To remove an element from an array in C++, you'll need to create a new array without the specified element, as arrays have a fixed size; here's a simple example demonstrating this process:
#include <iostream>
using namespace std;
void removeElement(int arr[], int &n, int element) {
int newArr[n]; // New array to hold elements after removal
int j = 0;
for (int i = 0; i < n; i++) {
if (arr[i] != element) {
newArr[j++] = arr[i]; // Only copy elements that are not the target
}
}
n = j; // Update the size of the new array
for (int i = 0; i < n; i++) {
arr[i] = newArr[i]; // Copy elements back to original array
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
removeElement(arr, n, 3);
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of items stored at contiguous memory locations. This data structure is fundamental to C++, providing a way to group similar data types together, which allows for efficient data management and manipulation. Arrays enable fast access to elements via indexing, making them invaluable for handling data where you need to retrieve, add, or remove elements frequently.
Despite their advantages, static arrays come with limitations, such as fixed size, which can impact performance and flexibility when dealing with dynamic datasets.
Types of Arrays in C++
C++ supports various types of arrays, each with its own characteristics and use cases:
- Single-dimensional arrays: These are the simplest forms of arrays, often used to store a series of values of the same type in a linear fashion.
- Multi-dimensional arrays: Used for complex data structures, such as matrices, these arrays allow the representation of data in two (or more) dimensions.
- Dynamic arrays: Using pointers, dynamic arrays can be resized during runtime, offering significant flexibility compared to static arrays.
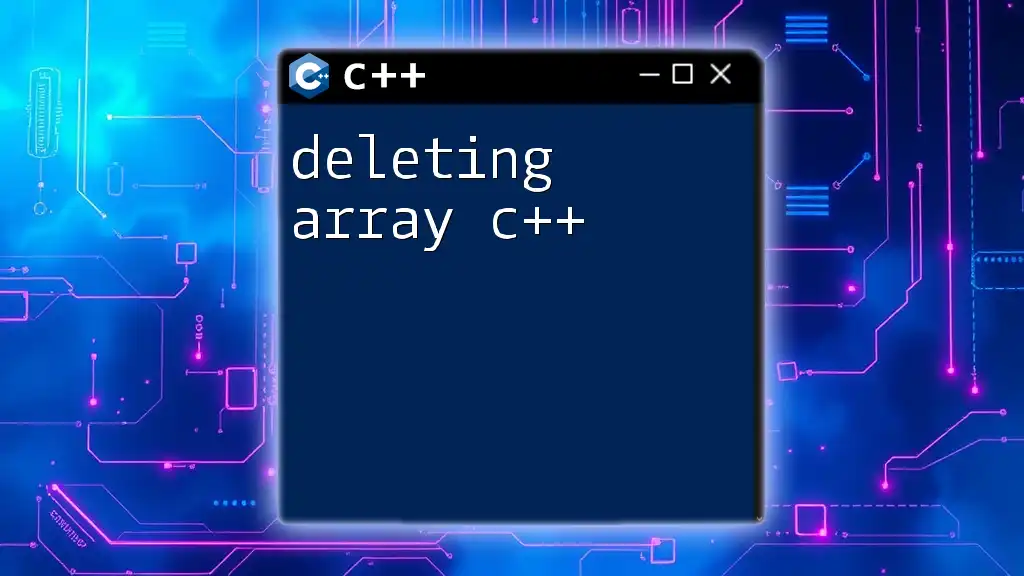
How to Remove an Element from an Array in C++
The Basics of Removal
When discussing how to remove an element in an array C++, it's essential to understand what this means from a structural perspective. Removing an element doesn't physically delete it from memory; instead, it involves shifting subsequent elements to close the gap, effectively shortening the array's logical size.
Removing an Element from a Static Array
Logic for Removal
To remove an element by its index, you need to:
- Identify the index of the element to be removed.
- Shift subsequent elements to the left to fill the gap left by the removed element.
- Decrease the logical size of the array.
By following this approach, the logical structure of the array remains intact, even though the physical memory remains allocated.
Code Snippet
#include <iostream>
void removeElement(int arr[], int& size, int index) {
for (int i = index; i < size - 1; ++i) {
arr[i] = arr[i + 1]; // Shift elements left
}
--size; // Decrease logical size
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = 5;
removeElement(arr, size, 2); // Removes the element at index 2 (3)
for (int i = 0; i < size; ++i) {
std::cout << arr[i] << " "; // Output: 1 2 4 5
}
}
This code defines a function that demonstrates how to remove an element from a static array. It takes the array and its size as input and shifts all elements following the specified index. After executing this function, the array will no longer contain the removed element, and the logical size of the array is updated accordingly.
Removing an Element from a Dynamic Array
Using the `std::vector` Class
In modern C++, the `std::vector` class offers a dynamic and highly flexible way to manage arrays. Unlike static arrays, vectors can grow and shrink as needed. This capability simplifies the removal process significantly.
Code Snippet
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 2); // Removes the element at index 2 (3)
for (const int& num : vec) {
std::cout << num << " "; // Output: 1 2 4 5
}
}
Using `std::vector`, the removal of an element becomes highly efficient thanks to built-in functions like `erase`. This method abstracts away the complexity of element shifting, enabling rapid array manipulation and management without the overhead of manual operations.
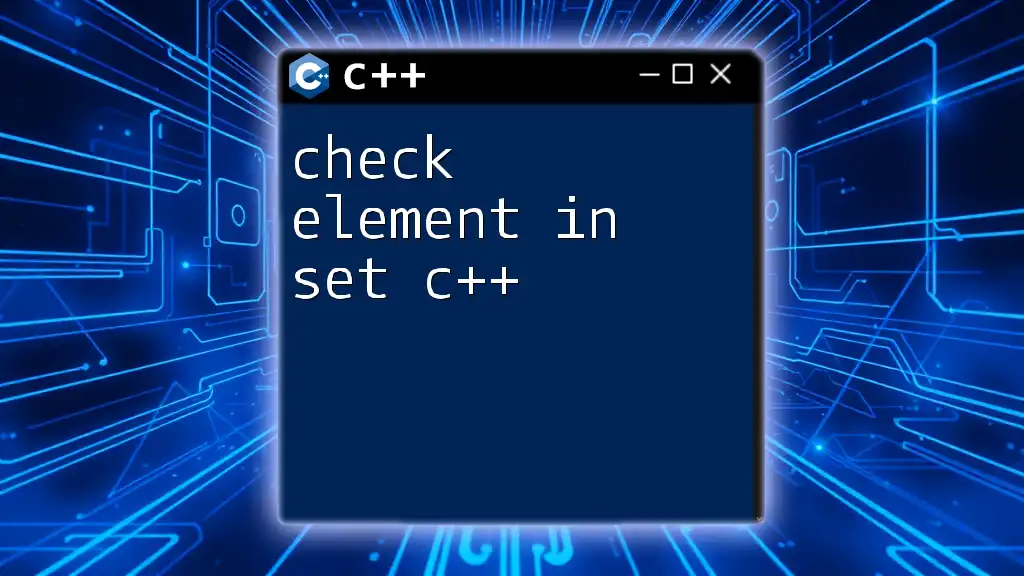
Common Scenarios for Removing Elements
Removing Elements by Value vs. Index
When working with arrays, it's critical to differentiate between removing an element by its index (position) and by its value. While removing by index is straightforward, removing by value involves searching for the element first, which can add complexity and processing time.
Examples of Use Cases
Dynamic data updates are a common scenario for removing elements. For instance, when handling user inputs in real-time applications, one may frequently need to remove outdated or irrelevant entries. Similarly, filtering unwanted values from a dataset—such as excluding negative numbers from a list of scores—requires the removal of elements efficiently.
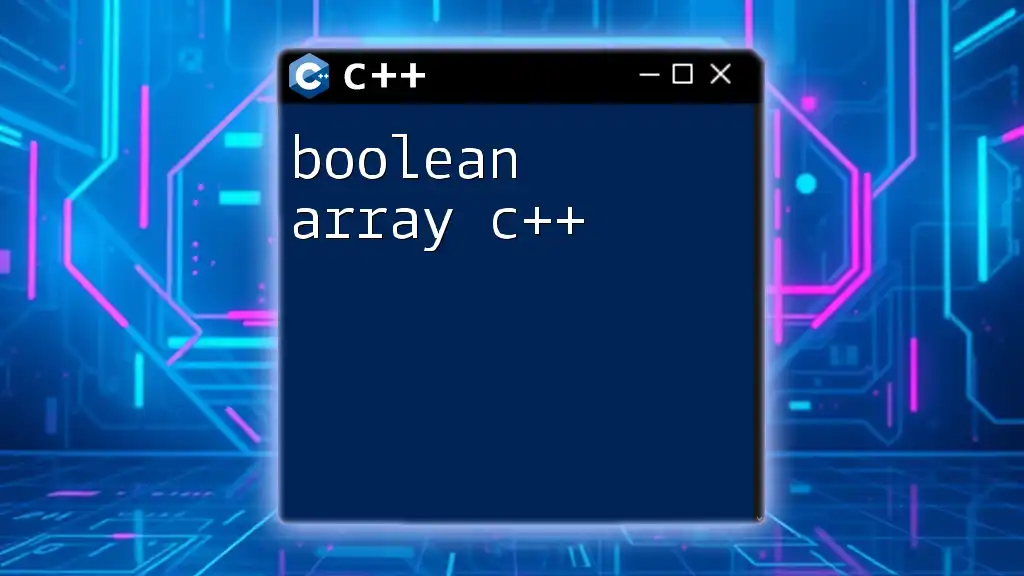
Error Handling and Edge Cases
Handling Invalid Indexes
Passing an invalid index during removal can lead to undefined behavior. It is crucial to check if the index lies within the valid range of the array before performing any operations. Implementing bounds checking or using exceptions can help prevent runtime errors and safeguard against misuse.
Empty Arrays
Working with empty arrays poses another challenge; attempting to remove an element from an empty array can also lead to errors. Implementing checks to verify that the array has elements available for removal is a good programming practice to avoid potential issues.
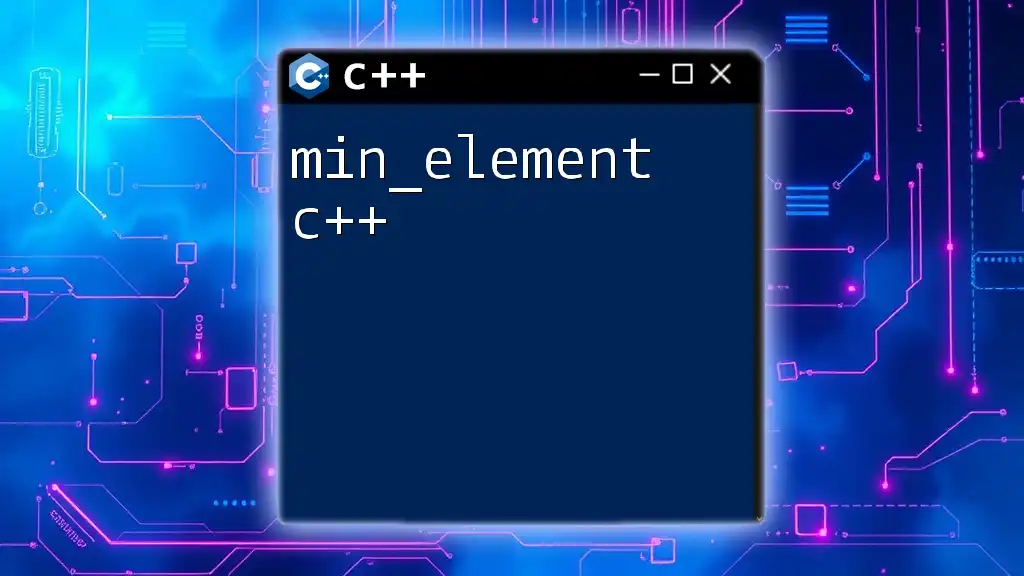
Conclusion
In conclusion, knowing how to remove an element in an array C++ is a fundamental skill that can enhance efficiency in data handling. By understanding both static and dynamic arrays and employing the right methods for removal, developers can create flexible and robust applications.
Practicing removal functions, along with familiarizing oneself with error handling techniques, is essential for mastering arrays in C++. This knowledge not only empowers you to manipulate data effectively but also elevates your overall C++ programming proficiency.
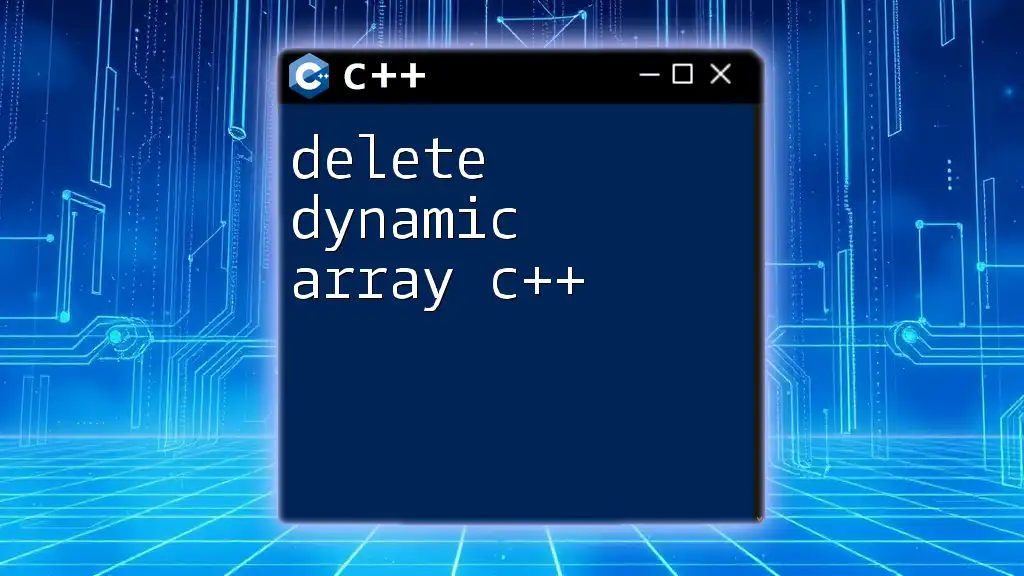
Additional Resources
For those seeking further understanding, consider exploring the official C++ documentation, tutorials on array manipulation, and advanced algorithms that utilize arrays to deepen your knowledge and skills in C++.