In C++, the `cout` object is used to output data to the standard output stream, typically the console, and is part of the iostream library.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding cout
What is cout?
In C++, cout (short for "character output") is the standard output stream used to display information on the console. It is an integral part of the iostream library and allows developers to send data to the standard output device, usually the screen. Beyond simply printing text, cout can handle a variety of data types, making it versatile for different programming scenarios.
How cout Works
Cout communicates with the standard output through buffering, which temporarily holds data before it is displayed. This buffering mechanism improves performance by reducing the number of input/output operations, ensuring efficient output delivery. Understanding buffering is key to grasping how and when your output appears on the console.
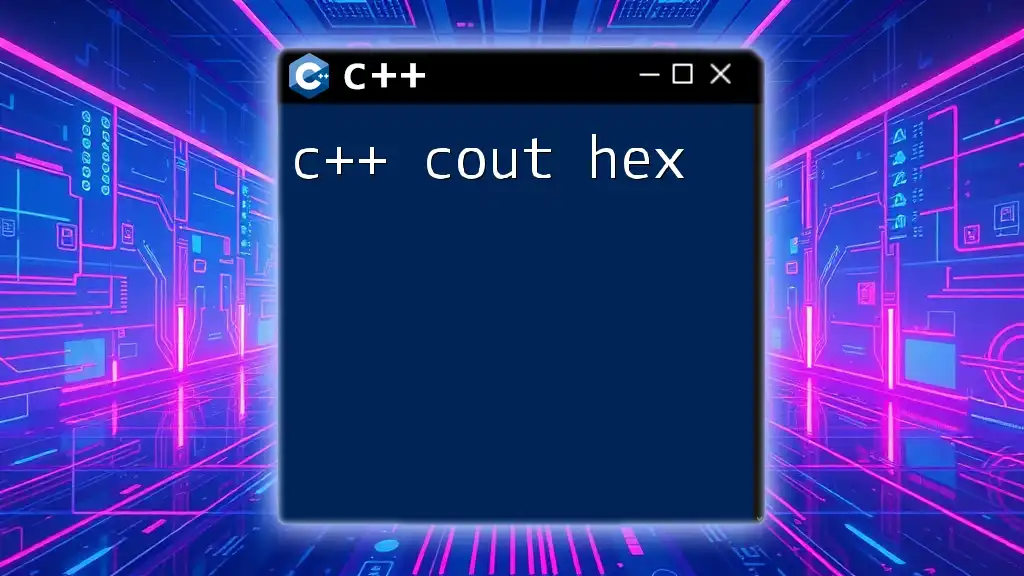
Setting Up Your C++ Environment
Required Tools
To get started with using c++ cout, you'll need a suitable C++ compiler or Integrated Development Environment (IDE). Popular choices include:
- GCC: A widely-used open-source compiler that works on various platforms.
- Microsoft Visual Studio: A robust IDE that comes with built-in support for C++.
- Code::Blocks: A free C++ IDE that is user-friendly for beginners.
Example Setup
To set up a simple C++ project:
- Install your chosen compiler or IDE.
- Create a new project and ensure your main file has a `.cpp` extension.
- Write your initial code to include the necessary libraries, such as `#include <iostream>`, to use cout.
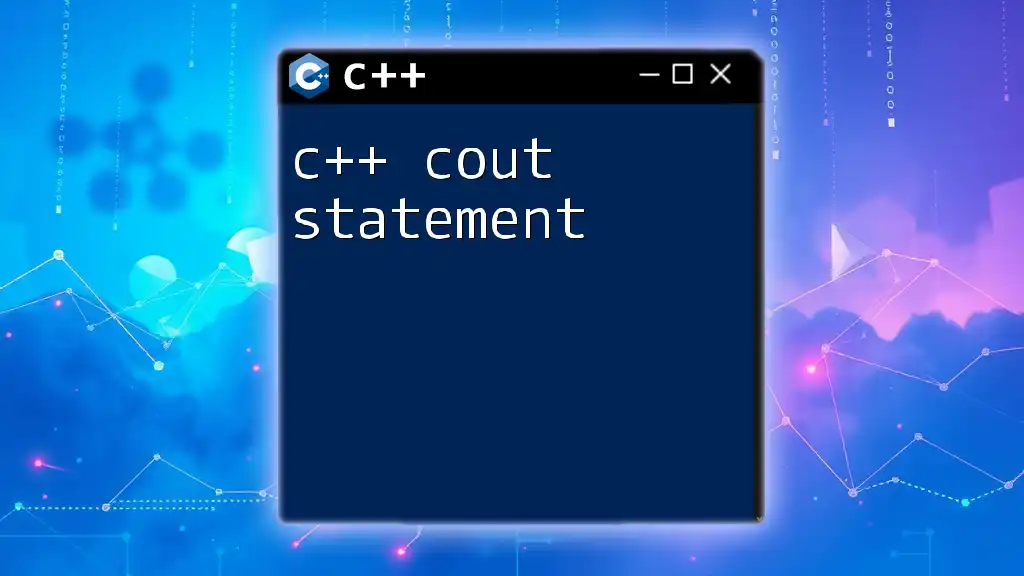
Basic Syntax of cout
Using cout for Simple Output
The basic syntax for using cout is straightforward:
std::cout << "Your message";
This line sends the string “Your message” to the standard output.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Sends output to standard output
return 0;
}
Here, `std::cout` is the standard output stream, and "Hello, World!" is printed to the console. The `<<` operator is known as the stream insertion operator, which is crucial for sending data to cout.
Sending Messages to cout
Strings and Characters
Cout can effortlessly output strings and individual characters, making it a versatile tool for displaying various types of data.
Code Snippet
cout << "Welcome to C++!" << endl;
cout << 'A' << endl;
In this example, the string "Welcome to C++!" and the character 'A' are both printed on the console with the help of cout and followed by an `endl`, which adds a new line.
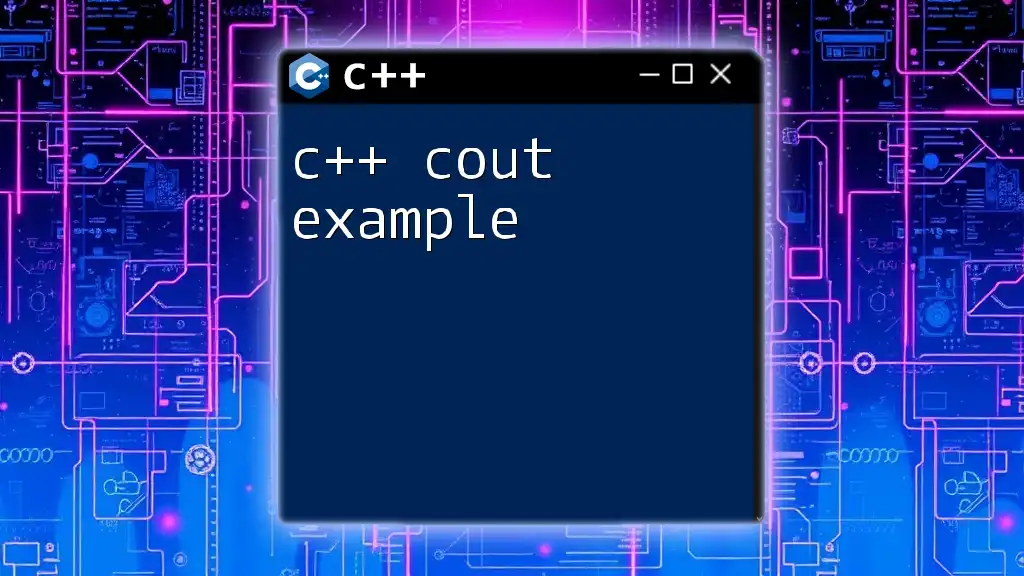
Formatting Output
Manipulating Output with endl
When working with cout, using endl to create new lines improves readability by separating output clearly. It's particularly useful when you want structured output.
Using Escape Sequences
Escape sequences enhance the formatting of output. Common escape sequences include:
- `\n`: New line
- `\t`: Horizontal tab
- `\\`: Backslash
Example Code Snippet
cout << "Line 1\nLine 2\n" << endl; // Using newline
cout << "Name:\tJohn Doe" << endl; // Using tab
In this example, the first line breaks into two lines. The second line inserts a tab space before "John Doe".
Controlling Decimal Places
To control the number of decimal places in floating-point output, include the iomanip library, particularly using `std::fixed` and `std::setprecision`.
Code Snippet
#include <iomanip>
cout << fixed << setprecision(2) << 123.456 << endl; // Outputs 123.46
This guarantees that the output displays two decimal places, rounding where necessary.
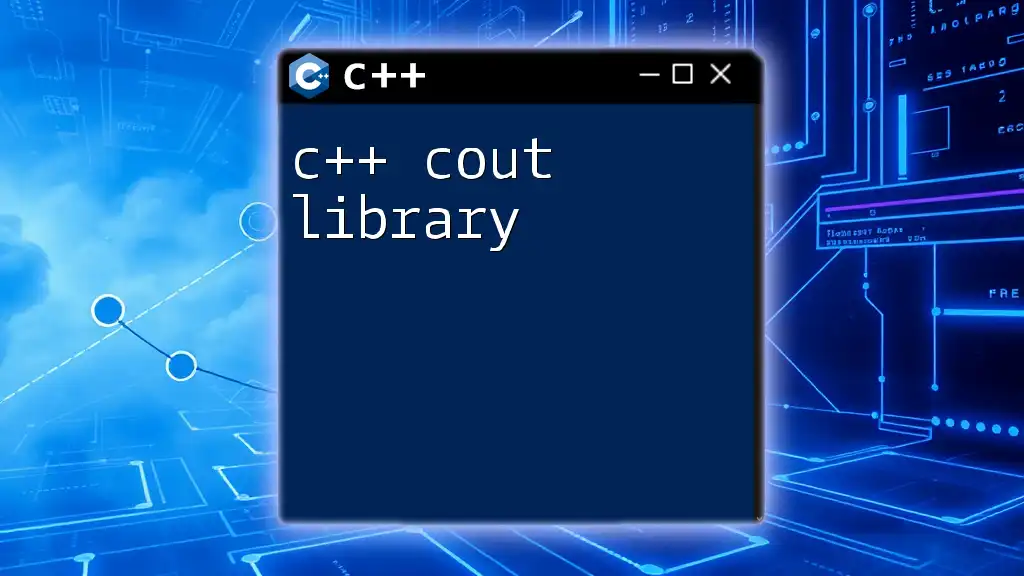
Combined Outputs
Multiple Outputs in One Line
Cout allows multiple outputs to be concatenated in one line, providing a seamless way to display combined messages.
Example Code Snippet
cout << "Value: " << 42 << ", Status: " << "Success" << endl;
This code snippet uses multiple `<<` operators to send various data types to the output stream simultaneously.
Outputting Variables
Using Different Data Types
Cout can handle diverse data types, including integers, doubles, and strings, showcasing its flexibility.
Code Snippet
int number = 5;
double pi = 3.14;
cout << "Integer: " << number << ", Floating point: " << pi << endl;
Here, both an integer and a floating-point number are output together, demonstrating cout's capability to manage different data types.
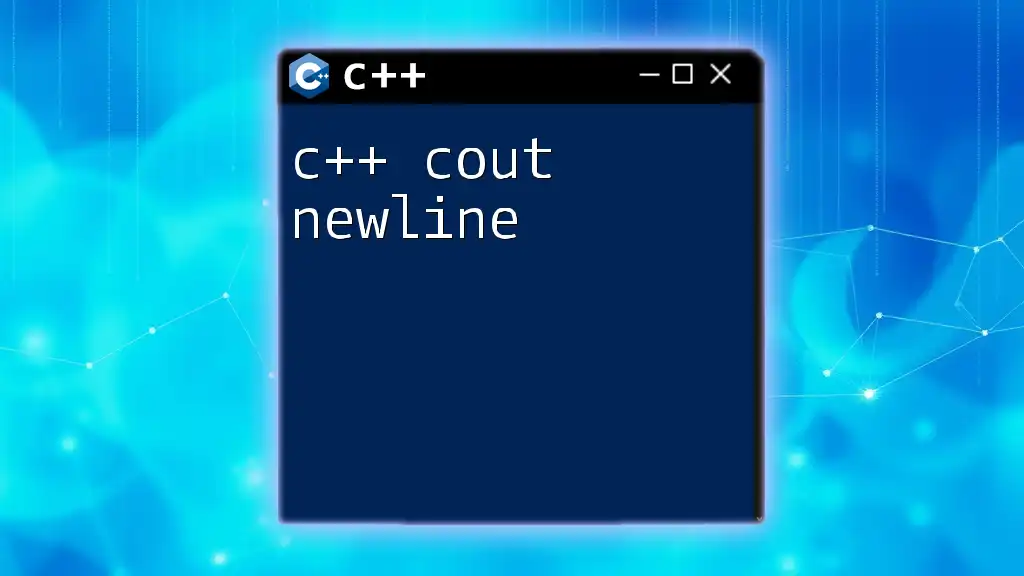
Common Pitfalls with cout
Errors and How to Avoid Them
Beginners often overlook essential include directives like `#include <iostream>`, leading to compilation errors. Always ensure that these directives are at the top of your code to enable cout.
Output Buffering Issues
Occasionally, output may not appear on the console as expected. This is often due to buffering. If output does not seem to display immediately, consider using:
std::cout.flush(); // Forces the output buffer to flush
This will ensure that any buffered output is displayed on the console without delay.
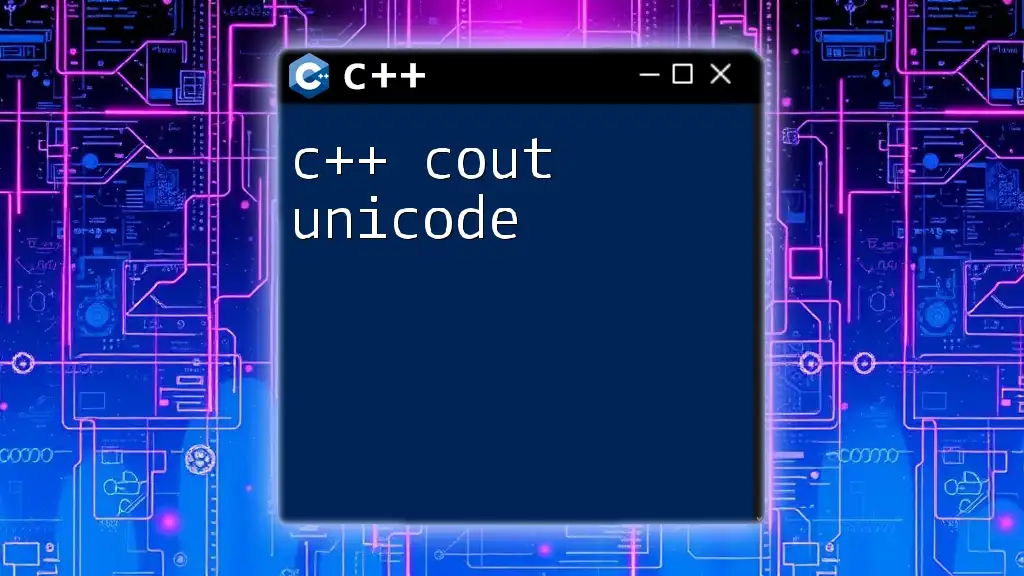
Conclusion
The power of c++ cout lies in its simplicity and versatility as a standard output stream. By mastering the use of cout, including formatting options and output control, you can significantly enhance your C++ programming skills. This guide has navigated through basic syntax, formatting outputs, and common pitfalls, providing a solid foundation upon which to build further knowledge.
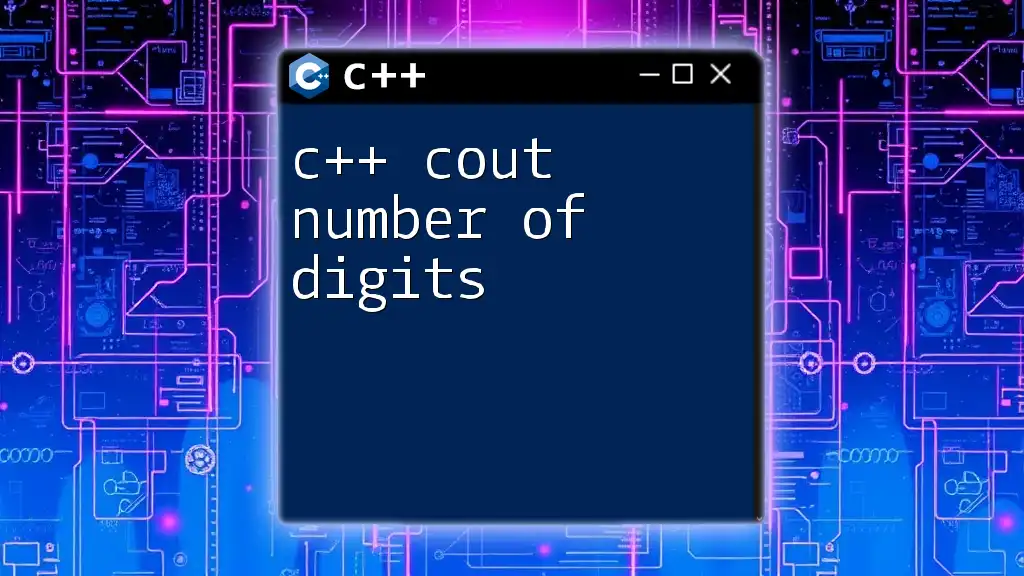
Call to Action
Experiment with the examples provided, and don’t hesitate to modify them to see how different outputs behave. Share your experiences or questions in the comments section for further discussion. For more in-depth learning, explore additional resources to expand your C++ capabilities!
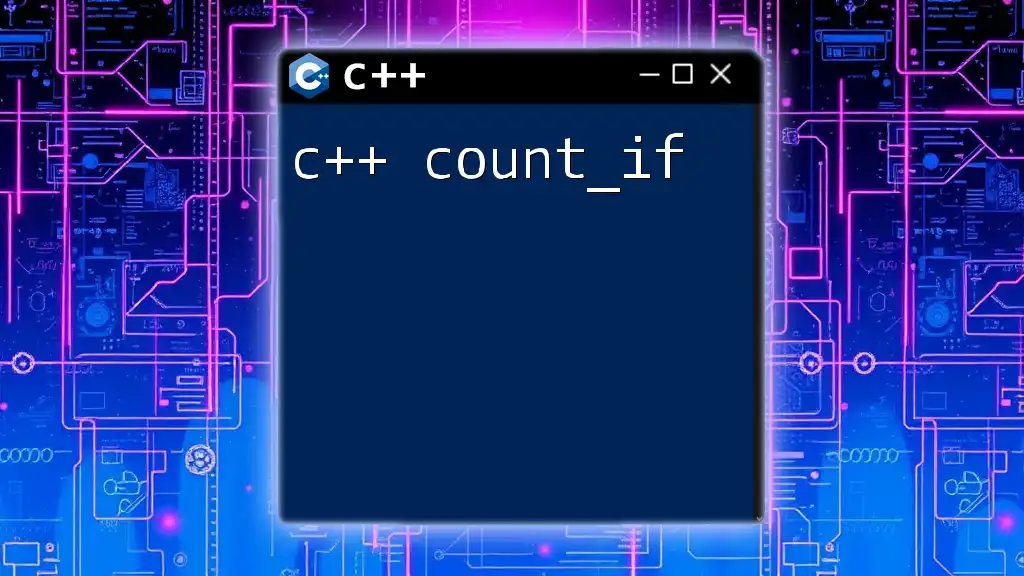
Further Reading
For continued development in C++, consider diving into advanced output techniques, writing custom output functions or exploring the STL (Standard Template Library). Engaging with C++ communities online can also provide valuable insights and support as you advance your skills.