C++ AI refers to the use of C++ programming language to develop artificial intelligence applications, leveraging its performance and efficiency for tasks such as machine learning and neural networks.
Here's a simple example of how to use a C++ library for machine learning, specifically the k-Nearest Neighbors (k-NN) algorithm:
#include <iostream>
#include <vector>
#include <cmath>
class KNN {
public:
void fit(const std::vector<std::vector<float>>& data, const std::vector<int>& labels) {
this->data = data;
this->labels = labels;
}
int predict(const std::vector<float>& point, int k) {
std::vector<std::pair<float, int>> distances;
for (size_t i = 0; i < data.size(); ++i) {
float distance = euclideanDistance(data[i], point);
distances.push_back({distance, labels[i]});
}
std::sort(distances.begin(), distances.end());
// Count the votes for each class
std::map<int, int> votes;
for (int i = 0; i < k; ++i) {
votes[distances[i].second]++;
}
return std::max_element(votes.begin(), votes.end(),
[](const auto& a, const auto& b) { return a.second < b.second; })->first;
}
private:
std::vector<std::vector<float>> data;
std::vector<int> labels;
float euclideanDistance(const std::vector<float>& a, const std::vector<float>& b) {
float sum = 0.0;
for (size_t i = 0; i < a.size(); ++i) {
sum += (a[i] - b[i]) * (a[i] - b[i]);
}
return std::sqrt(sum);
}
};
What is C++?
C++ is a high-performance programming language that extends the capabilities of the C language with object-oriented features such as classes and inheritance. Developed by Bjarne Stroustrup in the early 1980s, C++ has become a vital language for software development due to its flexibility, efficiency, and control over system resources. Its applications range from system programming to game development and, increasingly, artificial intelligence (AI).
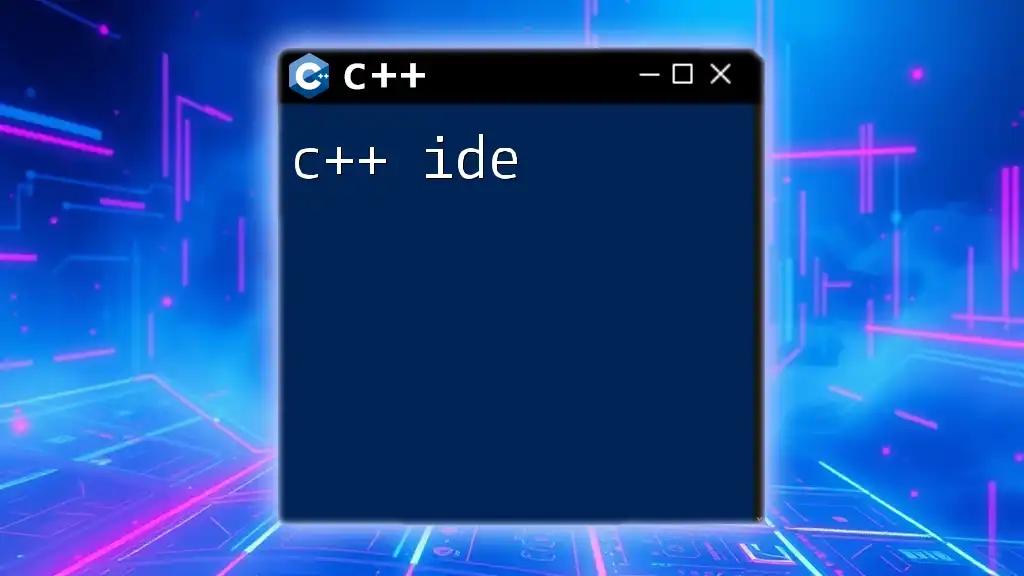
Understanding Artificial Intelligence
Artificial Intelligence refers to the simulation of human intelligence in machines programmed to think and learn like humans. AI encompasses various technologies like machine learning, natural language processing, and robotics. Its applications are vast, including:
- Autonomous vehicles
- Voice recognition systems
- Predictive analytics
With the rapid advancements in AI technology, the need for robust programming languages like C++ is more crucial than ever, enabling developers to build high-performance AI applications.
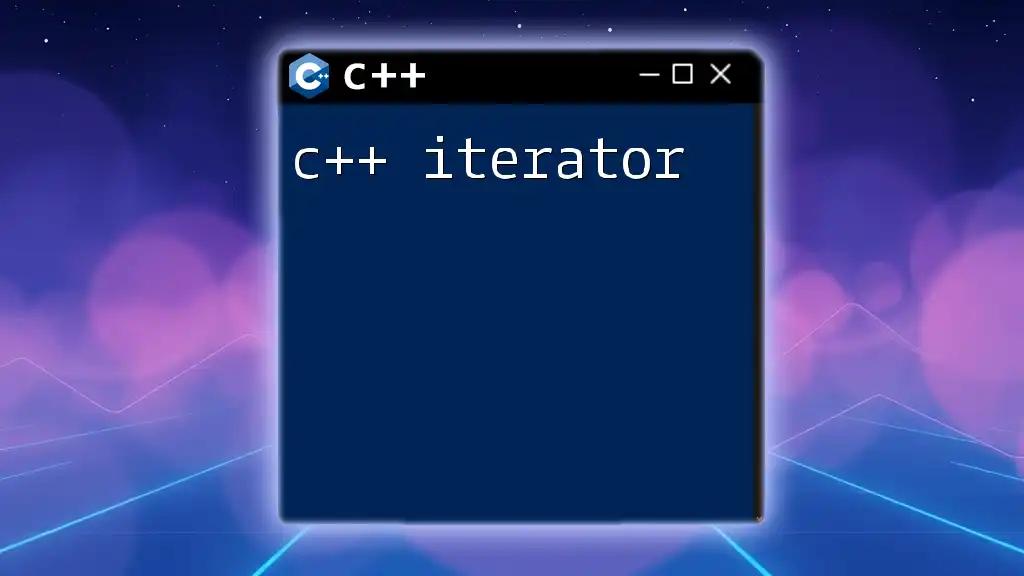
The Relevance of C++ in AI Development
Performance and Efficiency
C++ is known for its speed and efficiency, which is paramount for AI applications that often involve complex computations and large datasets. Unlike many languages that rely on garbage collection, C++ allows developers direct control over memory, which means they can optimize for performance.
Libraries and Frameworks
C++ collaborates seamlessly with numerous libraries designed explicitly for AI:
- TensorFlow: Originally developed for Python but has a C++ API that harnesses performance benefits.
- Caffe: A deep learning framework that is written in C++ and optimized for speed.
By utilizing these libraries, developers can expedite the creation of AI models while still benefitting from C++'s performance advantages.
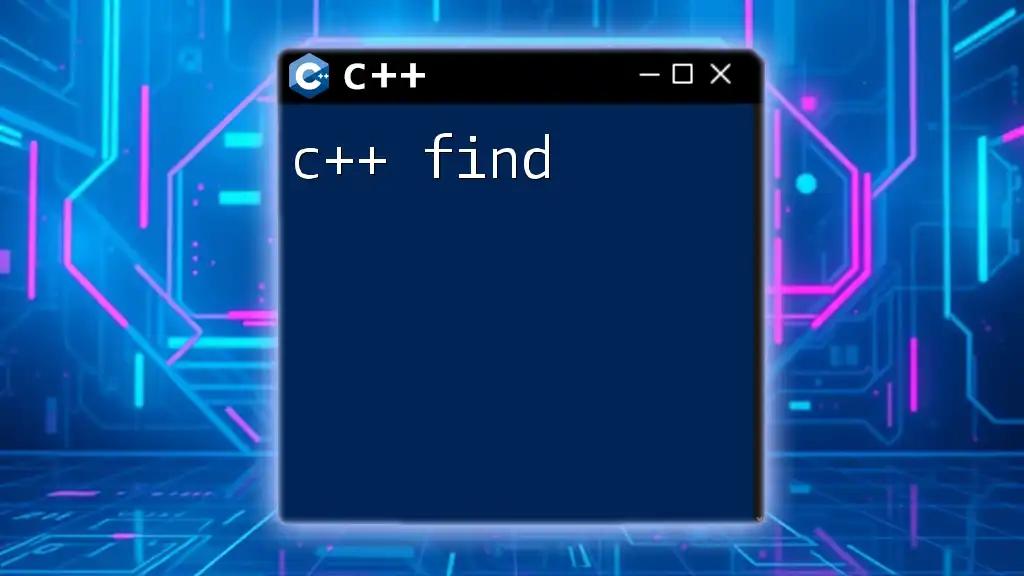
Getting Started with C++ for AI
Setting Up the Environment
To start coding in C++ for AI, it is essential to set up an effective environment for development:
- Choosing the Right IDE: You have several options such as Visual Studio, Code::Blocks, or Eclipse. Each offers unique features that can enhance productivity.
- Installing Necessary Libraries: Ensure you install critical libraries like TensorFlow or OpenCV on your system, allowing you to access the functionalities needed for AI projects.
Basic C++ Syntax and Concepts
Before diving into AI, familiarize yourself with the basics of C++:
- Data Types, Variables, and Operators: Understand the fundamental data types (int, float, string) and operators (arithmetic, logical).
- Control Structures: Learn how to use `if`, `for`, and `while` statements to manage program flow.
- Functions and Classes: Functions help modularize your code, while classes allow you to implement object-oriented principles.
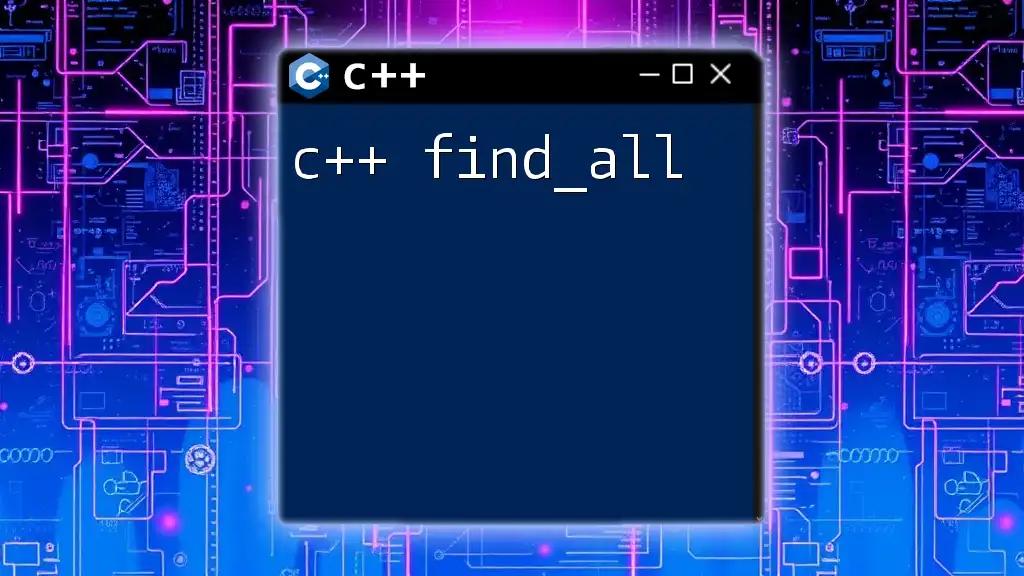
Essential C++ Concepts for AI
Object-Oriented Programming
A core concept of C++ is Object-Oriented Programming (OOP). Understanding OOP enables you to design your AI applications in a more structured manner:
- Classes and Objects: A class is a blueprint for creating objects, which encapsulates data and functions.
- Inheritance and Polymorphism: These features allow for code reusability and flexibility, essential in developing scalable AI applications.
Templates and Generic Programming
Templates allow developers to work with generic types. This is particularly useful in AI libraries for writing functions that can operate on different data types without sacrificing performance. For instance:
template<typename T>
T add(T a, T b) {
return a + b;
}
Memory Management in C++
C++ provides robust memory management capabilities, which is crucial for high-performance applications:
- Pointers and References: Keep track of memory addresses and avoid unnecessary copies.
- Dynamic Memory Allocation: Use `new` and `delete` to allocate and free memory as required.
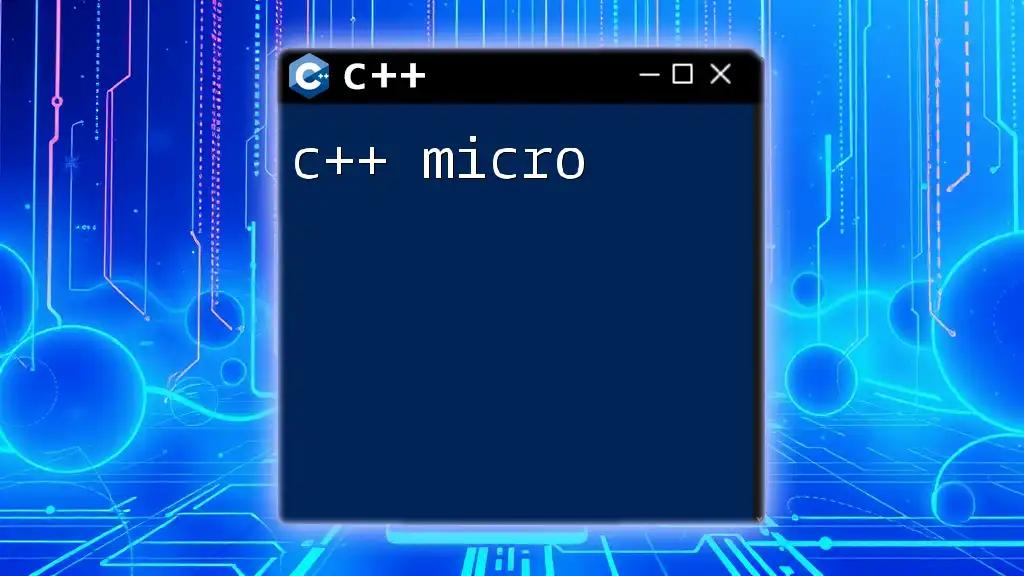
Built-in Data Structures and Algorithms
Standard Template Library (STL)
The STL is a powerful feature of C++ that includes collections of algorithms and data structures:
- Overview of STL Containers: Using containers like `vector`, `list`, and `map` allows efficient data manipulation.
- Algorithms for AI: The STL provides essential algorithms for searching, sorting, and more, which are crucial in AI applications.
Custom Data Structures
Sometimes, you may need to create custom data structures such as trees or graphs. These are vital for many AI algorithms, including decision trees or neural networks.
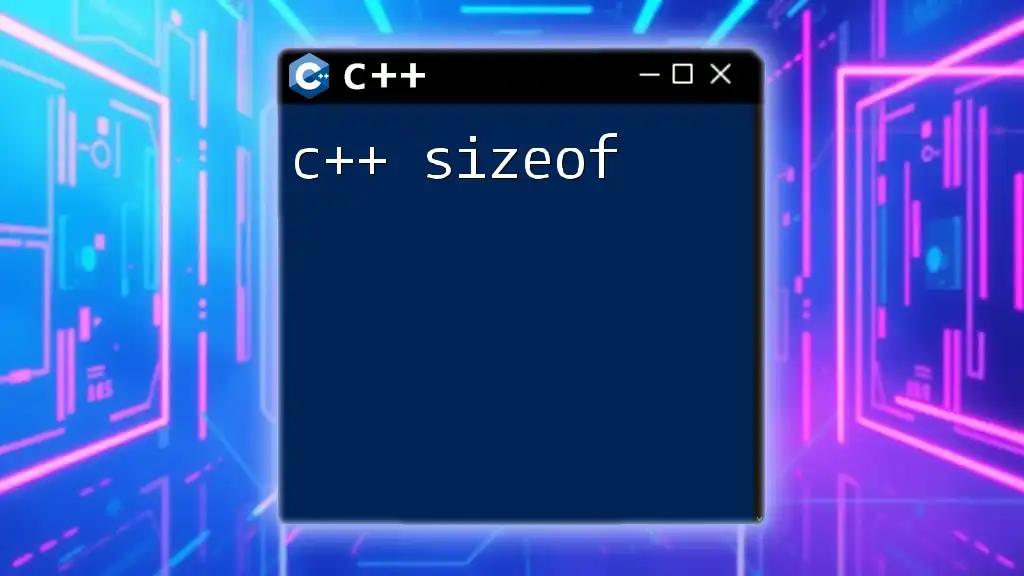
Developing AI Models in C++
Introduction to Machine Learning
Machine Learning is a subset of AI that focuses on building systems that can learn from data. It can be categorized as:
- Supervised Learning: Learning from labeled data.
- Unsupervised Learning: Discovering patterns in unlabeled data.
Implementing a Simple AI Model
To understand AI development in C++, let’s implement a basic linear regression model.
#include <iostream>
#include <vector>
class LinearRegression {
public:
LinearRegression() : slope(0), intercept(0) {}
void fit(const std::vector<double>& x, const std::vector<double>& y) {
// Fit model to data
// Simple implementation of linear regression
double x_mean = computeMean(x);
double y_mean = computeMean(y);
// Calculate slope and intercept
slope = ...; // Calculate the slope
intercept = ...; // Calculate the intercept
}
double predict(double x_value) const {
return slope * x_value + intercept;
}
private:
double slope, intercept;
double computeMean(const std::vector<double>& data) {
double sum = 0;
for (auto val : data) sum += val;
return sum / data.size();
}
};
In this snippet, we create a simple `LinearRegression` class to fit a linear model to our data. The methods `fit` and `predict` provide the core functionality to train and use the model.
Deep Learning with C++
Deep Learning consists of complex neural networks, which can also be implemented in C++. To illustrate, here is a simple example of a neural network setup using a C++ framework.
#include <iostream>
#include "tensorflow/core/public/session.h"
#include "tensorflow/core/protobuf/meta_graph.pb.h"
class SimpleNeuralNet {
public:
void createModel() {
// Code for creating a neural network model using TensorFlow C++ API
}
void trainModel() {
// Code for training the neural network
}
void predict() {
// Code for making predictions
}
};
This code snippet serves as a placeholder to indicate where your neural network's architecture, training routines, and prediction methods would be implemented.
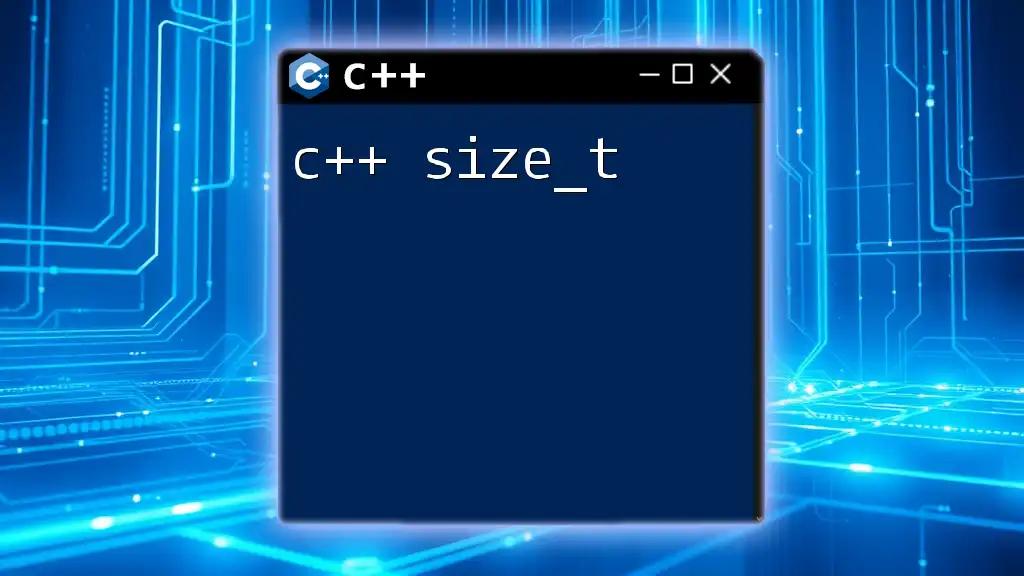
C++ Libraries for AI
TensorFlow C++ API
TensorFlow's C++ API provides robust functionalities to build complex models. It's particularly useful when performance is a critical factor. With its extensive set of features, developers can implement efficient algorithms for large datasets.
OpenCV for Computer Vision
OpenCV is an open-source computer vision library that allows you to perform various image processing tasks. It is widely used in AI for applications such as face recognition and object detection. Here’s a simple object detection snippet:
#include <opencv2/opencv.hpp>
void detectObjects(const std::string& image_path) {
cv::Mat img = cv::imread(image_path);
// Object detection logic here
}
Other Notable Libraries
Several other libraries complement C++ for AI, including:
- Dlib: For machine learning algorithms.
- Shark: For fast optimization and machine learning.
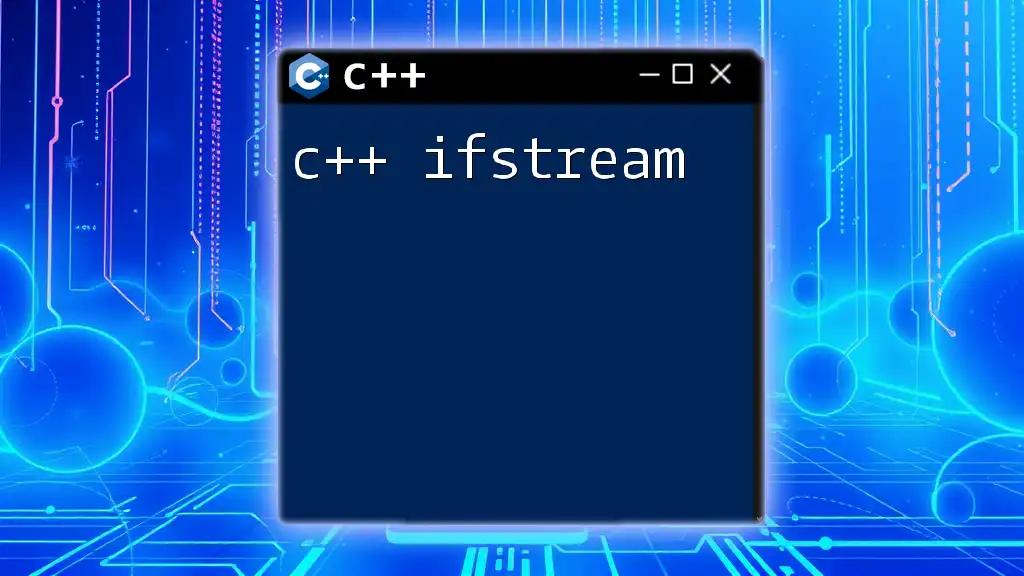
Challenges and Best Practices
Common Challenges in C++ AI Development
Developing AI applications in C++ is not without challenges:
- Debugging and Profiling: C++ can be challenging for debugging due to complexity. Use tools like Valgrind and GDB.
- Managing Dependencies: Handling libraries can sometimes lead to conflicts; understanding the build process is essential.
Best Practices for Writing C++ Code
To ensure robust AI applications, adhere to the following best practices:
- Code Clarity and Maintainability: Write clean and well-commented code to facilitate teamwork and future maintenance.
- Testing AI Models: Implement unit tests and validation checks to ensure accuracy and effectiveness before deployment.
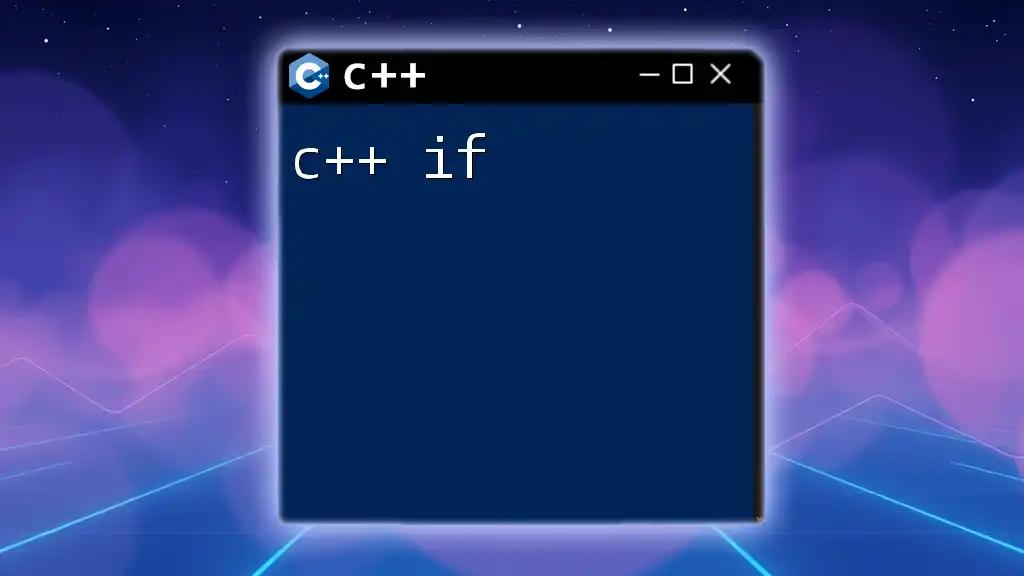
Future of C++ in AI
Emerging Trends
As AI technology progresses, C++ will continue to play a vital role, especially in areas requiring high performance, such as real-time data processing and deployment of AI models in resource-constrained environments.
Career Opportunities
With the growing demand for AI solutions, specializing in C++ can provide lucrative career opportunities in various sectors, including finance, healthcare, and tech.
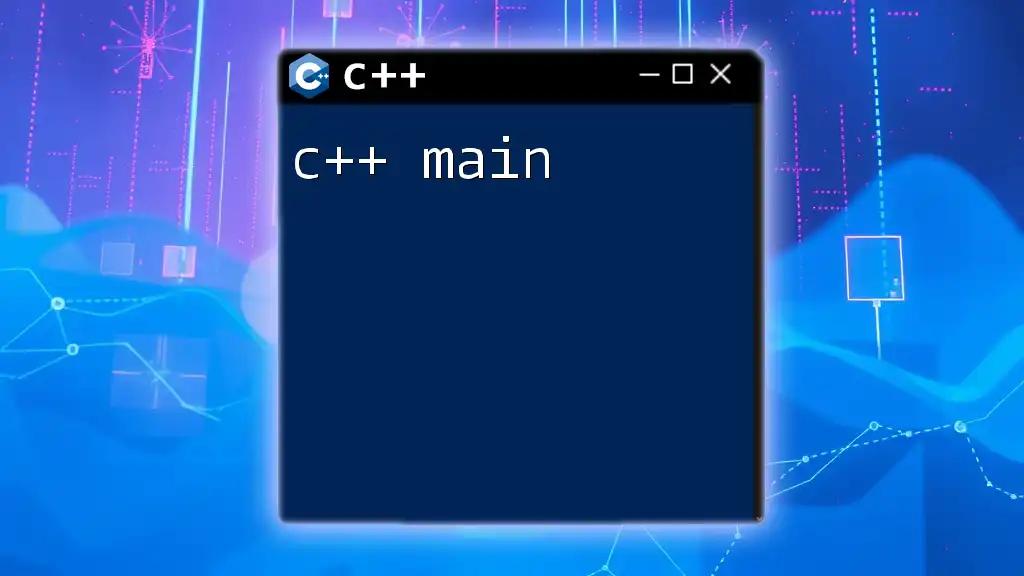
Conclusion
As we've explored, C++ serves as a powerful ally in developing AI solutions, providing high performance, extensive libraries, and the ability to manage system resources effectively. By mastering C++ and its applications in AI, you can be well-prepared to tackle the challenges and opportunities presented by this exciting field.
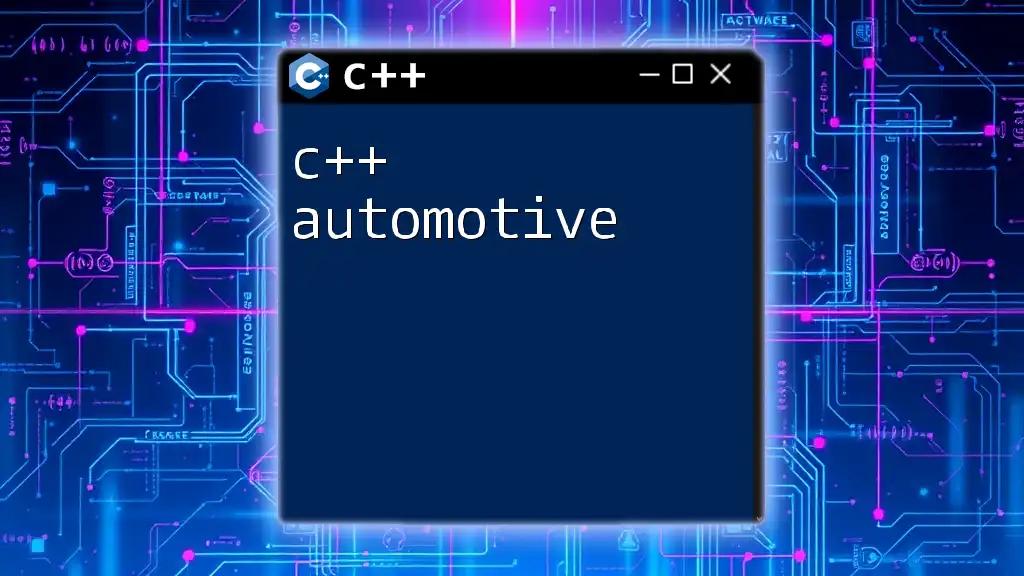
Additional Resources
To further enhance your learning journey in C++ AI, consider exploring the following resources:
- Recommended Books: Classic texts like "Effective C++" and "C++ Primer."
- Online Courses and Tutorials: Platforms like Coursera and edX offer dedicated courses on C++ and AI.
- Communities and Forums: Engaging within communities such as Stack Overflow or GitHub can provide valuable insights and peer support.