C++ reserved words, also known as keywords, are predefined terms that have a special meaning in the C++ language and are not allowed to be used as identifiers (such as variable or function names).
int main() {
int reservedKeyword = 5; // 'int' and 'return' are reserved words in C++
return 0; // 'return' is also a reserved word
}
What are Reserved Words?
Definition
In programming languages, reserved words (or keywords) are predefined words that hold special meaning. In C++, these words are integral to the syntax and structure of the language, and they cannot be used as identifiers (e.g., variable names, function names). Understanding reserved words is crucial for any developer, as they dictate how the compiler interprets the code.
Characteristics of Reserved Words
Reserved words have distinct characteristics that set them apart from regular identifiers:
- Fixed Meaning: Each reserved word has a specific purpose, and its meaning is defined by the C++ language standard.
- Cannot Be Redeclared: These words cannot be assigned new meanings or used as identifiers. Attempting to do so will lead to compilation errors.
- Context Dependency: Many reserved words are context-dependent, meaning their relevance and application can change based on where they are used in the code.
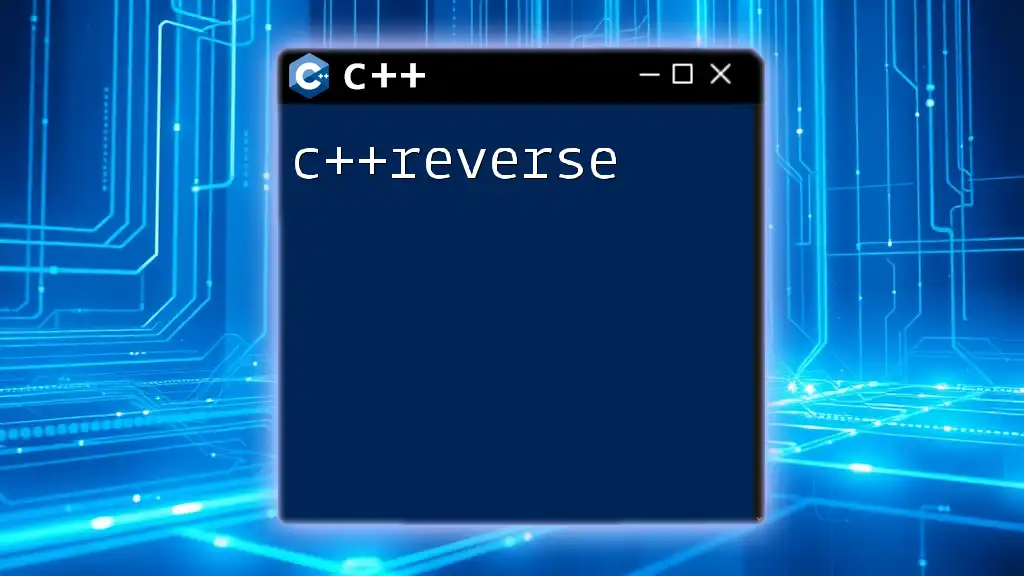
List of C++ Reserved Words
Fundamental Keywords
auto
The `auto` keyword automatically deduces the type of a variable from its initializer. It improves code flexibility and readability.
auto x = 5; // x is of type int
break
Used within loops and switch statements, `break` exits the current loop or switch-case block. This is especially useful for conditionally terminating a loop.
for(int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exits the loop when i equals 5
}
}
case
Within a `switch` statement, `case` defines individual branches. Each `case` represents a constant, and the `switch` checks its variable against them.
switch(day) {
case 1:
cout << "Monday";
break;
case 2:
cout << "Tuesday";
break;
}
Data Type Keywords
bool
The `bool` keyword represents Boolean values, either true or false. It is fundamental in control structures and logical operations.
bool isActive = true;
char
The `char` keyword designates single character types and is often used for storing characters.
char letter = 'A';
int
The `int` keyword is one of the primary data types in C++, used to represent integer values.
int count = 10;
Control Flow Keywords
if
This keyword initiates a conditional statement, where the block of code executes if the condition evaluates to true.
if (x > 5) {
cout << "x is greater than 5";
}
for
The `for` keyword is used to create a loop that executes a block of code a predetermined number of times, based on initialization, condition, and increment/decrement.
for (int i = 0; i < 10; i++) {
cout << i; // Prints numbers 0 to 9
}
while
The `while` keyword starts a loop that continues as long as the specified condition remains true.
while (x < 10) {
x++; // Increment x until it reaches 10
}
Class and Object Keywords
class
With `class`, users can define custom data types in C++. It encapsulates data and functions together, forming the basis of object-oriented programming.
class Animal {
public:
void sound() {
cout << "Generic animal sound";
}
};
public, private, protected
These keywords are access specifiers in C++, determining the visibility of class members.
- public allows access from outside the class.
- private restricts access to within the class.
- protected permits access to derived classes.
class Car {
private:
int speed; // can't be accessed outside Car
public:
void setSpeed(int s) {
speed = s;
}
};
Miscellaneous Keywords
const
The `const` keyword marks variables as constants, which means their values cannot change once initialized. This is useful for maintaining the integrity of data.
const int MAX_VALUE = 100;
nullptr
Introduced in C++11, `nullptr` is a special pointer that signifies a null pointer, providing type safety over the older `NULL`.
int* p = nullptr; // pointer p is initialized to a null pointer
virtual
The `virtual` keyword is essential in implementing polymorphism in object-oriented programming. It allows derived classes to override methods defined in base classes.
class Base {
public:
virtual void show() {
cout << "Base class show";
}
};
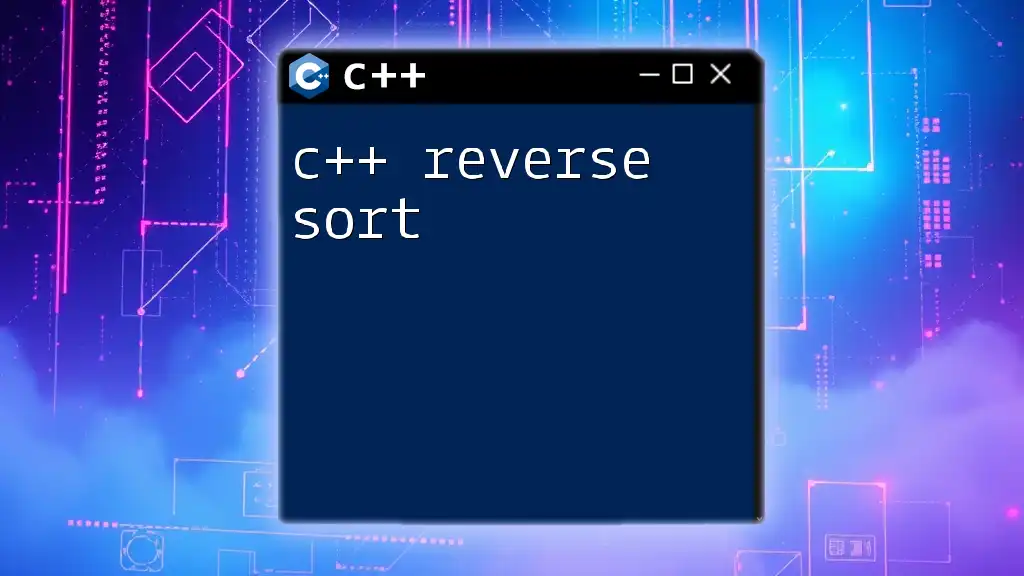
Common Errors Due to Reserved Words
Naming Conflicts
Using reserved words as identifiers can lead to naming conflicts, resulting in compiler errors. For example, declaring a variable named `int` will result in an error because it conflicts with the reserved word.
int int = 5; // Compiler error: 'int' is a reserved keyword
Best Practices to Avoid Errors
To minimize issues with reserved words:
- Use Descriptive Names: Avoid common names that closely resemble reserved words.
- Follow Naming Conventions: Use consistent naming strategies, such as prefixes or suffixes, to prevent unintentional conflicts.
- Utilize IDE Features: Many Integrated Development Environments (IDEs) highlight reserved words. Use this feature to be mindful of your naming choices.
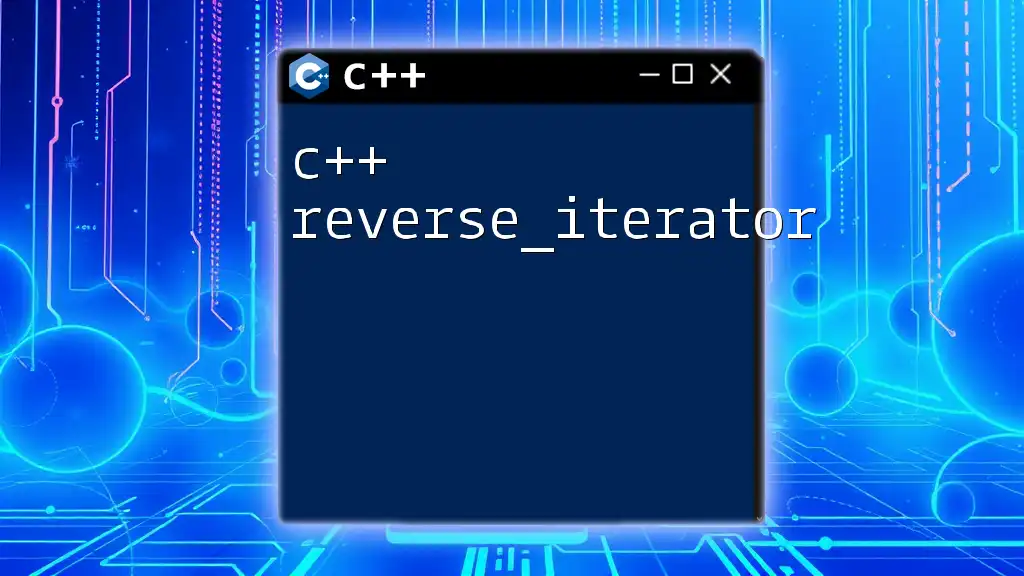
Conclusion
Understanding C++ reserved words is vital as they are fundamental components of the language. By recognizing their specific functions and places within code, you can avoid errors and write more efficient, professional code. Practice implementing these reserved words through exercises or projects to solidify your understanding.
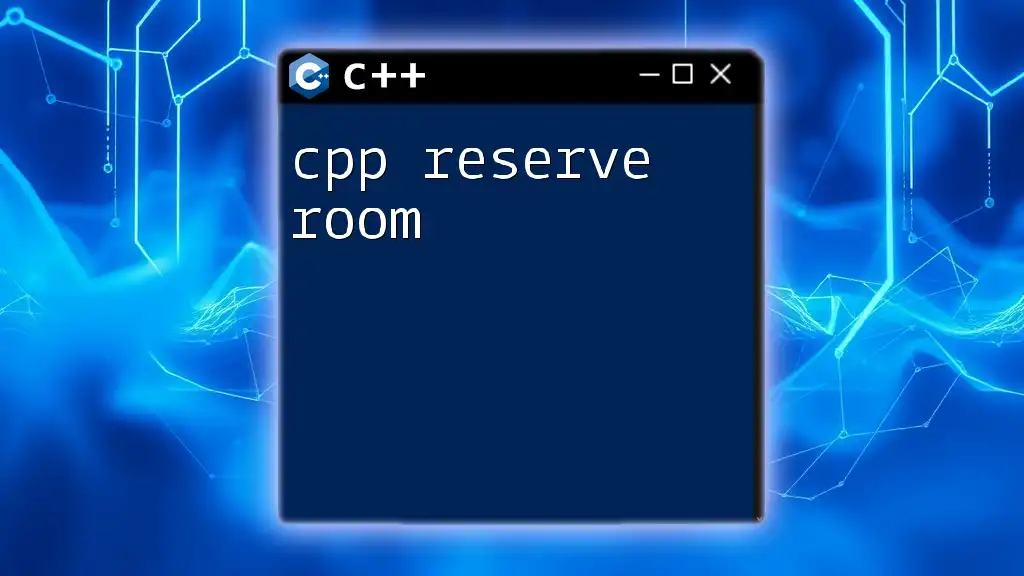
Additional Resources
For those wishing to explore further, consider the following:
- Recommended books on C++ programming
- Online learning platforms featuring comprehensive C++ courses
- Coding platforms that offer hands-on practice focusing on C++ reserved words and syntax