In C++, the heap is a region of memory used for dynamic memory allocation, allowing programs to allocate and deallocate memory during runtime as needed.
Here’s a simple code snippet demonstrating the allocation and deallocation of memory on the heap:
#include <iostream>
int main() {
// Allocate memory on the heap
int* ptr = new int(42);
// Use the allocated memory
std::cout << "Value: " << *ptr << std::endl;
// Deallocate the memory
delete ptr;
return 0;
}
Understanding Memory Allocation in C++
In C++, memory is managed through several areas, primarily the stack and the heap. Understanding the distinctions between these two types of memory allocation is crucial for effective C++ programming.
Stack Memory
Stack memory is a region of memory that stores temporary variables created by a function. It is known for its fast access speed, as memory allocation and deallocation are handled in a last-in-first-out (LIFO) manner. Variables stored in the stack are automatically freed when the function call is completed. However, stack memory has limitations:
- Fixed Size: The size of stack memory is determined at compile time and cannot be changed at runtime.
- Lifetime Management: The lifetime of stack variables is limited to the scope of the function.
Heap Memory
In contrast, heap memory is used for dynamic memory allocation. It allows programmers to allocate memory at runtime, offering the flexibility needed for complex data structures and larger data sets. The heap does come with its own set of characteristics:
- Variable Size: The heap can grow as needed, as long as there is available memory.
- Manual Management: Developers are responsible for deallocating memory, which can lead to errors if not managed correctly.
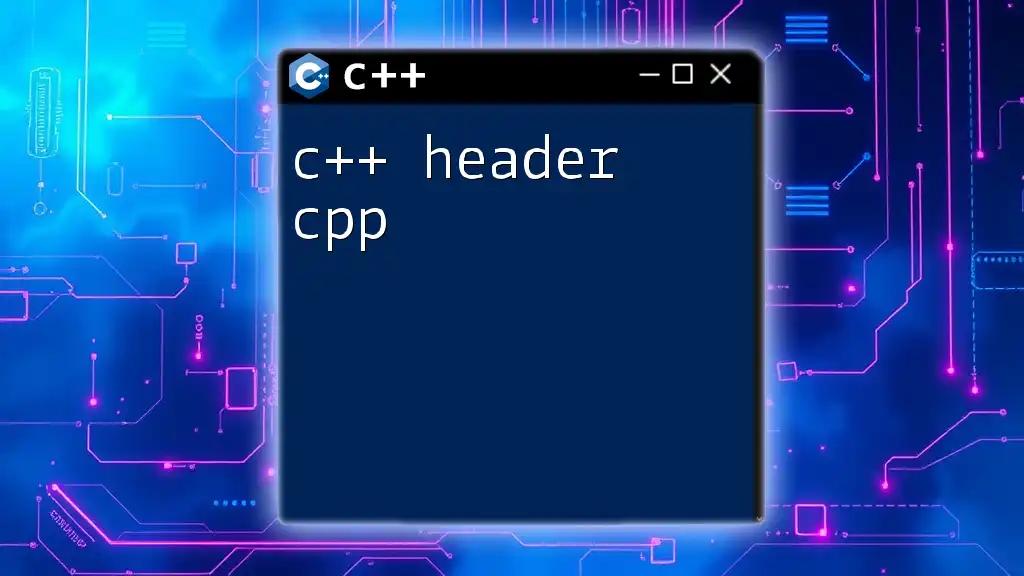
Dynamic Memory Allocation
Dynamic memory allocation is a critical feature in C++. It allows developers to request memory from the heap for variable-sized data structures.
How to Allocate Memory on the Heap
To allocate memory on the heap, the `new` operator is used. This operator returns a pointer to the newly allocated memory.
For example, if you want to create an array of integers, you can do so like this:
int* myArray = new int[10]; // Allocating an array of 10 integers
This line of code allocates an array that can hold 10 integers, and `myArray` points to this memory.
How to Deallocate Memory
It is essential to free up memory once it is no longer needed to avoid memory leaks. The `delete` operator is used for this purpose. If you allocated an array using `new`, you should use `delete[]` to free it:
delete[] myArray; // Deallocating the array
Failing to deallocate the heap memory will lead to memory leaks, which can hinder program performance and resource availability.
Mistakes to Avoid
When dealing with heap memory in C++, it is easy to make accidental errors:
- Memory Leaks: Not freeing allocated memory can result in leaks, as the memory remains permanently allocated. Always ensure that every allocation has a corresponding deallocation.
- Double Deletions: Trying to delete memory that has already been freed can lead to undefined behavior. It's important to set pointers to `nullptr` after deletion to avoid such errors.

Advantages of Using Heap Memory
Heap memory comes with several advantages that make it useful for various programming scenarios:
Flexibility in Memory Management
The heap allows you to allocate memory dynamically, which is particularly useful for building complex data structures like arrays, linked lists, and trees. This flexibility enables you to manage varying amounts of data without worrying about the fixed sizes of stack allocations.
Lifetime of Allocated Memory
Variables allocated on the heap live until they are explicitly deallocated. This is beneficial when you need data to persist beyond the scope of the function that created it. For example, you can allocate memory for data that a function returns, ensuring that the caller can use it as long as it’s needed.
Data Structures Utilization
Complex data structures rely heavily on heap memory to manage their dynamic nature. For instance, linked lists use nodes that are typically allocated on the heap to allow for dynamic insertion and deletion.

Disadvantages of Using Heap Memory
While beneficial, heap memory also has notable disadvantages:
Slower Access Speed
Accessing memory from the heap is generally slower than accessing the stack due to the nature of dynamic allocation and pointer arithmetic. This can impact performance, especially in applications where speed is critical.
Fragmentation
Over time, the allocation and deallocation of chunks of memory on the heap can lead to fragmentation. This makes it challenging to allocate large contiguous blocks of memory, as gaps between used spaces enlarge.
Complexity in Management
Managing heap memory adds complexity to your program. Developers must ensure they properly allocate and deallocate memory, which introduces the potential for errors if not handled correctly.

Smart Pointers and Modern C++
To mitigate many of the issues associated with manual memory management, C++11 introduced smart pointers, a powerful feature that simplifies memory management.
Introduction to Smart Pointers
Smart pointers automatically manage memory through reference counting and scoped management. They ensure that memory is properly deallocated without leading to memory leaks or dangling pointers.
Types of Smart Pointers
-
`std::unique_ptr`: Represents exclusive ownership of dynamically allocated memory. The memory is automatically released when the `unique_ptr` goes out of scope.
Example:
std::unique_ptr<int> p1(new int(5)); // Ownership is unique
-
`std::shared_ptr`: Allows multiple pointers to share ownership of a single resource, automatically managing the reference count.
Example:
std::shared_ptr<int> p2 = std::make_shared<int>(10); // Shared ownership
-
`std::weak_ptr`: A weak reference to an object managed by `shared_ptr`, preventing cyclic references and memory leaks.
Benefits of Using Smart Pointers
Smart pointers provide several advantages:
- Automatic Memory Management: They automatically invoke destructors when they go out of scope, reducing the risk of leaks.
- Safety and Convenience: They simplify pointer usage and encapsulate resource management, leading to safer code.
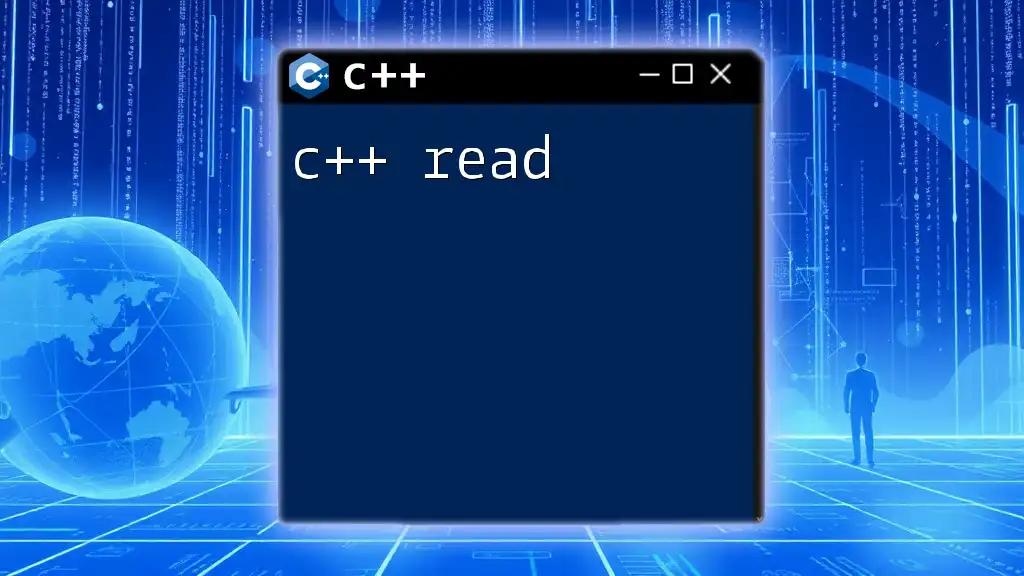
Common Heap-related Errors and Debugging
Even with careful management, heap memory can produce errors:
Memory Leaks
Memory leaks occur when dynamically allocated memory is not deallocated. This can lead to decreased performance and crashes. Tools like Valgrind are invaluable for detecting memory leaks in your applications.
Dangling Pointers
Dangling pointers occur when you access memory after it has been deallocated. For example, if a pointer points to a memory location that has been freed, it leads to undefined behavior. Always ensure that a pointer is set to `nullptr` after deletion.
Segmentation Faults
A segmentation fault happens when a program tries to access memory it is not allowed to. Common causes include dereferencing wild pointers or accessing memory beyond allocated boundaries.
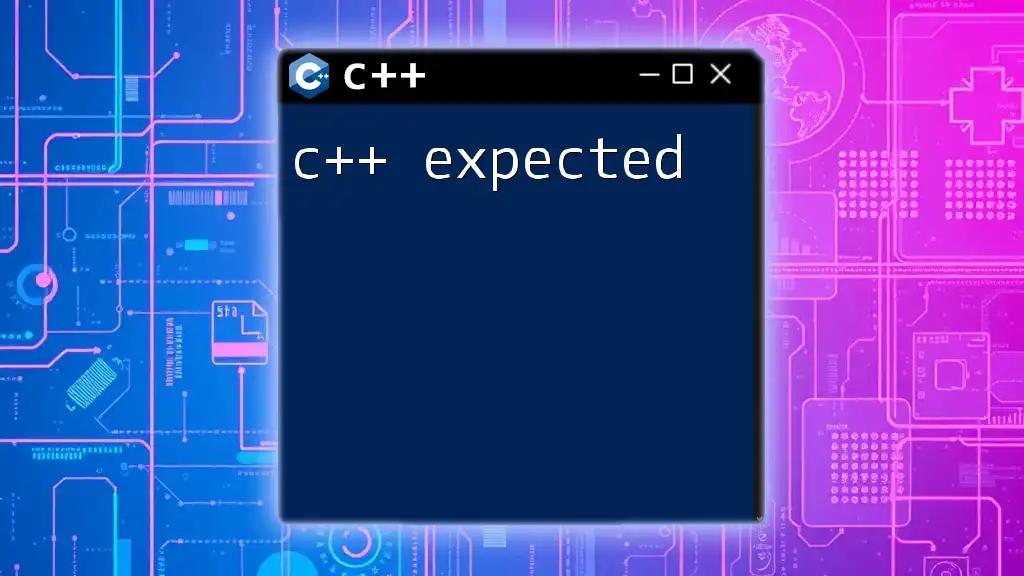
Best Practices for Heap Management
Navigating heap memory effectively requires adherence to best practices:
When to Use the Heap
Consider the heap for data that:
- Needs to persist beyond the function scope.
- Requires dynamic memory sizing.
- Is part of complex data structures that change in size frequently.
Resource Management Techniques
Follow the RAII (Resource Acquisition Is Initialization) pattern. This concept ensures resources are tied to object lifetimes, naturally managing allocation and deallocation. For example:
class Resource {
public:
Resource() { /* Allocate */ }
~Resource() { /* Deallocate */ }
};
By using RAII, you ensure that resources are automatically released when the object goes out of scope, reducing the complexity of memory management.

Conclusion
Understanding the intricacies of the C++ heap is vital for efficient memory management in modern programming. Balancing the benefits of dynamic memory allocation with its potential pitfalls is crucial for developing robust C++ applications. By utilizing modern techniques such as smart pointers and adhering to best practices, developers can mitigate risks while harnessing the full power of heap memory.