C++ header guards prevent multiple inclusions of the same header file, avoiding redefinition errors during compilation.
#ifndef HEADER_NAME_H
#define HEADER_NAME_H
// Declarations and definitions
#endif // HEADER_NAME_H
What are Header Guards?
Definition of Header Guards
C++ header guards are a programming construct that prevents multiple inclusion of the same header file within a single compilation unit. This is crucial for avoiding the common problem of multiple definitions, which can lead to compilation errors and unexpected behavior in a program.
How Header Guards Work
When you include a header file, C++ reads its contents and processes any definitions within it. If the same header file is included again within the same translation unit, the compiler will encounter redefinitions of the same entities. Header guards solve this problem by using the preprocessor to check whether a particular file has already been included. If it has, the content will not be processed again.
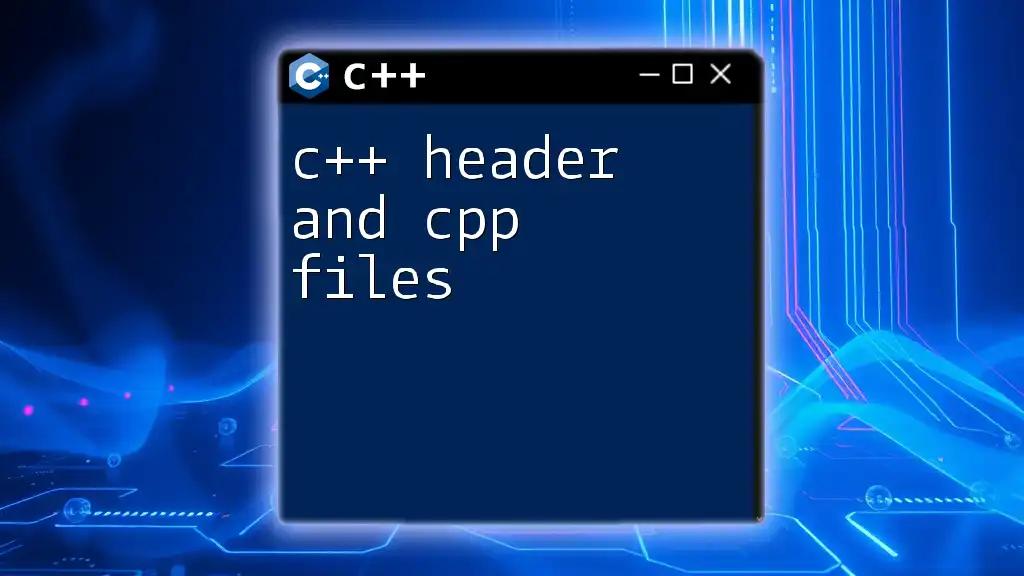
Syntax of Header Guards
Basic Structure
The basic structure of a header guard is a combination of three preprocessor directives: `#ifndef`, `#define`, and `#endif`. Here’s a simple example to illustrate its use:
#ifndef HEADER_FILE_NAME
#define HEADER_FILE_NAME
// Declarations and definitions
#endif // HEADER_FILE_NAME
Breakdown of Each Component
-
`#ifndef HEADER_FILE_NAME`: This checks if the identifier `HEADER_FILE_NAME` has not been defined yet. If it hasn’t, the code between this directive and the corresponding `#endif` will be included in the compilation.
-
`#define HEADER_FILE_NAME`: This directive defines the identifier so that any future inclusion of this same file will skip over its content due to the preceding `#ifndef`.
-
`#endif`: This marks the end of the conditional inclusion started by `#ifndef`.
Utilizing unique identifier names is crucial to prevent conflicts with other header files.

Best Practices for Using Header Guards
Choosing Unique Identifiers
When creating unique identifier names for header guards, it’s best to follow a systematic approach. A common practice is to use the underscore-separated file name in all uppercase letters. For example, if your header file is named `MyClass.h`, a good identifier might be `MY_CLASS_H`.
This practice helps avoid naming clashes, especially in large projects or when using libraries from different sources.
Nesting Header Guards
In more complex C++ projects, where header files might themselves include other headers, careful use of header guards is paramount. Ensure that guards are consistently named across all files to avoid confusion. This helps in maintaining clear dependencies and understanding of how files interact.
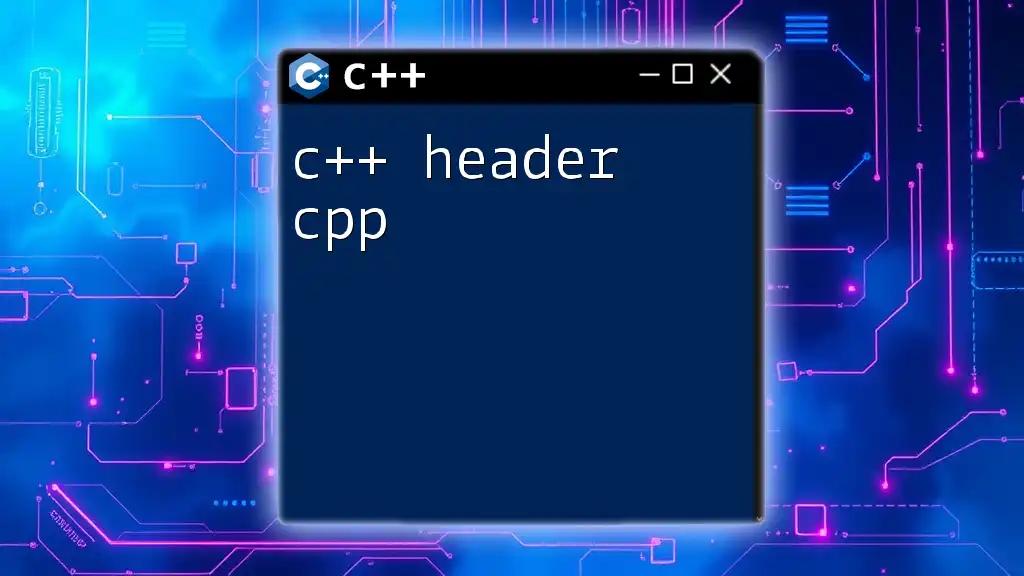
Alternative to Header Guards: `#pragma once`
What is `#pragma once`?
`#pragma once` is a modern alternative to traditional header guards. It is a preprocessor directive that tells the compiler to include the header file only once during a single compilation, regardless of how many times it is referenced. Here’s a simple use of `#pragma once`:
#pragma once
// Declarations and definitions
Pros and Cons of Using `#pragma once`
- Advantages:
- It simplifies the process by reducing boilerplate code. This can make your code tidier and easier to read.
- Disadvantages:
- While most modern compilers support `#pragma once`, some older compilers do not, which may lead to porting problems. Always consider the environments where your code will run.

Common Mistakes with Header Guards
Typographical Errors
One of the primary pitfalls people encounter with header guards is typographical errors. For instance, if you accidentally change the identifier in the `#define` directive but forget to update the corresponding `#ifndef`, your header guard will fail. This can result in redefinition errors, leading to compiler confusion. Always double-check your identifiers for consistency.
Consider this erroneous example:
#ifndef HEADER_FILE_NAME
#define HEADER_FIL_NAME // Typo here
// Declarations and definitions
#endif // HEADER_FILE_NAME
In this case, the second inclusion will not be recognized, and problems will arise.
Failure to Use Header Guards
Omitting header guards altogether can lead to a slew of complications, particularly in larger projects. If a header file is included multiple times in various source files, the compiler may face numerous redefinition errors. Here’s a brief example without proper guards:
// MyExample.h
void myFunction(); // Declaration
// Source file
#include "MyExample.h"
#include "MyExample.h" // This causes duplicate declaration errors
In the example above, the direct inclusion of `MyExample.h` twice will lead to errors. Implementing header guards resolves this issue immediately.

Real-World Applications of Header Guards
Enhancing Code Maintainability
Header guards are fundamental when it comes to maintaining clean, organized code. In extensive codebases, where numerous developers may work on different modules, the risk of header file complications rises significantly. By using header guards consistently, developers can eliminate clashing definitions that typically complicate code maintenance.
For instance, in a large software project that involves graphics and physics engines, header guards ensure that every module can interact with each other without causing cascading inclusion problems.
Libraries and Frameworks
In library development, header guards are indispensable. They ensure that the library can be used consistently across various projects without creating naming conflicts or redefinition errors. An excellent example would be the Standard Template Library (STL) in C++. By integrating header guards, STL can be included in multiple files without error, making it a robust choice for C++ developers.
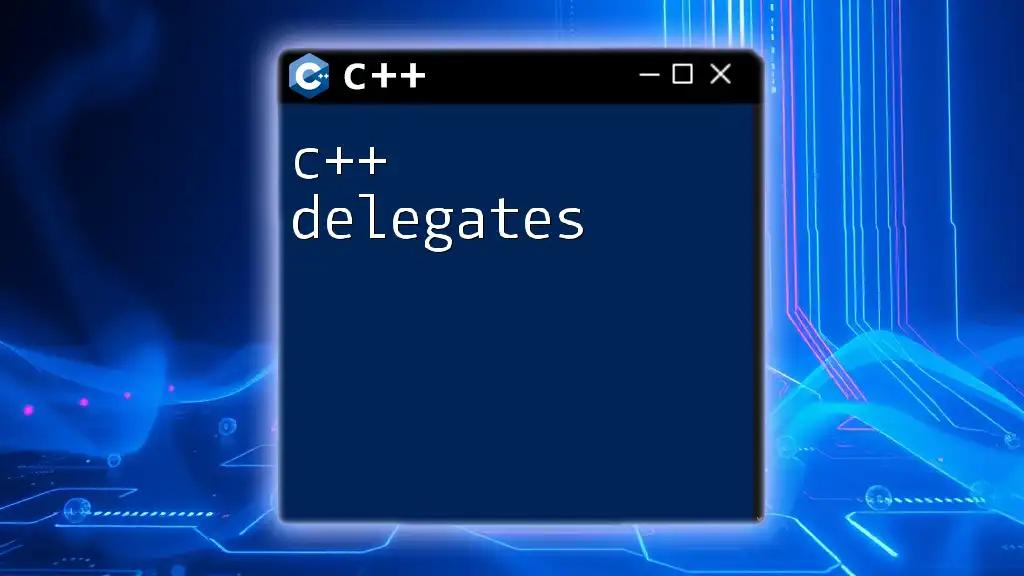
Conclusion
In conclusion, understanding and implementing C++ header guards is essential for any C++ developer. They not only provide a mechanism to prevent multiple inclusions but also promote better organization within your code. By adhering to best practices when defining unique identifiers and considering alternatives like `#pragma once`, you can enhance both the readability and maintainability of your code. Always remember to incorporate header guards in your header files—it's a small step that yields substantial benefits in the long run.

Additional Resources
For further exploration, consider checking out authoritative C++ resources, online forums, and communities dedicated to C++ programming. Each of these may provide valuable insights into mastering C++ header guards and other advanced topics.