C++ AIO (Asynchronous Input/Output) allows for non-blocking I/O operations, making it possible to perform tasks without waiting for operations like file or network access to complete.
Here's a simple example of using C++ AIO for reading a file asynchronously:
#include <iostream>
#include <fstream>
#include <future>
void readFileAsync(const std::string& filename) {
std::ifstream file(filename);
std::string content((std::istreambuf_iterator<char>(file)), std::istreambuf_iterator<char>());
std::cout << content << std::endl;
}
int main() {
std::future<void> result = std::async(std::launch::async, readFileAsync, "example.txt");
result.get(); // Wait for the async operation to complete
return 0;
}
Understanding C++ Redistributables
What is a C++ Redistributable?
A C++ redistributable is a collection of files required to run applications developed with Visual C++. These files include dynamic link libraries (DLLs) and runtime components essential for the execution of software. When an application is developed using Visual Studio, it may depend on a specific version of the C++ libraries. If the same libraries are not present on the user's system, the application may fail to run, prompting the need for these redistributables.
Common Visual C++ Redistributables
There are several versions of Visual C++ Redistributables, including:
- Visual C++ 2005
- Visual C++ 2008
- Visual C++ 2010
- Visual C++ 2013
- Visual C++ 2015-2019
Each version corresponds to a particular set of libraries that align with the version of Visual Studio used for development. It's important to install the correct version based on the application requirements.
Why Use AIO Packages for Redistributables?
Using AIO packages for C++ redistributables simplifies the installation process, making them a convenient choice for developers and users alike. Consider the following benefits:
- Simplified Installation Process: One AIO installer can manage multiple redistributables, saving time and ensuring all required versions are set up correctly.
- Reduced Potential Conflicts: Some applications may require different versions of the C++ libraries. An AIO package reduces the chances of conflicting installations that can cause runtime errors.
Use Case: Many applications depend on various versions of C++ runtimes. For instance, if a user attempts to run software that requires both Visual C++ 2008 and 2015, installing these as separate packages can lead to conflicts. An all-in-one installer helps manage this complexity.
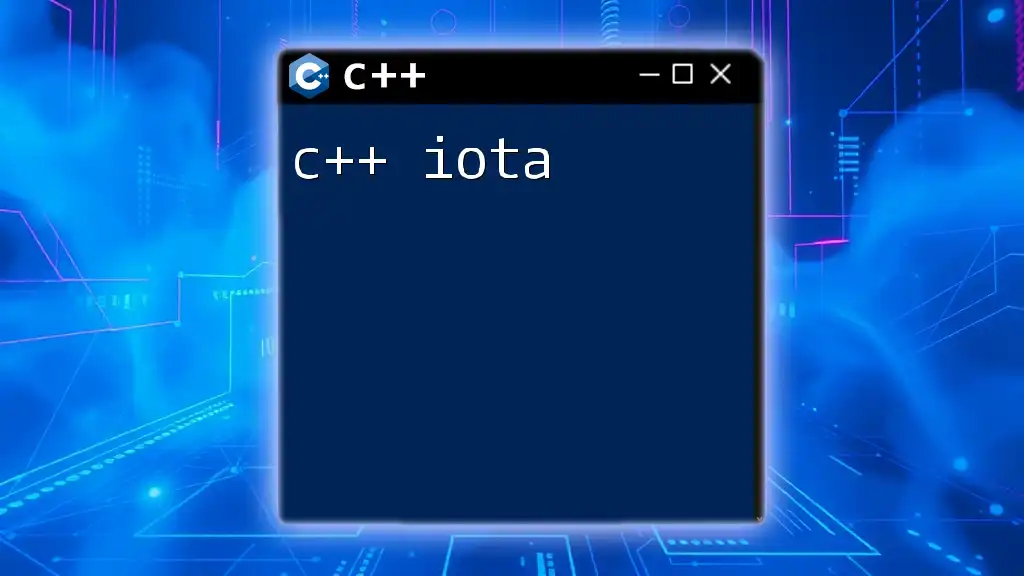
Components of Visual C++ AIO
The Role of Visual C++ Runtime
The Visual C++ runtime is critical for the execution of C++ applications. It includes libraries that handle common tasks such as memory allocation, file I/O, and other system calls that an application may require. Without the correct runtime installed, applications won't launch and will often present error messages stating missing dependencies.
Overview of Visual C++ All-In-One Packages
Visual C++ AIO packages bundle several versions of these critical files into a single installer. This means that users don't need to worry about the specific requirements of every application they install; they can trust that the AIO package will cover the necessary dependencies. These packages typically incorporate:
- Multiple versions of Visual C++ redistributables.
- Direct access to the runtime libraries that applications need.
- Configuration settings that minimize the risk of future conflicts.
Third-party developers provide many AIO packages, and it is essential to use trusted sources to avoid the risks associated with unverified software.
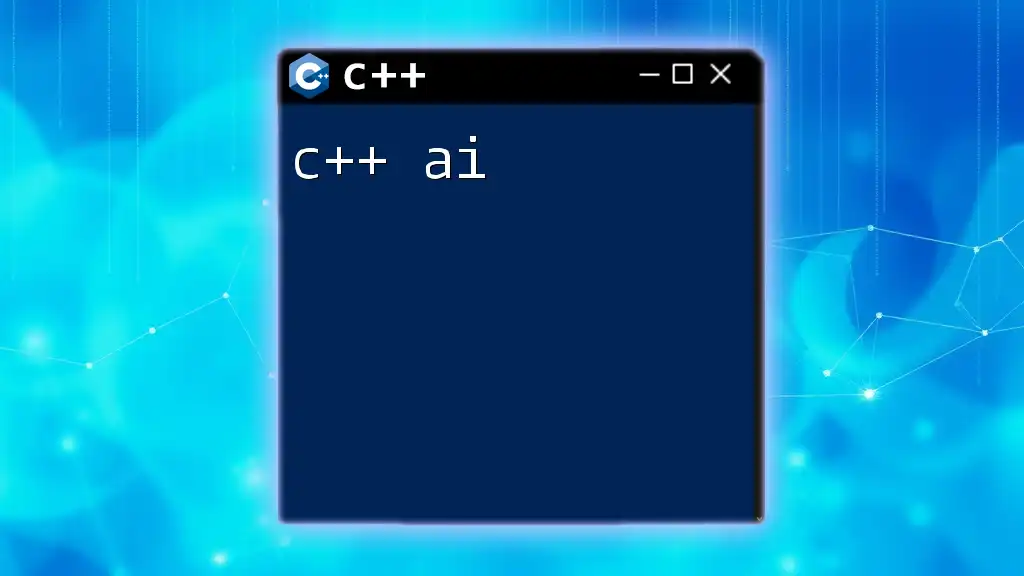
How to Install C++ AIO Packages
Step-by-Step Installation Guide
To successfully install a C++ AIO package, follow these steps:
-
Prerequisites for Installation:
- Ensure your system meets the necessary specifications for the installation.
- Backup any existing installations if you think it may lead to conflicts.
-
Using the AIO Installer:
- Download the AIO package from a reliable source.
- Run the installer file, and you may be prompted to accept a license agreement.
- The installer will detect any existing versions and install the required libraries and runtimes.
Example: Here’s a typical command used to install an AIO package from the command line:
C++_AIO_Package.exe /S /D=C:\Path\To\Install
- Finalize the Installation:
- Once installed, it’s good practice to restart your computer to ensure all changes take effect.
Troubleshooting Common Installation Issues
While installing AIO packages can be straightforward, potential issues can arise:
- Installation Fails: Ensure that you're running the installer with administrative rights.
- Dependencies Not Found: Check for logs to identify which components failed. You might need to install them separately.
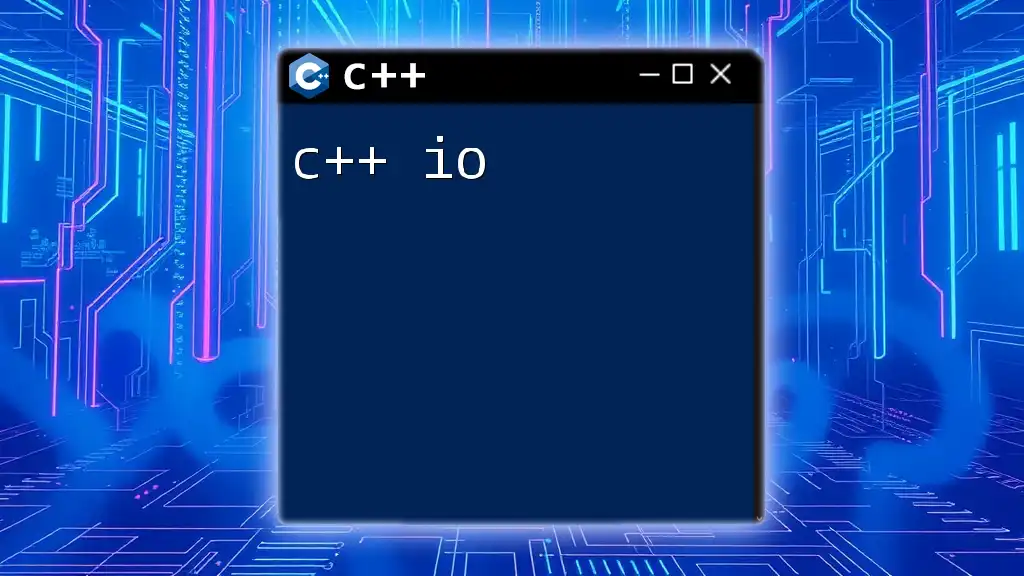
Best Practices for Managing Visual C++ Runtimes
Keeping Your Runtimes Up to Date
To maintain the security and efficiency of your applications, keeping C++ runtimes updated is essential.
Why Regular Updates Are Necessary:
Outdated libraries may expose your systems to vulnerabilities. Therefore, ensuring you have the latest security patches and enhancements is critical.
To check for updates, visit the official Microsoft website or use your operating system's built-in tools for detecting software updates.
Minimizing Conflicts Between Different Versions
Managing multiple Visual C++ redistributables can be tricky. Here are some tips to mitigate conflicts:
- Use AIO Packages: These packages help ensure that all required runtimes exist without conflicts.
- Monitor Installation Logs: When installing applications, watch for messages indicating which redistributable versions are being installed.
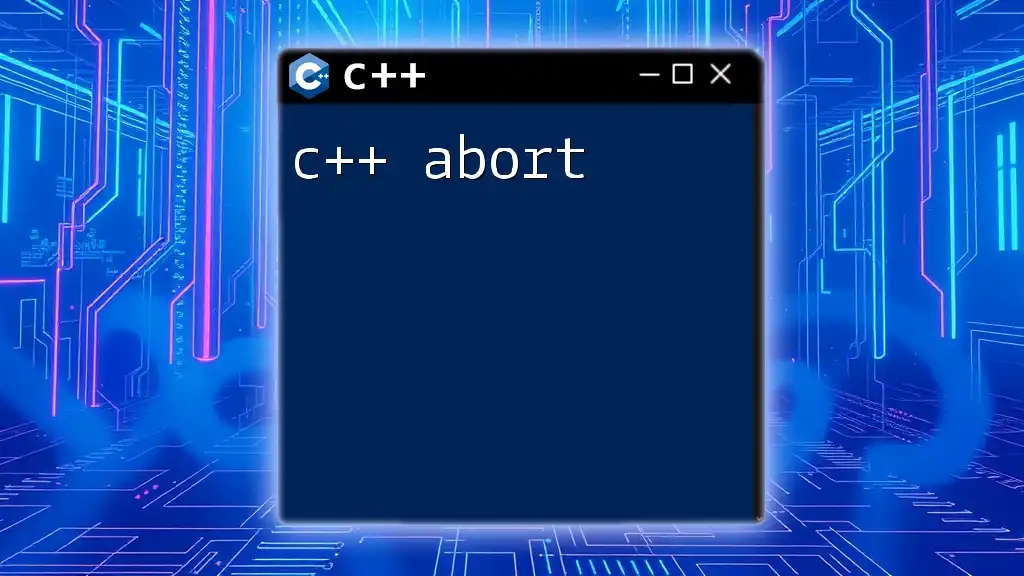
Advantages of Using C++ AIO Packages
Simplifying the Setup Process
By utilizing C++ AIO packages, developers and users benefit from a streamlined setup process. One installation process takes the place of potentially multiple steps across various components, saving both time and hassle.
Enhancing User Experience
For end-users, reduced errors related to missing libraries result in a seamless experience. When all runtime environments are adequately supplied, applications launch as intended, leading to higher satisfaction.
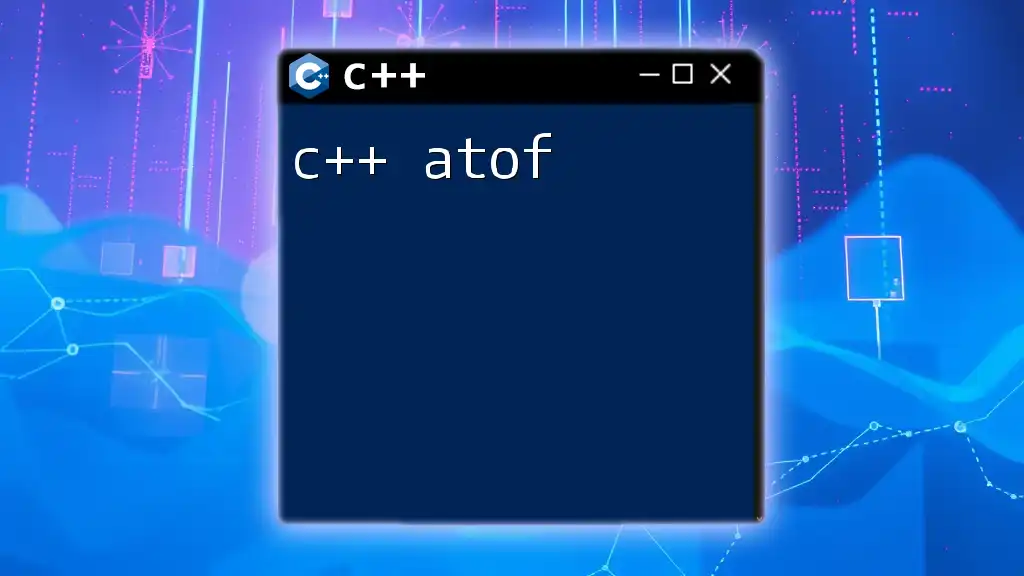
Conclusion
Understanding C++ AIO packages is vital for both developers and users, as they facilitate the installation and management of essential runtimes. By leveraging these packages, one can significantly simplify application deployment and execution while enhancing overall user experience.