In C++, a lambda can capture the `this` pointer to access members of the enclosing class, enabling the use of instance variables and methods within the lambda expression.
Here's an example:
class MyClass {
public:
int value = 42;
void useLambda() {
auto lambda = [this] { return value; }; // Capturing 'this'
std::cout << lambda() << std::endl; // Outputs 42
}
};
Understanding C++ Lambdas
What are Lambdas in C++?
C++ lambdas are a powerful feature that allows for the creation of anonymous functions. Introduced in C++11, they enable you to write concise and more readable code by allowing the definition of functions at the point of usage. This helps especially when you need a function for a short time and do not want to clutter your code with named functions.
The advantages of lambdas include:
- Inline function definition: You can define functions directly at the call site, avoiding the need for separate function declarations.
- Enhanced readability: By keeping related logic together, your code can be both shorter and clearer.
- Flexibility: They allow for easy use in standard algorithms and event handling.
Syntax of C++ Lambdas
Understanding the syntax of a C++ lambda is crucial. A lambda consists of several parts:
- Capture Clause: This defines which variables from the surrounding scope will be accessible inside the lambda.
- Parameter List: Just like a regular function, this defines the parameters that the lambda can take.
- Return Type: Optionally, you can specify the return type of the lambda.
- Lambda Body: This is where the actual code to be executed resides.
Here’s a simple example of a lambda that prints "Hello, World!":
auto myLambda = []() { std::cout << "Hello, World!"; };
myLambda(); // Outputs: Hello, World!
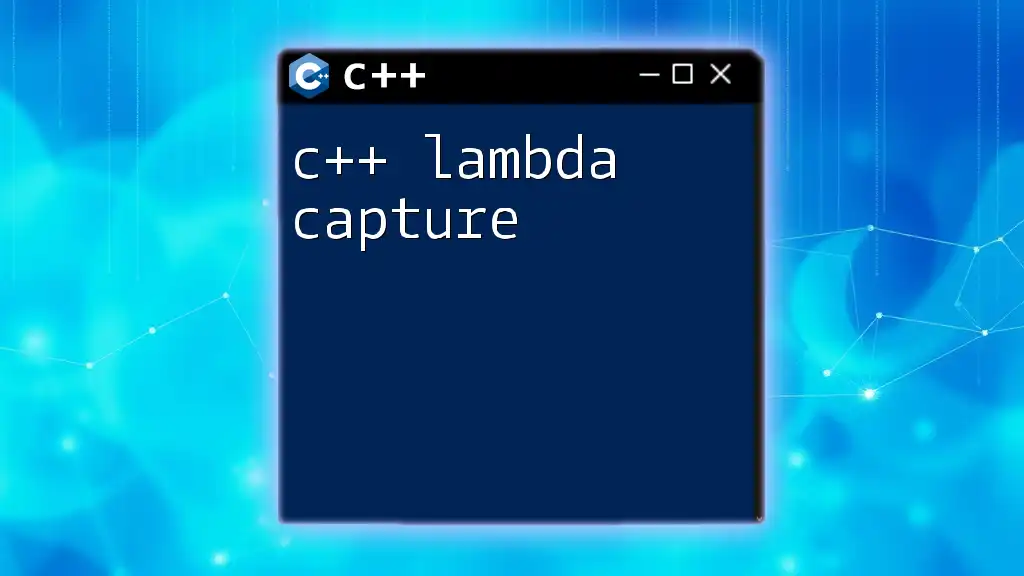
The Capture Clause in C++ Lambdas
Introduction to Capture Clause
The capture clause of a lambda is critical because it allows the lambda to access variables from the enclosing scope. This provides significant flexibility, as the lambda can use local variables without needing to pass them explicitly as parameters.
Types of Capture
There are two primary types of capture in C++ lambdas:
Value Capture
Value capture means that a copy of the variable is made, so any changes inside the lambda do not affect the original variable. For instance:
int x = 10;
auto lambdaValue = [x]() { return x + 5; };
std::cout << lambdaValue(); // Outputs: 15
In this example, `x` is captured by value, so the lambda returns `15`, but the original `x` remains unchanged.
Reference Capture
Reference capture allows the lambda to refer directly to the variable in the outer scope, meaning that any changes made inside the lambda affect the original variable. Here’s an example:
int y = 10;
auto lambdaRef = [&y]() { y += 5; };
lambdaRef();
std::cout << y; // Outputs: 15
In this case, `y` is captured by reference; thus, after the lambda executes, `y` is incremented to `15`.
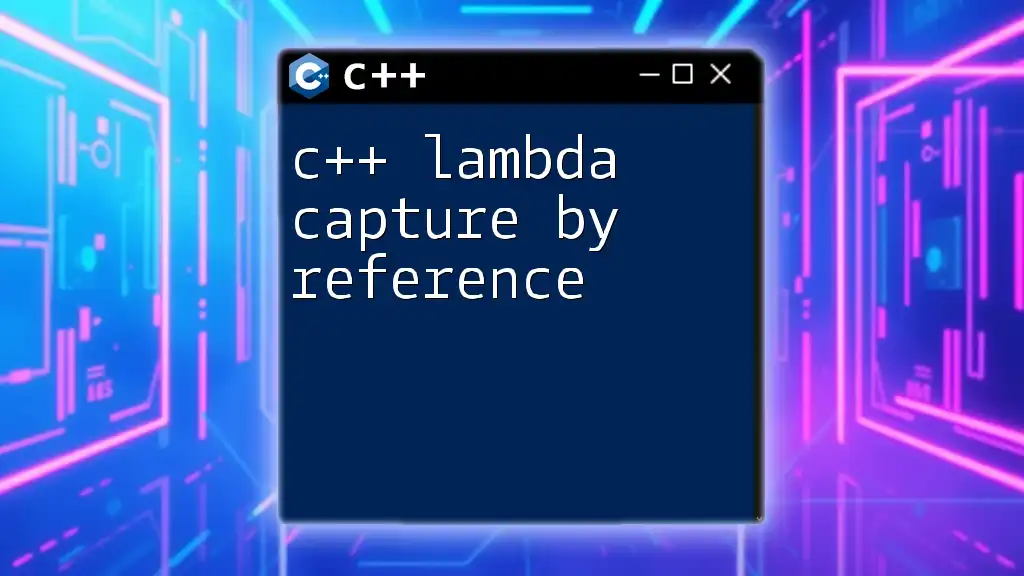
The 'this' Pointer in Lambda Capture
What is the 'this' Pointer?
In C++, the `this` pointer is an implicit pointer available within member functions of a class. It allows access to the class’s members (variables and methods) from within the class's member function.
Capturing 'this' in Lambdas
Capturing `this` in a lambda is especially useful within classes. It enables the lambda to access member variables and functions directly, simplifying the code and maintaining context.
Example of Capturing 'this'
Consider the following class definition that demonstrates capturing `this` in a lambda:
class MyClass {
public:
int value;
MyClass(int val) : value(val) {}
void demonstrateLambda() {
auto lambdaThis = [this]() { return this->value + 10; };
std::cout << lambdaThis(); // Outputs: value + 10
}
};
In this example, the lambda captures `this` to access the `value` member. When `demonstrateLambda` is called, it executes the lambda and outputs the result by accessing the member variable directly.
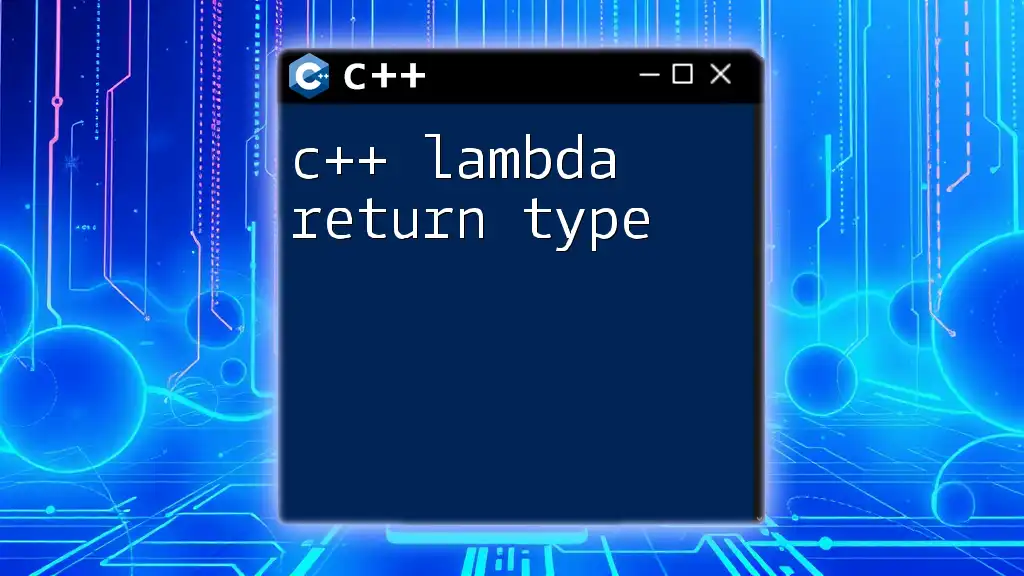
Practical Use Cases for 'this' Capture in C++
Class Member Functions and Lambdas
Capturing `this` in lambdas becomes critical when designing complex member functions where you want to perform operations on class members. It allows for clear, concise, and maintainable code.
Event Handling
Lambda functions are particularly useful for event handling in GUI programming. You can easily connect user actions to methods within a class using a lambda that captures `this`. Here’s how this can be accomplished:
connect(button, &QPushButton::clicked, [this]() {
std::cout << "Button clicked! Value: " << this->value;
});
In this snippet, when the button is clicked, the lambda runs, using the class's `value`, demonstrating how encapsulating functionality can lead to cleaner and more efficient code execution.
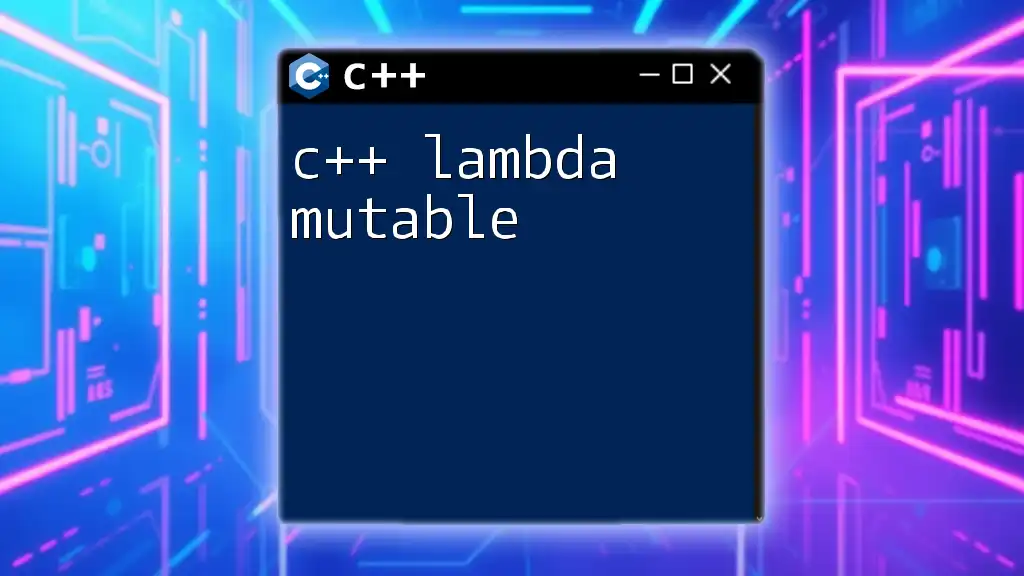
Best Practices When Using C++ Lambda with 'this'
Scope Considerations
When capturing `this`, it's crucial to be aware of scope. If the lambda outlives the `this` object it captures, such as when stored in a future or passing to another thread, it leads to undefined behavior. To avoid such pitfalls, ensure that the lifetime of `this` exceeds that of the lambda usage.
Readability and Maintainability
While using lambdas can enhance readability by reducing boilerplate code, overusing them can lead to decreased clarity. It’s important to keep lambdas concise and to the point. If a lambda is particularly large or complex, consider extracting it into a named function to maintain code readability.
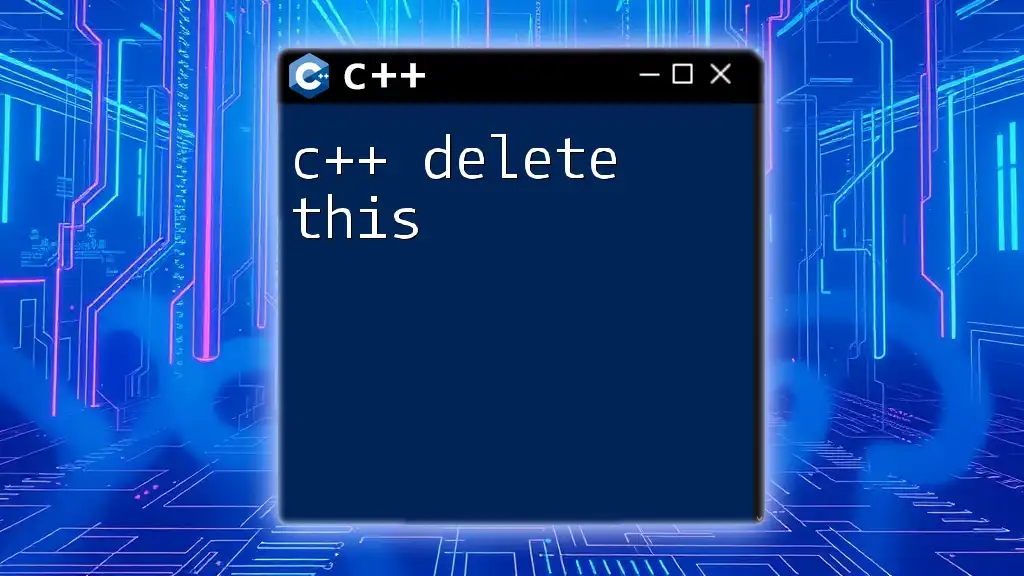
Conclusion
C++ lambdas, especially with the `this` capture, offer a powerful tool for writing functional-style code within object-oriented paradigms. By understanding how to capture variables effectively, including `this`, you can leverage lambdas to write more maintainable and clear code. Practicing these concepts will enhance your coding skills, making you a more efficient C++ programmer.