In C++, the `delete this` expression is used within a class's member function to deallocate the memory of the object's instance, effectively allowing the object to self-destruct.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
class MyClass {
public:
void destroy() {
std::cout << "Object is being destroyed." << std::endl;
delete this; // Deallocate memory for this object
}
};
int main() {
MyClass* obj = new MyClass();
obj->destroy(); // Calls destroy and deletes the object
return 0;
}
Understanding `this` Pointer
What is the `this` Pointer?
In C++, the `this` pointer is a special pointer available within class member functions. It points to the instance of the object that is currently being operated on. Every non-static member function of a class receives an implicit `this` pointer, allowing access to the object's members.
For instance, when you access a member variable in a member function, the compiler translates that to an access through the `this` pointer. This is crucial in situations where member variables have names that are shadowed by parameters or local variables.
The Role of `this` in Object Context
The `this` pointer plays a critical role in understanding object context. Within a member function, `this` provides clarity, ensuring that when you refer to a member variable, you're indeed referring to the instance of the class, not any other variables that might have the same name.
For example:
class Sample {
public:
int value;
Sample(int value) {
this->value = value; // 'this' disambiguates the member from the parameter
}
};
Here, the `this` keyword allows the constructor to distinguish between the member variable and the parameter.
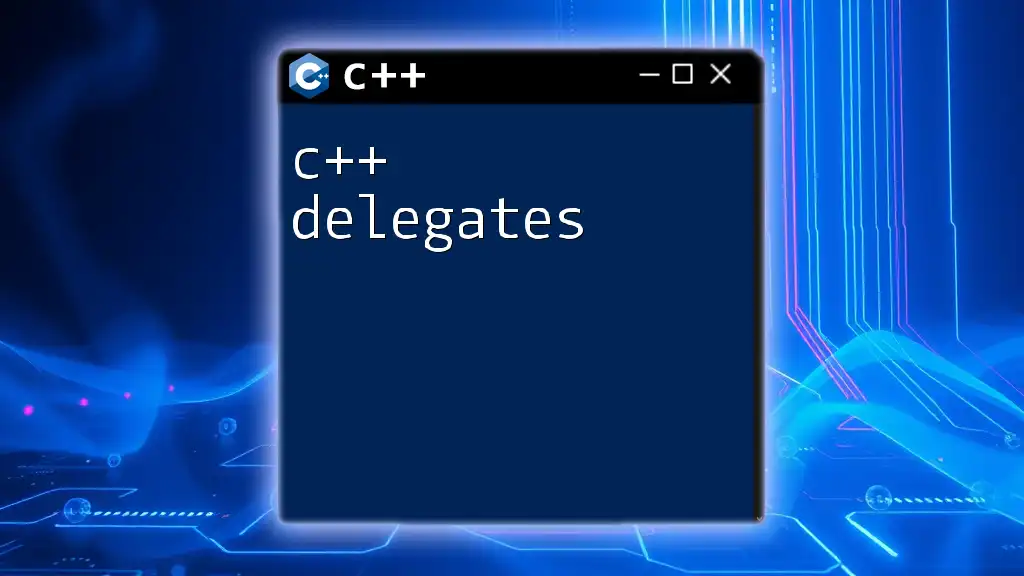
The Concept of Object Destruction
What Happens During Object Destruction?
Object destruction is a vital aspect of memory management in C++. Destructors are special member functions invoked when an object goes out of scope or is explicitly deleted. The destructor is responsible for releasing resources acquired by the object, such as memory or file handles.
Why Use `delete`?
In C++, dynamic memory allocation is handled using pointers. The `delete` operator is used to free memory that was previously allocated with `new`. This prevents memory leaks by ensuring that once an object is no longer needed, the memory it occupies is returned to the system.
It’s essential to differentiate between `delete` and `delete[]`. The former is used for single objects, while `delete[]` is meant for arrays:
int* arr = new int[10]; // allocation for array
delete[] arr; // proper way to delete the array
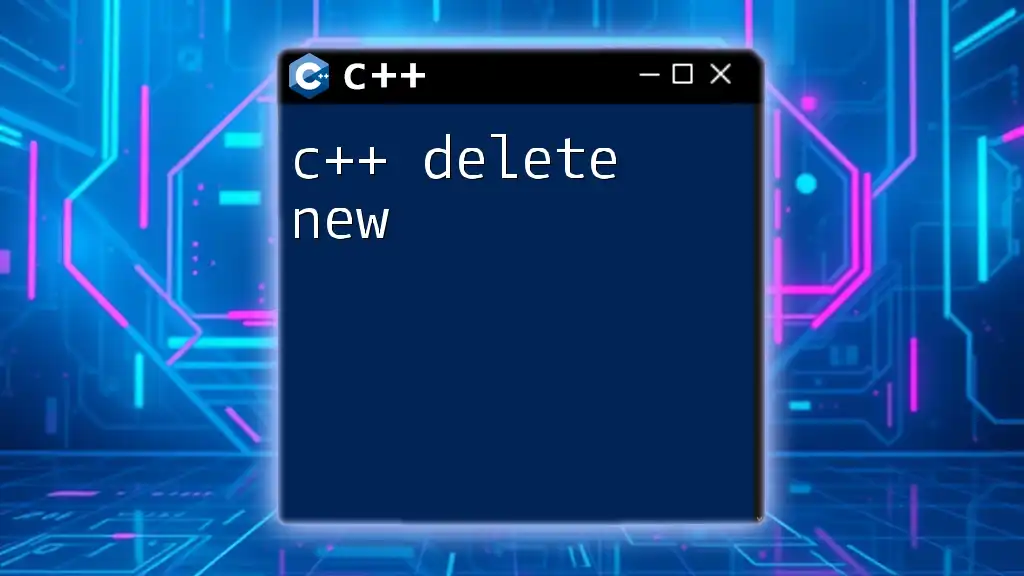
The `delete this` Command
What Does `delete this` Mean?
The `delete this` command allows an object to delete itself, effectively releasing its memory when it is no longer needed. This is typically used in dynamic polymorphic classes where the ownership of the object can be ambiguous. By calling `delete this`, you're explicitly indicating that the current object is responsible for its own deletion.
Use Cases of `delete this`
A common use case for `delete this` is within a class where an object might determine during its execution that it should destroy itself. For example, within a method, you could check certain conditions before calling `delete this`:
class Example {
public:
void doSomething() {
if (someCondition()) {
delete this; // safely deleting itself based on a condition
}
}
};
In this case, the object will be deleted only if `someCondition()` returns `true`.
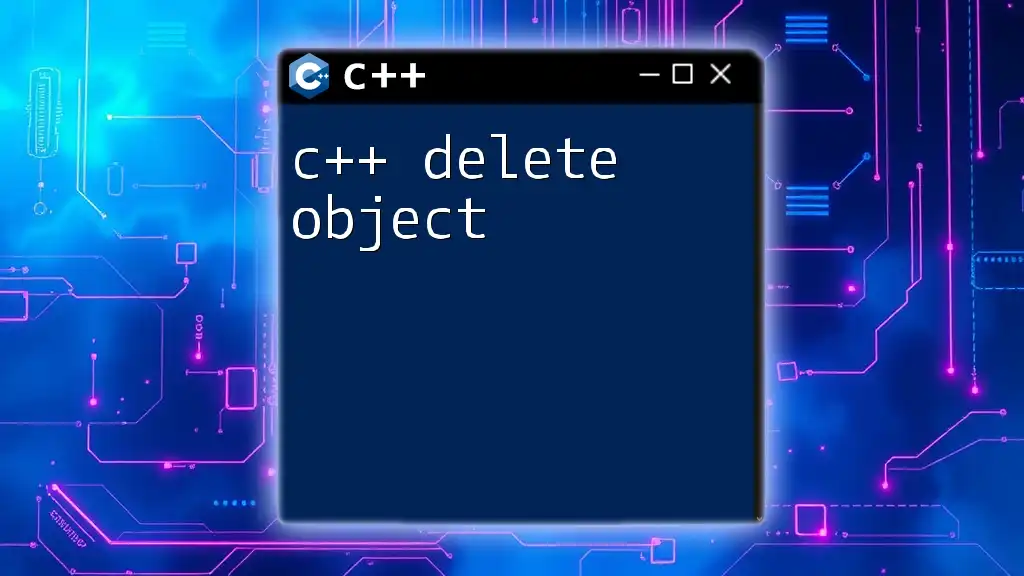
Risks and Drawbacks of `delete this`
Undefined Behavior Risks
While `delete this` can be useful, it carries significant risks, particularly concerning undefined behavior. After an object is deleted using `delete this`, any further attempt to access the object's members or methods leads to undefined behavior, which can lead to crashes or memory corruption.
One must ensure that no other references to the object exist after it has been marked for deletion. For example:
class Dangerous {
public:
void riskyFunction() {
delete this;
// Any access here, such as more function calls or member access, is undefined.
}
};
Ownership and Memory Management
Understanding ownership is critical in C++. If multiple pointers reference the same object, using `delete this` can lead to double deletion, which is when an object is attempted to be deleted more than once. This can have catastrophic results. It’s essential to structure classes and their relationships such that it's clear whether an object is responsible for its own deletion.
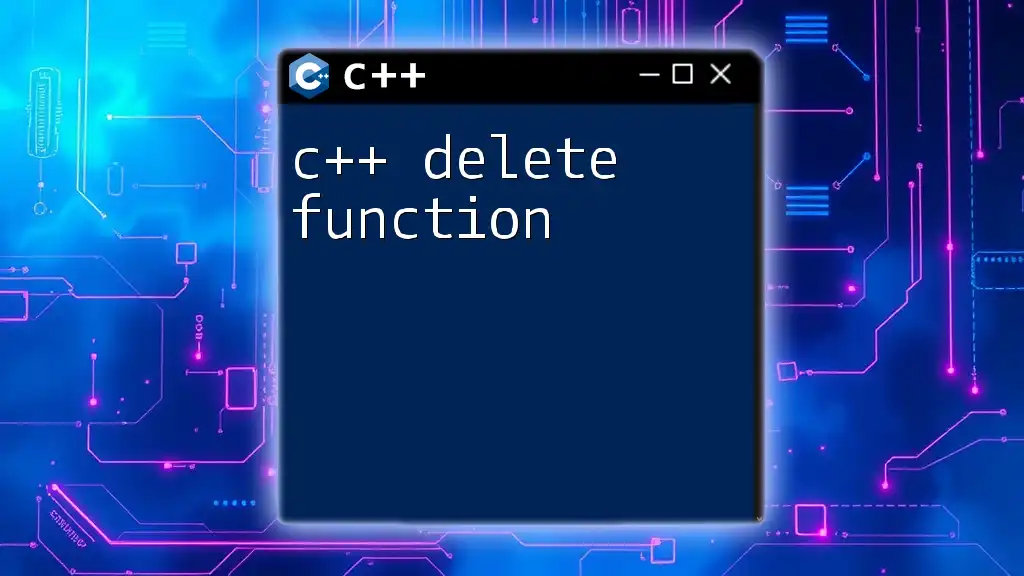
Best Practices for Using `delete this`
When to Use `delete this`
Using `delete this` should be a well-considered decision. Ensure that:
- The object was dynamically allocated using `new`.
- No other part of the program retains a reference to this object after calling `delete this`.
- You're working within environments that support explicit ownership semantics.
It's often seen in factory patterns where an object determines that it should clean itself up after an operation, particularly in callback situations.
Alternatives to `delete this`
Smart pointers such as `std::unique_ptr` or `std::shared_ptr` provide safer memory management compared to raw pointers. Smart pointers automatically manage object lifetimes and prevent issues with manual memory management, such as double deletions or memory leaks.
In scenarios where `delete this` seems appropriate, consider using these alternatives:
#include <memory>
class SmartExample {
public:
void doSomething() {
// Instead of delete this, use smart pointers
std::shared_ptr<SmartExample> ptr(this, [](SmartExample* p) { delete p; });
}
};
Using smart pointers can greatly simplify memory management, making it less error-prone.
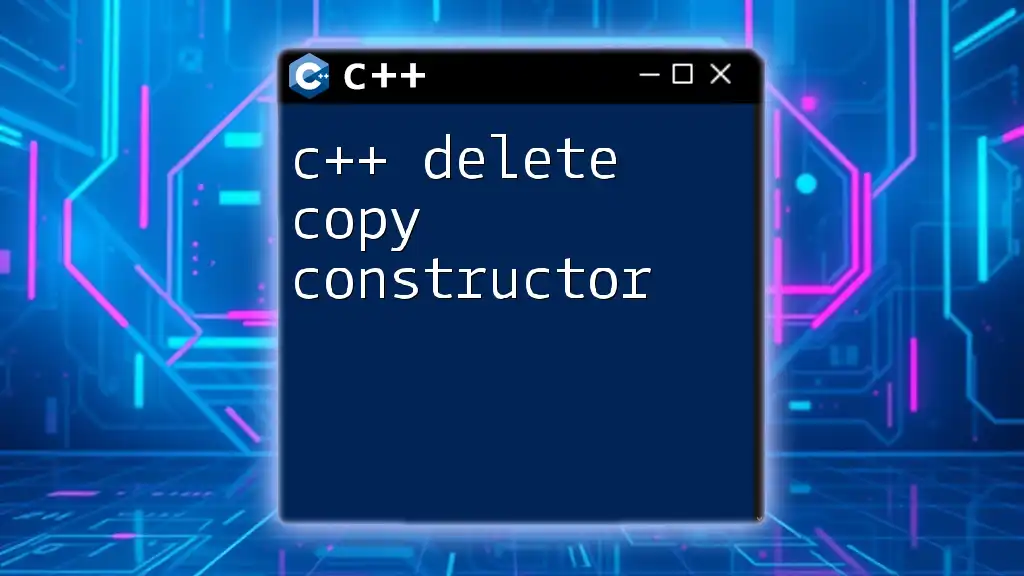
Conclusion
Understanding `c++ delete this` is crucial for effective memory management in C++. While it offers a powerful tool for dynamic object destruction, it must be used judiciously to avoid potential pitfalls such as undefined behavior and memory issues. Cautiously considering ownership and opting for modern alternatives like smart pointers can lead to clearer, safer, and more maintainable code.
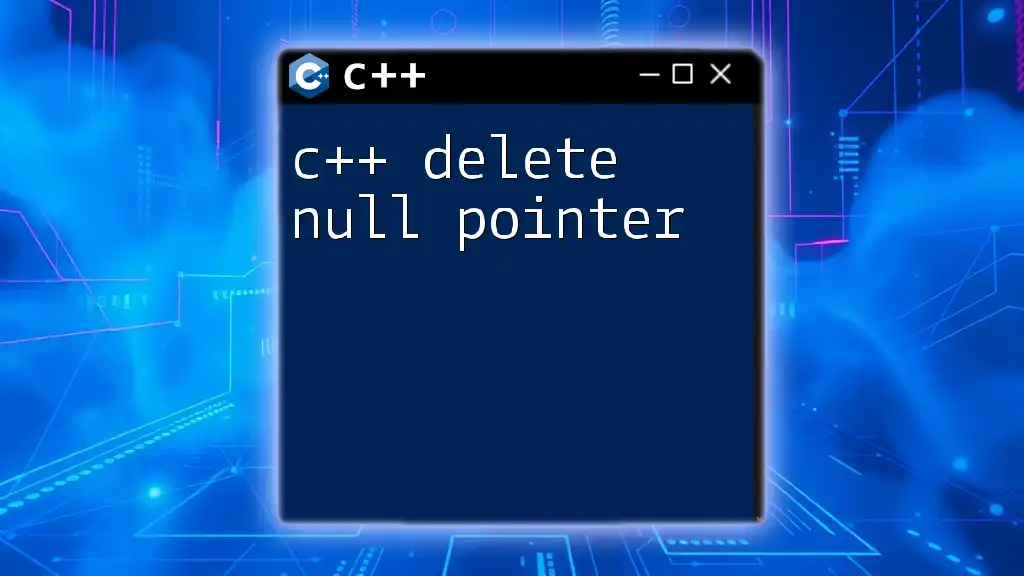
Additional Resources
For those looking to delve deeper into C++ memory management, consider the following resources:
- Official C++ documentation and guidelines
- Books on modern C++ practices
- Online coding platforms for hands-on experience and exercises with memory management concepts
Code References
To find more examples and interact with code snippets designed to help understand `delete this` and other memory management strategies, feel free to check repositories or community forums dedicated to C++.