In C++, you can add characters to a string by using the `push_back()` method or the `+=` operator to concatenate the character to the existing string.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
str.push_back('!'); // Using push_back
// or alternatively
// str += '?'; // Concatenating using +=
std::cout << str << std::endl; // Output: Hello!
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to represent text. A string can be defined in two primary ways:
- C-style strings: These are arrays of characters terminated by a null character (`'\0'`). While they are fundamental to C and early C++ programs, they require manual memory management and can lead to issues like buffer overflows.
- `std::string`: This is a part of the C++ Standard Library that provides a convenient and safe way to work with strings. It handles memory management automatically, making it less error-prone.
Why Use `std::string`?
Using `std::string` offers several advantages over C-style strings:
- Memory Management: `std::string` automatically allocates and deallocates memory as needed. You don't have to worry about memory leaks or buffer overflows.
- Functionality: `std::string` comes with numerous built-in methods that allow for versatile string manipulation without additional overhead or complexity.
- Ease of Use: The syntax for string manipulation with `std::string` is clear and intuitive.
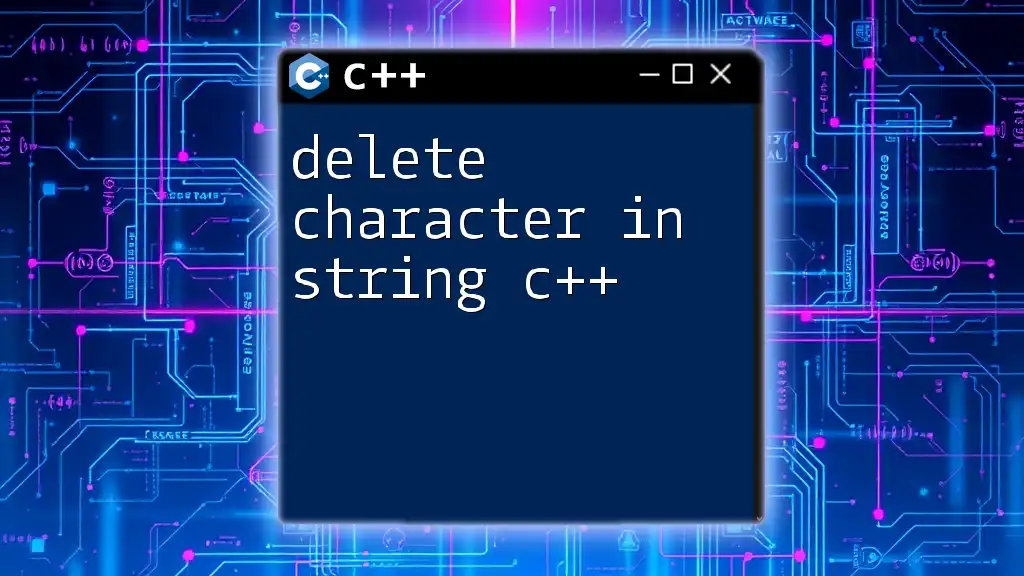
Methods to Add Characters to a String
Using the `push_back()` Method
The `push_back()` method is a simple way to add a single character to the end of a string. This method is intuitive and frequently used for building strings incrementally.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello";
myString.push_back(' ');
myString.push_back('W');
myString.push_back('o');
myString.push_back('r');
myString.push_back('l');
myString.push_back('d');
std::cout << myString << std::endl; // Output: Hello World
return 0;
}
In this example, we start with the string `"Hello"`. As we use the `push_back()` method, spaces and additional characters are sequentially appended to create the final output: "Hello World".
Using the `+=` Operator
Another common method for adding characters is by using the `+=` operator. This operator allows you to concatenate additional characters or strings with ease.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Good";
greeting += ' ';
greeting += 'M';
greeting += 'o';
greeting += 'r';
greeting += 'n';
greeting += 'i';
greeting += 'n';
greeting += 'g';
std::cout << greeting << std::endl; // Output: Good Morning
return 0;
}
In this snippet, we initialize `greeting` with "Good" and then use the `+=` operator to add a space and each subsequent character. The result is "Good Morning", demonstrating the operator's effectiveness for string concatenation.
Using the `append()` Method
The `append()` method allows you to add a string or multiple characters to the end of an existing string, making it another versatile choice for string manipulation.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string text = "C++ is";
text.append(" awesome!");
std::cout << text << std::endl; // Output: C++ is awesome!
return 0;
}
As shown in this code, we begin with "C++ is" and utilize `append()` to add " awesome!". This method is particularly useful when you want to concatenate larger segments of text in a single call.
Inserting Characters at a Specific Position
Sometimes, you may want to insert characters at a specific index within a string. The `insert()` method provides this functionality.
Using the `insert()` Method
The `insert()` method accommodates the insertion of characters or substrings at any specified location within the string.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string phrase = "hello";
phrase.insert(5, " world");
std::cout << phrase << std::endl; // Output: hello world
return 0;
}
In this example, "hello" is modified by inserting " world" at index 5. The functionality of `insert()` allows for dynamic string construction even after the initial value has been set.
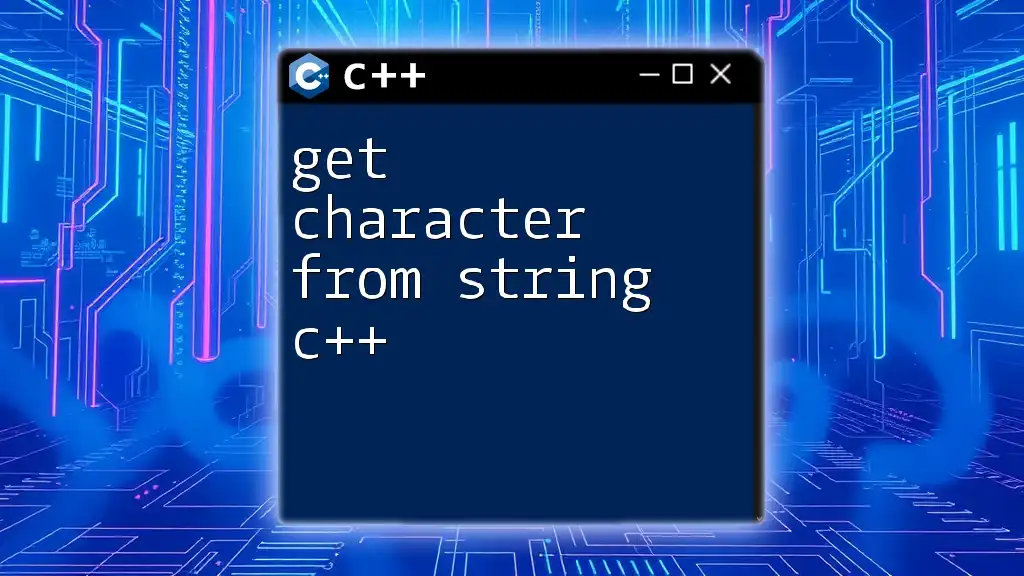
Summary
Understanding how to add characters to a string in C++ is essential for effective string manipulation. The `push_back()`, `+=`, and `append()` methods offer streamlined, practical means of enhancing strings, while `insert()` provides great flexibility for positioning characters.
Each of these methods has specific use cases that can improve your programming efficiency. Whether you are incrementally building strings or combining segments of text, choosing the appropriate method can save time and prevent errors.
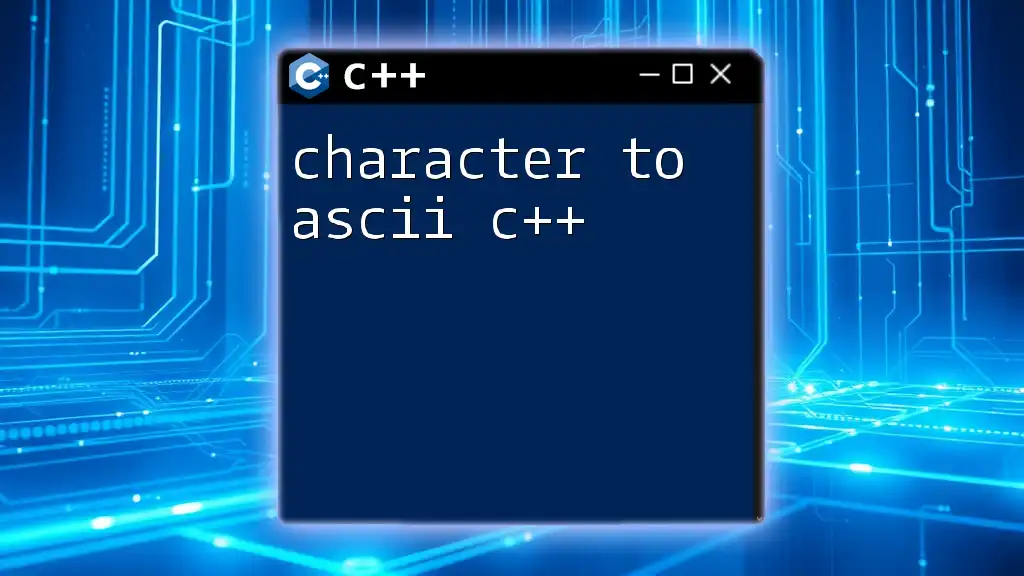
Additional Resources
For further exploration into the topic of strings, you can refer to the C++ documentation on the Standard Template Library (STL). Various online platforms and books provide in-depth materials, exercises, and examples for those seeking to master string manipulation in C++.
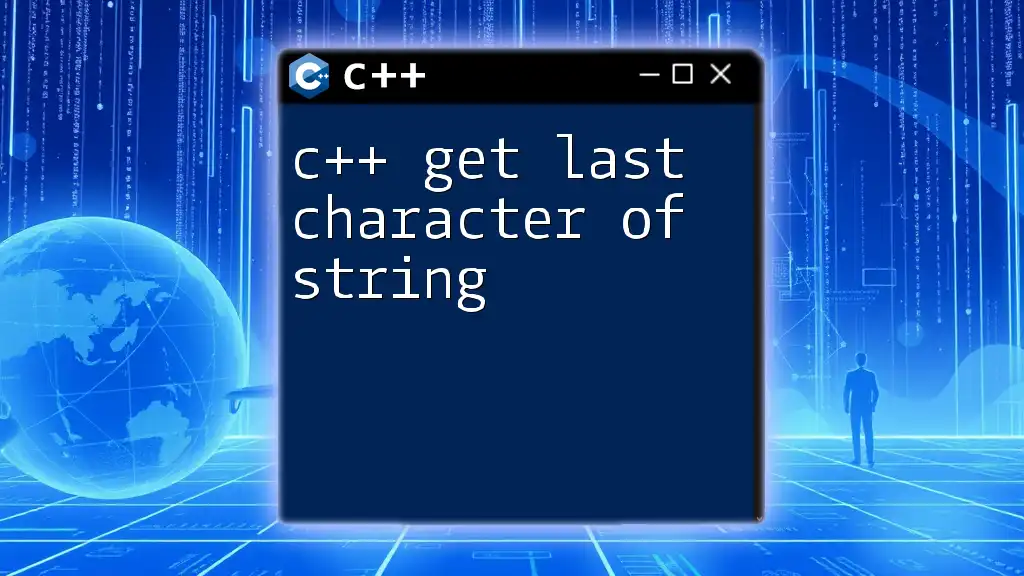
Conclusion
Mastering how to add characters to a string in C++ not only enhances your programming skills but also opens the door for more sophisticated data handling. Engaging with these string manipulation techniques will equip you to tackle various coding challenges with confidence. Share your experiences or questions about string operations as you delve deeper into the world of C++ programming!