To add elements to a vector in C++, you can use the `push_back()` method to append an element at the end of the vector.
#include <vector>
int main() {
std::vector<int> myVector;
myVector.push_back(10); // Adds 10 to the end of the vector
myVector.push_back(20); // Adds 20 to the end of the vector
return 0;
}
What is a Vector in C++?
Definition of Vector
A vector in C++ is a dynamic array that can resize automatically when elements are added or removed. Unlike traditional arrays, which have fixed sizes, vectors offer a flexible alternative for managing collections of data.
Benefits of Using Vectors
Vectors come with notable advantages, such as:
- Automatic Memory Management: They manage memory allocation and deallocation automatically, allowing for efficient use of resources.
- Dynamic Resizing: When elements are added beyond its current capacity, vectors automatically resize themselves, ensuring that you're not limited by pre-defined sizes.
- Built-in Functions: Vectors come with numerous utility functions that simplify various operations, including searching, sorting, and iterating.
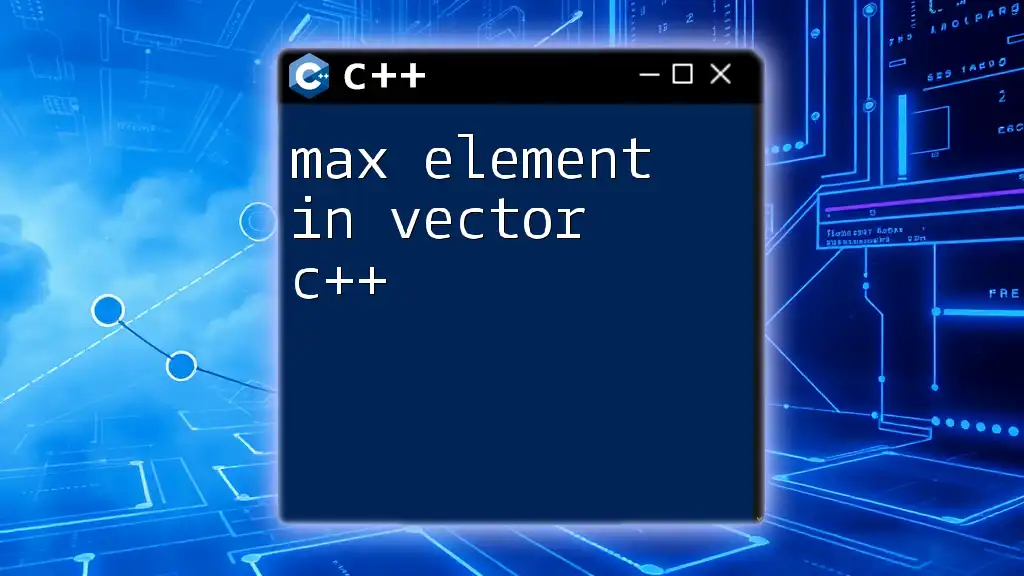
How to Add Elements to a Vector in C++
Using the `push_back()` Method
The `push_back()` method is one of the simplest ways to add an element to a vector in C++. It appends the element to the end of the vector.
Usage: The syntax is straightforward:
vector_name.push_back(value);
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
vec.push_back(10);
vec.push_back(20);
std::cout << "Vector elements: ";
for (int element : vec) {
std::cout << element << " ";
}
return 0;
}
Explanation: In this example, elements `10` and `20` are added to the vector `vec`. By using `push_back`, the vector reallocates memory as needed to accommodate the new elements, simplifying the process of managing array sizes.
Using the `insert()` Method
The `insert()` method allows you to add elements at a specific position in the vector—this makes it versatile for various insertion strategies.
Usage: The syntax for the `insert()` method is as follows:
vector_name.insert(iterator_position, value);
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3};
vec.insert(vec.begin() + 1, 10); // Inserts 10 at position 1
std::cout << "After insert: ";
for (int element : vec) {
std::cout << element << " ";
}
return 0;
}
Explanation: In this example, `10` is inserted at index `1`, shifting existing elements to the right. The use of `vec.begin() + 1` specifies the location to insert the new element. Understanding iterators is crucial here, as they allow you to navigate through the vector easily.
Using the `emplace_back()` Method
The `emplace_back()` method is similar to `push_back()`, but more efficient in some situations because it constructs the object in place.
Usage: The method can be used like this:
vector_name.emplace_back(value);
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<std::string> vec;
vec.emplace_back("Hello");
vec.emplace_back("World");
std::cout << "After emplace_back: ";
for (const auto &str : vec) {
std::cout << str << " ";
}
return 0;
}
Explanation: The `emplace_back()` method adds `"Hello"` and `"World"` to the `vec` vector. Here, the string objects are constructed directly in the vector's memory, which can lead to fewer allocations and improved performance.
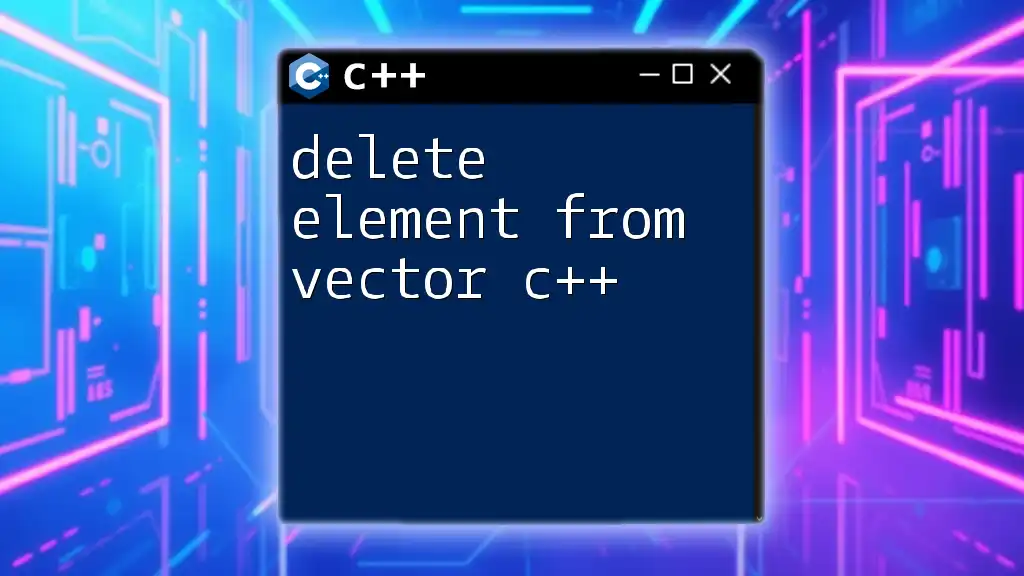
Adding Multiple Elements
Using the `insert()` Method with a Range
If you need to add multiple elements at once, `insert()` can also accept a range of elements, making it efficient for batch inserts.
Usage: When inserting multiple elements from another vector, you specify the range by using two iterators.
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3};
std::vector<int> newElements = {4, 5, 6};
vec.insert(vec.end(), newElements.begin(), newElements.end()); // Insert range
std::cout << "After inserting multiple elements: ";
for (int element : vec) {
std::cout << element << " ";
}
return 0;
}
Explanation: In this snippet, the vector `newElements` containing `{4, 5, 6}` is added to the end of `vec`. The `vec.end()` iterator indicates the end of the current vector, where new elements will be inserted.
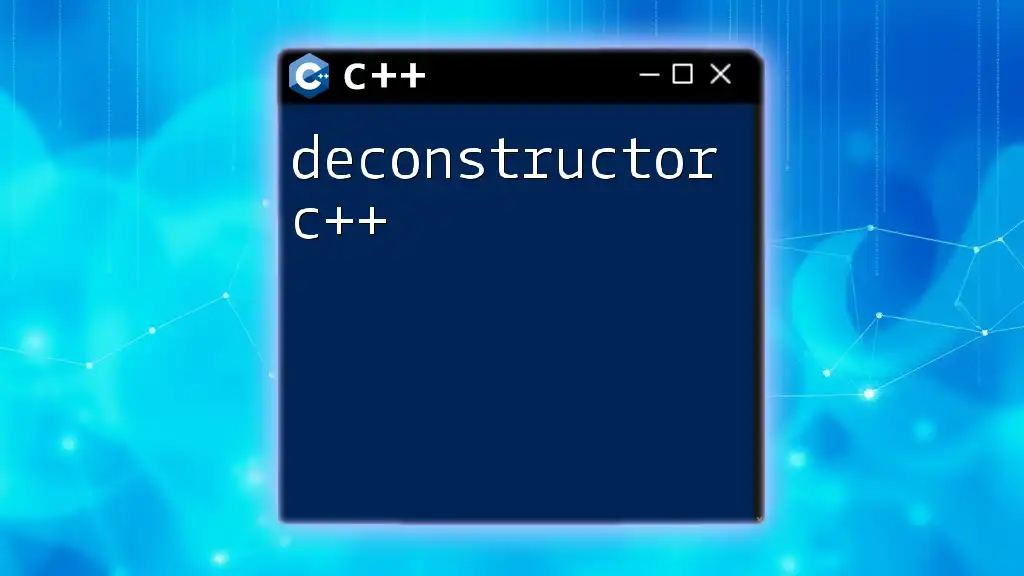
Common Mistakes to Avoid
Exceeding Capacity
One common issue when working with vectors is exceeding their capacity. When you add too many elements, the vector automatically resizes, which can be costly in terms of performance.
Solution: Consider using the `reserve()` method to allocate enough memory ahead of time if you know the expected size.
Mixing Data Types
C++ vectors must contain elements of the same type, and attempting to mix types will result in a compilation error.
Solution: Always ensure that the data type of the elements you are adding is consistent with what the vector was defined to hold.
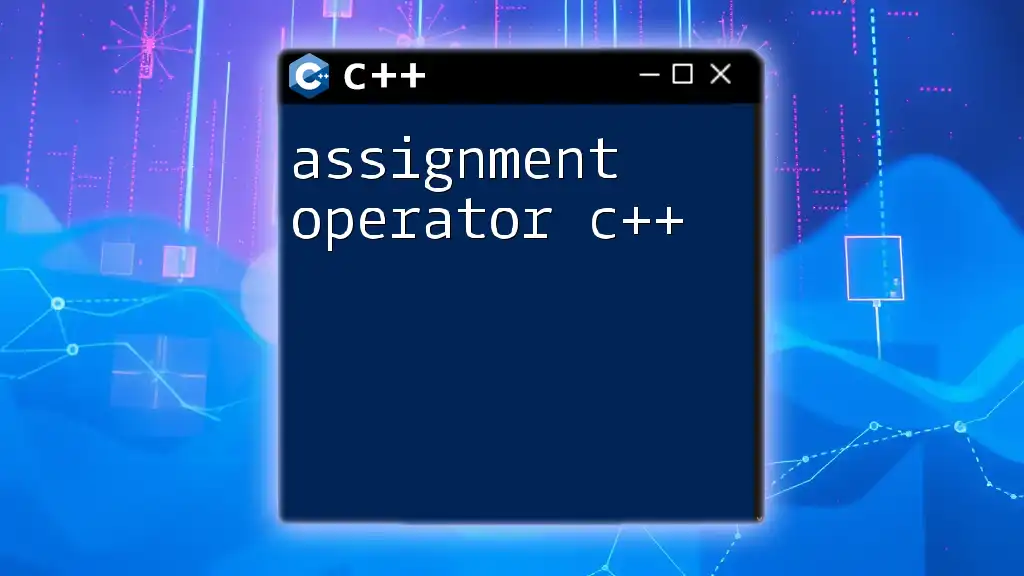
Best Practices for Adding Elements
Choosing the Right Method
Understanding when to use `push_back`, `insert`, or `emplace_back` greatly impacts usability and performance.
- Use `push_back()` when frequently adding elements to the end of the vector.
- Use `insert()` when you need to add elements elsewhere in the vector.
- Use `emplace_back()` if constructing an object in place will enhance efficiency, especially for classes with overhead in their constructors.
Pre-allocating Space
When dealing with large vectors, consider using the `reserve()` method, which pre-allocates space without initializing the elements. This leads to improved performance for large datasets.
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
vec.reserve(100); // Reserve space for 100 elements
for (int i = 0; i < 100; i++) {
vec.push_back(i);
}
std::cout << "Capacity: " << vec.capacity() << " Size: " << vec.size();
return 0;
}
Explanation: This code snippet demonstrates how to reserve space for 100 integers in the vector. This is particularly useful for reducing the number of reallocations as the vector grows.
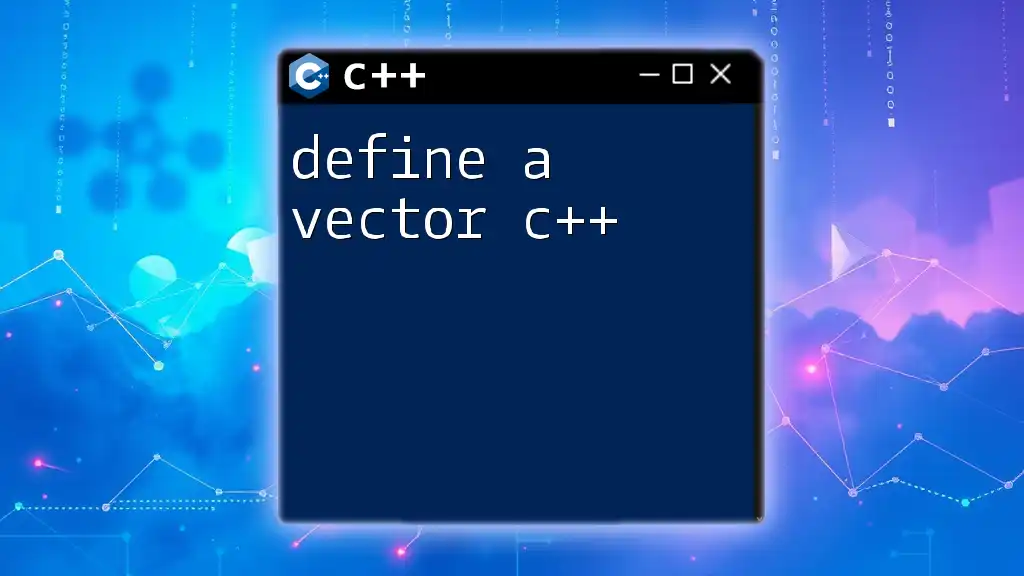
Conclusion
In summary, understanding how to add elements to a vector in C++ is an essential skill for effective programming. By mastering methods like `push_back()`, `insert()`, and `emplace_back()`, you can efficiently manage and manipulate data structures. Be mindful of common pitfalls like exceeding capacity and maintaining type consistency, and consider best practices to enhance performance.
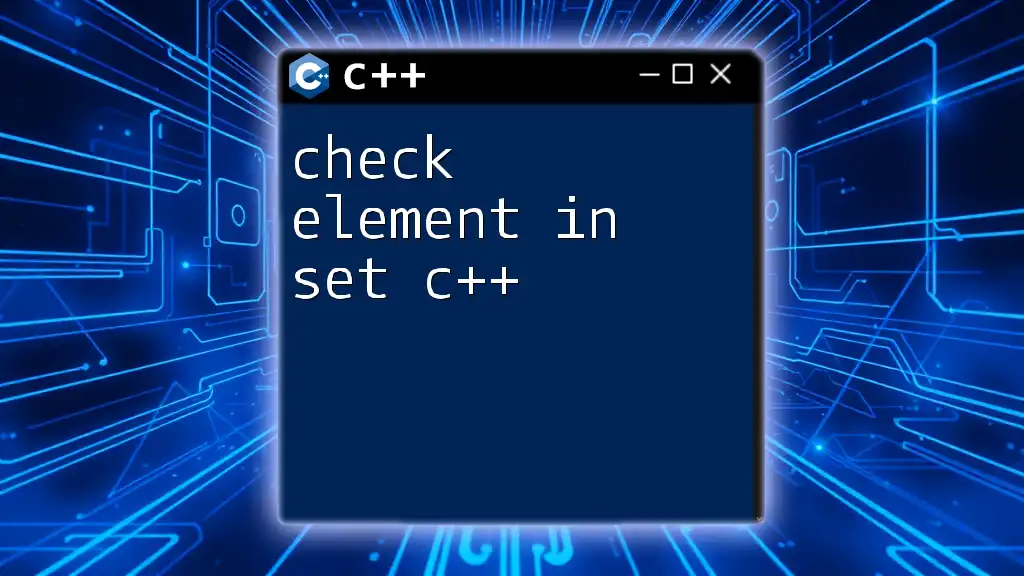
Call to Action
Join our community to receive more quick guides and tips on C++ commands. Feel free to share your experiences, questions, and learning challenges in the comments below!