In C++, you can append an integer to a string by converting the integer to a string using `std::to_string()` and then using the `+=` operator.
Here's a code snippet to demonstrate this:
#include <iostream>
#include <string>
int main() {
std::string str = "The number is: ";
int num = 42;
str += std::to_string(num);
std::cout << str << std::endl; // Output: The number is: 42
return 0;
}
Understanding Strings in C++
What are Strings?
Strings in C++ are a sequence of characters used to represent text. They are crucial for manipulating text-based data in applications. C++ has two main types of strings:
- C-style strings: Arrays of characters ending with a null character (`\0`). They can be more complex to manage due to manual memory handling.
- Standard Strings (`std::string`): A part of the C++ Standard Library, offering a robust framework for string manipulation with built-in memory management and countless functions.
Why Append Integers to Strings?
Appending integers to strings is a common requirement in various applications. Practical scenarios include displaying logs, generating user-based messages, and naming files dynamically. For instance, when displaying a countdown timer, appending the current time left can enhance user experience.
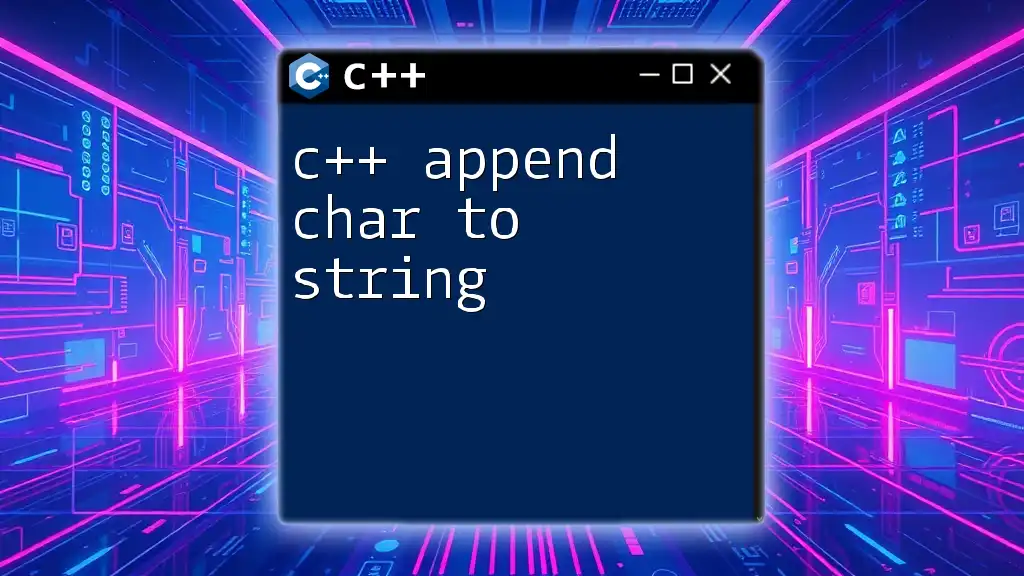
Preparing to Append Integers to Strings
Converting Integers to Strings
Before you can append an integer to a string, you need to convert the integer to a string format. C++ provides several methods for this conversion.
Using `std::to_string()`
The simplest way to convert an integer to a string is by using the `std::to_string()` function. This built-in function effectively transforms numerical values into their string representations.
Example code snippet:
int number = 42;
std::string str = std::to_string(number);
This converts the integer `42` into the string `"42"`. The output can then be manipulated or combined with other strings easily.
Using `std::stringstream`
If you're looking for more control over the conversion process or need to append various data types together, `std::stringstream` is a great alternative. This class allows you to create a stream where you can insert different types of data.
Example code snippet:
#include <sstream>
int number = 42;
std::stringstream ss;
ss << number;
std::string str = ss.str();
This code snippet uses `std::stringstream` to convert the integer into a string. The `str()` method is called to retrieve the string representation from the stringstream.
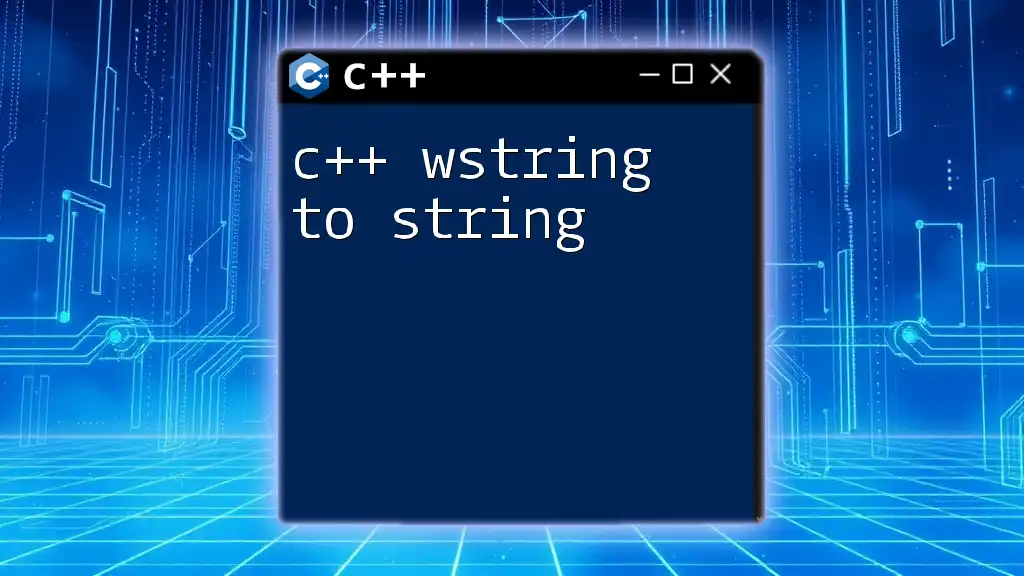
Appending Integer Strings
Using the `+` Operator
Once you have your integer as a string, appending it to another string can be done seamlessly using the `+` operator.
Example code snippet:
std::string base_str = "The answer is ";
int number = 42;
std::string combined = base_str + std::to_string(number);
This results in `combined` containing `"The answer is 42"`. The beauty of this approach is its simplicity and clarity, making your code easy to read.
Using `append()` Method
Alternatively, the `append()` method of `std::string` can also be employed to concatenate strings. This method is beneficial when building strings incrementally.
Example code snippet:
std::string base_str = "The answer is ";
int number = 42;
base_str.append(std::to_string(number));
Here, `base_str` is modified directly to include the integer string, resulting in `"The answer is 42"` at the end. Using `append()` can sometimes yield a slight performance improvement in scenarios where strings grow significantly during processing.
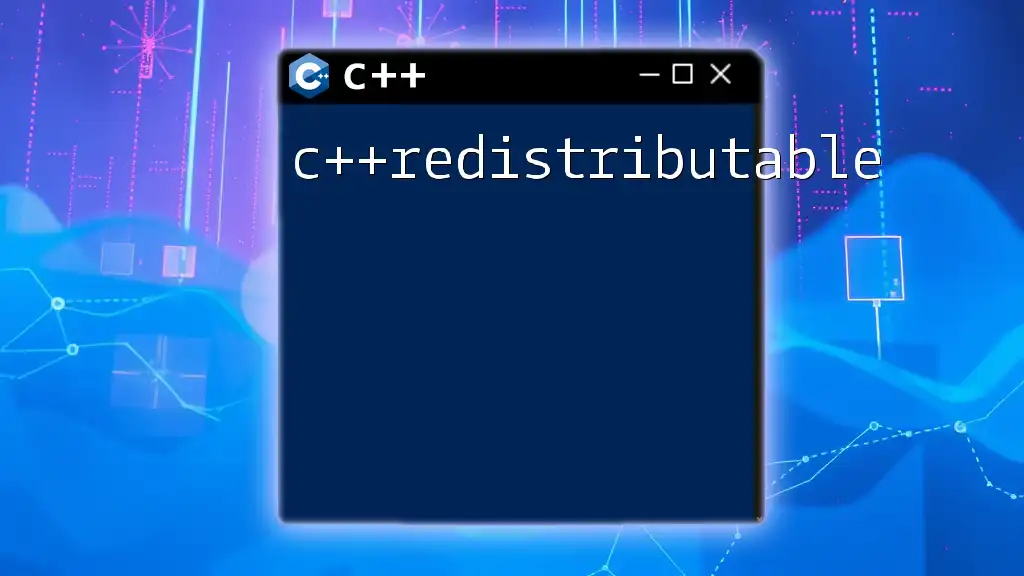
Advanced Techniques
Handling Multiple Integers
In some contexts, you might need to append several integers to a string. Using a loop can streamline this process.
Example code snippet:
std::string result = "Numbers: ";
for (int i = 0; i < 5; ++i) {
result += std::to_string(i) + " ";
}
This loop appends the numbers from `0` to `4` to the `result` string, producing `"Numbers: 0 1 2 3 4 "` as the final output. By adding a space after each number, we maintain readability.
Using `std::format` in C++20
If you have access to C++20 features, `std::format` provides a sleek way to format your strings. This standard enables cleaner and more understandable string interpolation.
Example code snippet:
#include <format>
int number = 42;
std::string str = std::format("The answer is {}", number);
The above code will produce the string `"The answer is 42"`. This method allows you to clearly visualize how strings and variables are combined, making your code less prone to errors.
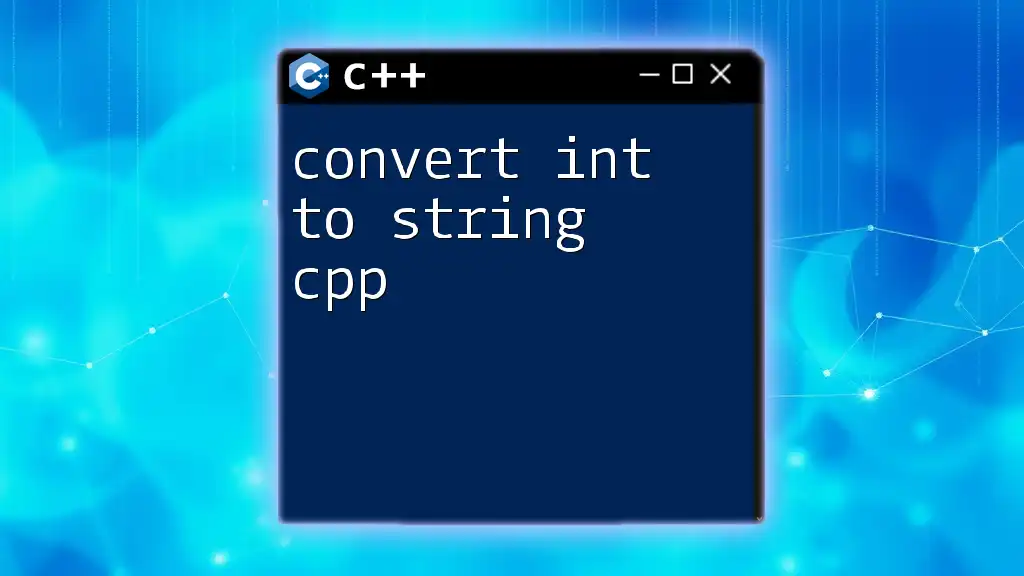
Performance Considerations
Comparing Methods
When it comes to performance, different methods suit varying use cases. Generally, `std::to_string()` is the go-to for its simplicity. However, in applications requiring frequent data manipulation, `std::stringstream` can sometimes outperform `std::to_string()` due to its buffered nature.
When constructing strings in scenarios involving many string concatenations, it's best to analyze each method's overhead and choose accordingly.
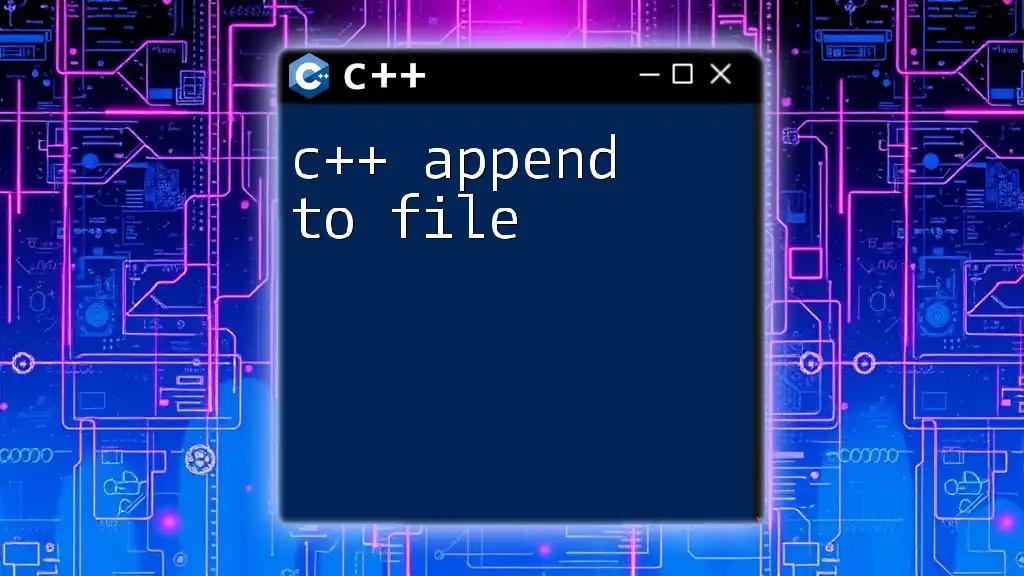
Common Pitfalls
Type Mismatches
A common issue that developers face when appending integers to strings is type mismatch. Always ensure you're converting the correct integer type. For example, using `long` or `unsigned int` without proper conversion may lead to unexpected behavior.
Memory Management
Remember that while `std::string` handles memory automatically, misuse of C-style strings can lead to memory leaks or buffer overflows. Always prefer standard strings unless you specifically require C-style strings for compatibility.
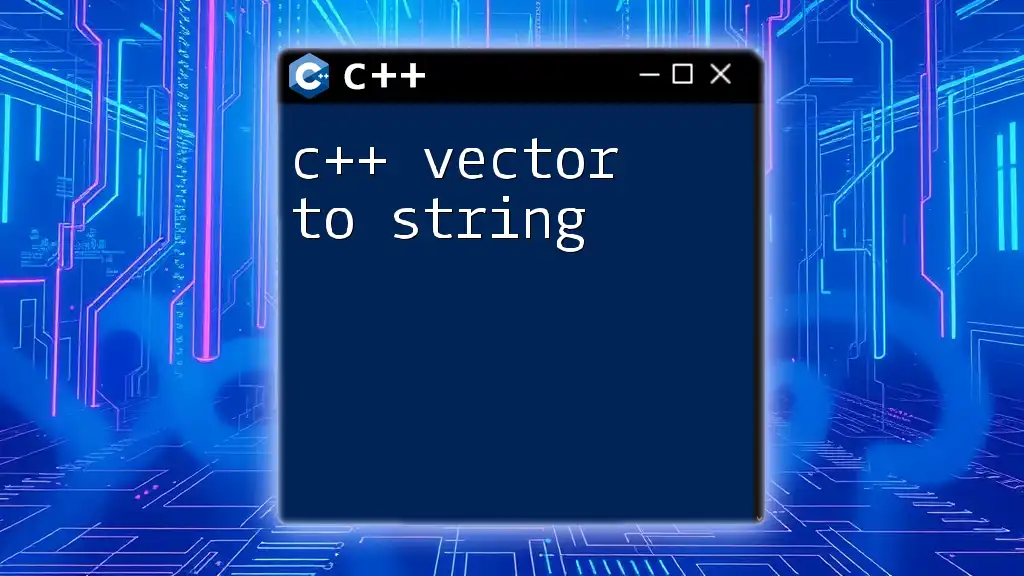
Conclusion
In this guide, we've explored how to effectively c++ append int to string using various methods like `std::to_string()`, `std::stringstream`, and `std::format`. Each method has its advantages, allowing you to choose based on your specific needs. Practice these techniques to deepen your understanding of string manipulation in C++, transforming your programming skills and enhancing your applications.