A C++ app typically refers to a software application developed using the C++ programming language, enabling users to harness powerful features and performance for various computational tasks.
Here's a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Apps
A C++ app can be defined as a software application developed using the C++ programming language. These applications can vary from simple console-based programs to complex graphical user interfaces (GUIs). Understanding the type of app you want to create is fundamental to your approach.
Console applications are typically easier to develop and run in a command-line interface, whereas GUI applications provide a user-friendly interface and can handle more complex interactions.
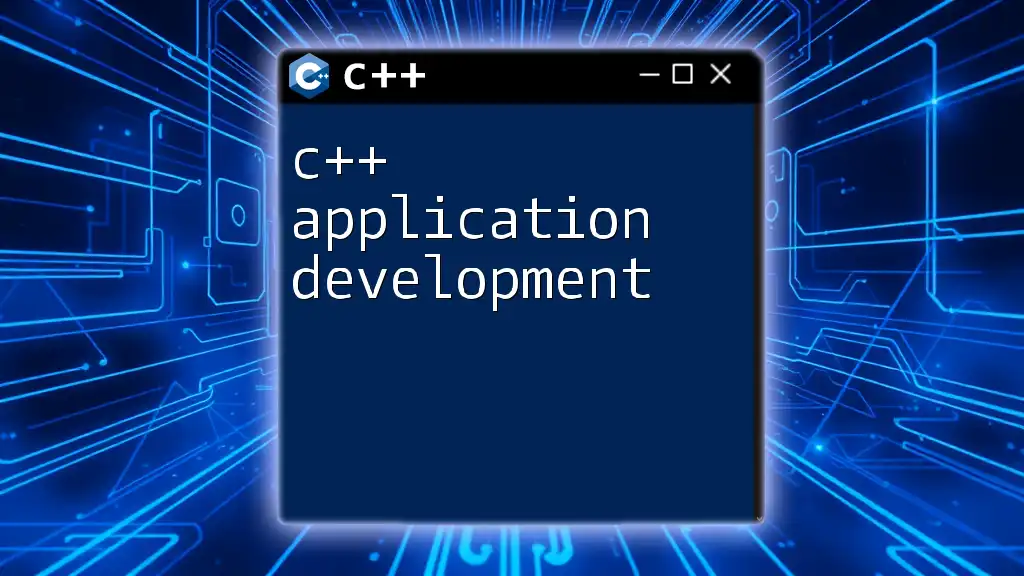
Setting Up Your C++ Development Environment
Choosing the Right Tools
To start developing your C++ app, you first need the right tools. Consider the following popular Integrated Development Environments (IDEs) and compilers:
-
IDE Options:
- Code::Blocks: Open-source and highly customizable.
- Visual Studio: Best for Windows development with extensive features.
- CLion: A professional-grade IDE from JetBrains.
-
Compilers:
- GCC: Widely used and available for multiple platforms.
- MSVC: Microsoft's compiler, especially strong for Windows applications.
- Clang: Known for its fast compilation and excellent diagnostics.
Installing the Development Tools
Once you've chosen your tools, the next step is to install them. Follow the instructions specific to your OS to get started with your selected IDE and compiler. Many IDEs also come with built-in compilers, simplifying this process.
Testing Your Installation
To ensure everything is set up correctly, test your installation with a simple program. Type the following code into your IDE:
#include <iostream>
using namespace std;
int main() {
cout << "Installation successful! Welcome to C++." << endl;
return 0;
}
Compile and run this application. If you see the output, you’re ready to start developing your C++ app!
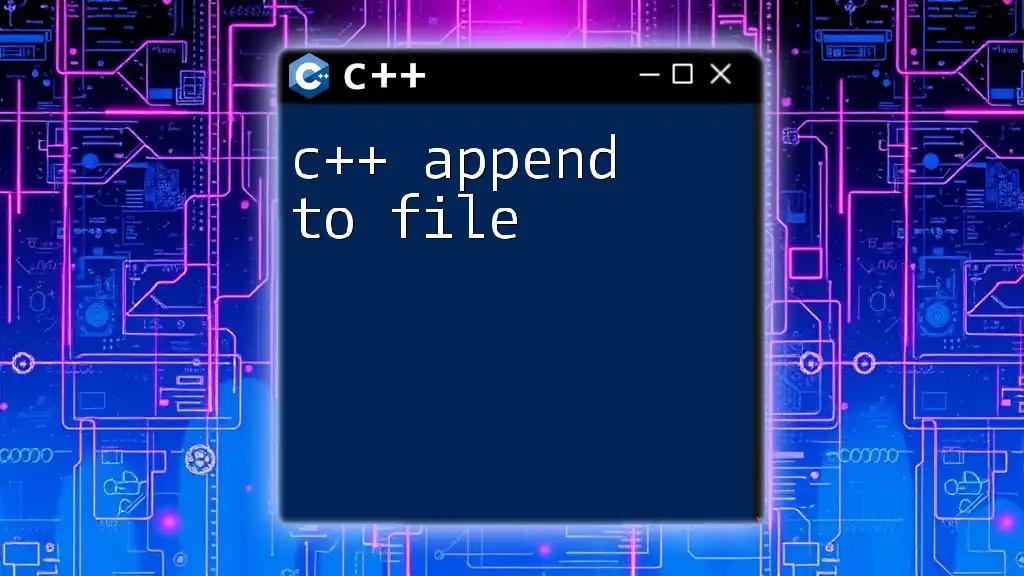
Basic C++ Concepts for App Development
Syntax and Structure
Understanding the basic structure of a C++ program is essential. Every C++ app starts with a `main()` function, where the execution of your program begins. Here’s a simple structure to illustrate this:
int main() {
// Your code here
return 0;
}
Data Types and Variables
C++ supports several data types, including integers, floats, characters, and strings. Knowing how to declare and initialize variables is crucial.
Example:
int age = 30;
float salary = 75000.50;
string name = "Alice";
In this example, we declare three variables that can be used throughout the app.
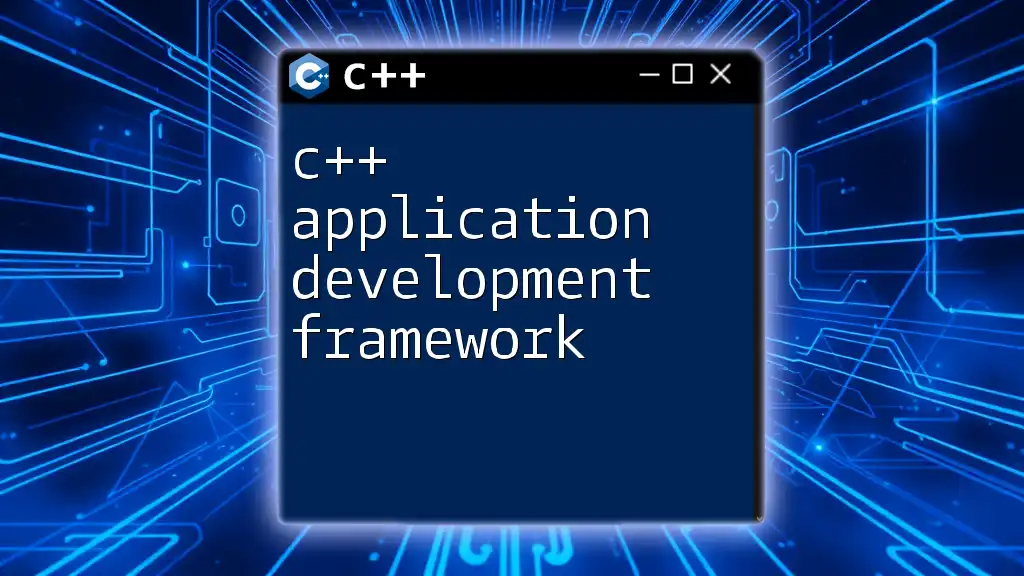
Building Your First C++ Application
Creating a Simple Console Application
Now that you understand the basic structure and data types, it’s time to create your first console application. Here’s how to do it:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to C++ Application!" << endl;
return 0;
}
Compiling and Running Your Application
After you write the code, you’ll need to compile your application using the appropriate command for your compiler. For G++, use:
g++ yourfile.cpp -o yourapp
Run your program by typing:
./yourapp
Congratulations! You've just built your first C++ app!
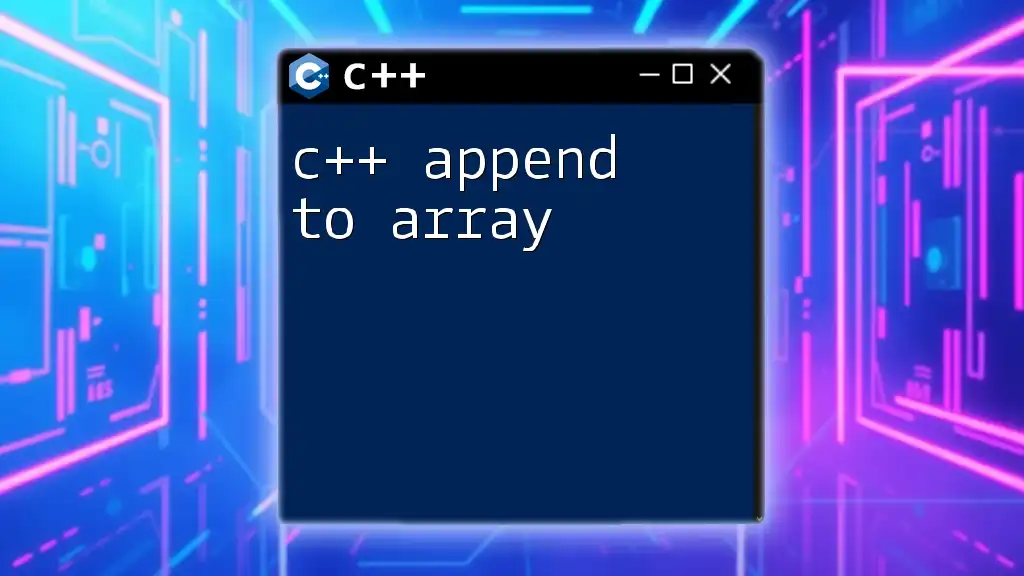
Handling User Input
Reading Input from Users
To make your C++ app interactive, you can read inputs from users. Use the `cin` command for this purpose:
string name;
cout << "Enter your name: ";
cin >> name;
cout << "Hello, " << name << "!" << endl;
This simple program will prompt the user to enter their name and display a greeting.
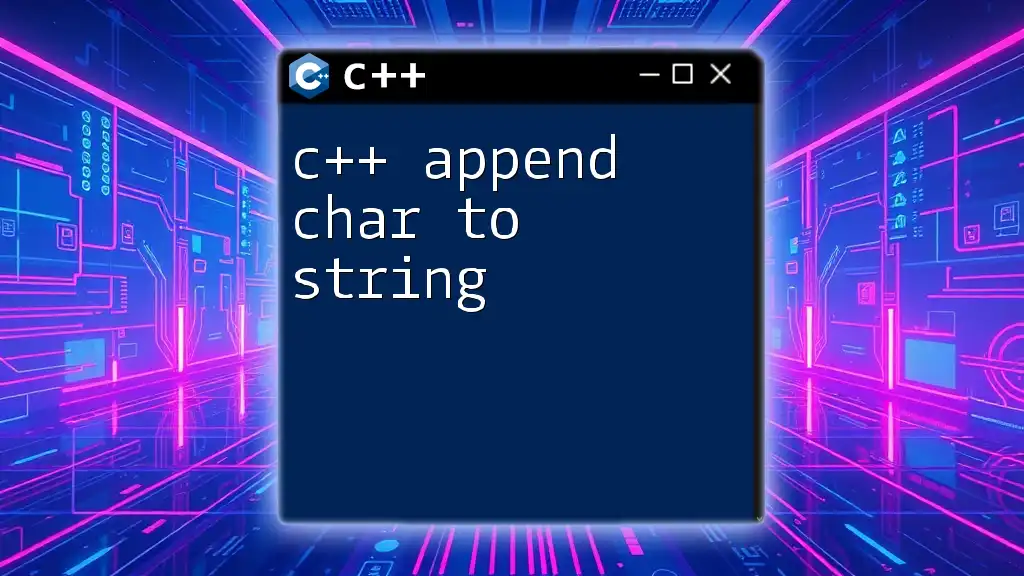
Control Structures in C++
Conditional Statements
Control structures allow your program to make decisions based on conditions. C++ offers `if`, `else if`, and `else` statements. Here’s an example:
if (age >= 18) {
cout << "You are an adult." << endl;
} else {
cout << "You are a minor." << endl;
}
Loops
Loops enable your app to repeat actions. C++ supports various loops, such as `for`, `while`, and `do-while`. Here’s a simple `for` loop:
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
This will print numbers 0 through 4.
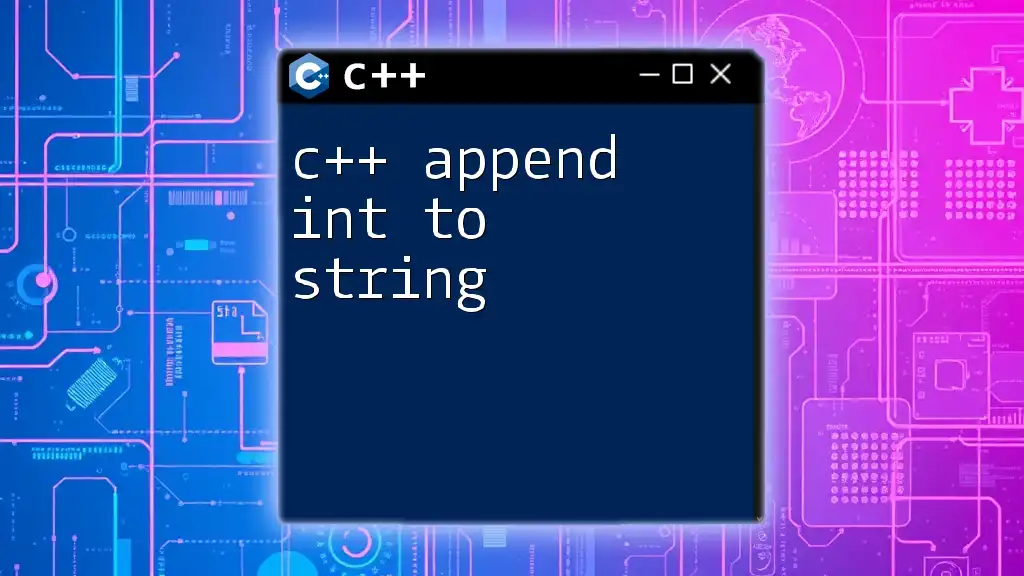
Functions in C++
Defining and Using Functions
Functions are essential for organizing code and promoting reusability. Here’s how to define a simple function:
int add(int a, int b) {
return a + b;
}
You can call this function in your main application to perform additions efficiently.
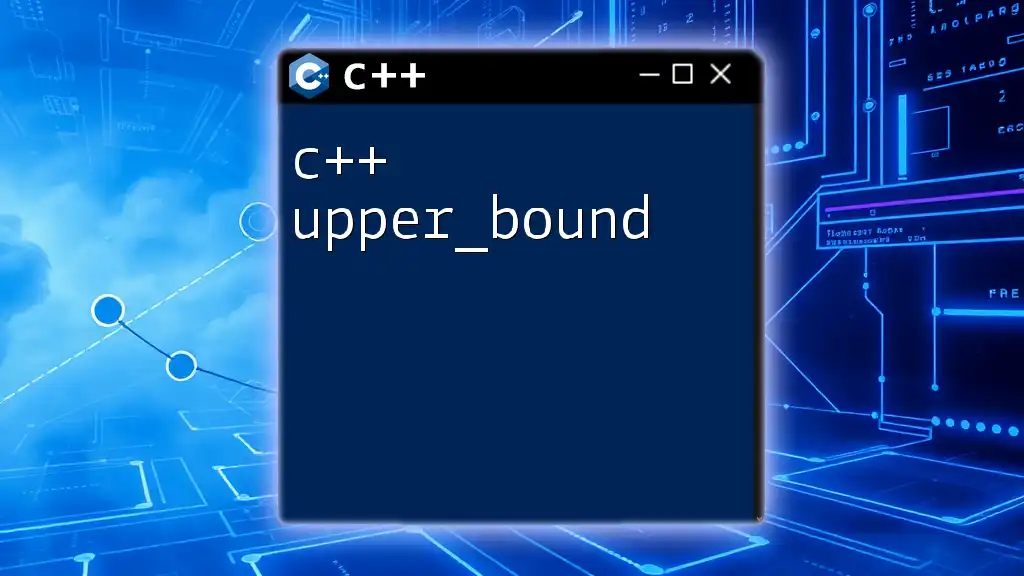
Introduction to Object-Oriented Programming
Concepts of OOP in C++
C++ is renowned for its support of Object-Oriented Programming (OOP), which includes concepts like classes, objects, inheritance, and polymorphism. These principles simplify complex program structures.
Creating a Class
To define a class in C++, you can use the following syntax:
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
You can create objects of the `Dog` class and invoke its methods.
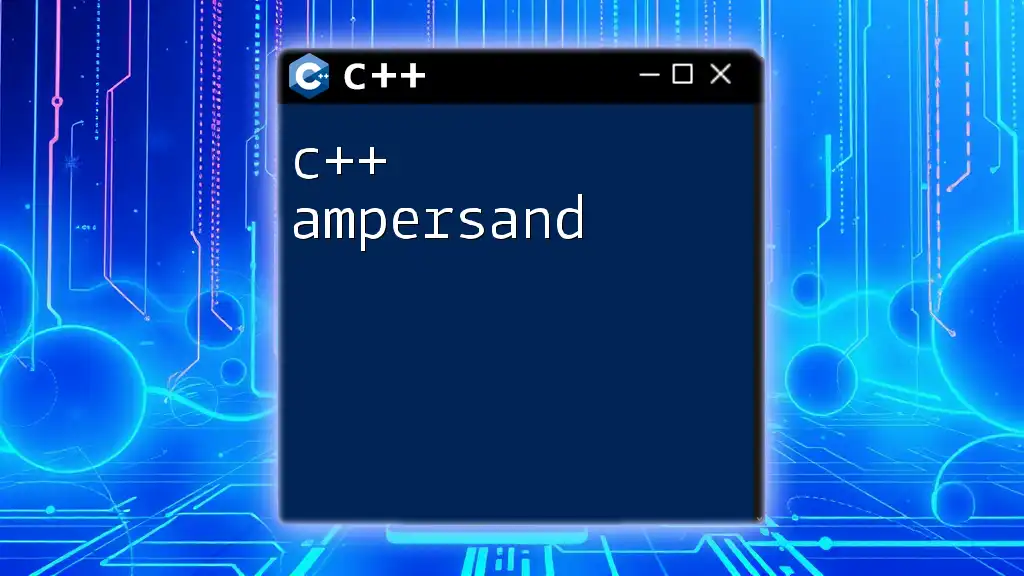
Building a Simple GUI Application (Optional)
Using Libraries for GUI
To build a GUI application in C++, you might consider libraries such as Qt or wxWidgets. These frameworks allow you to create visually appealing applications with comprehensive user interaction.
Basic Example of a GUI Application
While building a GUI app can be intricate, here’s a basic outline:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello World!");
button.show();
return app.exec();
}
Compile this with `Qt` tools to see a simple window with a button.
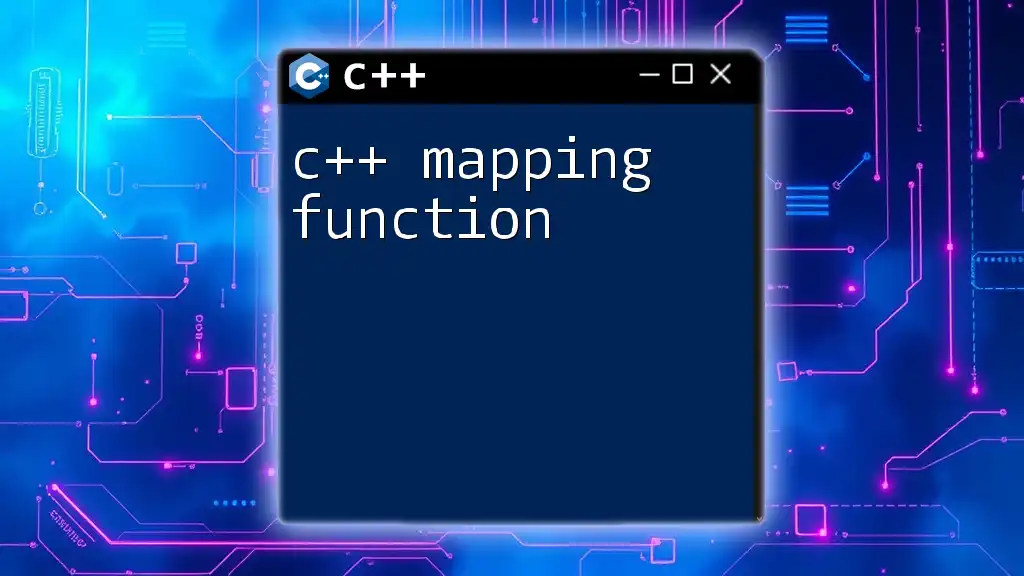
Debugging and Error Handling
Common C++ Errors
As you develop your C++ app, you will encounter both syntax errors and logical issues. Grasping the common types of errors, such as mismatched brackets or incorrect variable types, is essential for troubleshooting.
Utilizing Debuggers
Use a debugger integrated into your IDE to inspect your code line-by-line. This will help you identify where things might be going wrong and provide insights on how to resolve those issues.
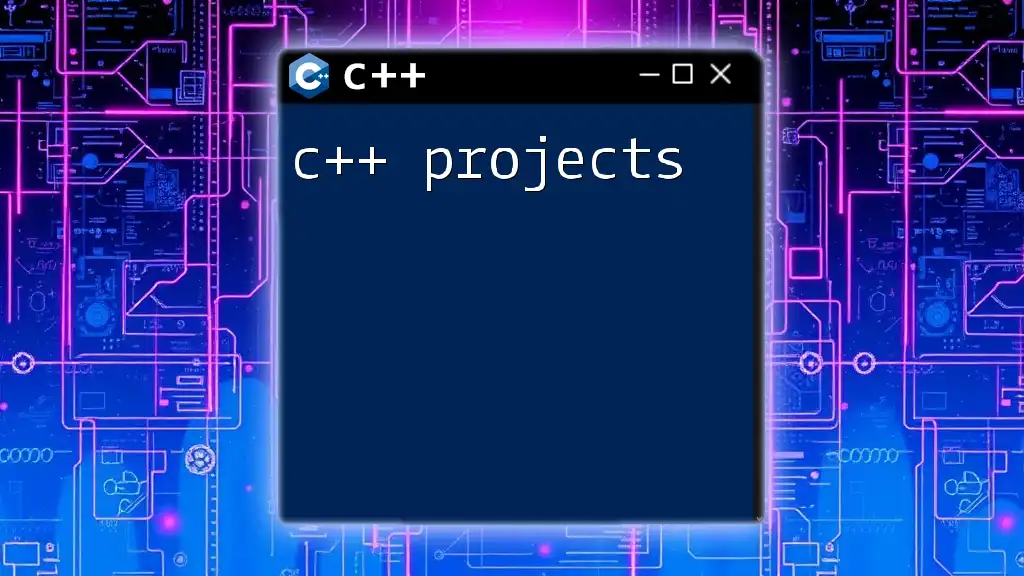
Conclusion
This guide provides you with a comprehensive overview of building a C++ app. With essential concepts like syntax structure, user input, control structures, functions, and object-oriented programming, you’re now equipped to start developing your applications.
Don't forget to continue learning through resources like online tutorials, books, and community forums. Your journey into the world of C++ is just beginning, and there’s a lot more to discover and create!
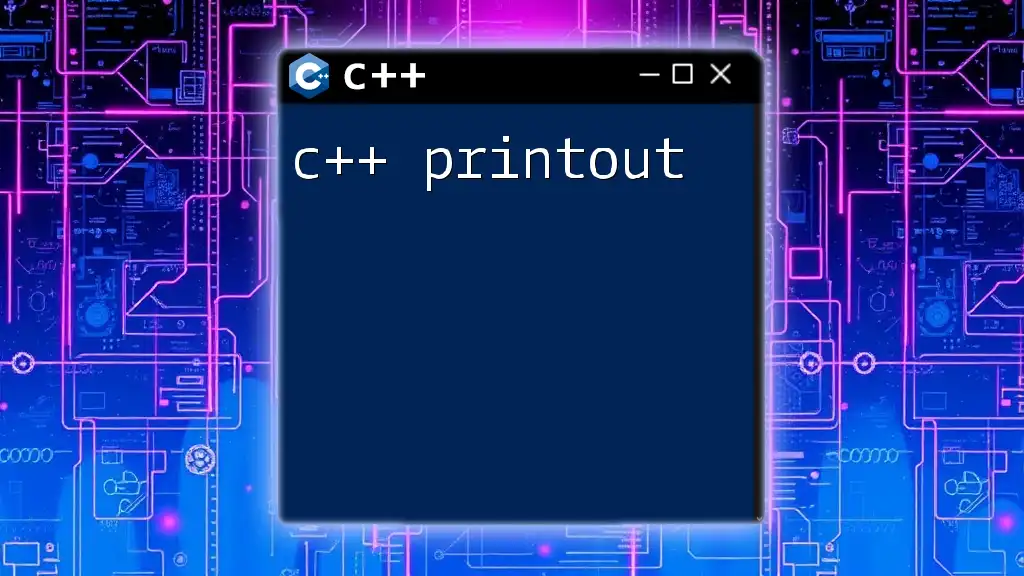
Additional Resources
For further exploration, consider looking into:
- C++ documentation for in-depth explanations on the language features.
- Online platforms like Codecademy, Coursera, or Udemy for hands-on courses.
- C++ forums and communities for problem-solving and knowledge sharing.