A CPP study guide is an essential resource that provides concise explanations and examples of C++ commands to streamline the learning process for beginners.
Here’s a simple code snippet demonstrating how to declare and initialize a variable in C++:
#include <iostream>
using namespace std;
int main() {
int number = 10; // Declare and initialize an integer variable
cout << "The number is: " << number << endl; // Output the variable
return 0;
}
Getting Started with C++
Setting Up Your Environment
Before diving into programming with C++, it's crucial to set up your development environment. You can choose from several compilers such as GCC, Clang, and MSVC. Each has its own advantages and works best on different systems. When selecting an IDE, consider options like Visual Studio, Code::Blocks, or Eclipse. Installing these tools and configuring them properly creates an efficient workspace where you can write, debug, and run your C++ code seamlessly.
Writing Your First C++ Program
Now that your environment is ready, let’s create your first C++ program—a classic "Hello, World!" example. This simple program demonstrates the basic structure of a C++ application.
Here’s the code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Breaking it down:
- `#include <iostream>`: This includes the standard input-output stream library.
- `int main()`: This marks the beginning of the main function.
- `std::cout`: This outputs text to the standard console.
- `return 0;`: This indicates that the program finished successfully.
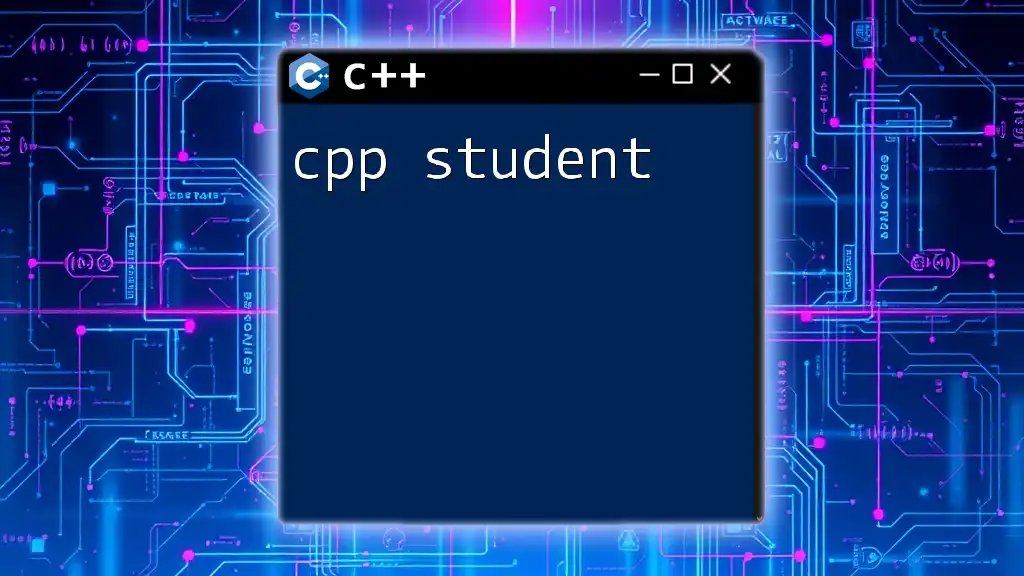
C++ Syntax and Structure
Basic Syntax Rules
C++ has specific syntax rules that you need to follow:
- C++ is case-sensitive, meaning `Variable` and `variable` are treated as different identifiers.
- Comments are vital for documentation. You can use single-line comments `//` or multi-line comments `/* ... */`.
Understanding Data Types
C++ includes several built-in data types, which are essential for defining variables:
- int: Used for integers.
- float: Used for single-precision floating-point numbers.
- double: For double-precision floating-point numbers.
- char: For single characters.
You can also create user-defined data types using struct, union, enum, and typedef. Here's a simple example of variable declaration:
int age = 30;
float salary = 50000.50;
char grade = 'A';
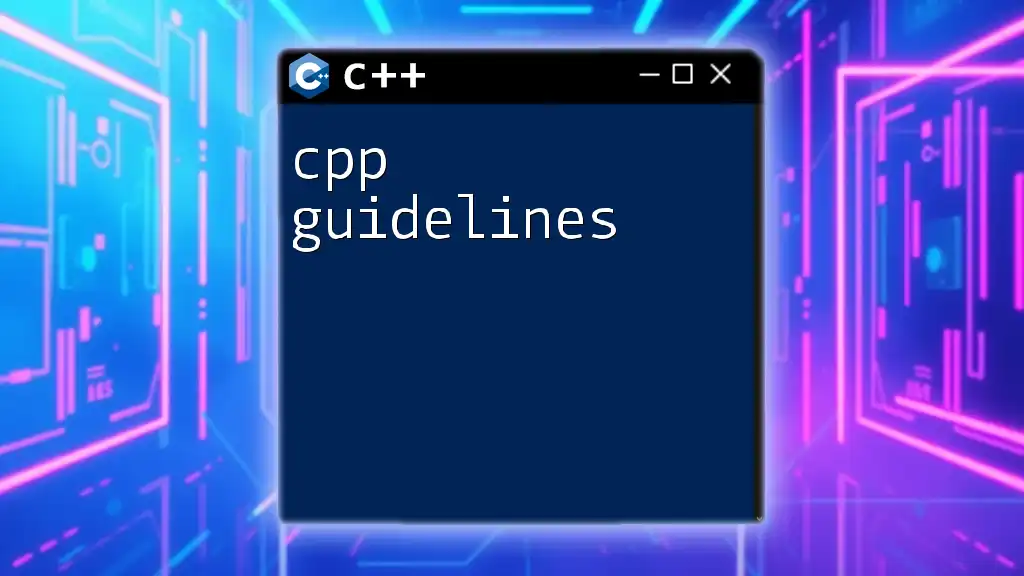
Control Structures
Conditional Statements
Control the flow of your program using conditional statements. The if, else if, and else keywords allow you to make decisions in your code. Alternatively, use the switch-case statement to choose between multiple options. Here is an example using switch-case:
int day = 4;
switch(day) {
case 1:
std::cout << "Monday";
break;
case 2:
std::cout << "Tuesday";
break;
//...
default:
std::cout << "Invalid day";
}
Loops
Loops are critical for repeating actions in your programs. C++ provides several loop types:
- for loop: Ideal when the number of iterations is known.
- while loop: Use this when the iterations depend on a condition.
- do-while loop: Similar to while, but it guarantees at least one iteration.
Example of a for loop:
for(int i = 0; i < 5; i++) {
std::cout << i << " ";
}
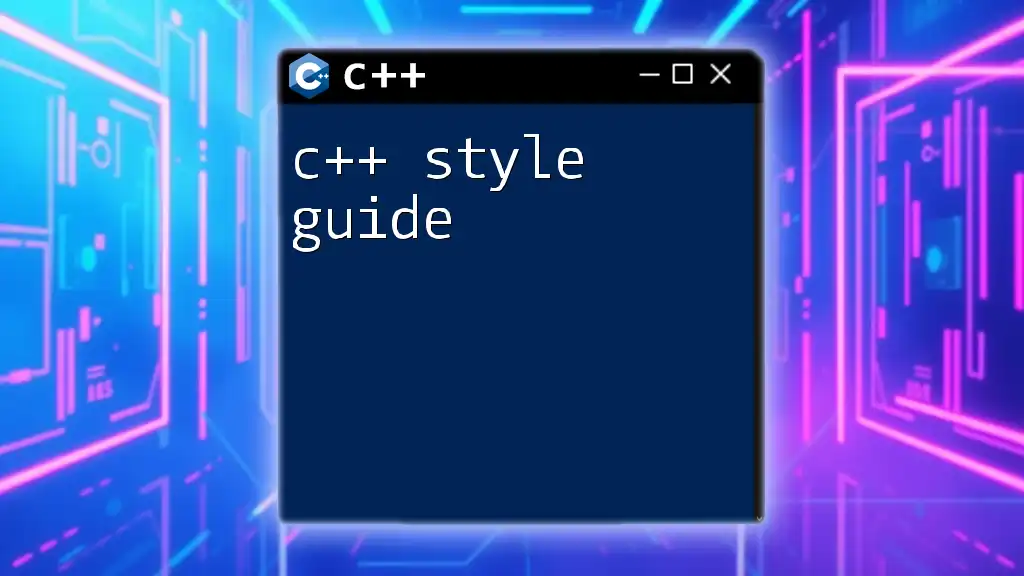
Functions in C++
Defining and Calling Functions
Functions allow you to encapsulate code for reuse. The syntax for defining a function in C++ is straightforward. Here’s how you can create a simple function:
void greet() {
std::cout << "Welcome to C++!" << std::endl;
}
To call this function, simply write `greet();`. Functions can also accept parameters and return values, enhancing their utility.
Here’s an example that illustrates function parameters:
int sum(int a, int b) {
return a + b;
}
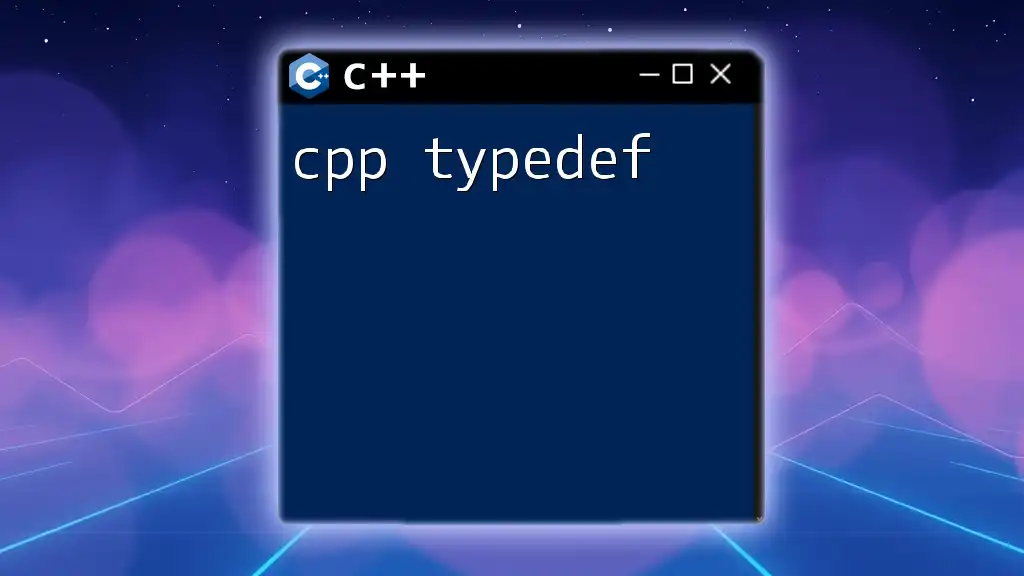
Object-Oriented Programming (OOP) in C++
Introduction to OOP Concepts
C++ is renowned for its strong support of Object-Oriented Programming (OOP) principles. Basic concepts include:
- Classes and Objects: A class is a blueprint for creating objects.
- Inheritance: Allows one class to inherit attributes and methods from another.
- Polymorphism: Enables a single interface to represent different underlying forms (data types).
Creating Classes
Here’s an example of defining a simple class in C++ that includes a method:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Understanding Constructors and Destructors
Classes in C++ can have constructors, which are special functions called when an object is created, and destructors, called when an object goes out of scope. This ensures proper resource management. You can define them as follows:
class MyClass {
public:
MyClass() { // Constructor
std::cout << "Constructor called!" << std::endl;
}
~MyClass() { // Destructor
std::cout << "Destructor called!" << std::endl;
}
};
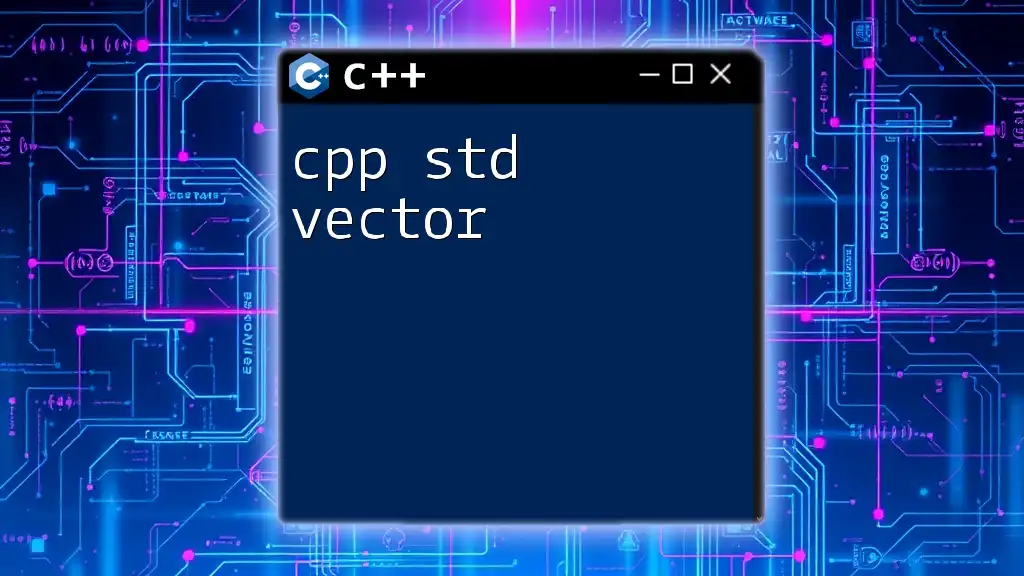
Standard Template Library (STL)
Introduction to STL
The Standard Template Library (STL) is a powerful feature of C++ that provides generic classes and functions. It plays a crucial role in writing efficient and reusable code.
Common STL Components
Some of the most commonly used components of STL include vectors, lists, and maps. Vectors are dynamic arrays that can grow or shrink in size. Here’s how you can use a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
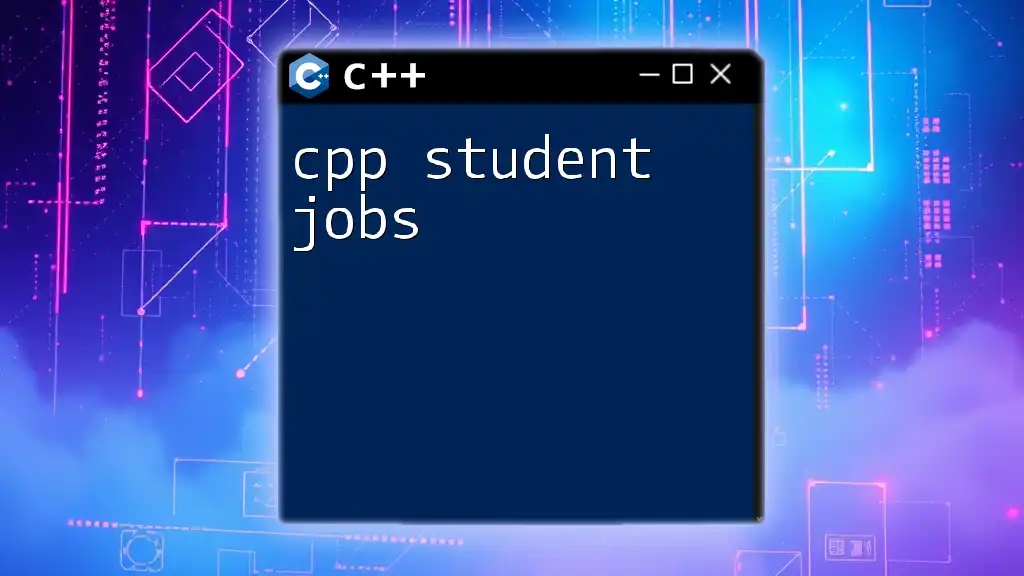
Best Practices in C++
Code Readability and Maintenance
Focus on writing clean, maintainable code. Use consistent naming conventions and keep your code well-commented. This makes it easier for others (and yourself) to understand your logic later.
Error Handling
Error handling is crucial for building reliable applications. Use exceptions to manage unforeseen issues gracefully. Here’s a basic example with try and catch:
try {
throw std::runtime_error("Error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
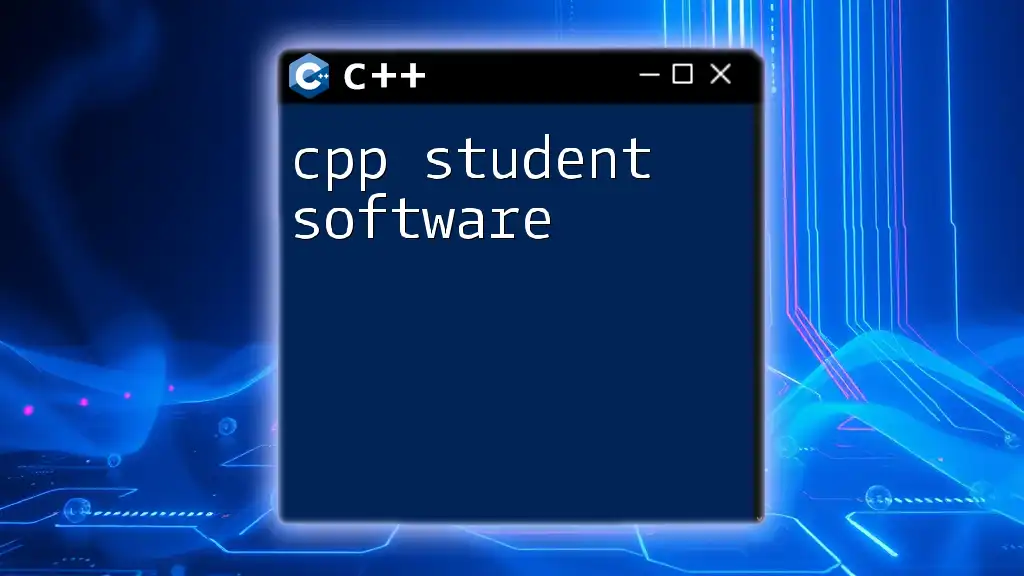
Conclusion
In summary, this cpp study guide has provided you with the essential tools to begin programming in C++. From understanding the basic syntax to leveraging OOP principles and the STL, you have a solid foundation to build upon. Continuously engage with C++ by exploring more advanced topics and practicing regularly to improve your skills.
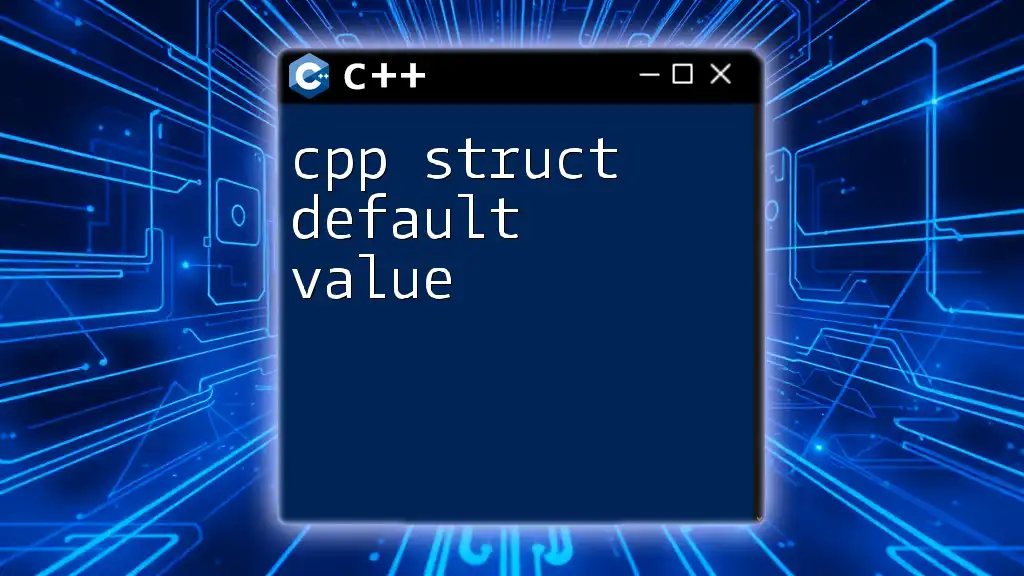
Call to Action
For more tutorials and resources, visit our website. Join our community where you can seek help and discuss ideas with fellow C++ enthusiasts. Happy coding!