A C++ style guide is a set of conventions and best practices designed to enhance code readability and maintainability by providing consistent formatting and naming conventions throughout the codebase.
// Example of a simple C++ function following style guide conventions
#include <iostream>
void PrintMessage(const std::string& message) {
std::cout << message << std::endl;
}
int main() {
PrintMessage("Hello, C++ Style Guide!");
return 0;
}
General Principles
Readability
Readability is essential for maintaining and collaborating on code. When code is easy to read, it becomes easier to understand and modify. Here are some techniques to enhance readability:
Whitespace: Properly utilizing spaces and newlines can greatly improve how code is perceived. For instance, separating function definitions and logical blocks with a blank line can make it easier for others to navigate through your code.
Indentation: Consistent indentation helps indicate code structure. Common practices recommend using 2 or 4 spaces per indentation level, rather than tabs.
Consistency
Consistency in formatting and naming conventions across your entire codebase enhances team collaboration. Adopting a common style, such as K&R or Allman, is essential. Choose a style and apply it uniformly. This consistency minimizes cognitive load when switching between different parts of the code.
Documentation and Comments
Importance of Code Comments
Comments are a form of documentation that can clarify complex code segments. However, it's important to differentiate between explaining what a code block does versus why it was written that way. Strive to comment on the intent rather than the implementation whenever possible.
Types of Comments
- Block Comments: Use them to provide context for larger code sections or to outline the purpose of a file. Example:
/*
* This function calculates the factorial of a number
* using a recursive approach.
*/
- Inline Comments: Use these for brief clarifications inline with the code. Example:
int factorial(int n) {
if (n == 0) return 1; // base case
return n * factorial(n - 1); // recursive step
}
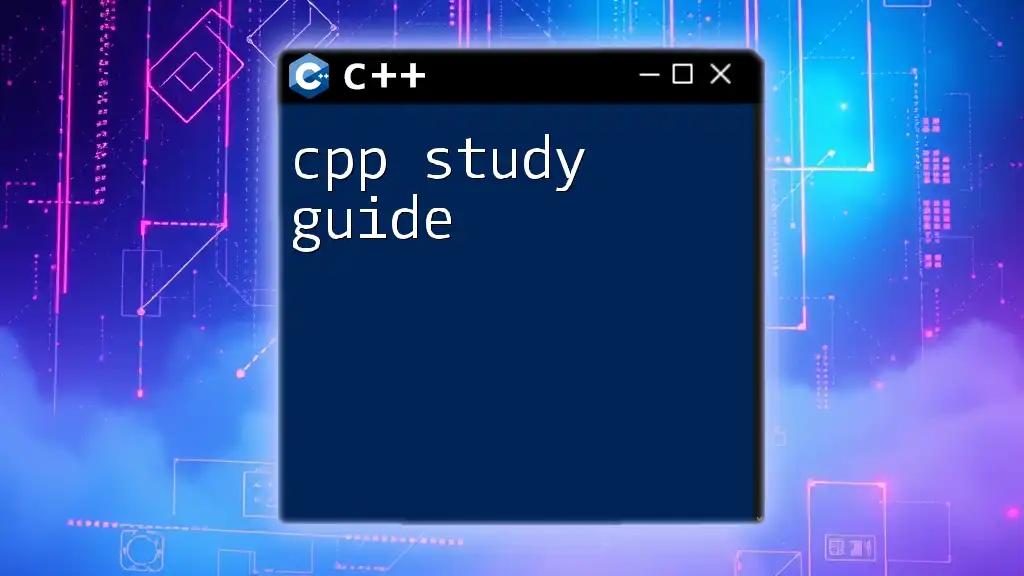
Naming Conventions
Variables
Following a suitable naming format is crucial. For example, you could use camelCase for local variables while preferring snake_case for global variables. Here are examples of both:
int myVariable; // camelCase
int my_variable; // snake_case
Good naming practices include using descriptive names that convey the variable's purpose:
int counter; // good
int c; // bad
Functions
Function names should be verbs that indicate what action they perform. Use clear and concise names:
void calculateTotal(); // good
void ctrTl(); // bad
Classes and Structs
Class names typically use PascalCase. Use meaningful names that reflect the role of the class.
Think of a `Car` class:
class Car {
// class definition here
};
For structs, you can use a similar naming convention but may choose to prefix with "S" (e.g., `SPoint`).
Constants
Constants make your code more readable when defined in UPPER_SNAKE_CASE. For example:
const int MAX_USERS = 100;
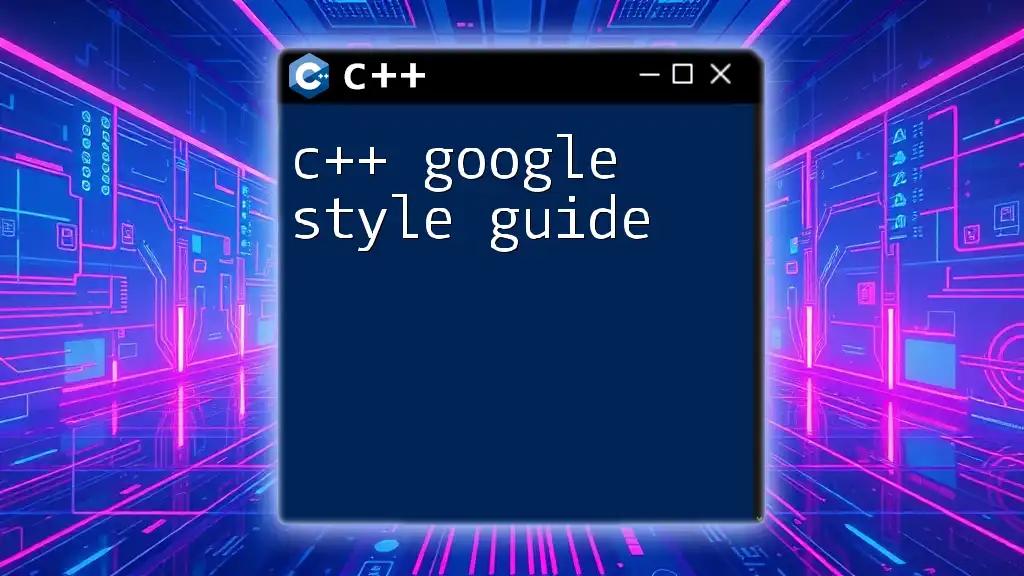
Code Formatting
Indentation and Braces
Consistent braces placement is vital. Many styles prefer placing opening braces on the same line as the statement. For example:
if (condition) {
// do something
}
Line Length
To promote readability, keep your lines to a maximum of 80 or 120 characters. This practice reduces the need to scroll horizontally, making your code easier to read.
Spacing Around Operators
Using spaces around operators can enhance readability. Here’s a comparison:
int total=a+b; // less readable
int total = a + b; // more readable
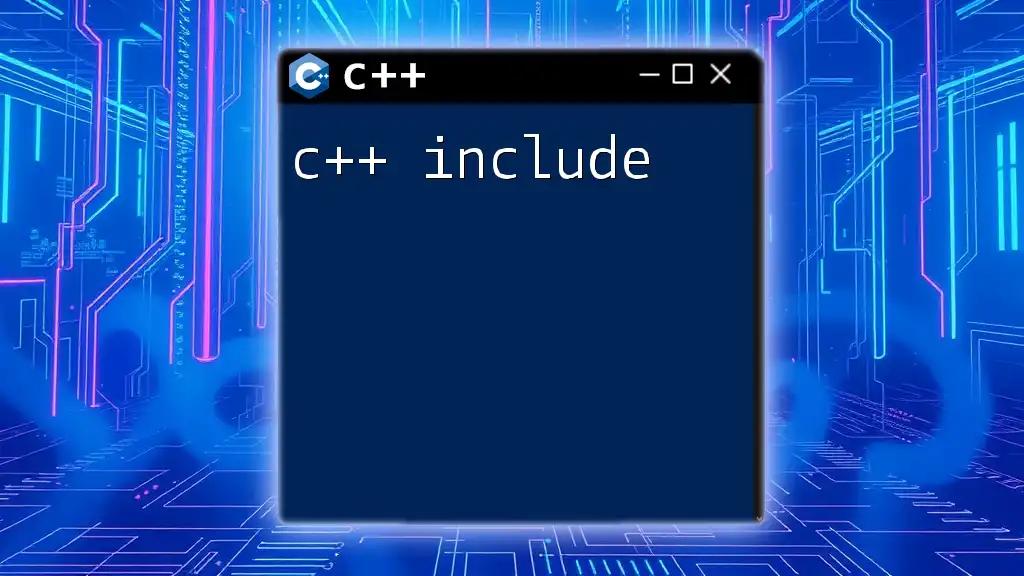
Object-Oriented Design Guidelines
Encapsulation
Encapsulation is a fundamental aspect of object-oriented programming. It promotes a separation of interface and implementation. For example:
class Account {
private:
double balance; // private variable
public:
void deposit(double amount) {
balance += amount;
}
double getBalance() const {
return balance;
}
};
Inheritance
When using inheritance, strive for clarity and relevance. Avoid deep inheritance trees that complicate understanding. Here's an example of correct usage:
class Animal {
public:
virtual void speak() = 0; // pure virtual function
};
class Dog : public Animal {
public:
void speak() override {
std::cout << "Woof!" << std::endl;
}
};
Polymorphism
Polymorphism allows for flexible code. Use it through function overriding to enable different behaviors for derived classes. Here's a brief illustration:
void makeAnimalSpeak(Animal* animal) {
animal->speak(); // calls speak() based on the derived class
}
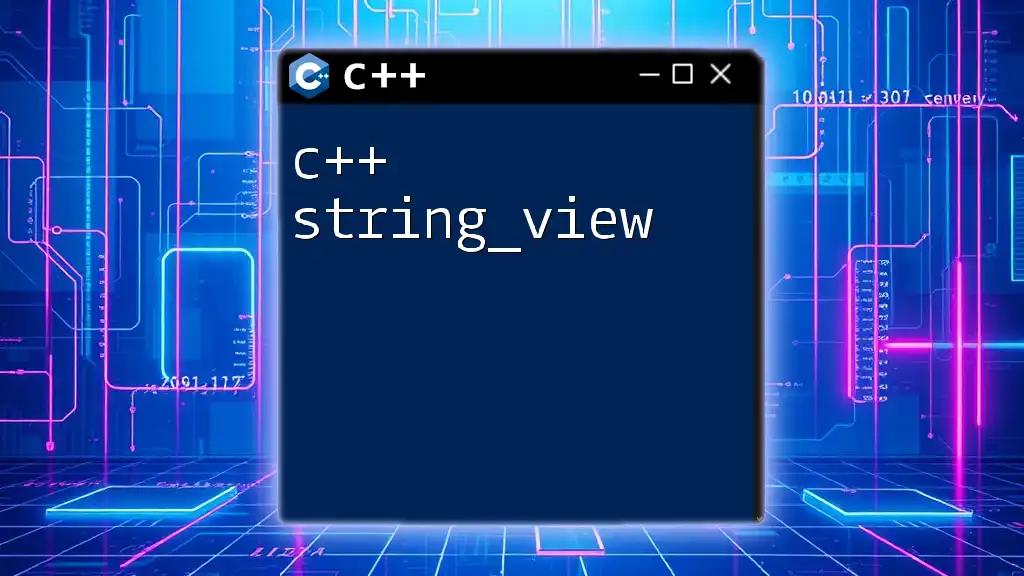
Error Handling
Exception Safety
Providing guarantees about exceptions is important. Aim to have a strong or basic guarantee for your operations. Here's an example of using a try-catch block for exception handling:
try {
// code that may throw
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
Assert vs. Exception
Use assertions for programmer errors and exceptions for conditions that can happen during program execution. An example of an assert:
assert(n >= 0); // check for non-negative input
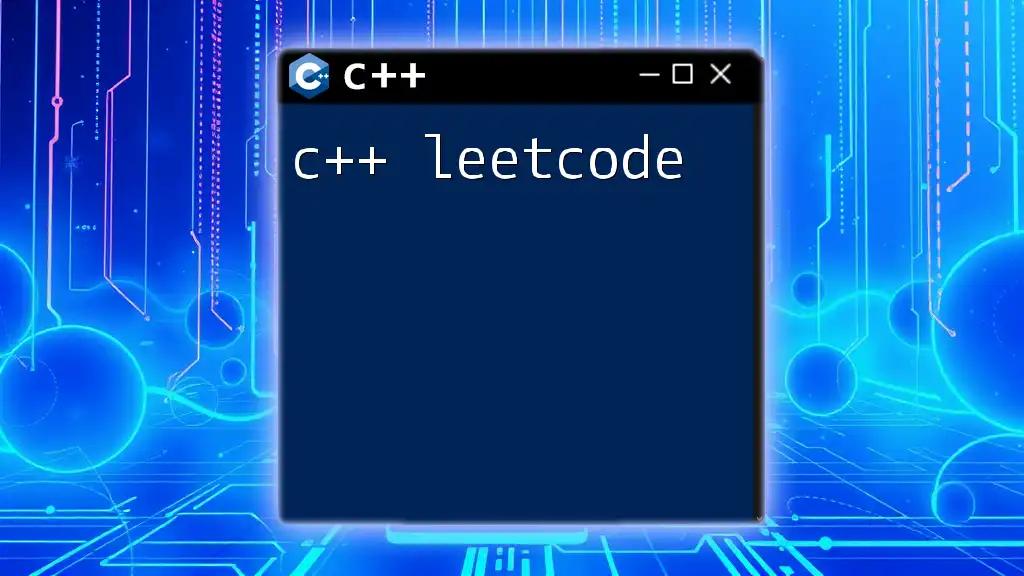
Best Practices for Use of Standard Library
STL Containers
The Standard Template Library (STL) offers several containers like `vector`, `list`, and `map`. Choose wisely based on your use case:
- Use `vector` for dynamic arrays when random access is crucial.
- Use `list` when frequent insertions and deletions are expected.
- Use `map` for key-value pair data storage when you require sorted access.
Algorithms
Leverage algorithms from `<algorithm>` to simplify operations. For instance, using `std::sort` in combination with a lambda function for custom sorting:
std::vector<int> numbers = {5, 3, 8, 1};
std::sort(numbers.begin(), numbers.end(), [](int a, int b) {
return a > b; // sort in descending order
});
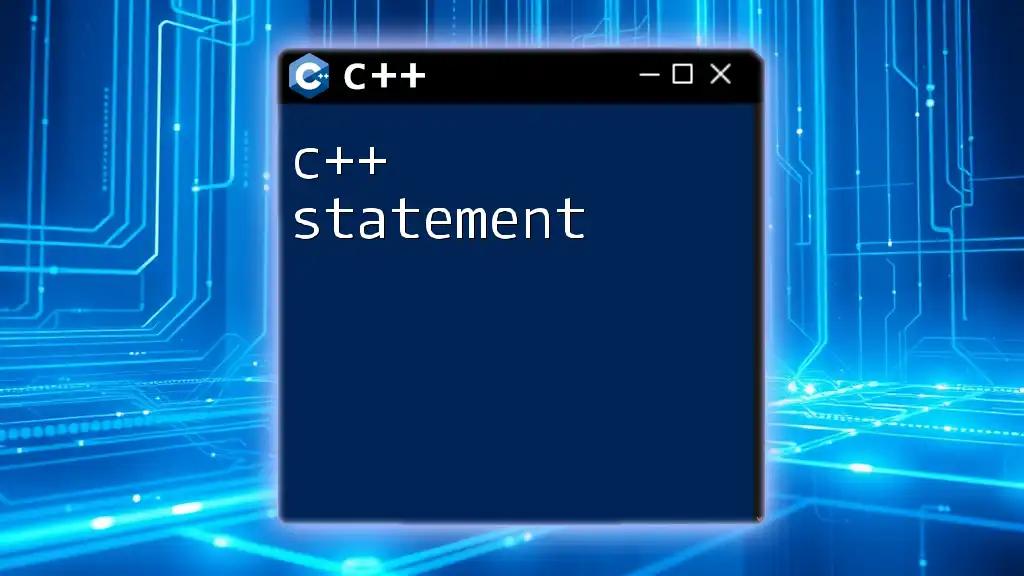
Testing and Debugging
Unit Testing
Unit testing is crucial for ensuring code reliability. Use frameworks like Google Test to automate and simplify this process. Here is a basic example of a unit test:
TEST(MyTestSuite, BasicAssertions) {
EXPECT_EQ(1, 1); // this will pass
}
Debugging Techniques
Employ techniques such as breakpoints and watch expressions to examine the state of the program. Using tools like `gdb` can help track down bugs effectively. An example command:
gdb ./my_program
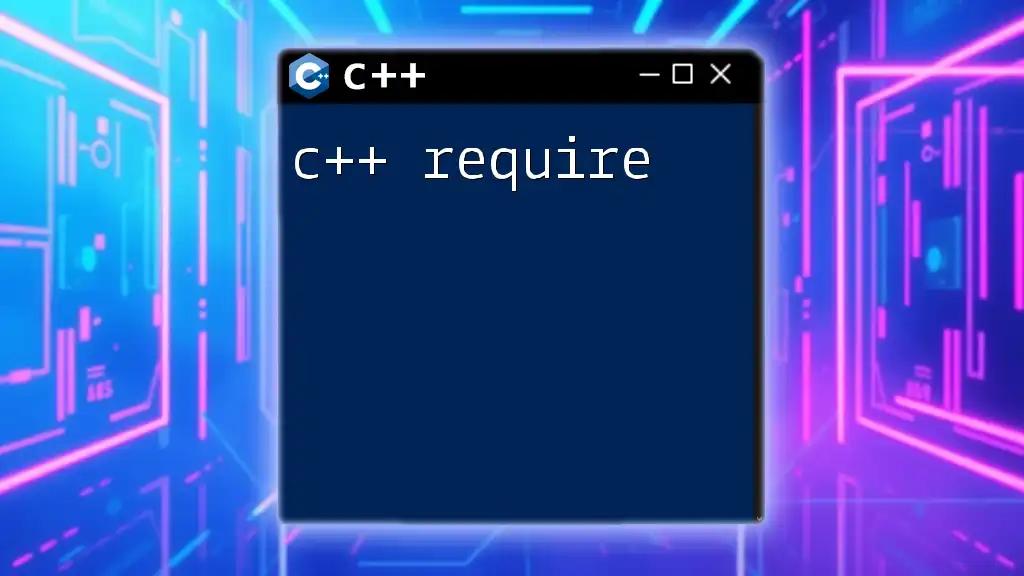
Conclusion
Throughout this guide, we’ve discussed the essential components of a C++ style guide, reinforcing the need for readability, consistency, and best practices. Following these guidelines not only enhances personal coding habits but also fosters better collaboration in team environments. The path towards adopting these practices is an ongoing journey; customize the style guide to suit your or your team’s needs as you grow in your C++ programming endeavors.
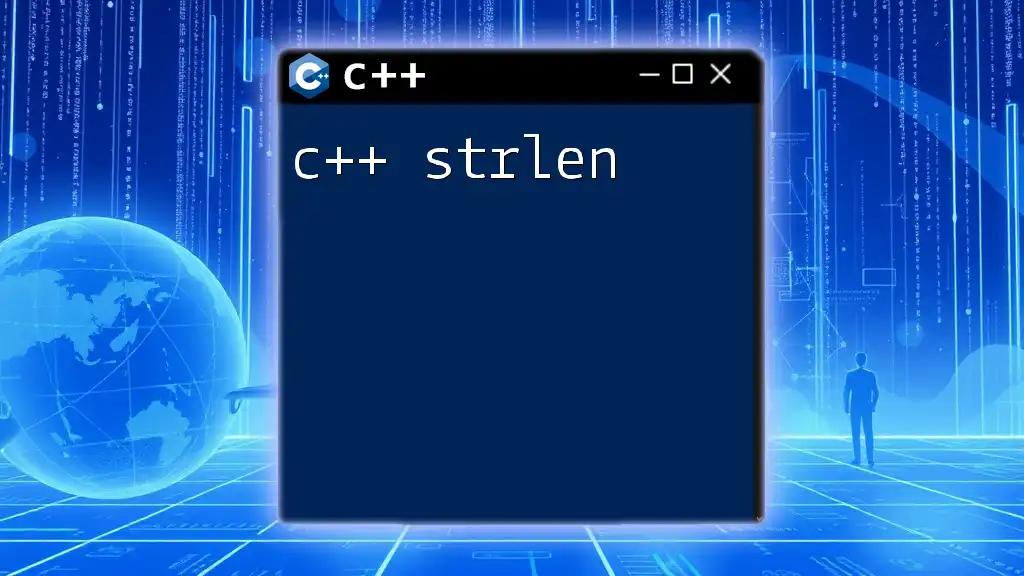
Additional Resources
For further exploration of C++ style conventions and coding practices, consider engaging with recommended books or online resources, including style guide templates available in various repositories. Embrace continuous learning as a key component of your development journey!