"CPP Euclid" refers to implementing Euclid's algorithm in C++ to efficiently calculate the greatest common divisor (GCD) of two integers.
Here’s a simple implementation:
#include <iostream>
int gcd(int a, int b) {
return b == 0 ? a : gcd(b, a % b);
}
int main() {
int num1 = 56, num2 = 98;
std::cout << "GCD of " << num1 << " and " << num2 << " is " << gcd(num1, num2) << std::endl;
return 0;
}
What is Euclid's Algorithm?
Euclid's Algorithm is a method for efficiently computing the greatest common divisor (GCD) of two integers. Originating from ancient Greek mathematics, it highlights the foundational principles of number theory. Understanding this algorithm is crucial for numerous applications in computer science and programming.
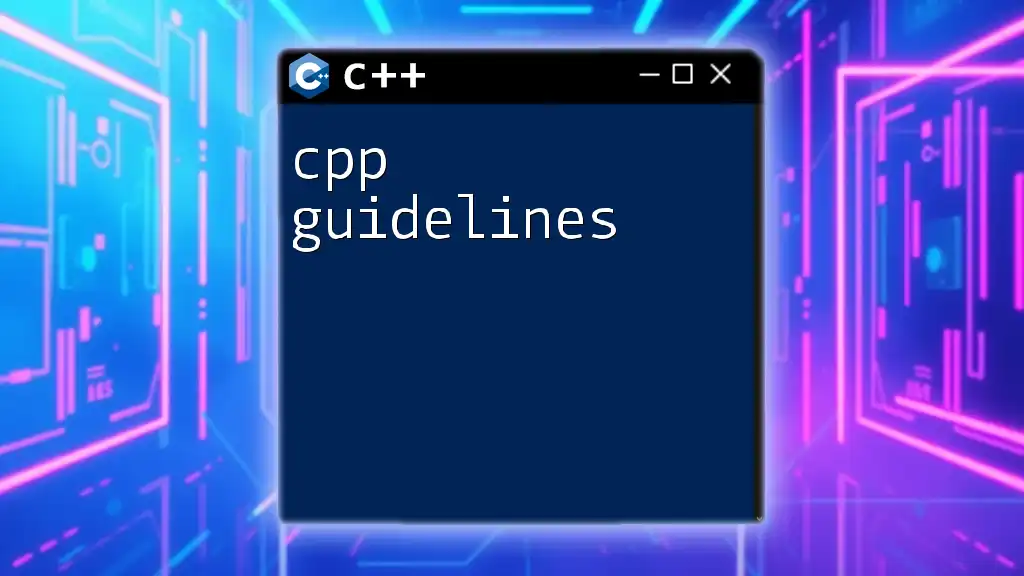
C++ Overview
C++ is a versatile programming language that combines features of both high-level and low-level languages. It is widely used for system software, game development, and applications requiring real-time processing. The language's ability to manipulate hardware resources efficiently makes it ideal for implementing algorithms like Euclid's.
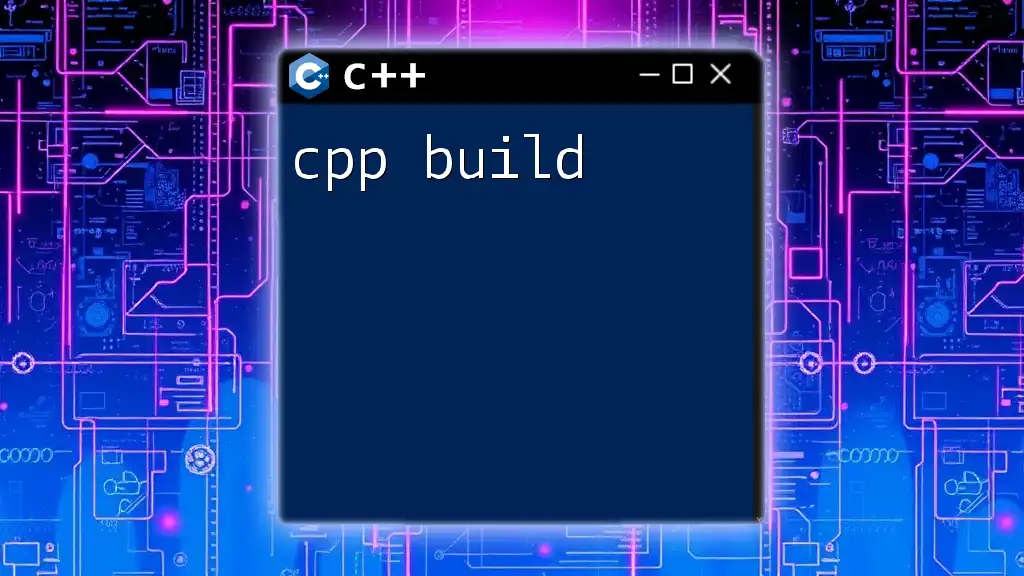
Euclid's Algorithm Explained
What is Euclid's Algorithm?
At its core, Euclid’s Algorithm is based on the principle that the GCD of two numbers can be determined by repeatedly applying the modulus operation. Given two integers, \( a \) and \( b \), the GCD can be recursively defined as follows:
- If \( b = 0 \), then \( \text{gcd}(a, b) = a \).
- Otherwise, \( \text{gcd}(a, b) = \text{gcd}(b, a \mod b) \).
This property greatly simplifies the process of finding the GCD.
How Euclid's Algorithm Works
Let's break down the algorithm step-by-step:
- Start with two integers \( a \) and \( b \).
- If \( b \) is zero, return \( a \) as the GCD.
- Otherwise, replace \( a \) with \( b \) and \( b \) with \( a \mod b \).
- Repeat this process until \( b \) becomes zero.
Recursive Implementation in C++
Understanding Recursive Functions
Recursion is a powerful programming technique where a function calls itself to solve smaller sub-problems. By understanding how to implement recursive functions, programmers can create elegant and compact solutions.
Code Snippet: Recursive GCD Function
Here's a simple implementation of the recursive version of Euclid’s Algorithm in C++:
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
Explanation of the Code Snippet:
- The base case checks if \( b \) is zero. If true, it returns \( a \), which is the GCD.
- The recursive call continues until the base case is met. The function computes the GCD by swapping the numbers and calling itself again with the modulus operation.
Iterative Implementation in C++
Understanding Iterative Functions
While recursion is elegant, iterative solutions can improve performance and reduce memory usage. Iterative solutions use loops instead of function calls and can be more efficient in some contexts.
Code Snippet: Iterative GCD Function
Here’s how you can implement the iterative version of Euclid's Algorithm:
int gcd(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
Explanation of the Code Snippet:
- The `while` loop continues until \( b \) equals zero.
- Inside the loop, a temporary variable `temp` holds the value of \( b \).
- The values are updated using the modulus operation. This process efficiently calculates the GCD.
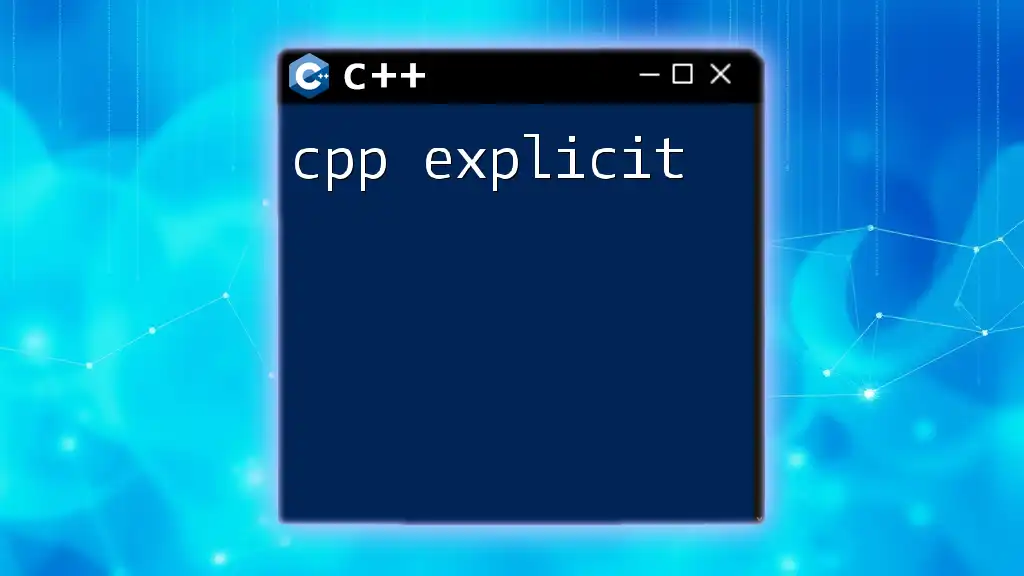
Real-World Applications of Euclid's Algorithm
Where is GCD Used? The GCD has numerous real-world applications, including:
- Cryptography: In public key encryption schemes like RSA, the GCD is used to determine coprimality of numbers, which is fundamental in creating secure keys.
- Data Compression: Algorithms like Huffman coding rely on the GCD in their operation.
Understanding these applications can motivate developers to grasp Euclid's Algorithm more deeply.
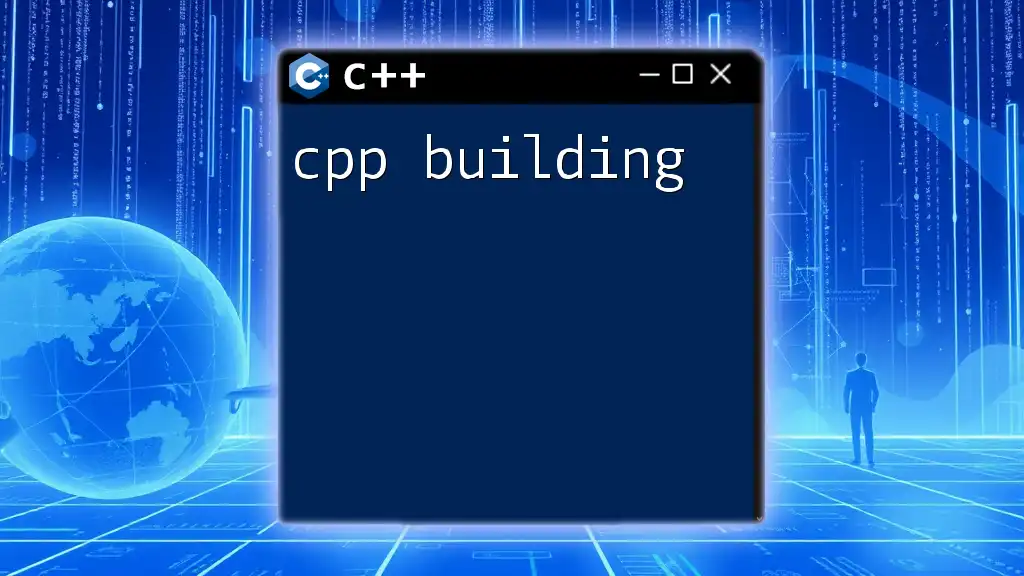
Optimization Techniques in Euclid's Algorithm
Enhancing Performance
Optimizing the GCD calculation can lead to significant performance improvements, particularly when dealing with large numbers. One common optimization is using bitwise operations.
Code Snippet: Optimized GCD using Bitwise Operations
Here’s an optimized implementation that uses bitwise operations:
int gcd(int a, int b) {
if (b == 0) return a;
if (a == 0) return b;
if ((~a & 1) && (b & 1)) return gcd(a >> 1, b);
if ((a & 1) && (~b & 1)) return gcd(a, b >> 1);
if ((~a & 1) && (~b & 1)) return gcd(a >> 1, b >> 1) << 1;
return gcd(abs(a - b), std::min(a, b));
}
Explanation of the Code Snippet:
- This function uses bitwise checks to determine if \( a \) or \( b \) are even, which allows it to reduce the numbers more efficiently.
- Bitwise shifts (`>>`) are used to divide numbers by 2 without the division operator, enhancing performance.
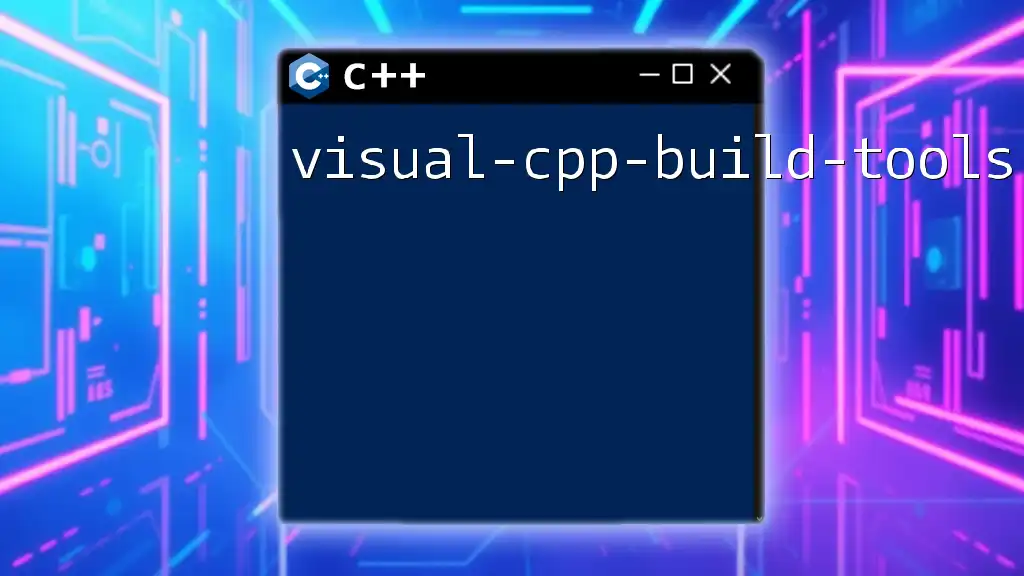
Common Errors and Troubleshooting
When implementing Euclid's Algorithm, programmers may encounter common pitfalls:
- Infinite Recursion: Ensure that the base case is properly defined to prevent an infinite loop in recursive implementations.
- Incorrect Modulus Operation: Carefully implement the modulus operation, particularly when dealing with negative numbers, to ensure accurate results.
For instance, if a function repeatedly outputs incorrect GCDs, review the logic behind the modulus and swapping of variables.
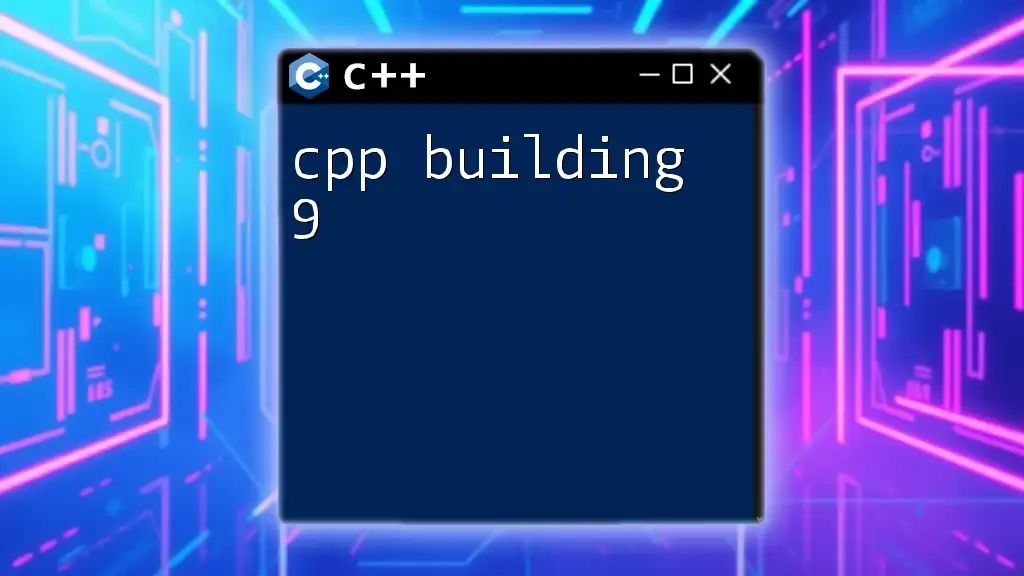
Recap of Key Points
Understanding cpp euclid is critical for both programming and mathematical insight. The efficiency of computing the GCD through recursion and iteration illustrates the elegance of algorithms. Furthermore, optimization techniques, such as utilizing bitwise operations and recognizing common errors, contribute to refining your programming skills.
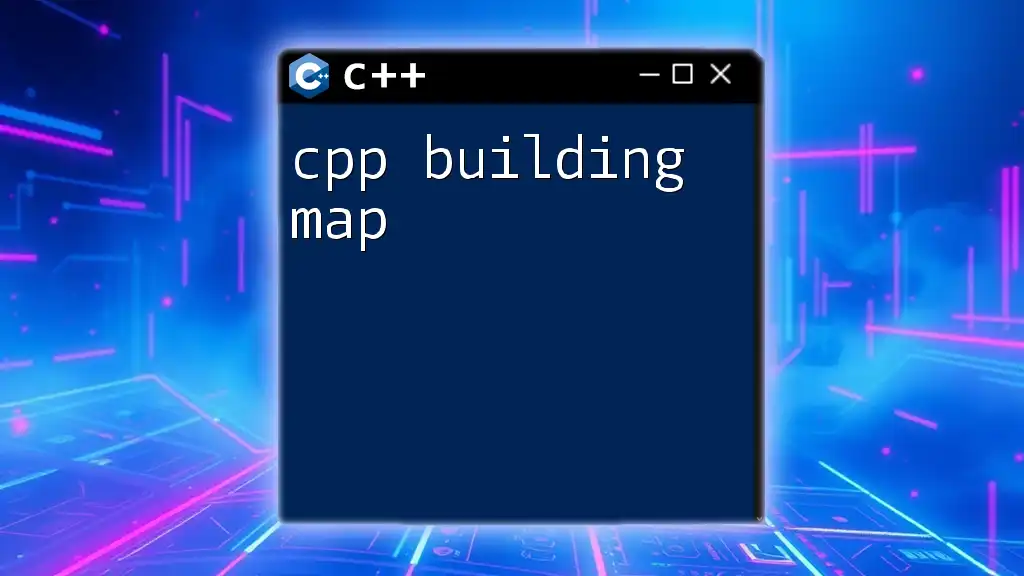
Encouragement for Further Learning
To become proficient in C++ and algorithmic thinking, try implementing variations of the GCD, understand other algorithms such as the Least Common Multiple (LCM), and explore different number theoretic applications.
Additional Resources
For continued learning, consider diving into recommended readings, online courses, and joining communities focused on C++ programming. Networking with fellow programmers can enhance your understanding and application of algorithms.
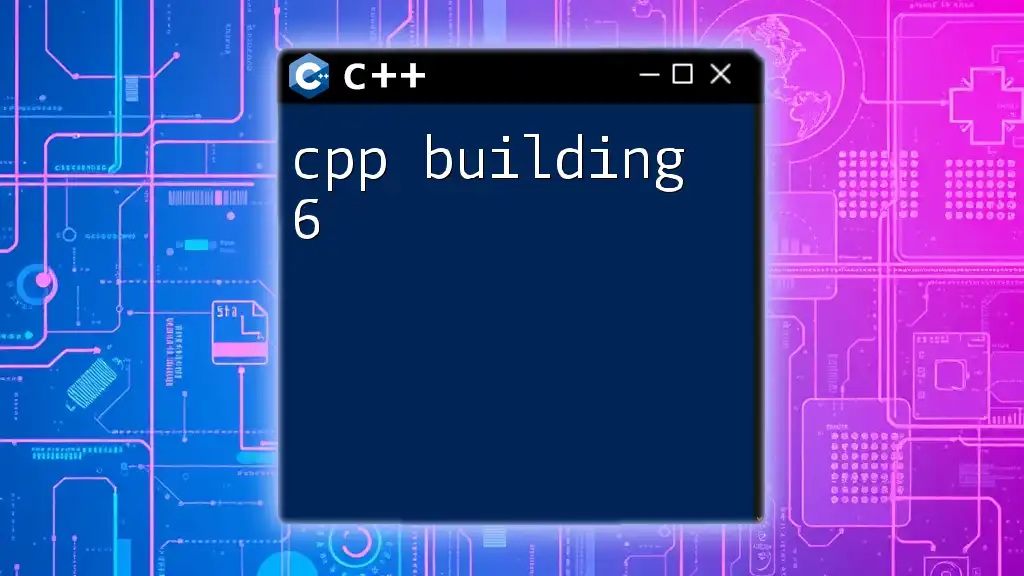
Join Our Community
Subscribe to our blog for more quick C++ tips and guidance on mastering programming concepts like Euclid's Algorithm. Practice regularly, share your experiences, and engage with peers to elevate your skills!