In C++, you can convert various data types to a string using the `std::to_string` function, which is part of the `<string>` header.
Here's a code snippet demonstrating this:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string numStr = std::to_string(number);
std::cout << "The string representation is: " << numStr << std::endl;
return 0;
}
Understanding the Basics of String Handling in C++
In C++, a string is a sequence of characters that can be manipulated as a data type. The language provides a dedicated `std::string` class to handle strings, which offers multiple member functions for string manipulation.
A crucial distinction to make is between character arrays (C-style strings) and the `std::string` class. While C-style strings are arrays of characters terminated by a null character (`\0`), the `std::string` class provides more flexibility with features like automatic memory management and various utility functions. Thus, utilizing `std::string` is often preferred for modern C++ development.
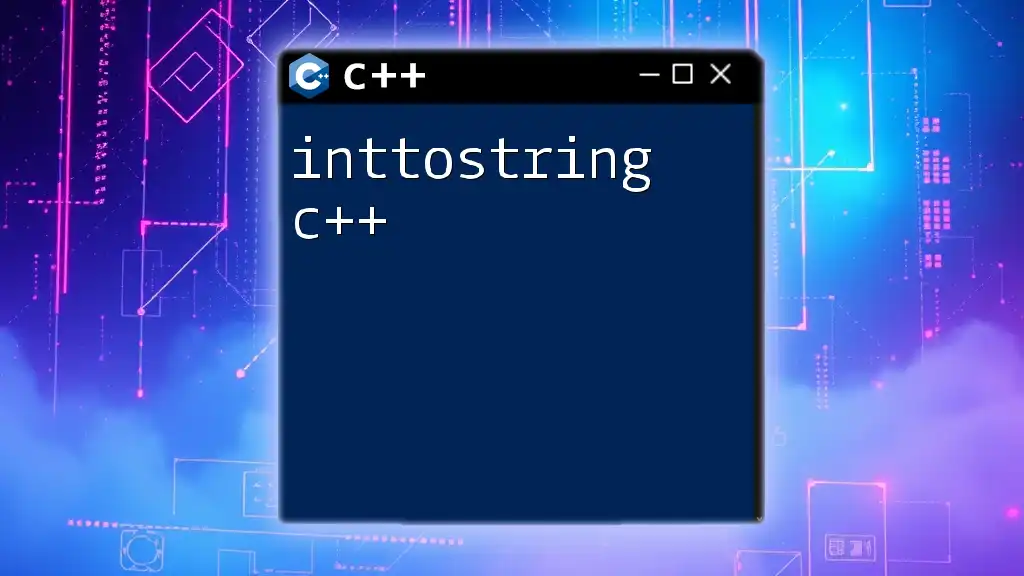
Converting Integer to String in C++
The Need for Conversion
Converting an integer to a string is a common operation in C++ programming. You might wish to do this for various reasons, such as displaying a numerical result as part of a message, logging output, or creating formatted strings for user interfaces.
Example scenario: You are developing an application that takes numerical input and presents the results in a user-friendly format. Without converting integers to strings, you would be unable to concatenate numbers with text.
Using `std::to_string()`
What is `std::to_string()`?
`std::to_string()` is a built-in function provided by the C++ Standard Library, included in the `<string>` header file. It allows for straightforward conversion of various numeric types (such as `int`, `float`, and `double`) to a string format.
How to Use `std::to_string()`:
The syntax is simple, and usage is as follows:
#include <iostream>
#include <string>
int main() {
int num = 42;
std::string str = std::to_string(num);
std::cout << "Converted integer to string: " << str << std::endl;
return 0;
}
In this code snippet, the integer `num` is converted to a string using `std::to_string()`, and the result is printed to the console.
Handling Different Data Types:
`std::to_string()` can convert not just integers but also floating-point numbers. For example:
double pi = 3.14159;
std::string piStr = std::to_string(pi);
std::cout << "Converted double to string: " << piStr << std::endl;
This versatility makes it a convenient option for various data types.
Using `std::ostringstream`
What is `std::ostringstream`?
`std::ostringstream` is part of the `<sstream>` header, providing a C++ way to manipulate strings using stream-based operations. This class allows you to construct strings by inserting various data types into a stream, ultimately converting it into a string.
How to Use `std::ostringstream`:
Here's how `std::ostringstream` can be used to convert an integer to a string:
#include <iostream>
#include <sstream>
int main() {
int num = 42;
std::ostringstream oss;
oss << num;
std::string str = oss.str();
std::cout << "Converted integer to string using ostringstream: " << str << std::endl;
return 0;
}
In this snippet, the integer is written into an `ostringstream`, and then the `str()` function is called to retrieve the formatted string.
Advantages of Using Streams:
Using `std::ostringstream` is particularly advantageous when you want to concatenate multiple strings or different data types without having to manually convert each type individually.
C-style String Conversion
Using `sprintf()` Function:
For those familiar with C or C-style strings, the `sprintf()` function can also be utilized to convert integers to strings. This function takes a formatting string and variables, writing the output into a `char` array.
Example of Using `sprintf()`:
#include <iostream>
#include <cstdio>
int main() {
int num = 42;
char buffer[50];
sprintf(buffer, "%d", num);
std::cout << "Converted integer to C-style string: " << buffer << std::endl;
return 0;
}
With this method, the integer `num` is formatted as a string and stored in the `buffer`.
Discussion on Safety and Risks:
While `sprintf()` can be convenient, it comes with risks. Primarily, it is subject to buffer overflow vulnerabilities. Always ensure that the buffer is large enough to avoid such issues, or consider using `snprintf()` for added safety.
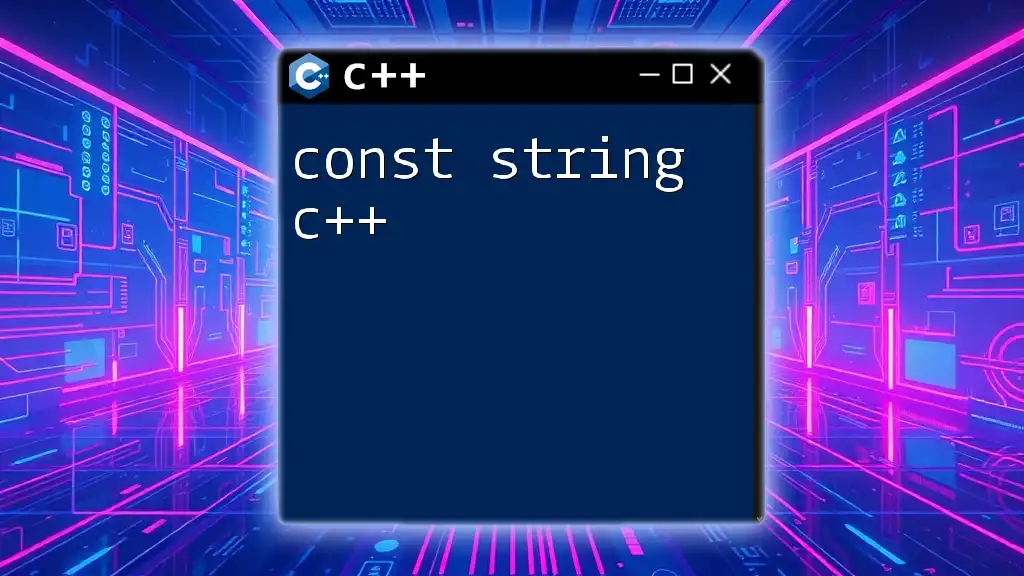
Common Pitfalls and Best Practices
Handling Edge Cases
When converting integers to strings, it's crucial to handle edge cases. For example, converting extremely large integers might lead to unexpected behavior, especially if you're working with data types like `int` and `long`.
Ensure that inputs are validated before conversion to avoid run-time errors and bugs.
Code Readability
Choosing the right conversion method can greatly affect the readability of your code. For most scenarios in modern C++ development, using `std::to_string()` is straightforward and clear. It eliminates the need to manage buffers or streams explicitly, making it easier for others (or yourself in the future) to understand your code.
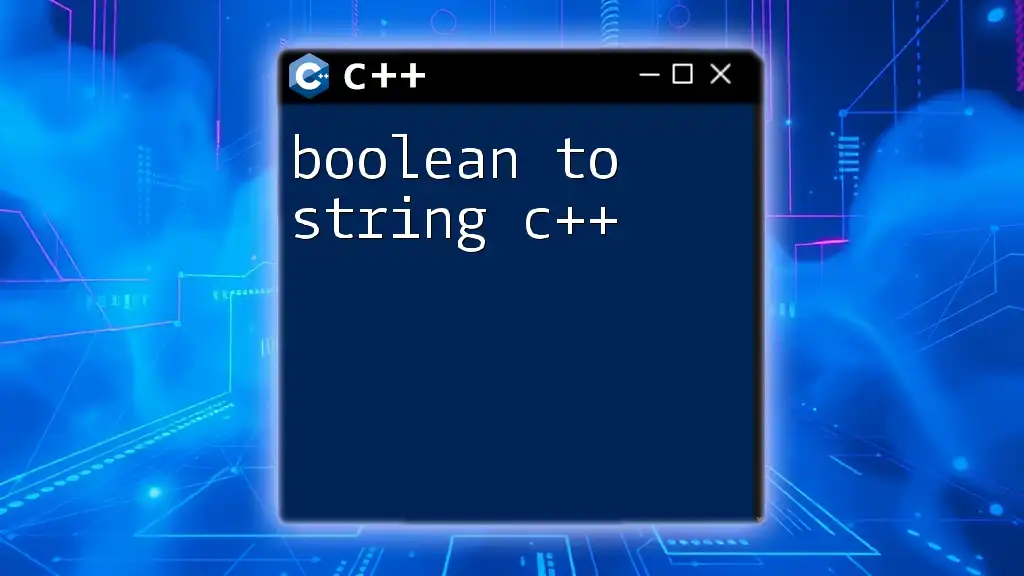
Conclusion
Converting an integer to a string in C++ can be accomplished through several methods, including `std::to_string()`, `std::ostringstream`, and C-style functions like `sprintf()`. Each of these methods has its own advantages and should be chosen based on the requirements of your project.
By understanding these techniques, you can effectively manipulate data types in C++, ensuring better interaction with users and more versatile program behavior. Practice these methods often, as they form a fundamental aspect of string handling in C++.