The C++ `std::reduce` algorithm from the Standard Library efficiently accumulates values in a range using a specified binary operation, often utilized for summing elements in a container.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
#include <vector>
#include <numeric> // for std::reduce
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int sum = std::reduce(numbers.begin(), numbers.end(), 0);
std::cout << "Sum: " << sum << std::endl; // Outputs: Sum: 15
return 0;
}
Understanding the C++ `reduce` Algorithm
What is the `reduce` Algorithm?
The `reduce` algorithm is a powerful feature in C++ that allows programmers to condense a sequence of values down to a single value using a specified binary operation. Originating from functional programming, reduce provides a clean and concise way to implement operations such as sums, products, or any aggregation of values.
Why Use `reduce`?
The main advantage of using `reduce` is its simplicity. Instead of iterating through an entire dataset manually, you can achieve the same effect with just a single line of code, enhancing both readability and maintainability. This method improves code efficiency, since the underlying implementation is optimized for performance.
Moreover, `reduce` shines in multi-threaded environments, allowing for parallel execution that can drastically improve performance in large data processing tasks. Its use extends to practical applications such as data analytics, numerical computations, and even in machine learning frameworks.

The Basics of C++ Standard Template Library (STL)
Overview of the C++ STL
The C++ Standard Template Library (STL) is a powerful collection of template classes and functions that provide standardized algorithms and data structures. The components of STL include containers, which store data, algorithms for processing data, and iterators, which act as pointers to traverse these data structures.
Introduction to the `<numeric>` Header
The `<numeric>` library is a crucial part of STL that specializes in numeric operations. When you want to perform calculations such as summing values, calculating inner products, or applying the `reduce` algorithm, this library is your go-to resource. It includes various functions, such as `accumulate` and `inner_product`, but in this context, we will focus on the `reduce` function.
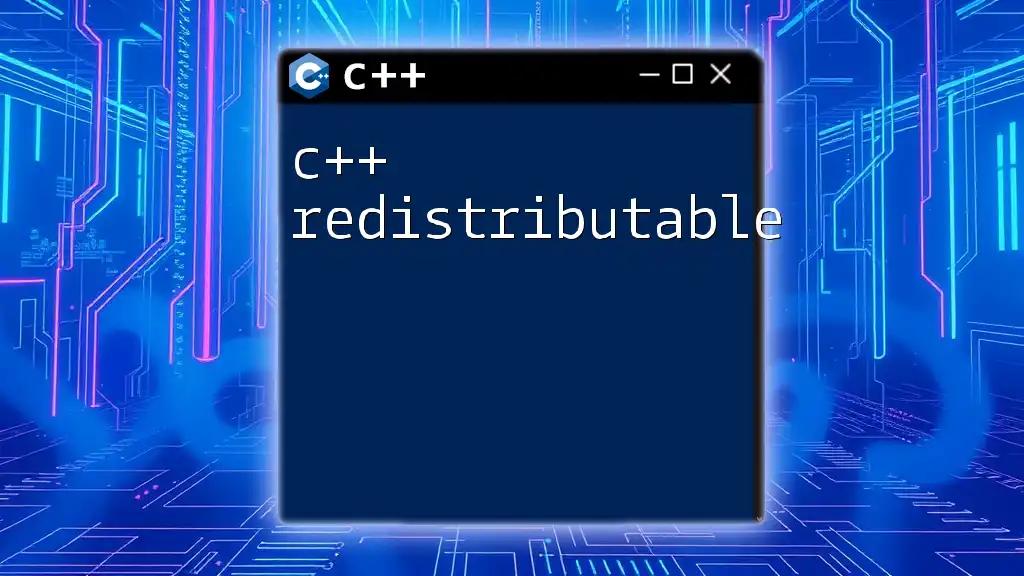
Implementing Reduce in C++
Syntax of the `reduce` Function
The syntax of the `reduce` function is designed to be straightforward. It typically takes the following form:
std::reduce(first, last, init, binary_op);
Where:
- `first` and `last` are iterators pointing to the beginning and end of the range you wish to reduce.
- `init` is the initial value for the aggregation.
- `binary_op` is a binary operation that defines how to combine elements, such as addition or multiplication.
Code Snippet: Using `std::reduce`
Here is a simple example of using `std::reduce` to sum up elements in a vector:
#include <iostream>
#include <vector>
#include <numeric> // for std::reduce
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int result = std::reduce(numbers.begin(), numbers.end(), 0);
std::cout << "Sum: " << result << std::endl;
return 0;
}
In this snippet, we declare a vector of integers, then use `std::reduce` to compute the sum of its elements, starting from an initial value of `0`. The expected output will be `Sum: 15`. This simple yet potent functionality encapsulates how `reduce` can simplify your C++ code.
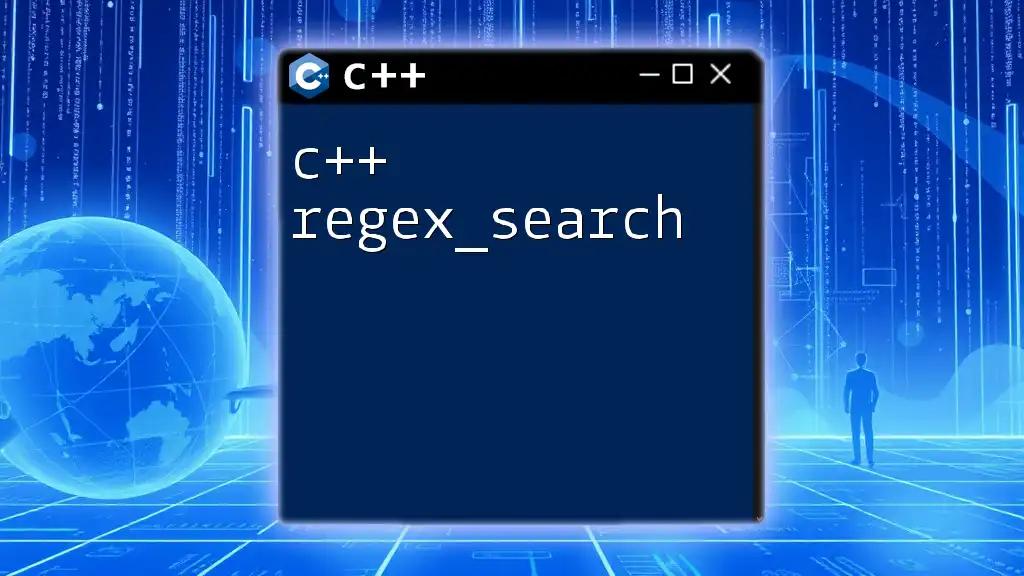
Exploring Execution Policies
Introduction to Execution Policies
Execution policies in C++ offer a way to specify how operations should be executed: sequentially or concurrently. The standard policies include sequential, parallel, and vectorized execution. These policies enable programmers to write performance-oriented code without delving into the details of threading.
Example: Using `reduce` with Parallel Execution
When you're dealing with larger datasets, using parallel execution can significantly reduce processing time. Here’s how you can implement `std::reduce` with a parallel execution policy:
#include <iostream>
#include <vector>
#include <numeric>
#include <execution> // for execution policies
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int result = std::reduce(std::execution::par, numbers.begin(), numbers.end(), 0);
std::cout << "Sum (Parallel): " << result << std::endl;
return 0;
}
In this example, `std::execution::par` tells the compiler to execute the operation in parallel, allowing for quicker computations on larger datasets. The output will still be `Sum (Parallel): 15`, but the time taken to compute this summary can be dramatically less compared to a sequential approach.
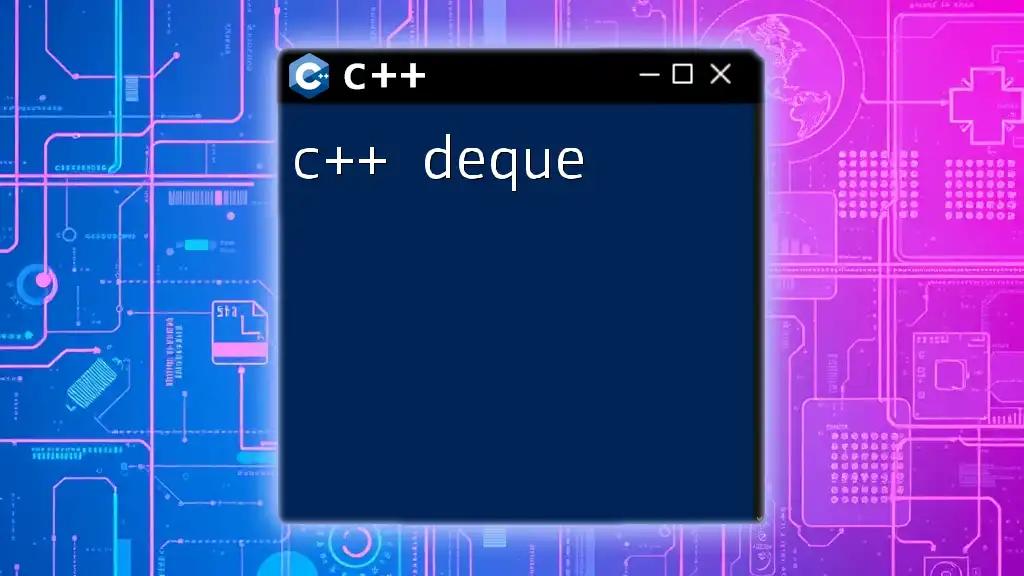
Custom Reductions: Using Custom Binary Operators
What is a Binary Operation?
A binary operation takes two inputs and combines them into a single output. Common binary operations include addition, multiplication, and more complex operations as defined by the programmer.
Creating a Custom Binary Operation
You can also define custom binary operations by creating a struct or class. Below is an example of a custom binary operator that adds integers while also incrementing the result by `1`:
#include <iostream>
#include <vector>
#include <numeric>
struct CustomSum {
int operator()(int a, int b) const {
return a + b + 1; // Custom logic
}
};
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int result = std::reduce(numbers.begin(), numbers.end(), 0, CustomSum());
std::cout << "Custom Sum: " << result << std::endl;
return 0;
}
Here, the custom operator defines an addition that includes an additional increment. The expected output will be `Custom Sum: 21`, which reflects how the custom operation affects the aggregate result.

Common Pitfalls When Using `reduce`
Issues with Range and Initial Values
One common pitfall is mistakenly choosing the wrong range or initial values. If the starting iterator is beyond the last iterator, or if the initial value doesn’t match the expected data type, this can lead to runtime errors or incorrect results. Therefore, always double-check the ranges you provide and choose an appropriate initial value, especially in situations with mixed data types.
Thread Safety Considerations
When utilizing the `reduce` function in multi-threaded environments, caution is necessary. Not all binary operations are thread-safe. It’s essential to ensure that operations don’t modify shared data between threads without proper synchronization mechanisms like mutexes or atomic operations.
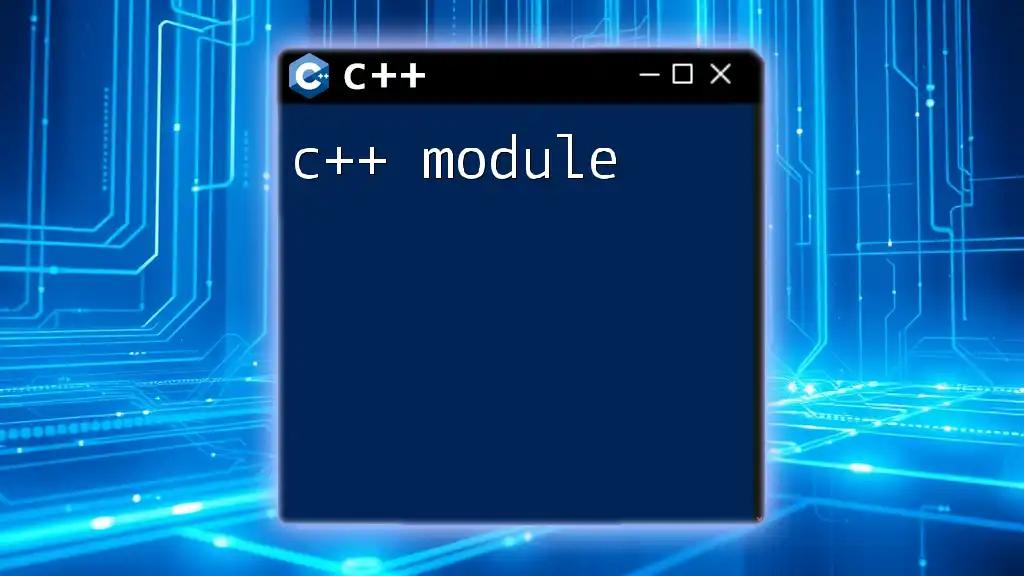
Best Practices for Using `reduce` in C++
When to Use `reduce`
`reduce` is most effective in scenarios where large datasets require accumulation or aggregation. If your dataset is small and performance isn’t critical, traditional loops may suffice, but for larger datasets, employing `reduce` can lead to both cleaner and faster code.
Code Readability and Maintainability
While `reduce` simplifies your code, it’s important to maintain clarity. Comment on complex operations clearly and choose descriptive names for custom operators. Well-commented and structured code not only helps you but also aids other developers who may work on your code in the future.
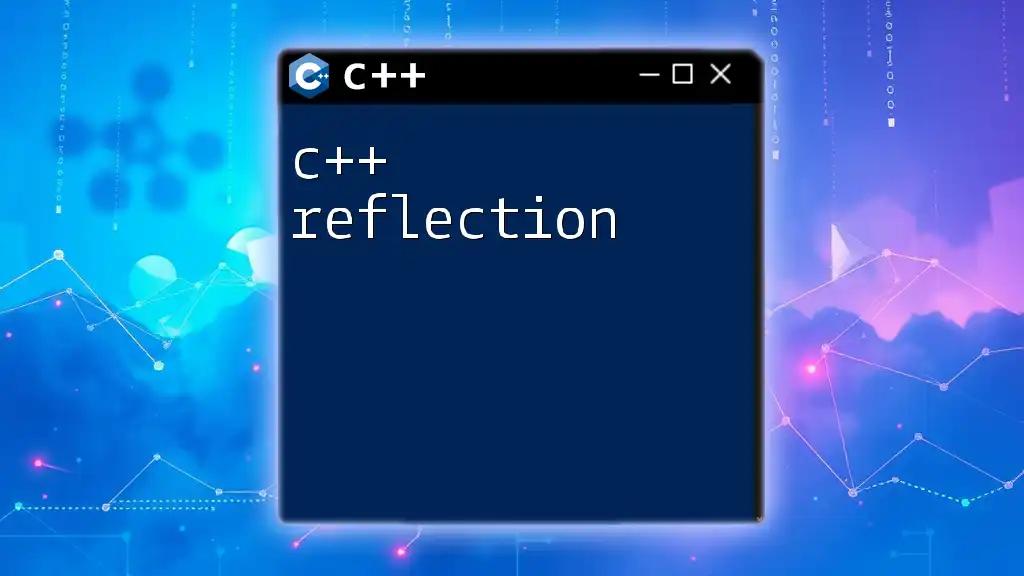
Conclusion
In summary, understanding the C++ `reduce` function can greatly enhance your ability to manipulate data efficiently and concisely. The blend of functional programming principles and the power of C++ STL creates an opportunity for coding with elegance and effectiveness. Whether you are summing numbers, performing parallel computations, or crafting your own binary operations, employing `reduce` will elevate your programming skill set.
Encouragement for Practical Application
Don’t hesitate to implement and experiment with `reduce` in your projects. The best way to master this powerful feature is through practice, and exploring various applications will deepen your understanding. Remember, great coding practices come from continued learning and hands-on experience.
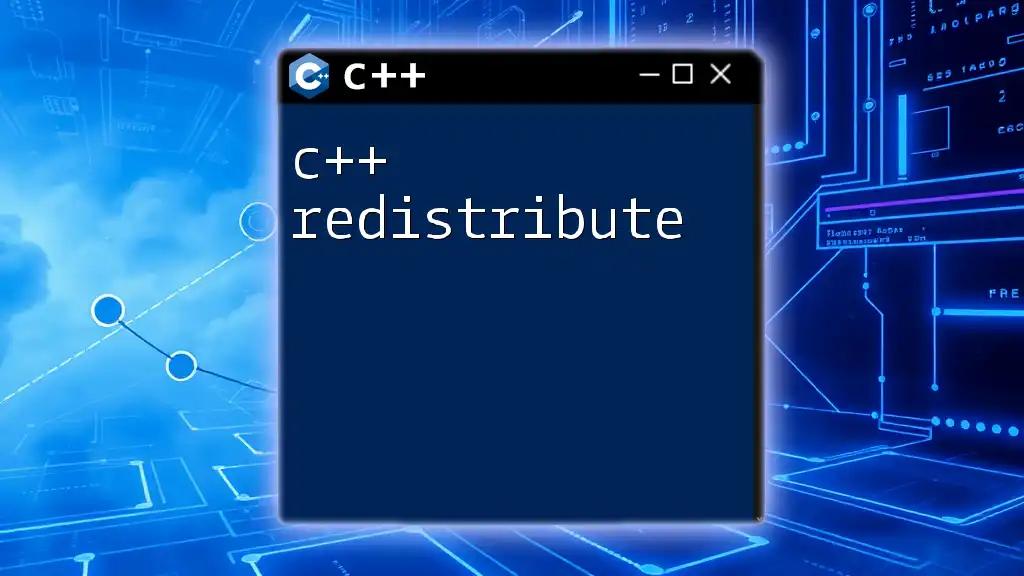
Additional Resources
For those eager to expand their knowledge, consider consulting specialized books on C++ or engaging with online courses that focus on modern C++ practices. Moreover, joining programming communities and forums can provide valuable insights and allow you to share your experiences with fellow enthusiasts.