In C++, the increment (`++`) and decrement (`--`) operators are used to increase or decrease the value of a variable by one, respectively, and they can be applied in either a prefix or postfix manner.
Here's a code snippet demonstrating their usage:
#include <iostream>
using namespace std;
int main() {
int a = 5;
int b = 5;
cout << "Prefix Increment: " << ++a << endl; // Outputs 6
cout << "Postfix Increment: " << b++ << endl; // Outputs 5 but b becomes 6
cout << "Current value of b: " << b << endl; // Outputs 6
int c = 5;
int d = 5;
cout << "Prefix Decrement: " << --c << endl; // Outputs 4
cout << "Postfix Decrement: " << d-- << endl; // Outputs 5 but d becomes 4
cout << "Current value of d: " << d << endl; // Outputs 4
return 0;
}
Overview of C++ Operators
C++ is a powerful programming language known for its efficiency and flexibility. Within C++, operators play a critical role in manipulating data. Among these operators, the increment and decrement operators are essential as they facilitate quick arithmetic operations, especially when iterating through loops or modifying values.
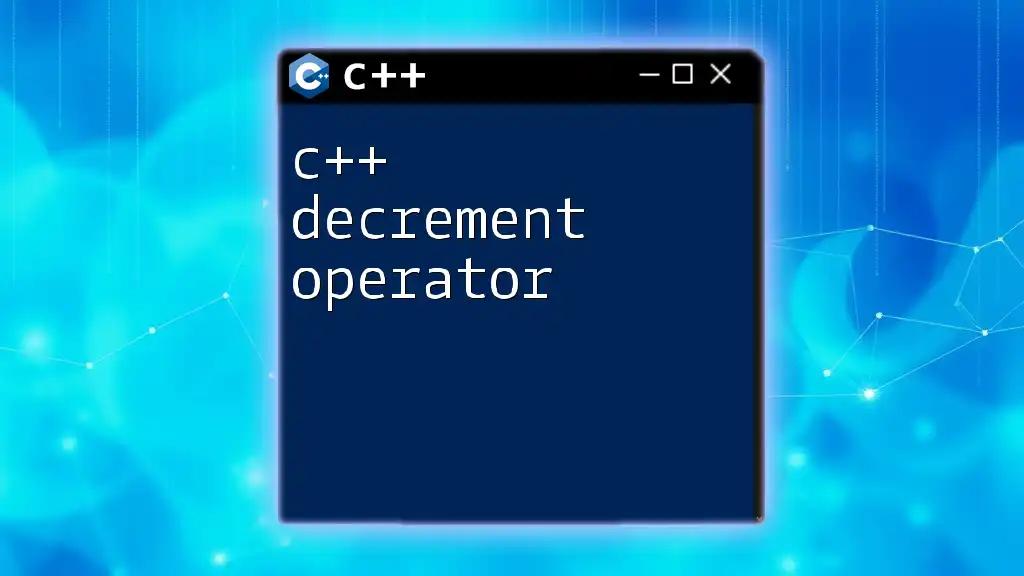
Importance of Increment and Decrement Operators in Programming
The increment and decrement operators enhance code readability and efficiency. They are preferred for altering values because they allow developers to modify variables concisely within expressions. By tapping into these operators, you can write cleaner and more efficient loops, making your code easier to maintain and understand.
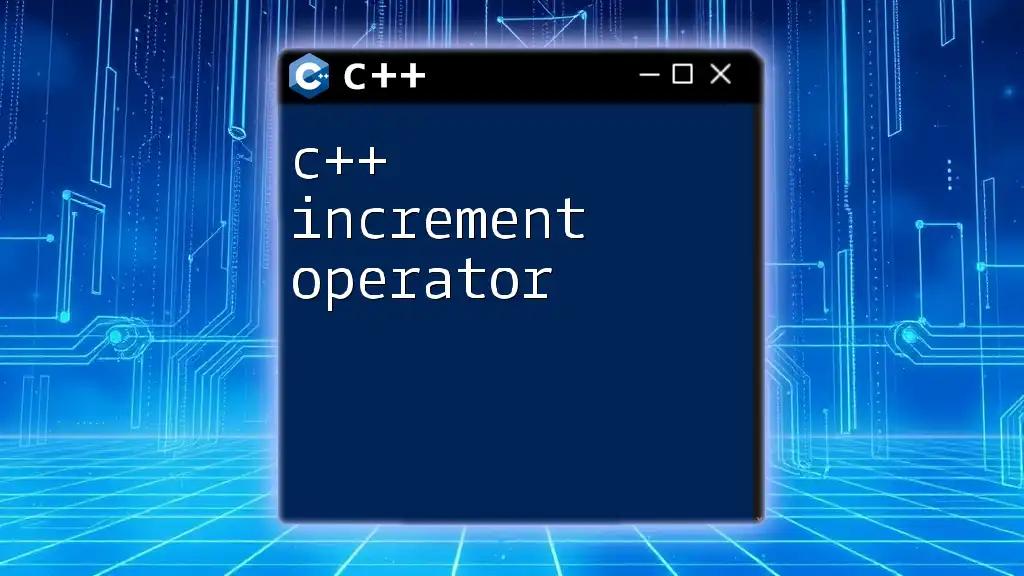
What are Increment and Decrement Operators?
In C++, increment operators (`++`) increase the value of a variable by one, while decrement operators (`--`) decrease it by one. These operations can be either prefix or postfix, meaning their effect can occur before or after the variable is used in an expression. Understanding the distinction between these two forms is crucial for avoiding subtle bugs in your code.

Prefix Increment Operator
The prefix increment operator (`++variable`) first increments the value of the variable and then returns that value.
Example Code Snippet
int a = 5;
int b = ++a; // a becomes 6, b is 6
In this example, `a` is incremented before its value is assigned to `b`. Thus, `b` receives the updated value of `a`.
Explanation of Example
Here, as the operation advances, `a` changes from 5 to 6, and since `b` is assigned the value of `a` after the increment, it also becomes 6. Understanding this flow is key for effective variable manipulation.
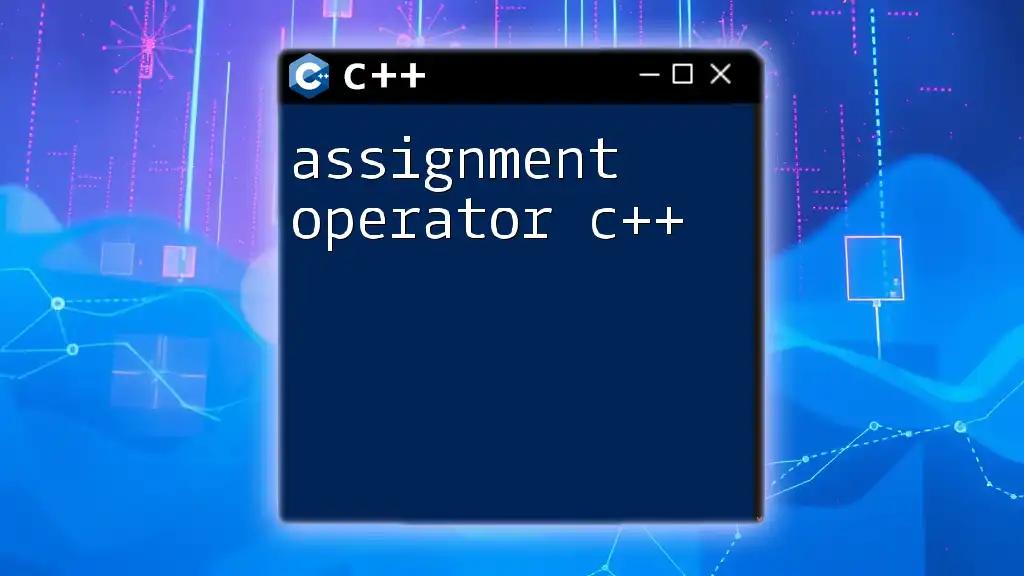
Postfix Increment Operator
The postfix increment operator (`variable++`) returns the value of the variable before applying the increment.
Example Code Snippet
int a = 5;
int b = a++; // a becomes 6, b is 5
Explanation of Example
In this scenario, the initial value of `a` (which is 5) is assigned to `b` before the increment takes place. As a result, `a` updates to 6, but `b` remains 5. This distinction can have significant implications when used in complex expressions.
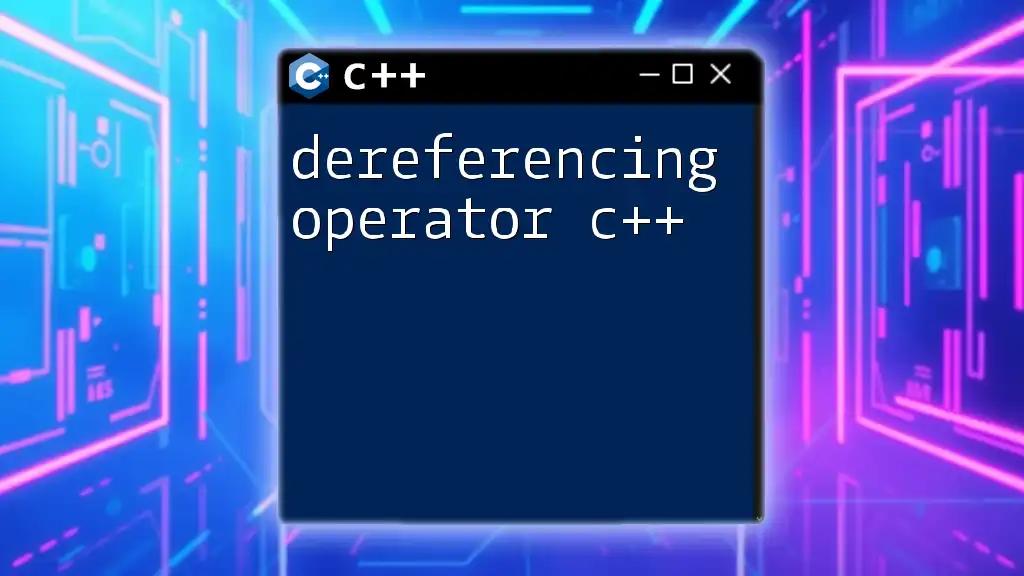
Prefix Decrement Operator
The prefix decrement operator (`--variable`) decreases the value before returning it.
Example Code Snippet
int a = 5;
int b = --a; // a becomes 4, b is 4
Explanation of Example
Just like with the prefix increment operator, the value of `a` is decremented first, changing it from 5 to 4, and this updated value is then assigned to `b`.
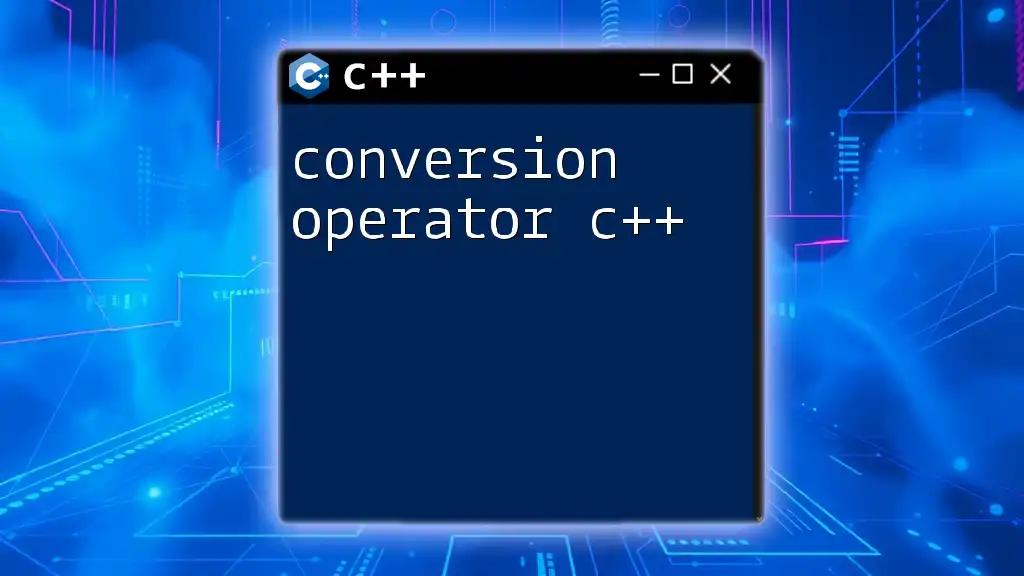
Postfix Decrement Operator
The postfix decrement operator (`variable--`) operates similarly to its counterpart, delaying the decrement action until after its current value is used.
Example Code Snippet
int a = 5;
int b = a--; // a becomes 4, b is 5
Explanation of Example
In this instance, `b` gets the original value of `a` (5), and then `a` decreases to 4 afterward. This logic is crucial when relying on the variable's value before it’s modified.
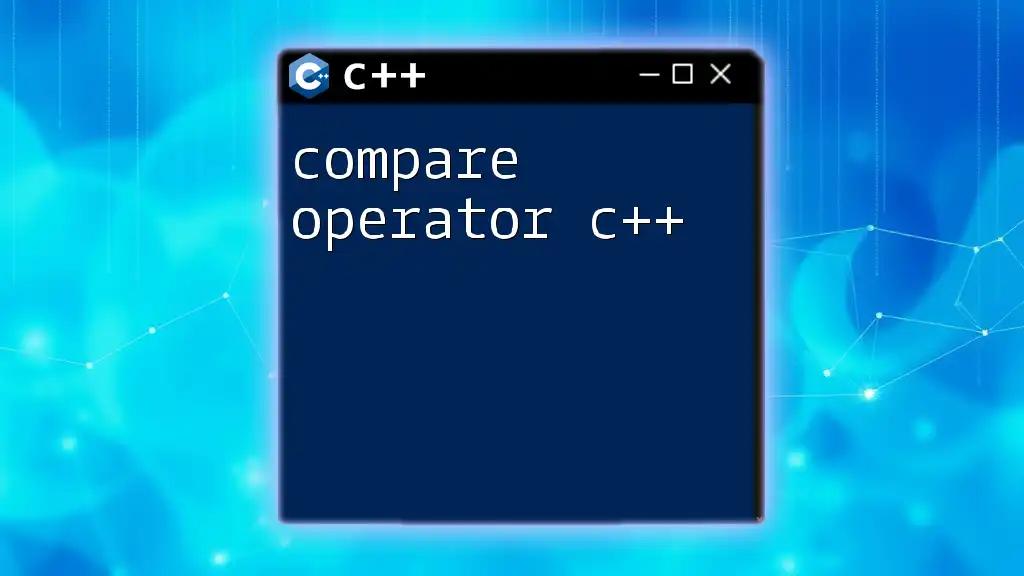
Practical Applications of Increment and Decrement Operators
Using with Looping Constructs
Increment and decrement operators are frequently utilized in loops, simplifying iteration.
For Loops: Example of Increment
The increment operator is commonly used in `for` loops to navigate through sequences:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
In this `for` loop, the increment operator increases `i` after each iteration, progressing from 0 to 4.
While Loops: Example of Decrement
Decrement operators are ideal for counters in reverse iterations:
int i = 5;
while (i--) {
std::cout << i << " ";
}
This loop prints numbers in descending order from 4 to 0, showcasing the decrementing effect.
Manipulating Arrays and Collections
Increment and decrement operators are invaluable for index manipulation when working with arrays or collections, allowing seamless iteration.
Example Code Snippet
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; ++i) {
std::cout << arr[i] << " ";
}
In this case, `i` serves as an index for accessing elements in the array. Using the increment operator here enhances clarity for other developers reading the code.
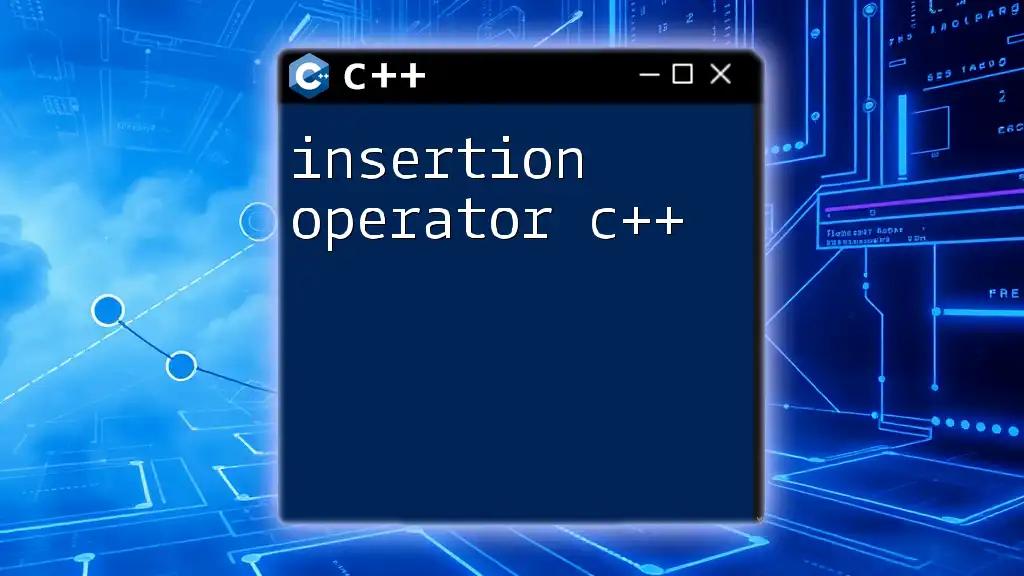
Best Practices and Common Pitfalls
Choosing between Prefix and Postfix
Deciding whether to use prefix or postfix increment/decrement depends on the context. Prefix versions can be more efficient in certain cases since they do not require a temporary variable to hold the old value.
Avoiding Unintended Consequences
One common mistake made with increment and decrement operators is misordering them in complex expressions which can lead to undefined behavior. Being mindful of operator precedence will help avoid these traps.

Recap of Increment and Decrement Operators in C++
In summary, the increment and decrement operators in C++ are crucial tools that facilitate concise manipulation of variables. Their prefix and postfix forms allow for versatile programming patterns, specifically in loops and data management. A solid grasp of these operators can significantly improve your coding efficacy.
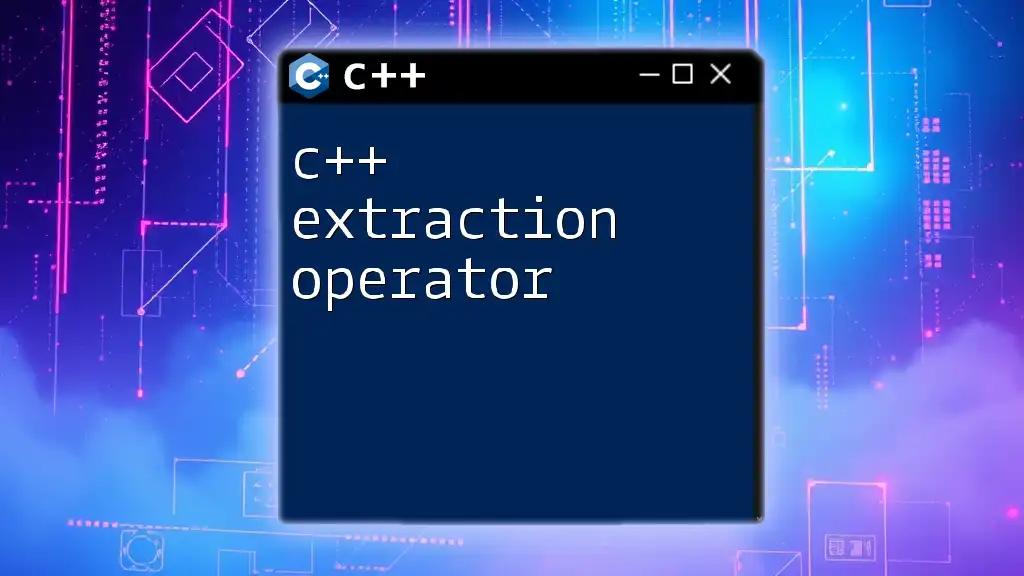
Frequently Asked Questions
What is the output when using both prefix and postfix in the same expression?
Using both forms in a single expression can lead to ambiguous results, so it's essential to understand their order of operations and scope.
How do these operators impact performance?
While the performance impact is minimal in most cases, using prefix over postfix may yield slight efficiencies, especially in loops.
Can increment and decrement operators be overloaded?
Yes, C++ allows overloading these operators, offering additional flexibility and customization as per user-defined types.

Additional Resources
For those looking to deepen their understanding of increment and decrement operators in C++, consider exploring online C++ compilers for hands-on practice, recommended C++ programming books for comprehensive learning, and forums or communities where you can connect with fellow developers to discuss strategies and share knowledge.