"CPP student software refers to educational tools and platforms designed to help learners master C++ commands and programming practices efficiently."
Here is a simple example of a C++ command to demonstrate basic output:
#include <iostream>
int main() {
std::cout << "Hello, C++ Student!" << std::endl;
return 0;
}
Understanding C++ Student Software
Definition of C++ Student Software
C++ student software encompasses a variety of tools and applications designed to assist learners in mastering the C++ programming language. These tools provide environments for writing, debugging, and compiling C++ code, making the learning process smoother and more engaging. Understanding the available software can significantly enhance your experience as you navigate the complexities of C++.
Benefits of Using C++ Student Software
Using C++ student software offers numerous advantages:
Enhanced Learning: The interactive nature of many software tools allows students to visualize concepts, improving their understanding of programming principles. This is especially valuable for beginners trying to grasp foundational ideas.
Practical Experience: C++ student software enables hands-on experience with code, preparing students for real-world applications. It allows learners to experiment, make mistakes, and learn from them in a controlled environment.
Collaboration: Many platforms facilitate peer interactions, enabling students to work together on projects. Collaborative features foster teamwork and help learners tackle complex problems collectively.
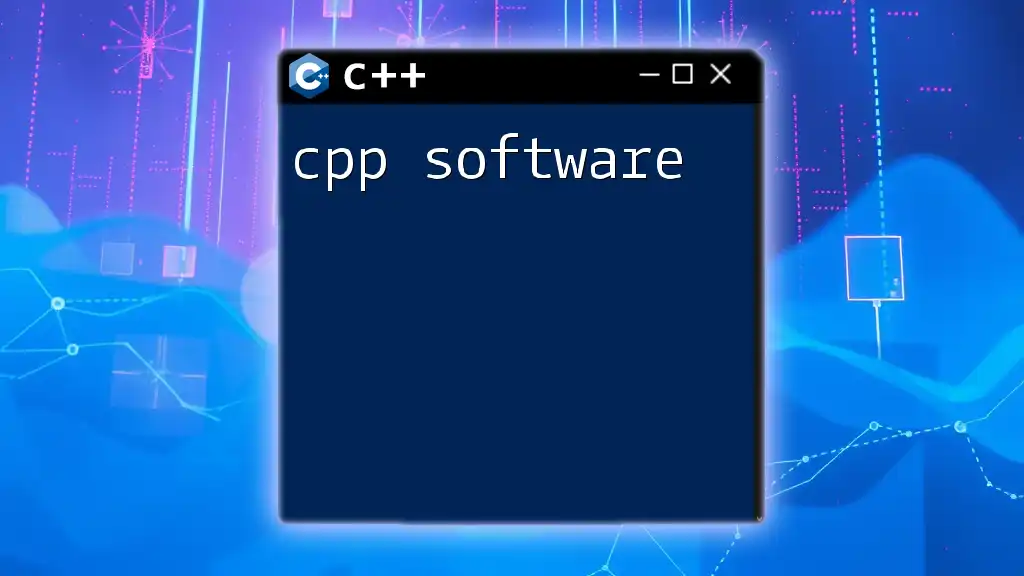
Key Features to Look for in C++ Student Software
User-Friendly Interface
When choosing C++ student software, a user-friendly interface is crucial. A clear and intuitive design makes navigating the software easier, especially for those new to programming. An ideal interface minimizes distractions and emphasizes functionality, allowing students to focus on learning rather than grappling with complex menus.
Code Compilers and Linters
Compilers play a vital role in transforming C++ source code into executable programs. It's essential for students to understand the difference between compilers, as performance and user experiences can vary widely. GCC (GNU Compiler Collection) and Clang are two of the most popular options.
Linters also enhance the learning experience by automatically checking code for errors and inconsistencies, helping students catch mistakes early. Using a linter alongside a compiler can build good coding habits by encouraging proper syntax and style.
Integrated Development Environment (IDE) Features
An Integrated Development Environment (IDE) offers a suite of tools that facilitate code development. When selecting an IDE, look for these key features:
- Syntax Highlighting: This feature helps to easily differentiate between keywords, variables, and types, improving readability.
- Debugging Tools: A robust debugger allows students to step through code and inspect variables, which is invaluable for understanding how programs operate.
- Code Completion: This feature provides suggestions as you type, reducing errors and improving coding speed.
- Project Management: An IDE that supports managing multiple projects aids in organization and makes it easier to switch between tasks.
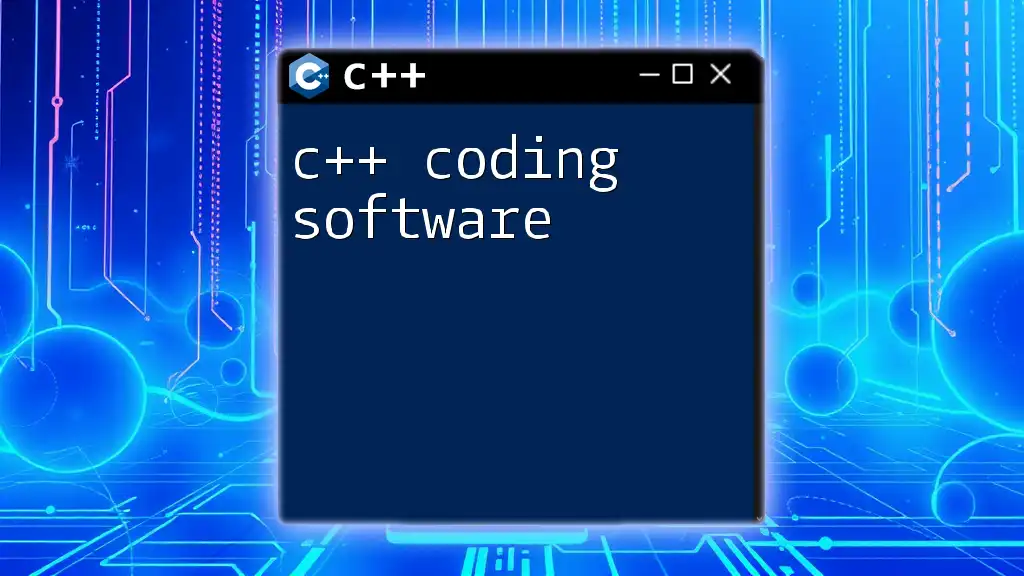
Recommended C++ Student Software
Online C++ Compilers
Repl.it
Repl.it is a popular online coding platform that allows you to write and execute C++ code directly in your browser. Its real-time collaboration feature enhances learning by allowing you to code with peers or instructors simultaneously. With Repl.it, you can easily test snippets like:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Students can engage with the community and learn from shared projects, making it an excellent resource for collaborative learning.
JDoodle
JDoodle is another user-friendly online compiler that supports multiple programming languages, including C++. It is simple to navigate and offers features such as code sharing. For example, you can execute a basic arithmetic operation with:
#include <iostream>
using namespace std;
int main() {
int a = 5;
int b = 10;
cout << "Sum: " << (a + b) << endl;
return 0;
}
JDoodle is beneficial for quick coding tests and experimenting with code snippets without downloading software.
Desktop IDEs
Code::Blocks
Code::Blocks is an open-source IDE specifically tailored for C++. It offers a range of features that are beneficial for students, including a robust debugger and project wizards. Setting up a project is straightforward, allowing you to write a simple program like:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to Code::Blocks!" << endl;
return 0;
}
Students can organize multiple projects effectively within the IDE.
Dev-C++
Dev-C++ is another excellent option, providing a lightweight IDE for C++ development. The setup is simple, with built-in features like syntax highlighting and project management. You can delve into libraries and create programs like:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
cout << num << " ";
}
cout << endl;
return 0;
}
Dev-C++ supports various compilers, giving students flexibility in their development environment.
Visual Studio
Visual Studio is a powerful IDE that offers a student-friendly version. It includes advanced debugging features and a plethora of resources for learning. To start a new project, just follow the setup wizard, which streamlines the initial process. A simple example code in Visual Studio can look like this:
#include <iostream>
using namespace std;
int main() {
cout << "Visual Studio is great for C++!" << endl;
return 0;
}
Students are encouraged to utilize the extensive online documentation and community support offered through Visual Studio.
Learning and Practice Platforms
Codecademy
Codecademy provides an interactive C++ course aimed at beginners. Its hands-on approach ensures that students can practice coding directly in their browser. For instance, students may learn to implement functions with exercises like:
#include <iostream>
using namespace std;
// Function to add two numbers
int add(int x, int y) {
return x + y;
}
int main() {
cout << "Sum: " << add(3, 4) << endl;
return 0;
}
This platform reinforces learning through immediate feedback.
LeetCode
LeetCode is invaluable for students interested in algorithm development and competitive programming. By practicing problems on LeetCode, learners can apply their C++ knowledge. Sample algorithm problems may include:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> nums = {2, 7, 11, 15};
int target = 9;
for (int i = 0; i < nums.size(); i++) {
for (int j = i + 1; j < nums.size(); j++) {
if (nums[i] + nums[j] == target) {
cout << "Indices: " << i << ", " << j << endl;
}
}
}
return 0;
}
This platform not only enhances programming skills but also prepares students for technical interviews.
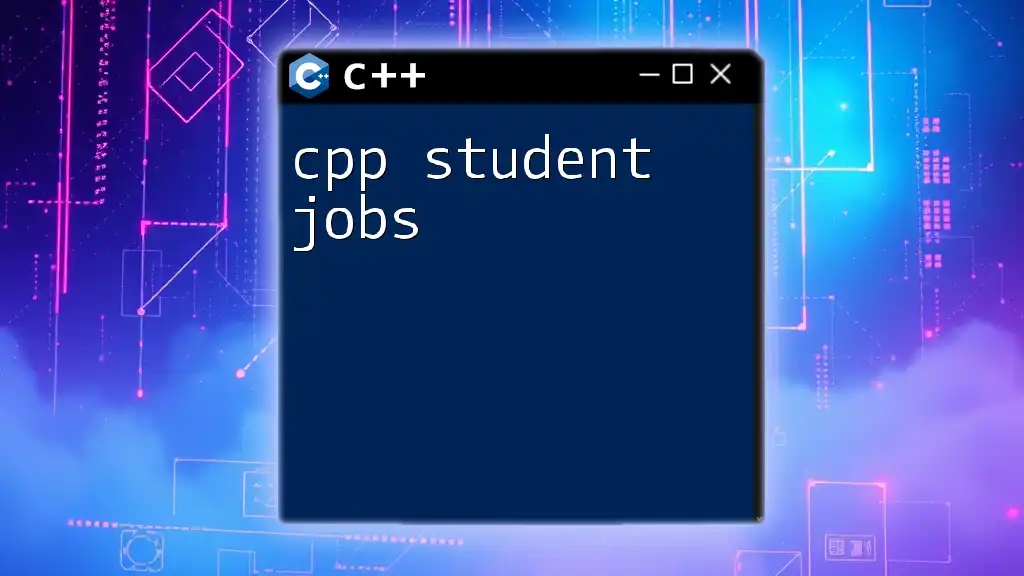
Best Practices for Using C++ Student Software
Tips for Maximizing Your Learning Experience
To get the most out of C++ student software, consider the following tips:
Stay Organized: Keep your projects and files structured. Use clear naming conventions for your files and folders to make them easily retrievable later.
Documentation is Key: Thoroughly read documentation and incorporate comments in your code. This will help you understand your code's functionality and assist anyone who might read it in the future.
Practice Regularly: Consistent practice is essential. Instead of cramming, set aside regular time for coding exercises and projects. The more you practice, the better you’ll understand C++.
Engaging with the Community
Connecting with others can be incredibly beneficial. Participating in forums like Stack Overflow or engaging with C++ subreddits allows you to ask questions, share knowledge, and learn from others’ experiences. These communities often provide support and resources that can elevate your understanding of C++.
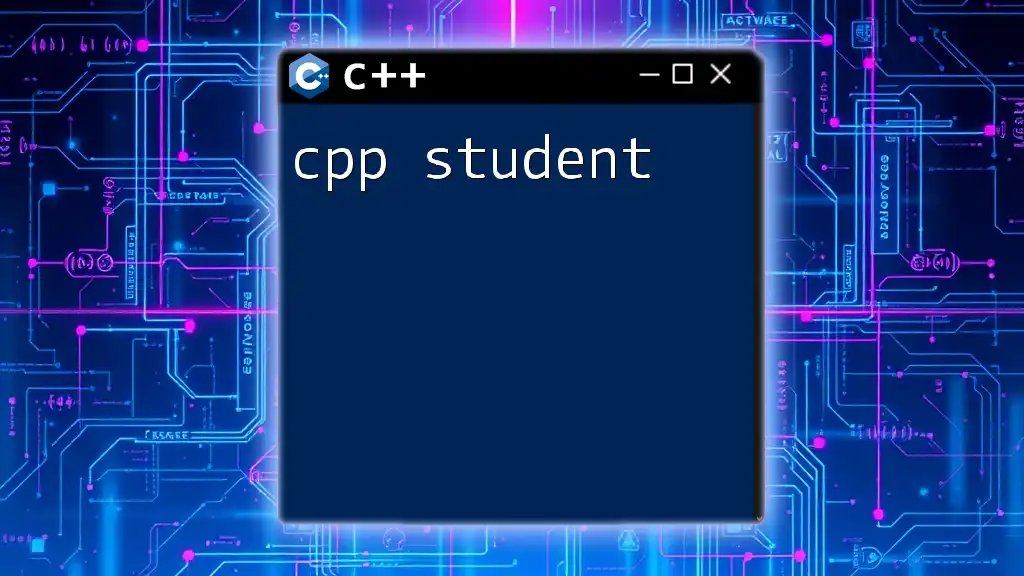
Common Mistakes to Avoid
Neglecting Error Messages
One common mistake many students make is ignoring error messages. Understanding error messages is crucial for debugging code effectively. For example, if encountering a compilation error, take the time to familiarize yourself with the terminology and implications of the messages provided.
Skipping the Fundamentals
It’s tempting to dive into advanced topics, but skipping foundational elements can hinder your long-term success. A solid grasp of basic concepts, such as variables, control structures, and functions, is vital. Utilize resources that reiterate these concepts, ensuring you have a strong foundation.
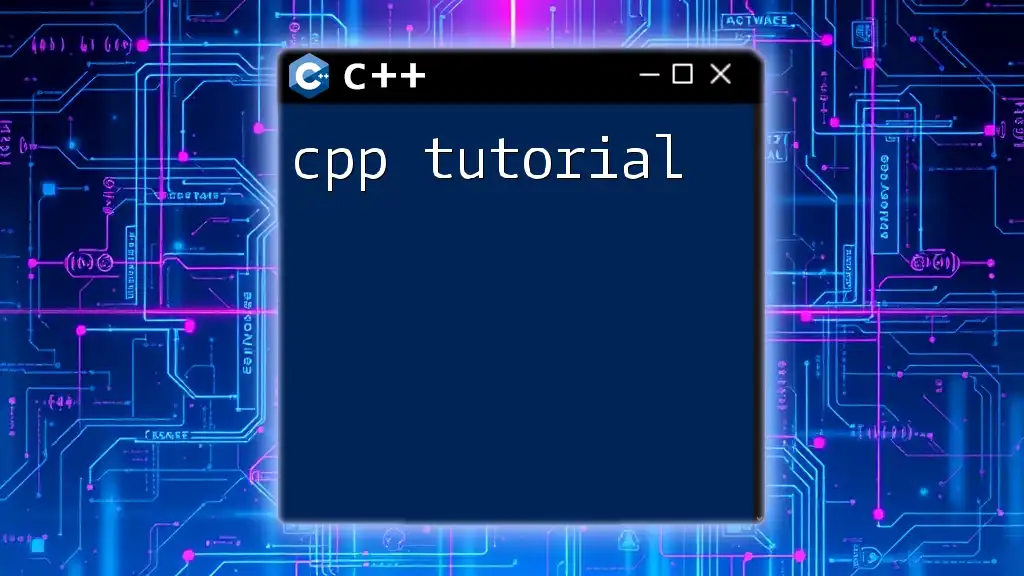
Conclusion
Incorporating the right C++ student software into your learning path can significantly enhance your programming skills. By leveraging IDEs, compilers, and interactive platforms, you will create a supportive environment that fosters growth and knowledge. As you explore these tools, remember to engage with the community, practice regularly, and avoid common pitfalls. Embrace the learning journey, and let these software tools guide you toward becoming a proficient C++ programmer.