The `namespace std` in C++ is a standard library namespace that contains all the standard functions and objects, allowing you to use them directly without prefixing them with `std::`.
Here's a code snippet that demonstrates its use:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Alternatively, you could use the `using` directive to avoid prefixing:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is the std Namespace in C++?
The `std` namespace is a crucial component of the C++ programming language, encapsulating the Standard Library's functionalities. Introduced as part of the C++ Standard Library, the `std` namespace contains essential classes and functions that enable developers to perform various tasks, from simple input/output operations to complex data manipulation.
Common components included in the `std` namespace encompass a wide array of functionalities, such as:
- Data structures: `std::vector`, `std::map`, `std::list`
- Algorithm support: utilities like `std::sort`, `std::find`, and `std::accumulate`
- Input/Output functionalities: `std::cin`, `std::cout`, `std::endl`
Understanding the `std` namespace is foundational for effectively leveraging the C++ Standard Library, which significantly boosts productivity and code efficiency.
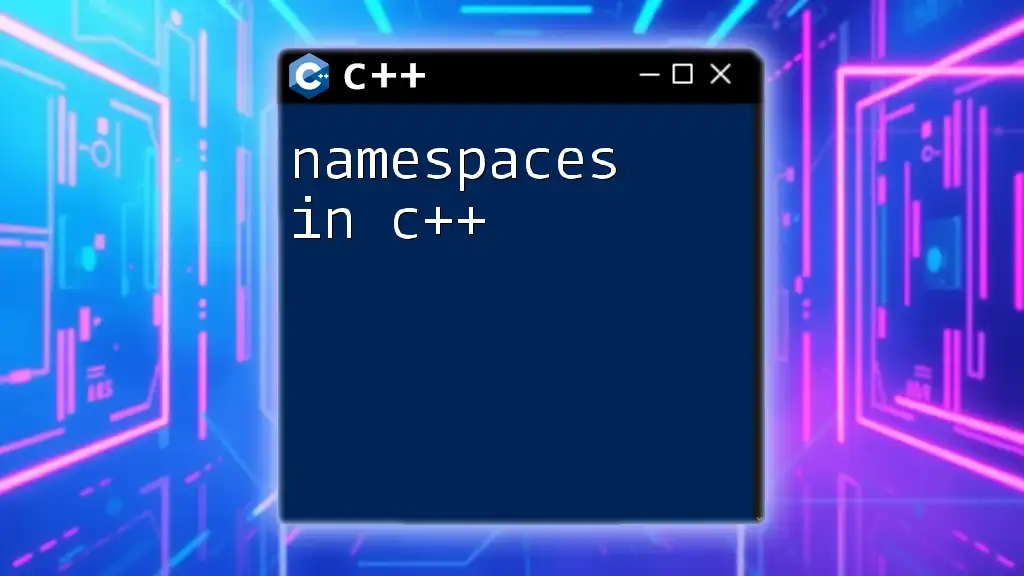
Why Use Namespace std?
Using the `std` namespace in C++ brings several advantages. By declaring `using namespace std;`, you reduce the chances of name conflicts when integrating multiple libraries or codebases. This becomes especially valuable in larger projects where function and type definitions might overlap. Moreover, employing the `std` namespace typically enhances code readability and maintainability, as it allows for cleaner and more concise code with fewer prefixes.
However, it’s important to maintain a balance; blindly utilizing the `std` namespace can lead to confusion and bugs, especially if different libraries use the same names.
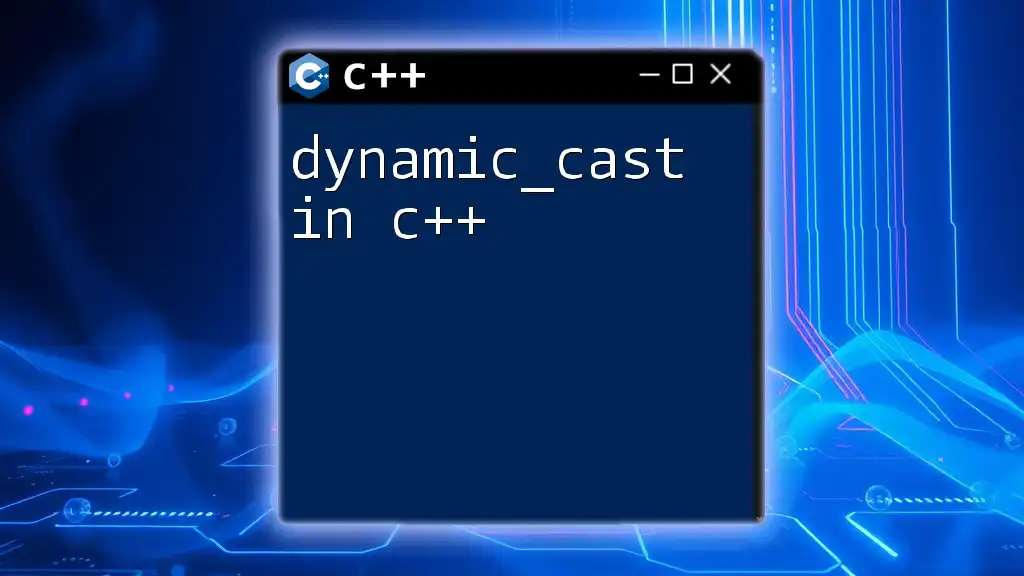
Using Namespace std in C++
What is Using Namespace std in C++?
Declaring `using namespace std;` at the beginning of your code signals to the compiler that you're opting to use the entities defined within the `std` namespace without needing to prepend `std::` to each reference. This directive can significantly reduce typing overhead and improve code clarity for small programs or learning purposes.
Code Example of Using Namespace std
Consider the following simple program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example, we include the `<iostream>` header file for input and output operations. By using `using namespace std;`, we can directly use `cout` and `endl` without the `std::` prefix, thereby making the code cleaner and easier to read.
Risks and Drawbacks of Using Namespace std
Despite the convenience, employing `using namespace std;` can lead to potential naming conflicts. For instance, if you have another custom function named `sort`, the compiler wouldn’t know whether to use your version or `std::sort()`. Such confusion could introduce subtle bugs into your application.
It is often recommended to limit the use of this directive to small scripts, teaching scenarios, or testing environments. For larger projects, consider minimizing its use.
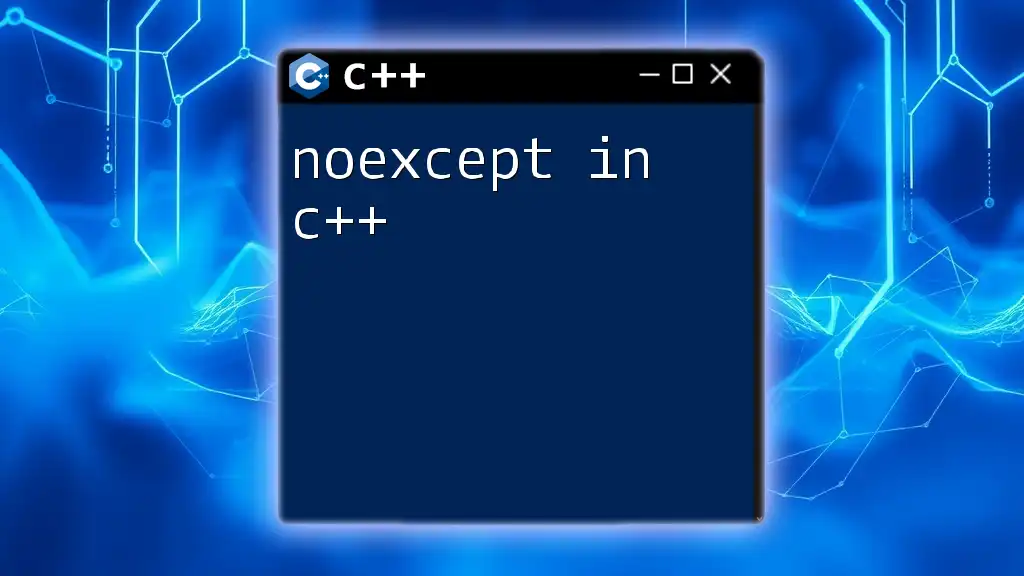
Alternative to Using Namespace std
Fully Qualifying Names in C++
An alternative to using `using namespace std;` is to fully qualify each entity from the `std` namespace. This approach is more verbose, as it requires prepending `std::` before every entity, but it greatly reduces the risk of conflict and ambiguity.
Pros and Cons of Fully Qualifying Names
Using fully qualified names enhances clarity by making it unequivocal where a function or type originates. For instance:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This snippet clarifies that `cout` and `endl` belong specifically to the `std` namespace. However, it also leads to more verbose code, which might slow down development speed and make the code appear cluttered, especially in lengthy codebases.
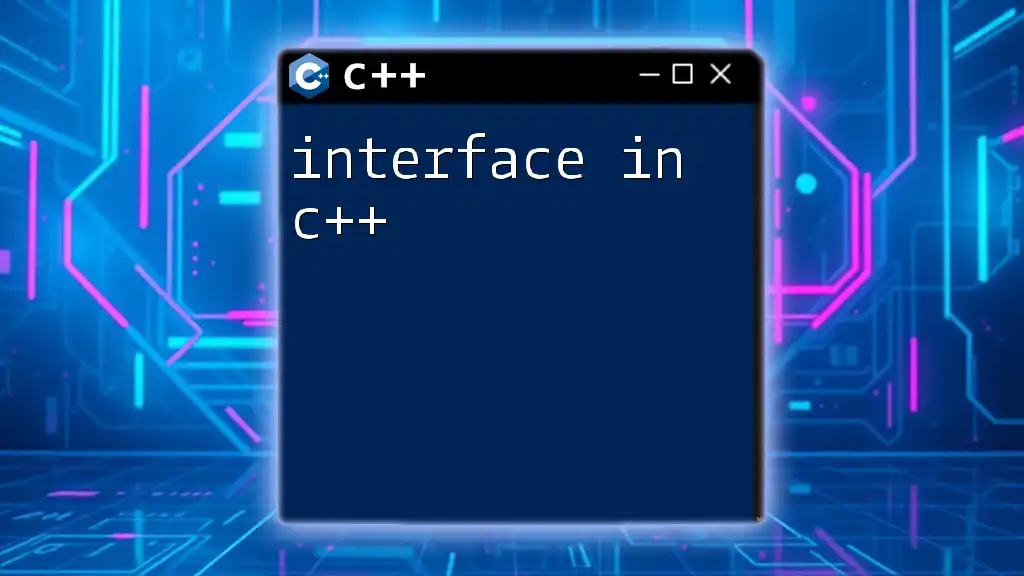
When to Use Namespace std
Best Practices for Using Namespace std
To maximize the benefits of the `std` namespace while minimizing risks, it’s recommended to:
- Limit the use of `using namespace std;` to small programs where the benefits outweigh the potential downside.
- Use explicit qualification (e.g., `std::cout`, etc.) in larger projects, particularly when multiple libraries are present.
- Be cautious with scope: If you absolutely must use `using namespace std;`, do so within limited scopes, such as inside functions, to avoid polluting the global namespace.
Examples of When Not to Use Namespace std
A practical scenario may involve using libraries that could conflict with standard definitions. As a case in point, if you utilize a specialized library for mathematical computations that defines its own `sqrt` function, calling `sqrt(x)` may inadvertently invoke either the library's or `std::sqrt()` based on the first one recognized by the compiler. Such ambiguity can lead to serious bugs and is a strong reason to avoid a blanket `using` directive.
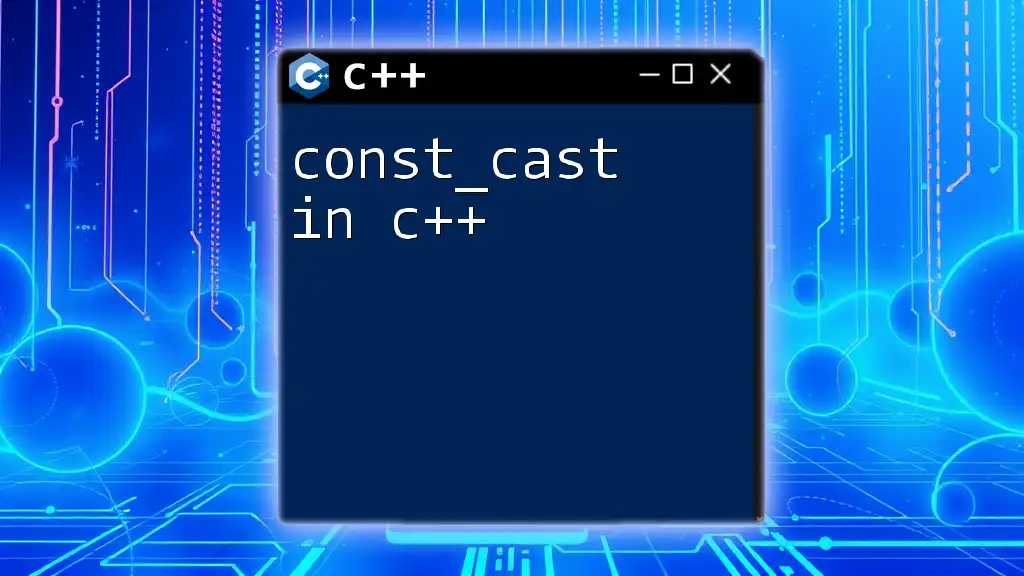
Conclusion
In summary, the `std` namespace in C++ is an integral part of the Standard Library that promotes code organization and efficiency. Understanding when and how to use it can bolster your programming practice significantly. By staying mindful of the practical implications of `using namespace std;` versus fully qualified names, C++ developers can harness the full power of the Standard Library while delivering clean, maintainable code. Embracing these best practices will lay a strong foundation for coding proficiency and project success.
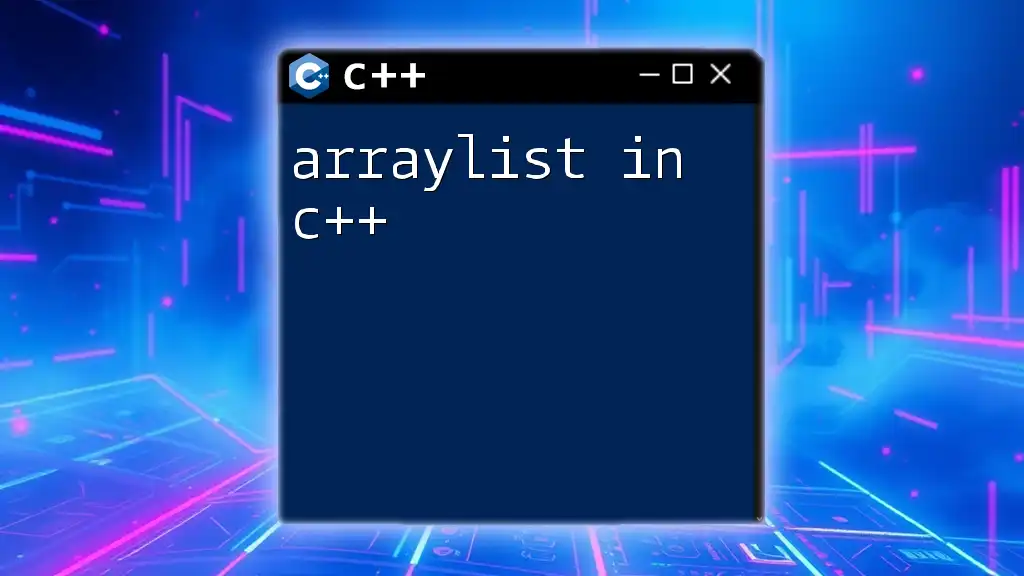
Additional Resources
To deepen your understanding of namespaces and the `std` library, consider exploring the following materials:
- C++ Documentation on Namespaces and the Standard Library
- C++ Community Forums for practical examples and discussions
- Books and online courses focused on advanced C++ programming techniques