To remove a file in C++, you can use the `remove` function from the C Standard Library, which deletes the specified file if it exists.
Here’s an example code snippet:
#include <cstdio> // Include the C standard library header
int main() {
const char* filename = "example.txt"; // Specify the file to be removed
if (remove(filename) == 0) {
printf("File removed successfully.\n");
} else {
perror("Error removing file"); // Display an error message if removal fails
}
return 0;
}
Understanding File Deletion in C++
Deleting a file in C++ involves removing its reference from the file system so that it becomes inaccessible. This action is crucial for various programming scenarios, including cleaning up temporary files, freeing up disk space, or removing unnecessary files after program execution.
Common use cases for deleting files include:
- Temporary data management: Many applications create temporary files during operation. Removing them improves performance and saves storage.
- User-driven clean up: Programs that allow users to manage and organize their files need effective mechanisms to delete unnecessary files.
- Data privacy: Programs handling sensitive information often require files to be deleted securely.
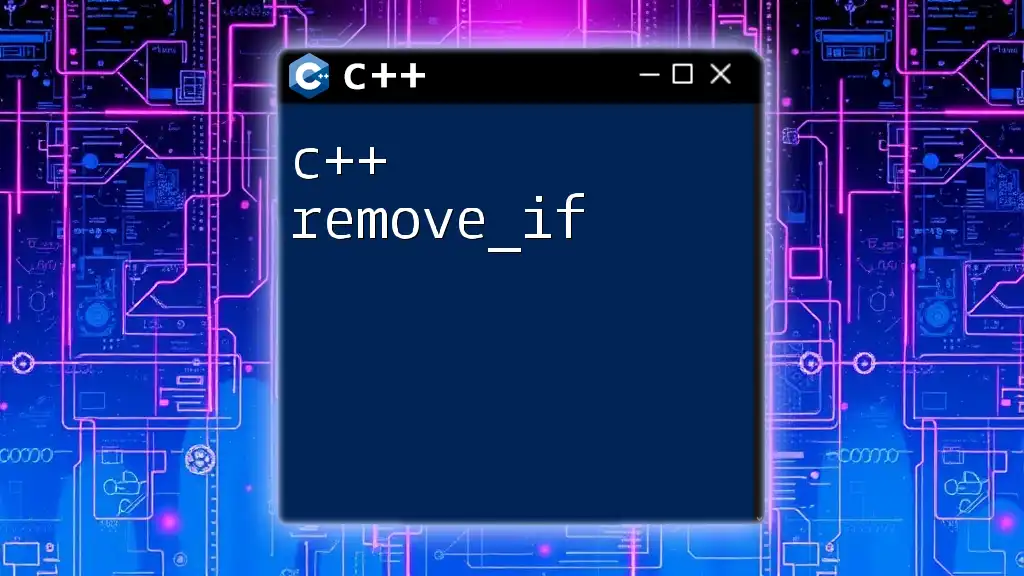
The C++ Library for File Manipulation
To manipulate files in C++, you will primarily work with the `<cstdio>` header. This header provides a range of functions for file operations, including those for file deletion. It is essential to ensure that the correct headers are included in your program to avoid compilation issues.
In addition to `<cstdio>`, you might also require `<iostream>` for basic input and output operations, especially when dealing with error handling or user messages.
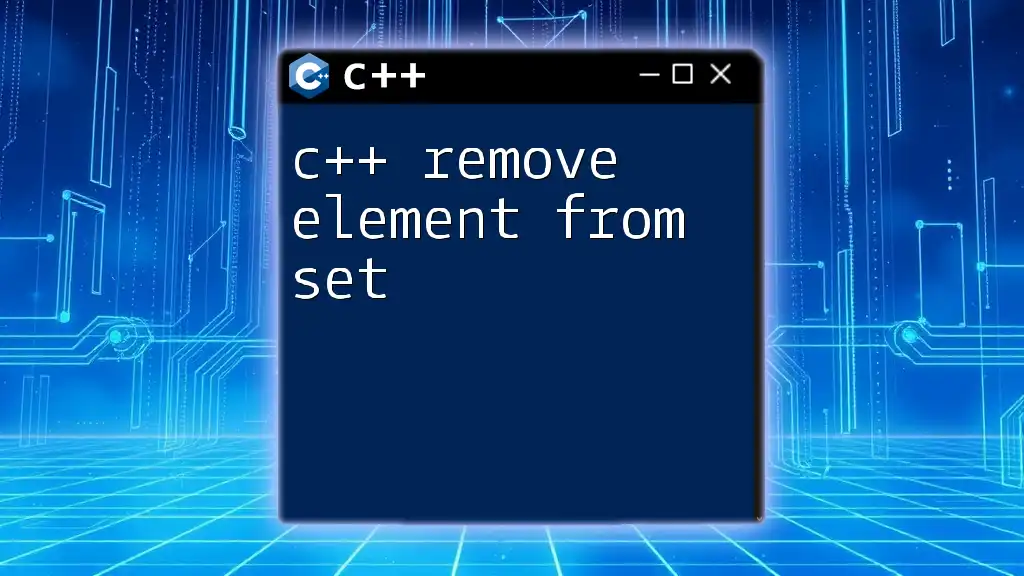
Using `std::remove` to Delete a File
Overview of `std::remove`
The `std::remove` function is the standard method to delete files in C++. It takes a single parameter, which is the name of the file to be deleted, and returns an integer indicating success or failure.
Syntax:
int remove(const char *filename);
Step-by-step Example
Here’s a simple example demonstrating how to delete a file using `std::remove`:
#include <cstdio>
#include <iostream>
int main() {
const char* filename = "example.txt";
if (std::remove(filename) != 0) {
perror("Error deleting file");
} else {
std::cout << "File successfully deleted\n";
}
return 0;
}
In this code snippet, the program attempts to delete the file named `example.txt`. If the operation fails, it uses `perror` to print an error message that describes why the deletion could not be completed. On success, it outputs a confirmation message.
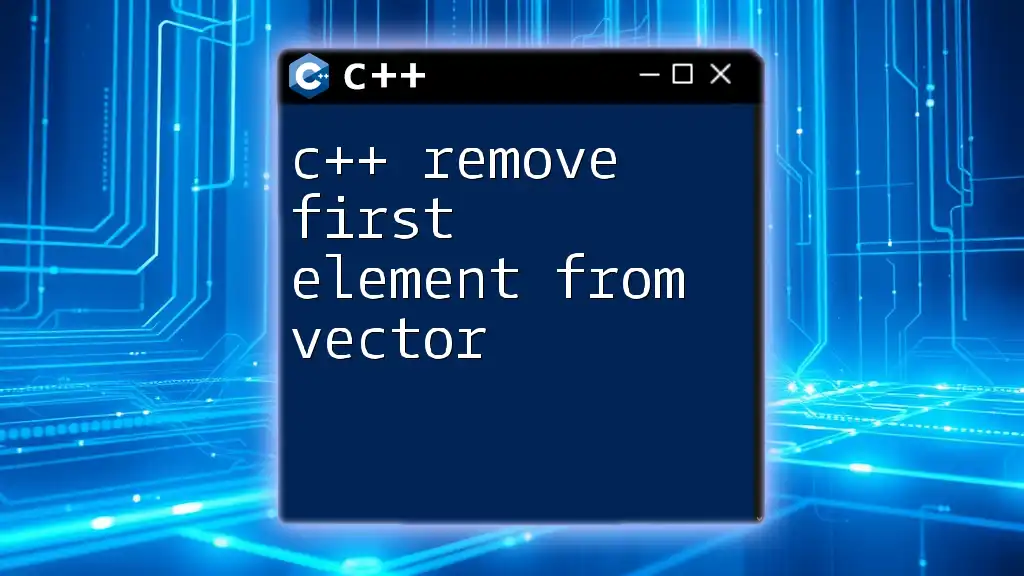
Error Handling When Deleting Files
Common Errors When Using `std::remove`
When working with file deletion, several errors might occur. Some common issues include:
- File does not exist: Attempting to delete a non-existent file results in an error.
- Permission denied: Lack of permissions to delete a file will cause the function to fail.
Implementing Error Handling in Your Code
To effectively manage errors, it's best to structure your delete operation with clear error messages. Here’s an updated version of the previous example that includes this error handling:
#include <cstdio>
#include <iostream>
void deleteFile(const char* filename) {
if (std::remove(filename) != 0) {
perror("Error deleting file");
} else {
std::cout << "File successfully deleted\n";
}
}
By encapsulating the delete operation into a function, you make it reusable across your program. This improves organization and allows centralized error handling.
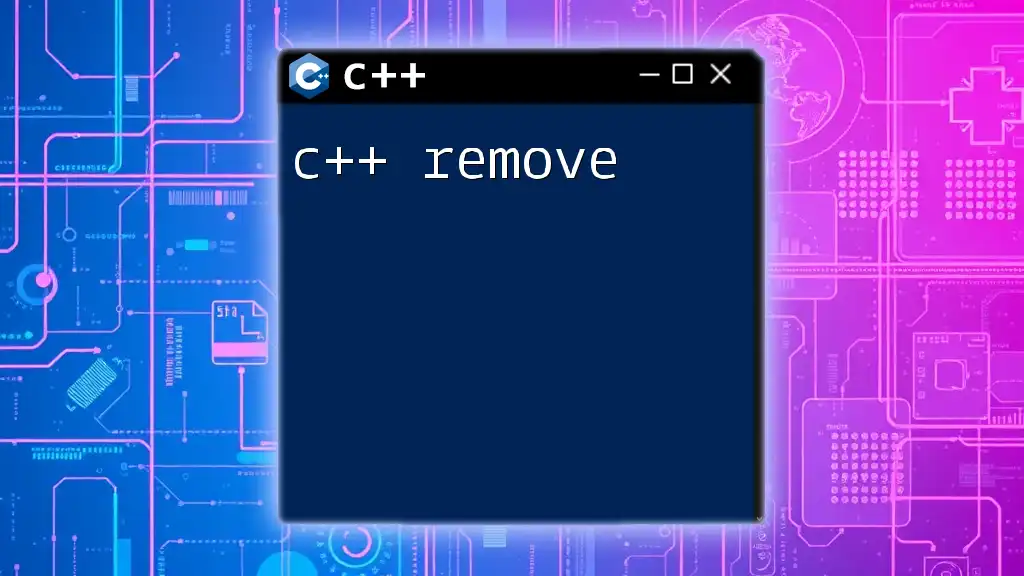
Best Practices for Deleting Files in C++
Ensure File Existence Before Deletion
Before attempting to delete a file, it's prudent to check if it exists. This practice helps prevent unnecessary error messages and improves user experience. Here’s how you can accomplish this:
#include <fstream>
#include <cstdio>
#include <iostream>
bool fileExists(const char* filename) {
std::ifstream file(filename);
return file.good();
}
int main() {
const char* filename = "example.txt";
if (fileExists(filename)) {
deleteFile(filename);
} else {
std::cout << "File does not exist. Cannot delete.\n";
}
return 0;
}
In this code, the `fileExists` function checks for the file's presence before calling `deleteFile`. If the file doesn't exist, it outputs a friendly message instead of trying to delete it.
Avoiding Duplicate Deletion Attempts
Implementing flags or states can effectively manage whether a file has already been marked for deletion or not. By checking these flags, you can prevent multiple attempts to delete the same file, which helps minimize errors and improves program robustness.
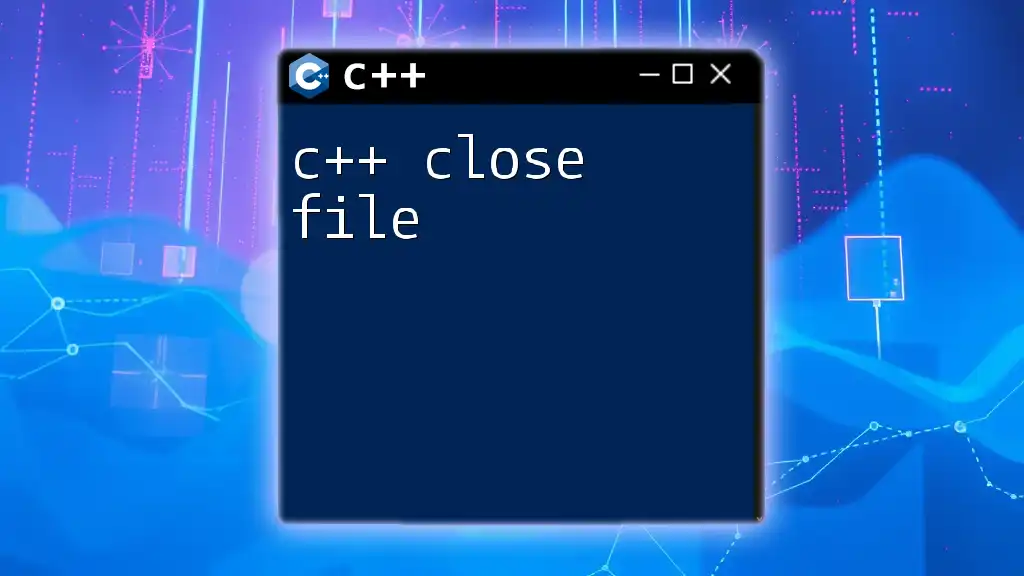
Alternative Methods for File Deletion
Using C++ File Streams (fstream)
You can also use C++ file streams to manage file deletion. Using `std::ifstream` to check for file existence or `std::ofstream` to create a reference is another approach. Here’s an example:
#include <fstream>
#include <cstdio>
#include <iostream>
void deleteFileUsingStream(const char* filename) {
std::ofstream file(filename);
if (!file) {
std::cout << "File does not exist.\n";
return;
}
file.close();
if (std::remove(filename) == 0) {
std::cout << "File deleted using fstream\n";
} else {
perror("Error deleting file");
}
}
In this example, before deleting the file, the program creates an output stream. The attempt to create an instance of `std::ofstream` checks the file's existence indirectly.
Pros and Cons of Various Methods
While `std::remove` is direct and straightforward, using file streams can provide additional context and control over file read/write operations. Each method has its advantages, and your choice depends on specific requirements within your application.
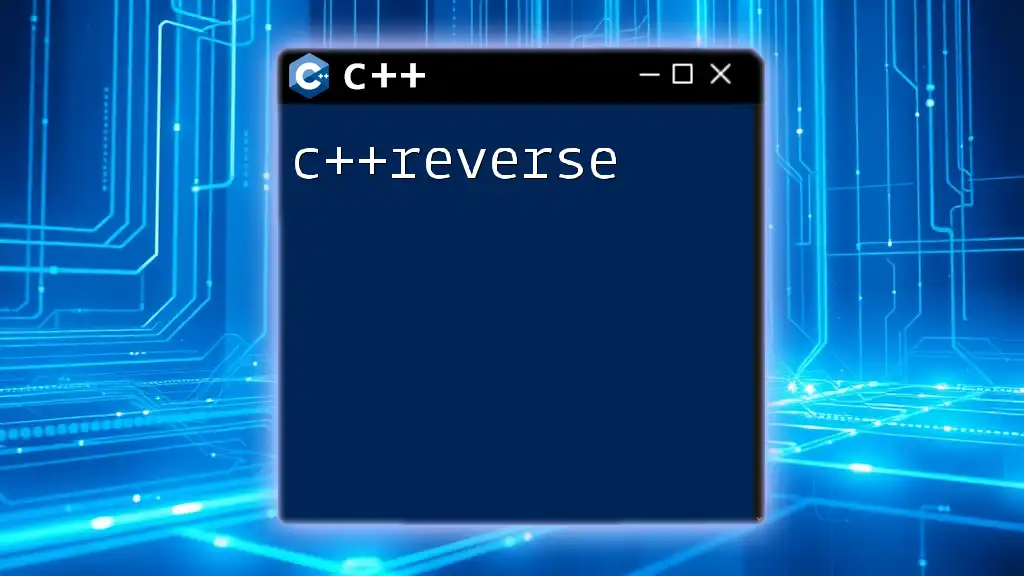
Conclusion
In summary, removing files in C++ using `std::remove` is straightforward when implemented with proper error handling and checking for file existence. By learning these concepts, you'll ensure that your programs are not only efficient but also user-friendly.
Effective file management, including deletion, is a fundamental skill in C++ programming. Practicing file manipulation will enhance your understanding of how file systems work, and it is crucial for responsible programming, especially when dealing with sensitive information or large amounts of data.
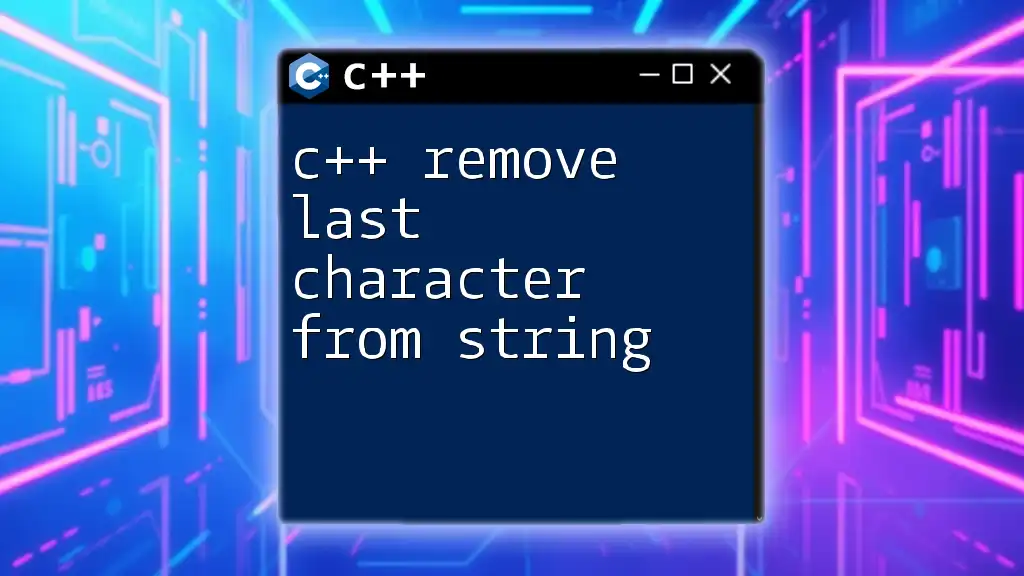
Frequently Asked Questions (FAQs)
What happens if you try to delete a file that doesn't exist?
If you attempt to delete a non-existent file, the `std::remove` function will return a non-zero value, indicating an error, and `perror` will display an appropriate error message related to the failure.
Can I delete multiple files at once in C++?
While `std::remove` only deletes one file at a time, you can loop through an array or vector of filenames and call `std::remove` for each file. This method provides flexibility in managing multiple deletions.
Is there a way to recover deleted files in C++?
Generally, C++ does not provide built-in mechanisms to recover deleted files once they have been removed using `std::remove`. File recovery relies on the underlying file system and may involve specialized software outside the C++ programming capability.
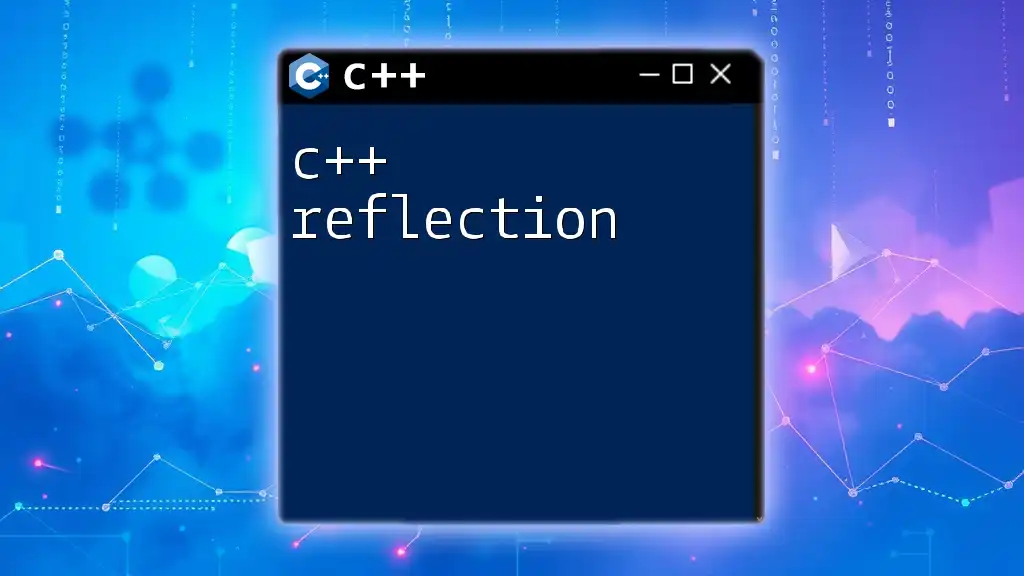
Additional Resources
For further reading on file operations in C++, consider exploring the official C++ documentation or engaging with programming communities that share valuable insights on file management techniques.