In C++, you can concatenate a string and an integer by converting the integer to a string using `std::to_string()` and then using the `+` operator to combine them.
#include <iostream>
#include <string>
int main() {
std::string str = "The number is: ";
int num = 42;
std::string result = str + std::to_string(num);
std::cout << result << std::endl;
return 0;
}
Understanding Data Types in C++
What is a String in C++?
In C++, a string is a sequence of characters that can be manipulated and used just like other data types. C++ provides a built-in class called `std::string`, which is part of the Standard Library, to handle strings conveniently. String data types allow you to perform various operations, such as concatenation, substring extraction, and transformation.
What is an Integer in C++?
An integer is a data type that represents whole numbers, both positive and negative. In C++, integers can come in various sizes, including `int`, `short`, and `long`, depending on the range of values needed. Understanding the difference between these types is crucial, as it can impact memory usage and performance.
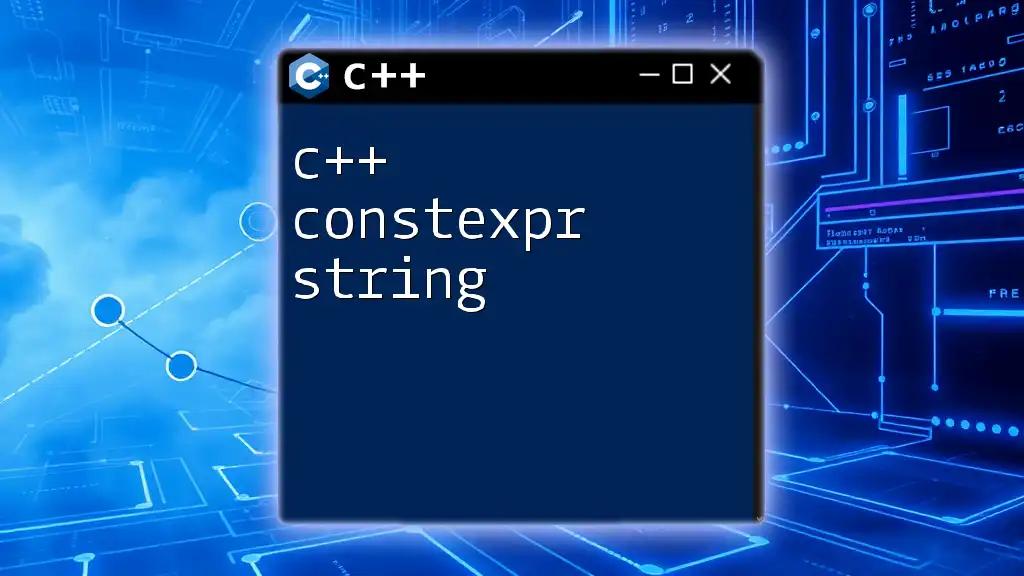
The Basics of Concatenation
What is Concatenation?
Concatenation is the process of joining two or more strings together to create a single, continuous string. In programming, especially in C++, concatenation is vital for constructing messages, generating dynamic content, and manipulating textual data. By concatenating strings and integers, you can easily create informative outputs and user feedback.
Importance of Concatenation in C++
Concatenating strings and integers serves many practical purposes in C++. For example, you can create user-friendly output messages, log information, or even format data for display in user interfaces. The ability to combine different data types quickly and efficiently is a key feature that makes C++ a powerful language.
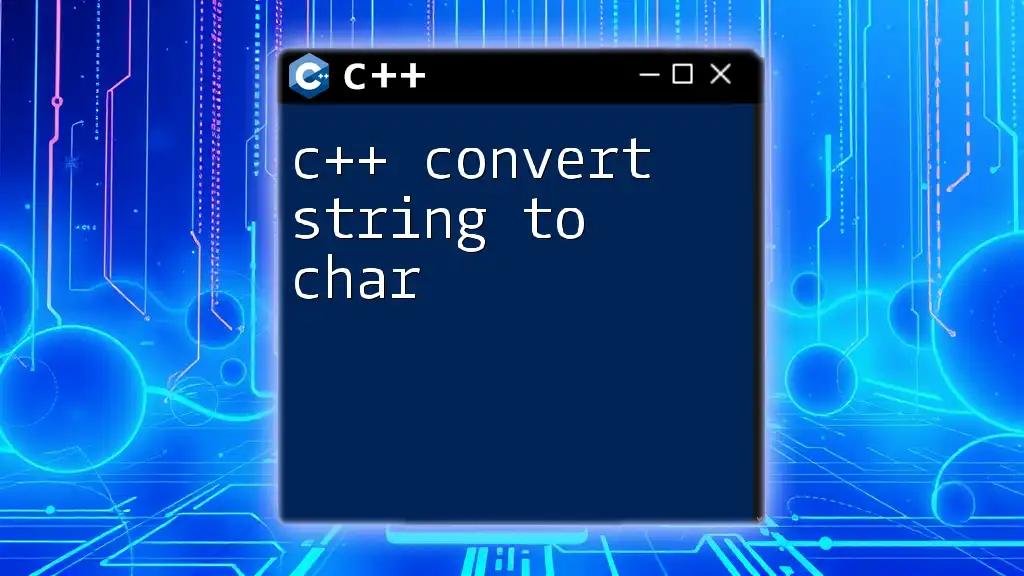
Ways to Concatenate String and Int in C++
Using `std::to_string()`
Explanation of `std::to_string()`
The `std::to_string()` function is a convenient way to convert numbers into strings. This function takes an integer or floating-point number as input and returns its string representation, allowing for easy concatenation with other strings. This is particularly useful because it helps eliminate type mismatches during the concatenation process.
Example Snippet
int number = 42;
std::string text = "The answer is: " + std::to_string(number);
std::cout << text << std::endl;
In this example, the integer `42` is converted to a string using `std::to_string()`, then concatenated with the preceding string. Step-by-step, this example shows how to create a meaningful message and demonstrate the ease of combining data types in C++.
Using String Streams
Introduction to String Streams
String streams, implemented through the `std::ostringstream` class from the `<sstream>` header, offer a flexible and powerful way to concatenate strings and integers. This approach is particularly advantageous when you are combining multiple data types or when concatenation is necessary within a larger block of code.
Example Snippet
#include <sstream>
int number = 42;
std::ostringstream oss;
oss << "The answer is: " << number;
std::string result = oss.str();
std::cout << result << std::endl;
In this snippet, the `std::ostringstream` object named `oss` is used to insert both a string and an integer into a single output stream. After concatenation, `.str()` is called to retrieve the complete string. This method is not only convenient but also effective when building complex strings.
Using `std::string::append()`
How `append()` Works
The `append()` method of the `std::string` class allows you to concatenate strings easily without the need for explicit conversions for included strings. This method can take both another string and characters, making it versatile for string manipulation.
Example Snippet
int number = 42;
std::string text = "The answer is: ";
text.append(std::to_string(number));
std::cout << text << std::endl;
In this example, you can see how the `append()` method builds up the `text` string by adding the string conversion of `number`. This keeps the base string intact while seamlessly adding new content.
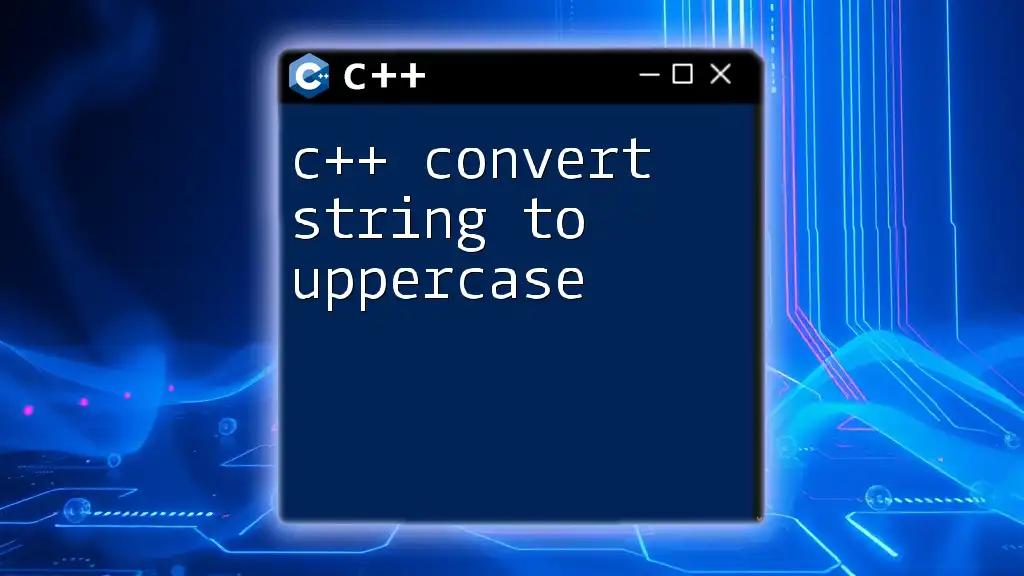
Exploratory Techniques
Concatenation with Other Data Types
Combining Multiple Data Types
Concatenating various data types can yield rich outputs. For example, you can combine integers, floats, and strings to create comprehensive output messages. Below is a demonstration that combines an integer and a float using string streams.
#include <string>
#include <sstream>
int integer = 5;
float floatingPoint = 3.14;
std::ostringstream oss;
oss << "Integer: " << integer << ", Float: " << floatingPoint;
std::string combined = oss.str();
std::cout << combined << std::endl;
In this code, both different numeric types are combined into a single string, exemplifying how versatile and powerful string streams are in accommodating varied data types.
Error Handling Considerations
When concatenating strings and integers, it’s essential to handle potential errors, such as invalid castings or conversions. Always ensure your integer or float conversions to strings using `std::to_string()` are correct to avoid undefined behavior.

Common Mistakes to Avoid
Mistakes When Concatenating Strings and Integers
One common mistake is neglecting type conversions before concatenation. For example, trying to concatenate a string and an integer directly will lead to compilation errors. Always ensure proper conversions are made to prevent such issues.
Performance Considerations
While methods like `std::to_string()` and string streams work well for small-scale applications, consider performance implications when dealing with large quantities of string concatenations. In such cases, you may want to benchmark different methods to identify the most efficient approach for your specific context.
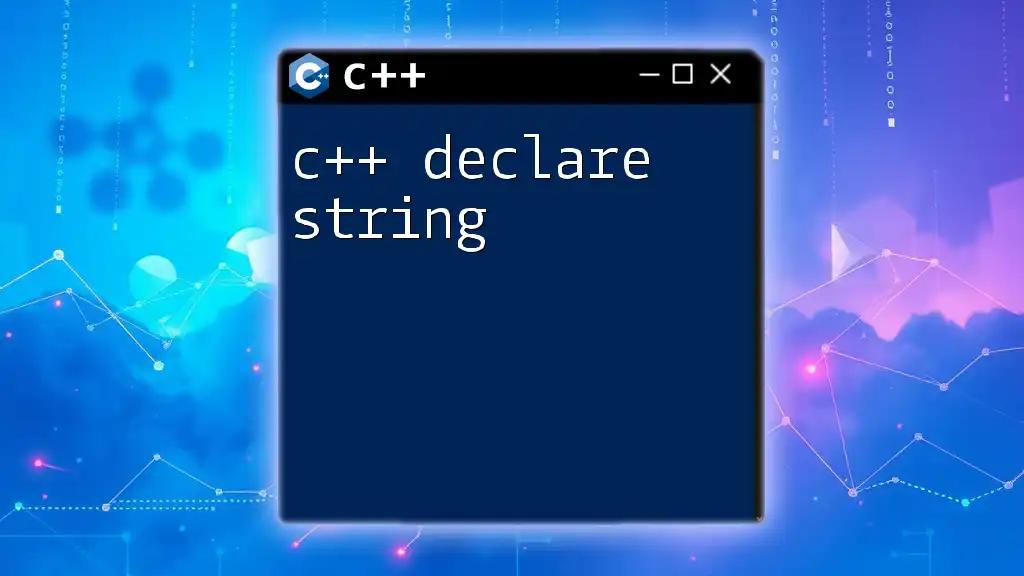
Conclusion
In summary, concatenating a string and an integer in C++ can be accomplished through several effective methods, including `std::to_string()`, string streams, and the `append()` method. Each method has its unique advantages, making it essential to choose the right one based on your needs. The ability to combine different data types seamlessly empowers programmers to create dynamic and informative outputs.
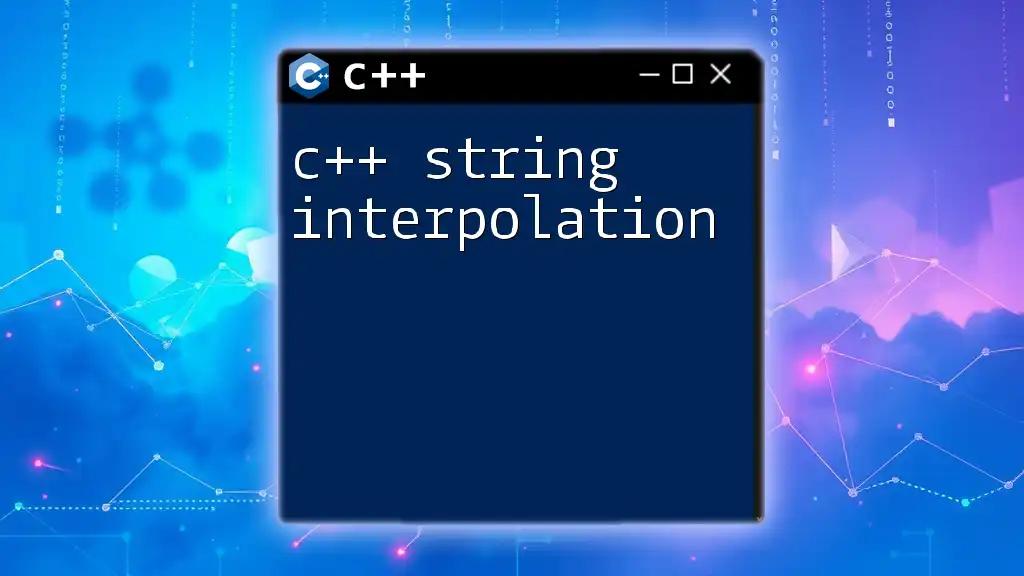
Additional Resources
Further Reading on C++ Basics
For readers eager to enhance their understanding of C++, consider diving deeper into comprehensive guides or online courses covering both foundational concepts and advanced techniques.
C++ Community and Support Channels
Engage with fellow learners and professionals through C++ forums, discussion boards, or social media groups to share experiences and troubleshoot challenges together.
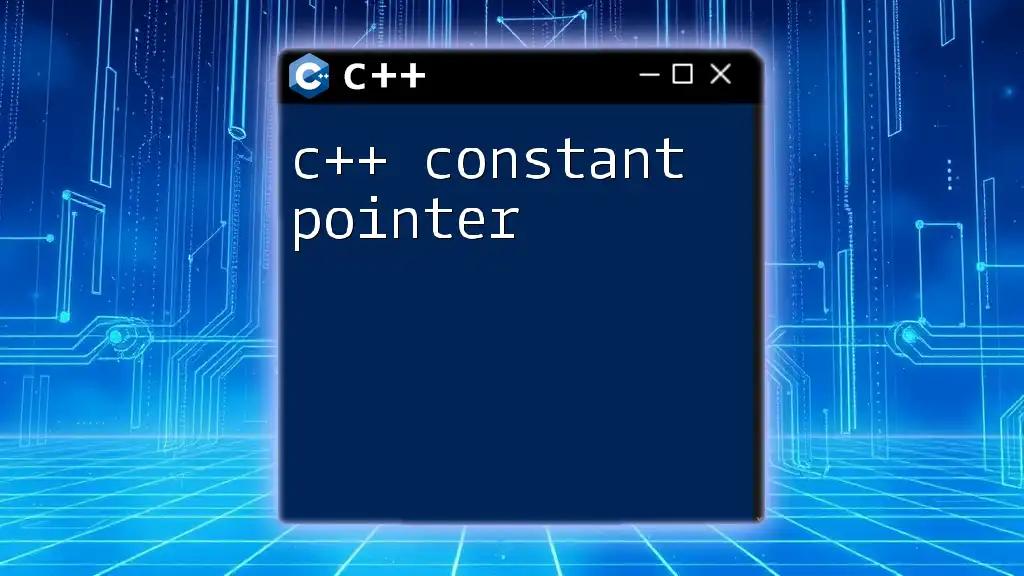
Call to Action
Feel free to share your own examples or unique methods of concatenating strings and integers in the comments below. Subscribe for more tailored C++ programming tips and tricks to boost your coding journey!