The `std::cin` object in C++ is used to read input from the standard input stream (usually the keyboard), allowing users to interactively enter data into a program.
Here's a simple code snippet demonstrating how to read an integer from user input:
#include <iostream>
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "You entered: " << number << std::endl;
return 0;
}
Understanding Input in C++
In programming, input refers to any data received by a program from an external source, such as user interactions or files. In C++, understanding reading input is crucial for developing interactive applications. The ability to read input allows a program to accept data, manipulate it, and produce meaningful results, making it a foundational skill for any C++ developer.
Input Streams in C++
An input stream is a sequence of data elements made available for processing. In C++, the standard input stream is represented by `std::cin`. This stream allows developers to read data entered by users directly from the console.
- Standard Input Stream (`std::cin`):
- `std::cin` is commonly used to capture user input. It plays a central role in C++ applications that interact with users.
- The basic syntax to read from `std::cin` is straightforward, allowing developers to directly capture variables.

Reading Input Using `std::cin`
One of the simplest ways to read input in C++ is by using `std::cin`. The operator `>>` can be used to read different types of data directly from the console.
Basic Reading of Variables
Reading basic types such as integers and strings is intuitive with `std::cin`. Here’s a simple code example that demonstrates how to read an integer and a string from the user:
#include <iostream>
#include <string>
int main() {
int number;
std::string name;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "Enter your name: ";
std::cin >> name;
std::cout << "You entered: " << number << " and " << name << std::endl;
return 0;
}
In this example, the program prompts the user to enter a number and a name. It then displays the entered values, showcasing the simple yet effective way to gather input.
Reading Different Data Types
C++ allows reading various data types, including characters, integers, and floating-point numbers.
Keyboard Input:
- To read a character:
char character;
std::cin >> character; // Reading a character
- And for a floating-point number:
float pi;
std::cin >> pi; // Reading a floating-point number
This flexibility makes C++ suitable for a wide range of applications.

Handling String Input
To read strings that may contain spaces, such as full names or phrases, utilize the `std::getline()` function. This method captures everything up to the newline character, which allows for better handling of string types.
Using `std::getline`
Understanding when to use `std::getline()` instead of `std::cin` is critical. While `std::cin` stops reading at whitespace, `std::getline()` continues until it reaches the end of the line.
Here's an example that shows how to read an entire line of text:
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter a line of text: ";
std::getline(std::cin, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
By using `std::getline()`, the program accurately captures input that might contain multiple words.

Input Validation
Validating user input is essential to ensure that the data being processed meets the expected format. Users may enter invalid or unexpected data, leading to errors or crashes in the program.
Basic Techniques for Input Validation
Implementing input validation can be achieved through:
- Data Type Checks: Confirming that the entered data matches the intended type.
- Using Loops for Retries: Re-prompting users until valid input is received.
Here’s a code example demonstrating how to validate integer input:
#include <iostream>
#include <limits>
int main() {
int number;
while (true) {
std::cout << "Enter a valid number: ";
if (std::cin >> number) {
break; // Valid input
} else {
std::cout << "Invalid input, try again." << std::endl;
std::cin.clear(); // Clear error state
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Ignore unwanted input
}
}
std::cout << "You entered: " << number << std::endl;
return 0;
}
In this scenario, the program continually prompts the user until a valid input is provided, enhancing user experience and robustness.
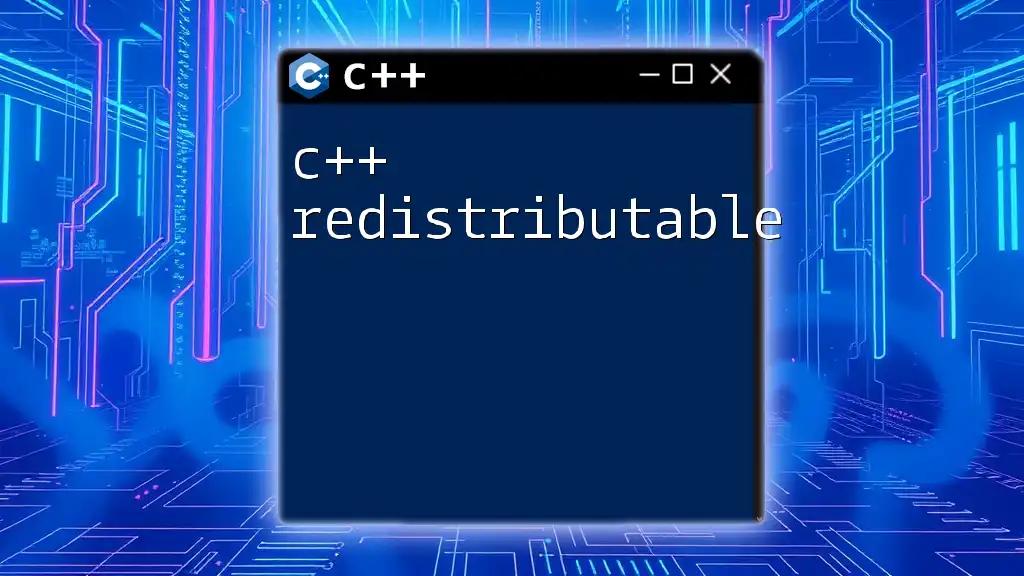
Advanced Reading Techniques
C++ offers advanced options for reading input data, including reading from files—an essential capability for many applications.
Reading from Files
Reading from a file allows programs to process large amounts of data stored externally. Here’s a simple code example illustrating how to read lines from a file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream infile("data.txt");
std::string line;
if (infile.is_open()) {
while (std::getline(infile, line)) {
std::cout << line << std::endl; // Output each line
}
infile.close();
}
return 0;
}
This code snippet opens a file called `data.txt`, reads each line, and outputs it to the console. It demonstrates how C++ can easily handle external data sources.
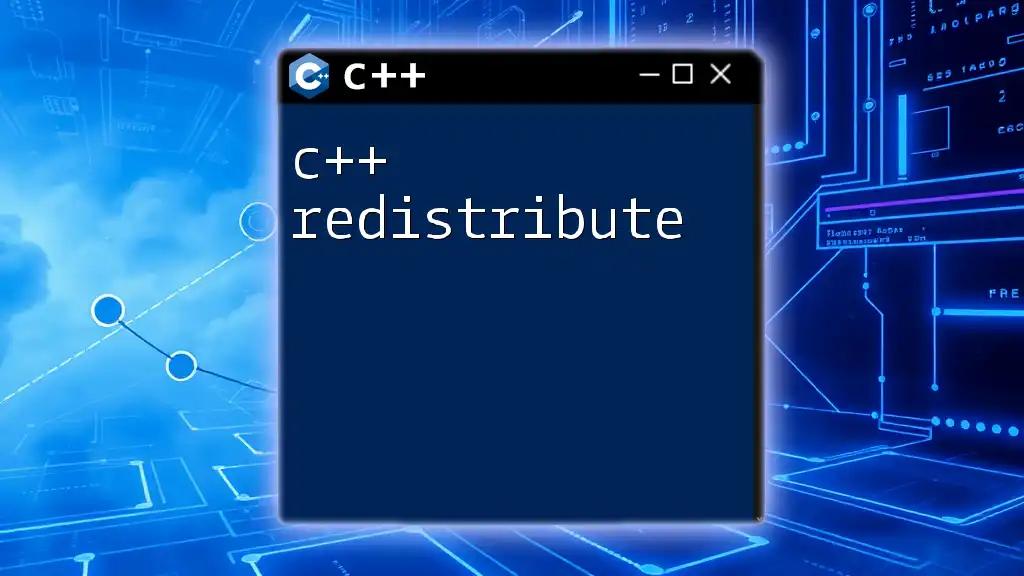
Common Pitfalls in Reading Input
While working with input, several common mistakes can occur.
- Infinite Loops: Forgetting to reset the error state can lead to infinite loops, as shown in the previous validation example.
- Mixed data types can cause unexpected behavior. For instance, attempting to read a string into an integer variable will fail.
- Using `std::cin` for string input where spaces are involved can result in only capturing the first word, leading to potential data loss.
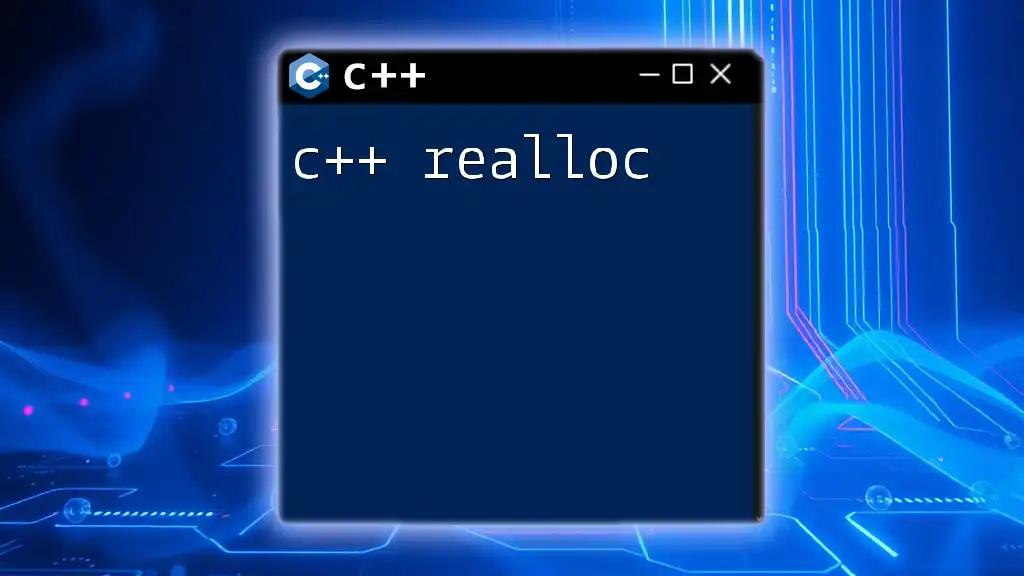
Best Practices for Input Handling
To improve the reliability and quality of user input handling in C++, consider the following best practices:
- Always validate input to ensure it’s in the expected format.
- Use appropriate data types based on the expected input. For example, use a string for text and integers for numeric values.
- Handle potential exceptions and errors gracefully to provide informative feedback to users.

Conclusion
Throughout this guide, we explored the crucial topic of `c++ read`, covering various techniques for accepting and processing user input. Whether using `std::cin` for standard input or `std::getline()` for capturing full lines, mastering these methods is essential for developing robust C++ applications. With a strong foundation in input handling, developers can create interactive programs that respond effectively to user data. Practice these concepts, and enhance your C++ skills further!
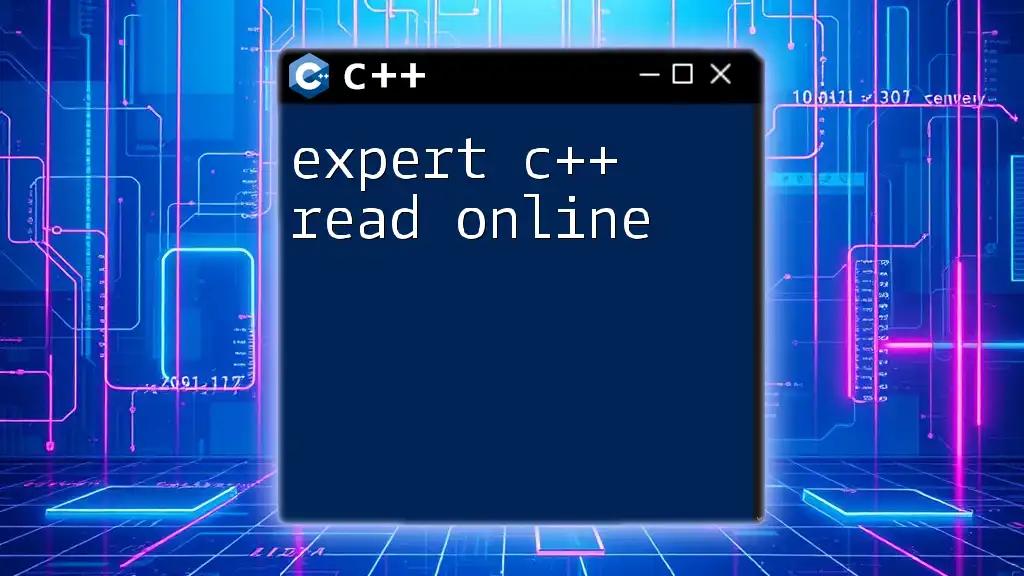
Additional Resources
For those eager to deepen their knowledge, consider exploring recommended books, online courses, and documentation on modern C++. Engage with community forums and C++ support groups to share ideas and seek further guidance, as collaboration is key in mastering programming.