To remove the last character from a string in C++, you can use the `pop_back()` method which effectively reduces the string size by one character. Here's an example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
str.pop_back(); // Removes the last character
std::cout << str << std::endl; // Outputs: Hello, World
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to represent textual data. The C++ Standard Library provides the `std::string` class, which simplifies string manipulation and memory management compared to traditional character arrays.
Common String Operations
C++ strings support a variety of operations, including concatenation, comparison, and modification. Being familiar with these operations, particularly ones that involve removing characters, can streamline your programming tasks significantly.
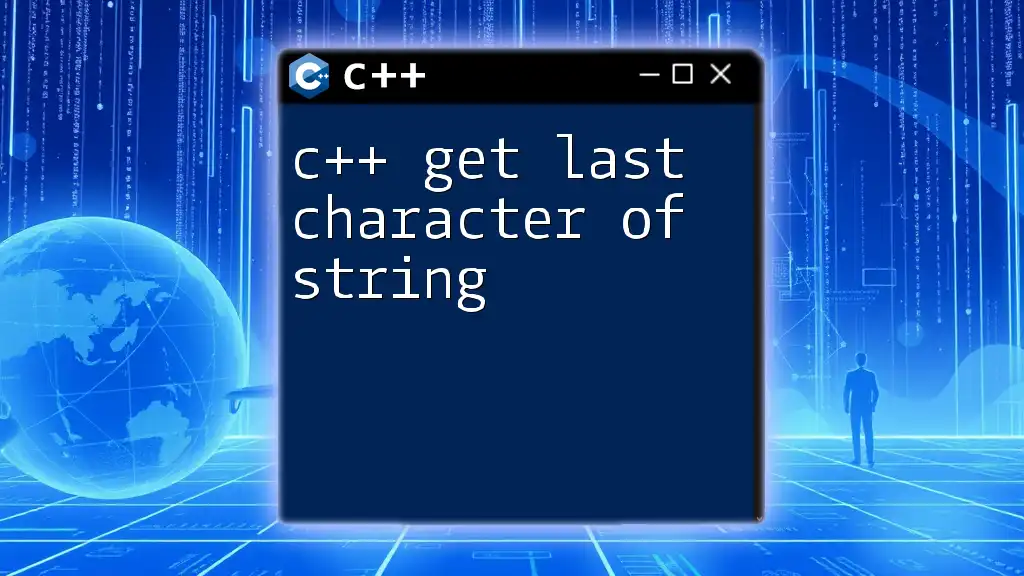
Methods to Remove the Last Character from a String
Why Remove the Last Character?
Removing the last character from a string can be crucial in several scenarios:
- Data Validation: Ensuring that user input adheres to certain formats, typically by eliminating unwanted characters like trailing spaces or punctuation.
- Text Processing: Preparing strings for further manipulation or display by refining their content.
Understanding the methods available to remove the last character from a string will enhance your ability to manipulate strings effectively.
Method 1: Using `std::string::pop_back()`
How it Works
The `pop_back()` function is a straightforward and efficient way to remove the last character from a `std::string`. It simply removes the last character in the string and does not return any value. However, you should ensure that the string isn't empty before using this method to avoid runtime errors.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
str.pop_back();
std::cout << str; // Output: Hello World
return 0;
}
Explanation of the Code
In the code above:
- We include the necessary headers for input and string manipulation.
- We declare a string variable `str` and initialize it with "Hello World!".
- By calling `pop_back()`, we remove the last character ('!') from the string.
- Finally, we print the modified string, showing "Hello World".
Method 2: Using `std::string::erase()`
Understanding `erase()`
The `erase()` function offers flexibility by allowing you to specify the position and number of characters you wish to remove. To remove the last character, you can provide the index of the last character along with the number of characters to erase.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
str.erase(str.size() - 1);
std::cout << str; // Output: Hello World
return 0;
}
Explanation of the Code
In this example:
- We again use the necessary headers.
- We create a string variable `str` with the same initial value.
- The call to `str.erase(str.size() - 1)` removes one character starting from the index of the last character (calculated using `str.size() - 1`).
- The modified string, now "Hello World", is printed.
Method 3: Using Substring
What is a Substring?
The substring method involves creating a new string that consists of a portion of the original string, effectively allowing you to exclude unwanted characters. This approach is particularly useful when you need to maintain the state of the original string while creating a modified version.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
str = str.substr(0, str.size() - 1);
std::cout << str; // Output: Hello World
return 0;
}
Explanation of the Code
In this code snippet:
- The string `str` is initialized as before.
- The `substr()` method is invoked, which takes two parameters: the starting index (0 in this case) and the length of the substring (the original size minus one).
- This creates a new string containing everything except the last character, which replaces the original string stored in `str`.
- The output remains "Hello World".
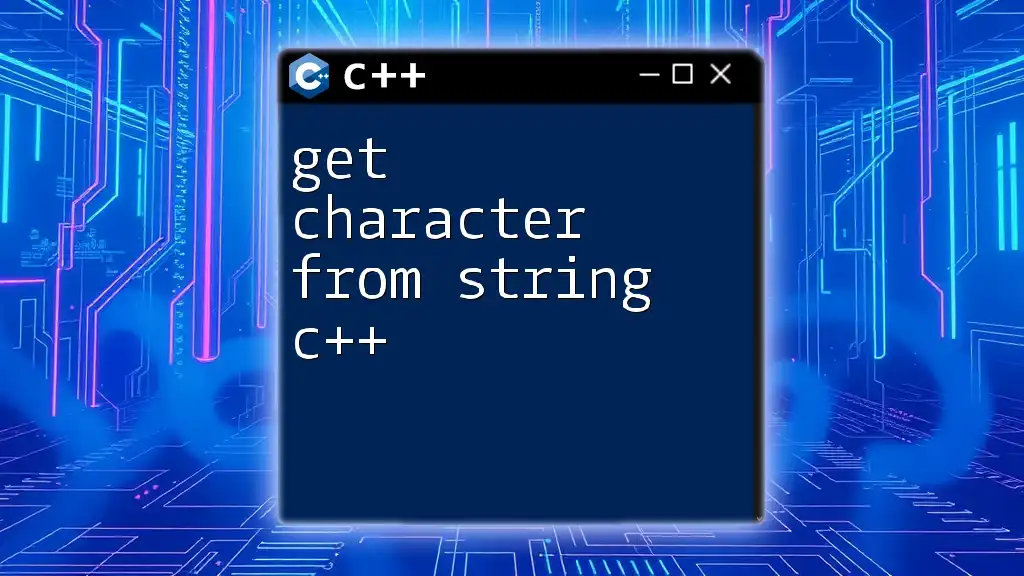
Considerations When Removing Characters
Handling Empty Strings
It's crucial to check the length of the string before attempting to remove a character to prevent exceptions. If you try to remove a character from an empty string, your program may crash. Safeguard your code with conditions like:
if (!str.empty()) {
str.pop_back();
}
Performance Considerations
When evaluating which method to use for removing the last character, consider both efficiency and readability.
- `pop_back()` is generally the most efficient, as it directly modifies the string.
- The `erase()` and substring methods may involve additional overhead due to memory allocations, especially for long strings, as they may create copies of the string.
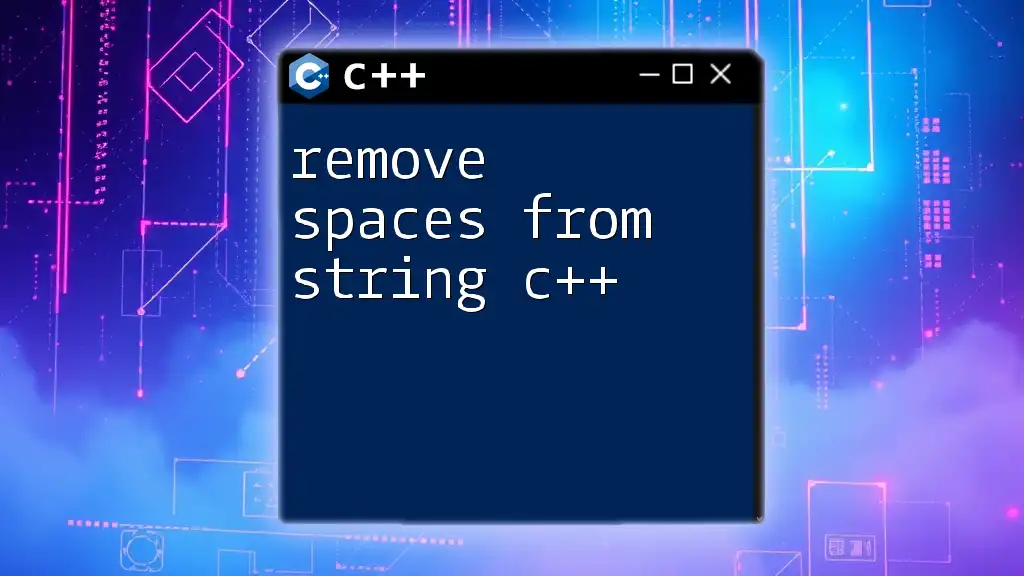
Conclusion
Being adept at string manipulation in C++ can significantly enhance your programming repertoire. This guide has provided you with various methods to remove the last character from a string— `pop_back()`, `erase()`, and `substr()`. Each method has its advantages and use cases, so choose one based on the specific requirements of your application. Practicing these methods with different scenarios will solidify your understanding and proficiency in handling strings.
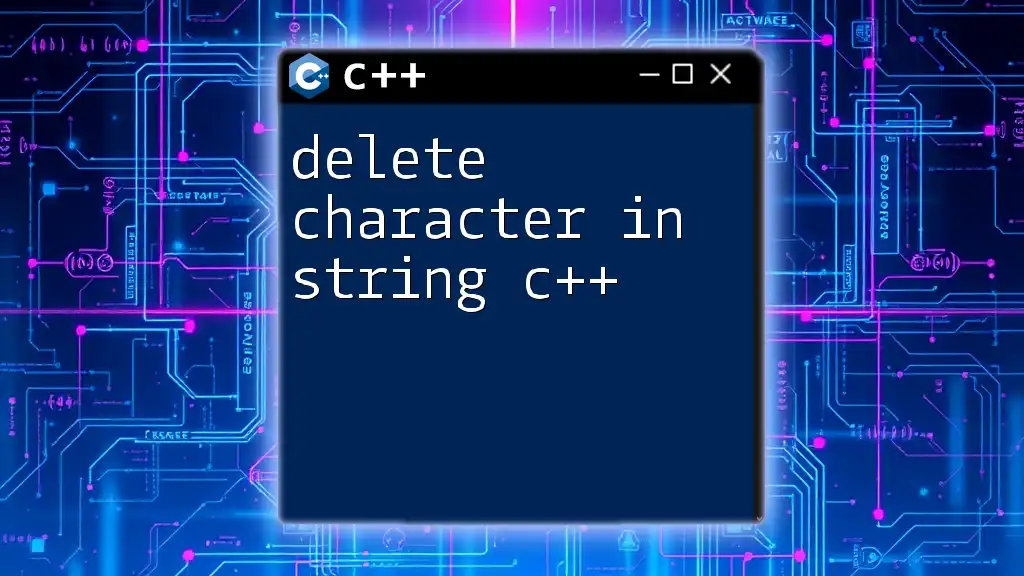
Additional Resources
Further Reading
- Explore the official C++ documentation to dive deeper into string methods. Familiarizing yourself with the Standard Library can empower you to write more efficient code.
Tutorials and Online Courses
- Consider enrolling in online platforms that offer C++ tutorials to enhance your learning journey. Whether you prefer hands-on coding or theoretical explanations, there’s a wealth of resources available to assist you.