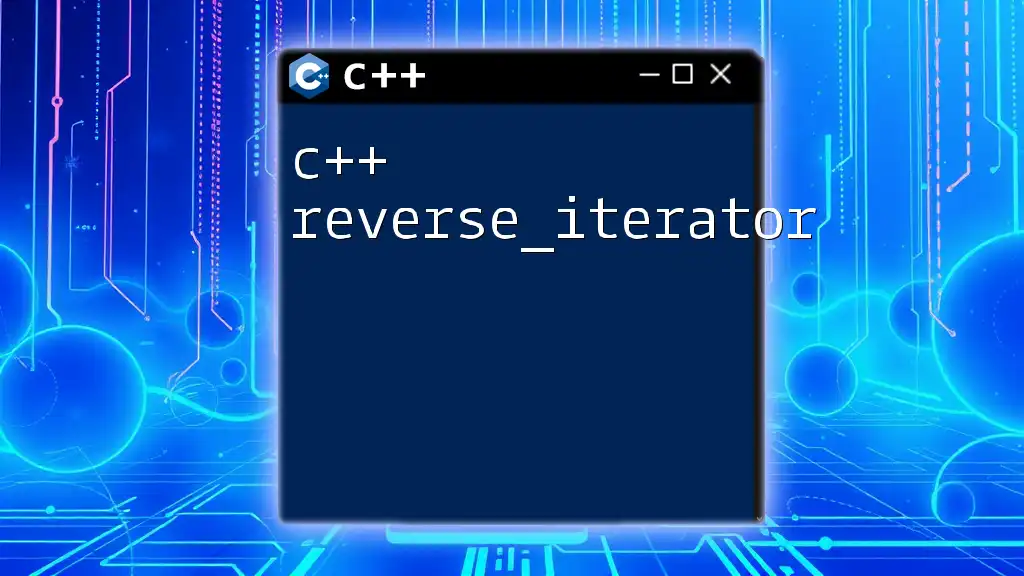
Common Pitfalls and Best Practices
Avoiding Invalid Iterators
One common mistake when removing elements from a set involves the invalidation of iterators. When you erase an element, any iterators pointing to it or iterators following it may no longer be valid. To mitigate this, always re-evaluate iterator positions after an erase operation, or use a loop wherein you check for the next position before erasing.
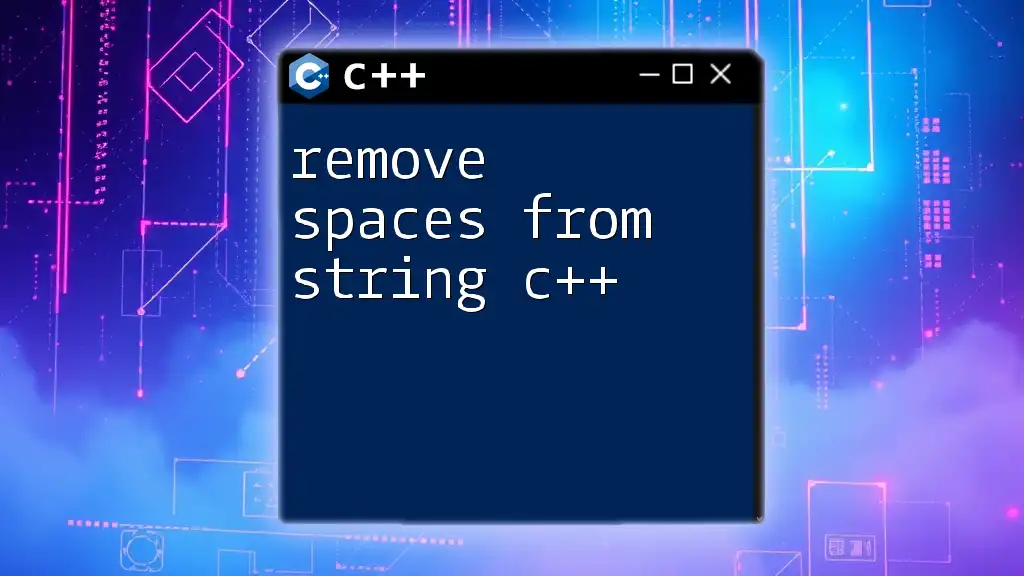
Conclusion
Knowing how to effectively remove elements from a set in C++ is a critical skill for developers. It aids in maintaining clean, efficient code and ensures data integrity by managing unique values. By mastering the `erase()` function and understanding its behavior, you can leverage the power of C++ sets in a variety of programming scenarios. For more in-depth tutorials and practice exercises, be sure to explore additional resources!