In C++, you can remove spaces from a string by iterating through it and appending non-space characters to a new string. Here's a simple code snippet to demonstrate this:
#include <iostream>
#include <string>
std::string removeSpaces(const std::string &str) {
std::string result;
for (char ch : str) {
if (ch != ' ') {
result += ch;
}
}
return result;
}
int main() {
std::string input = "Hello World!";
std::string output = removeSpaces(input);
std::cout << output; // Output: HelloWorld!
return 0;
}
Understanding C++ Strings
What is a String in C++?
In C++, a string is a sequence of characters that can be manipulated in various ways. Strings are a fundamental data type used to handle textual data, and they provide functionalities for operations such as accessing individual characters, concatenation, and substring extraction. In C++, the `std::string` class from the Standard Template Library (STL) makes string manipulation intuitive and efficient.
Basic Operations on Strings
Before diving into how to remove spaces from a string in C++, it's essential to understand some basic operations associated with strings:
- Concatenation: Combining two or more strings into a single string.
- Substring extraction: Retrieving a portion of the string based on specified indices.
- Length measurement: Determining the number of characters in the string using the `.length()` or `.size()` method.

Why Remove Spaces from a String?
Removing spaces from a string is a common requirement in programming, especially when dealing with user input or data processing. Some scenarios where space removal is crucial include:
- Data Cleaning: To ensure consistent formatting of data entered by users.
- Search Operations: To improve search efficiency by eliminating unnecessary white space.
- Performance Enhancements: In cases where excessive spaces could slow down the processing time, particularly in large datasets.
In each of these scenarios, an efficient way to remove spaces from a string in C++ can lead to cleaner, more effective code.
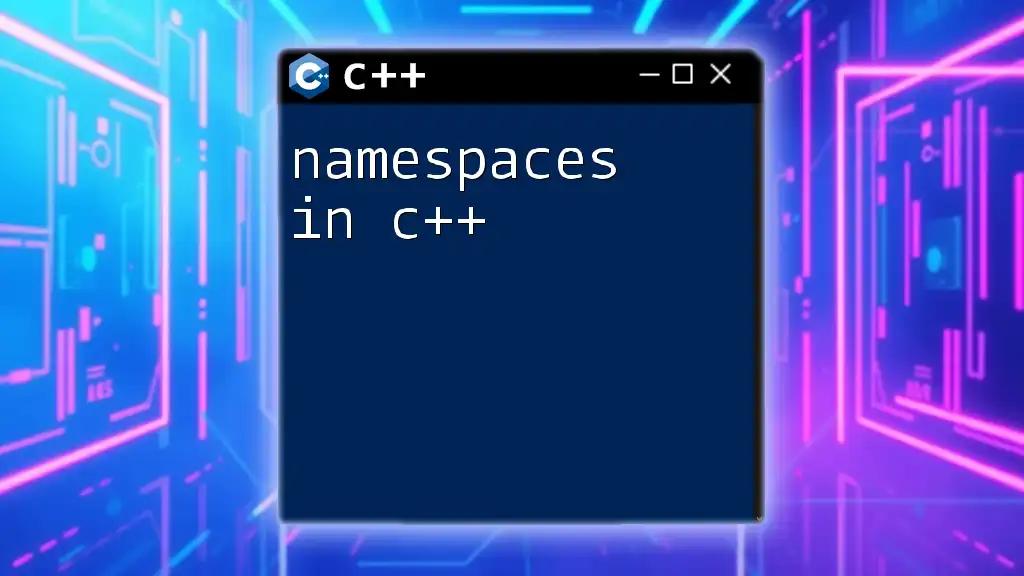
Methods to Remove Spaces from C++ Strings
Using `std::string::erase()` Method
One of the simplest methods to remove spaces from a string in C++ is using the `erase()` method in conjunction with `std::remove`. The `remove` algorithm rearranges the string by moving the non-space characters to the front, and `erase` trims the remaining characters.
Code Snippet: Basic Space Removal
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello World!";
str.erase(remove(str.begin(), str.end(), ' '), str.end());
std::cout << str; // Output: HelloWorld!
return 0;
}
In this example, the `remove` function is used to shift all non-space characters to the beginning of the string, and `erase` is called to eliminate the trailing spaces. This method is not only efficient but also straightforward to implement.
Utilizing `std::remove_if()` with Lambda Expressions
Another robust technique involves using `std::remove_if()` with lambda expressions. This method provides flexibility, making it easy to define custom conditions for space removal.
Code Snippet: Removing Spaces with Lambda
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string str = "Hello C++ World!";
str.erase(std::remove_if(str.begin(), str.end(), [](unsigned char x) { return std::isspace(x); }), str.end());
std::cout << str; // Output: HelloC++World!
return 0;
}
This code defines a lambda function that checks whether a character is a whitespace character using `std::isspace`. The `std::remove_if` algorithm then relocates non-whitespace characters, allowing us to call `erase` to finalize the removal of spaces. This method is advantageous for more complex conditions beyond just space removal.
Using String Stream for Space Removal
A more powerful approach to remove spaces employs `std::istringstream`, allowing us to read the string word by word and construct a new string without spaces.
Code Snippet: Stream Method
#include <iostream>
#include <sstream>
#include <string>
std::string removeSpaces(const std::string &str) {
std::istringstream iss(str);
std::string result, word;
while (iss >> word) {
result += word; // Concatenates words without spaces
}
return result;
}
int main() {
std::string str = "This is C++ programming!";
std::cout << removeSpaces(str); // Output: ThisisC++programming!
return 0;
}
In this method, an `istringstream` object processes the input string and extracts words separated by spaces. The result is built step by step to ensure that no spaces are included. Not only is this method clear, but it also allows for future adjustments, such as handling punctuation or other whitespace characters.
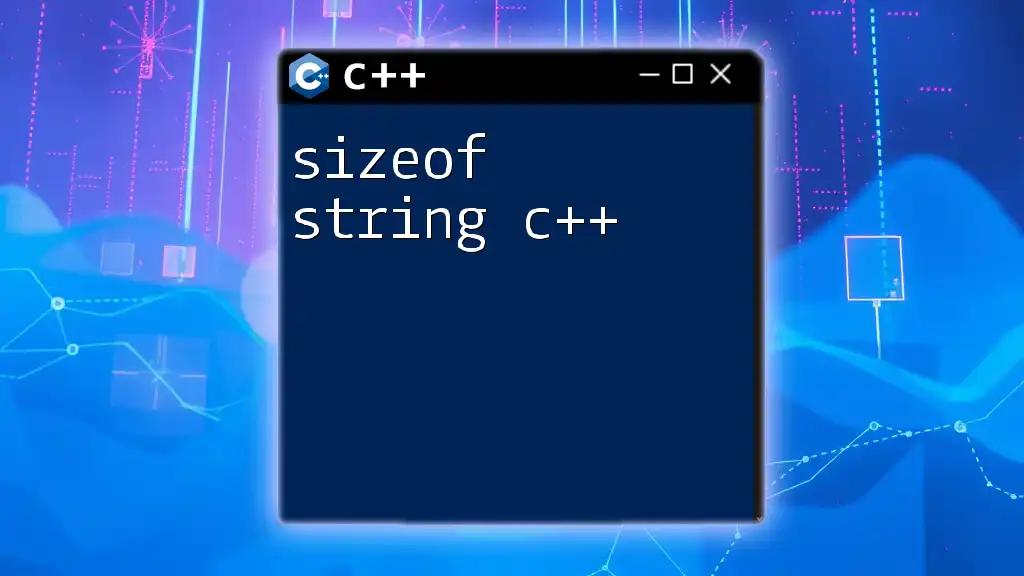
Performance Considerations
Efficiency of Each Method
When it comes to performance, each technique has its trade-offs. The `erase()` combined with `remove()` is generally efficient for smaller strings, having a time complexity of O(n). The `std::remove_if()` method involves more complex checks per character, which may increase processing time when dealing with very large datasets, but still typically operates in O(n) time.
Using string streams might seem slower due to multiple read/write operations, especially in a loop, but it can lead to more elegant code that is easier to maintain.
Memory Considerations
Memory usage should be a consideration as well. Methods that create new strings (like the string stream approach) can lead to higher memory consumption, particularly with larger datasets. Ensuring optimal usage of resources is crucial in performance-sensitive applications.
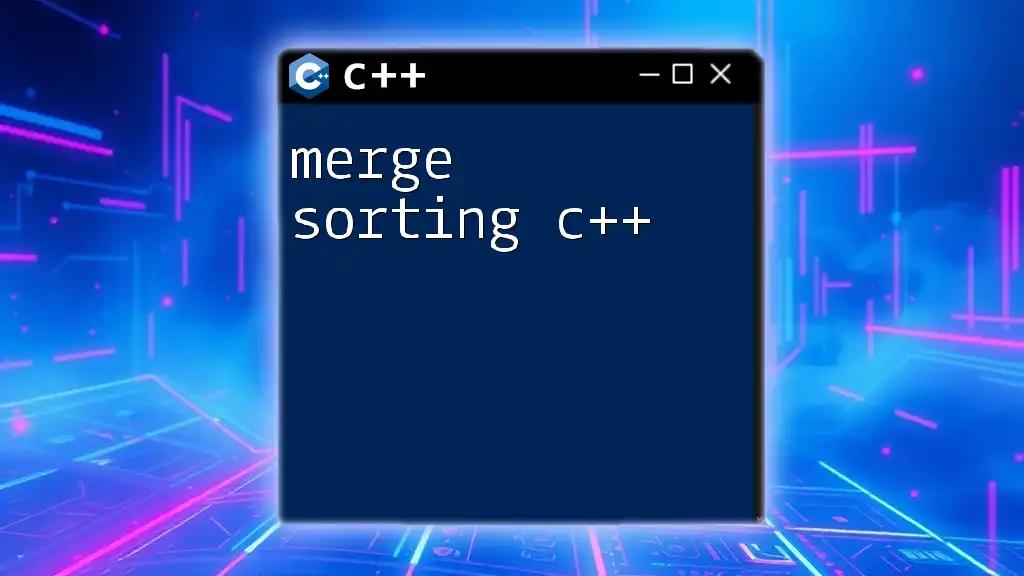
Summary
In this guide, we've examined several methods to remove spaces from a string in C++. From the straightforward `std::string::erase()` approach to the more complex `std::remove_if()` with lambda expressions, each method offers unique advantages. The string stream method showcases clarity and flexibility, making it an excellent choice for larger, more complicated tasks.

Additional Resources
For further reading or in-depth study, consider checking the following resources:
- C++ Standard Template Library Documentation
- Online coding communities such as Stack Overflow for practical use cases and discussions.

Conclusion
Mastering string manipulation in C++ is essential for any programmer looking to enhance their coding skills. By understanding how to efficiently remove spaces from a string in C++, you can improve your code's performance and readability. Practice these methods, experiment with variations, and share your findings as you delve deeper into the world of C++.