In C++, the `delete` operator is used to free memory that was dynamically allocated with `new`, preventing memory leaks.
int* ptr = new int; // dynamically allocate an integer
*ptr = 42; // assign value
delete ptr; // free the allocated memory
Understanding Memory Management in C++
What is Memory Management?
Memory management is an essential concept in programming that deals with the allocation, usage, and deallocation of memory in a computer's system. In languages like C++, effective memory management significantly impacts performance and stability. Dynamic memory allocation is a critical feature, allowing you to request memory as needed during runtime.
Stack vs Heap Memory
In C++, memory can be allocated in two main areas: stack and heap.
-
Stack Memory: Memory is often allocated on the stack for local variables. The stack follows a strict Last In, First Out (LIFO) structure. When a function exits, all the stack memory for local variables is automatically freed, ensuring swift memory recovery. However, stack memory has limited capacity.
-
Heap Memory: The heap allows dynamic memory allocation and deallocation during program execution. Unlike stack memory, you must manually manage the memory on the heap by using operators such as `new` and `delete`. This flexibility comes at the cost of potential issues like memory leakage if not handled properly.
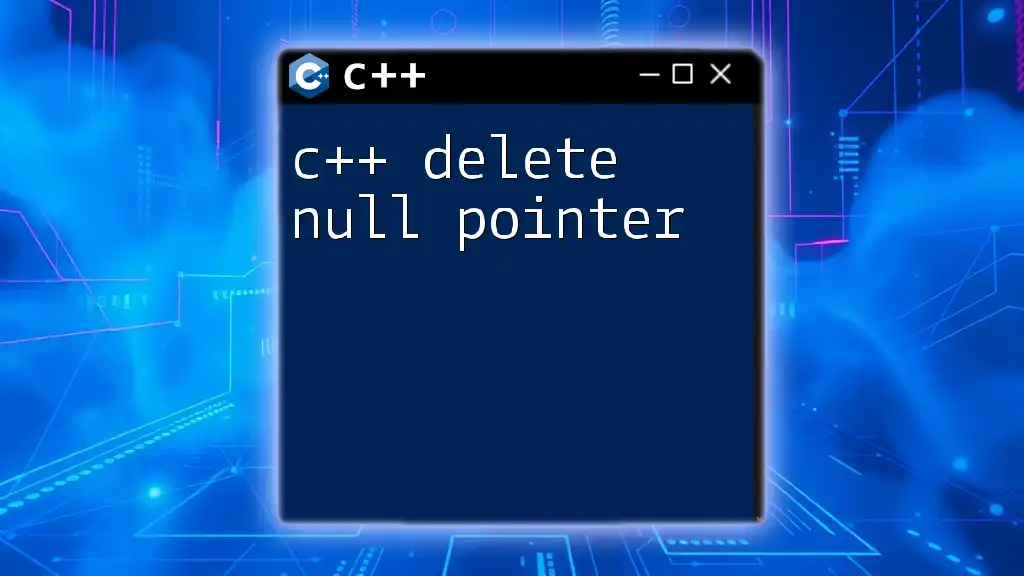
The C++ New and Delete Operators
What is the New Operator?
The `new` operator in C++ is designed for dynamic memory allocation. It creates an object or an array in the heap and returns a pointer to it. The syntax used with `new` resembles a function call and can allocate memory for single objects or arrays.
- Syntax:
Type* ptr = new Type;
- Difference Between `new` and `malloc`: While both allocate memory, `new` initializes the object and calls the constructor if applicable, while `malloc` merely allocates raw memory without any initialization.
Example of Using the New Operator
For instance, allocating an array of integers can be achieved using `new` as follows:
int* arr = new int[10]; // Allocating an array of 10 integers
In this example, `arr` points to a block of memory sufficient to hold ten integers on the heap, allowing for dynamic data handling.
What is the Delete Operator?
The `delete` operator is used to release memory that was previously allocated with `new`. Continuing to hold onto a pointer without deletion can lead to a memory leak, where allocated memory is no longer reachable, yet still "in use," ultimately leading to wasted resources.
- Syntax:
delete pointer;
- Like `new`, `delete` also comes in a variant for arrays.
Example of Using the Delete Operator
To free the memory allocated for an array, you can use:
delete[] arr; // Releasing the memory allocated for the array
Here, it’s crucial to match `delete` with `new` and `delete[]` with `new[]` to prevent undefined behavior.
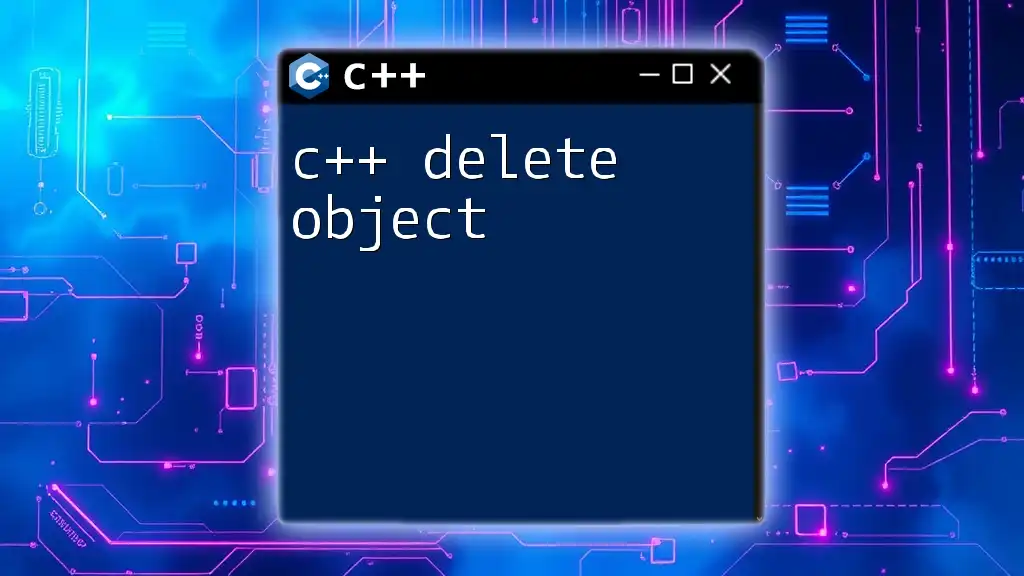
New and Delete in C++
Allocating Single Objects with New
When working with user-defined types, you can instantiate an object on the heap using the `new` operator:
MyClass* obj = new MyClass();
This code dynamically allocates a `MyClass` object and stores the address in the pointer `obj`, which allows for the object's lifetime to extend beyond the scope where it was created.
Deallocating Single Objects with Delete
To deallocate the memory occupied by a single object, you would use:
delete obj; // Deallocating single object
Failing to call `delete` will result in memory leaks, as that memory will remain allocated until the program terminates.
Allocating Arrays with New
To allocate an array of objects, use:
int* arr = new int[5];
Here, `arr` points to the first element of a dynamically allocated array containing five integers.
Deallocating Arrays with Delete
For arrays, make sure to use `delete[]` to free up the allocated memory:
delete[] arr; // Must use delete[] for arrays
Using `delete` instead of `delete[]` can lead to undefined behavior since it does not properly clean up the memory allocated for the entire array.
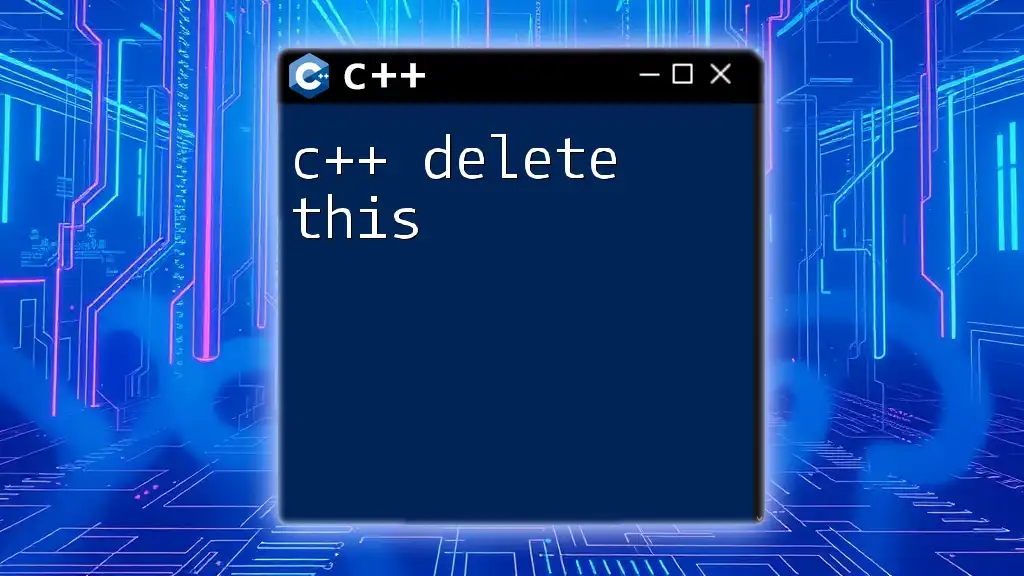
Common Mistakes with New and Delete in C++
Forgetting to Delete
One of the most common pitfalls in C++ programming is forgetting to delete dynamically allocated memory. This oversight can lead to memory leaks, where the unused memory consumes resources and slows down the application. For instance, if you repeatedly allocate memory without freeing it, the program could eventually exhaust the available memory, causing crashes.
Using Delete on Non-Dynamic Memory
Attempting to use `delete` on non-dynamic memory, such as stack-allocated variables, leads to undefined behavior. Consider the following example:
int x = 5;
delete &x; // Dangerous and incorrect!
The memory management system does not know how to handle this misuse, potentially leading to corruption or crashes.
Double Deletion
Another serious issue is "double deletion," where a pointer is deleted more than once. This can corrupt memory and lead to unpredictable behavior. For example:
int* ptr = new int;
delete ptr;
delete ptr; // Attempting to delete again causes undefined behavior
Always set pointers to `nullptr` after deletion to help avoid this mistake:
delete ptr;
ptr = nullptr; // Safe practice
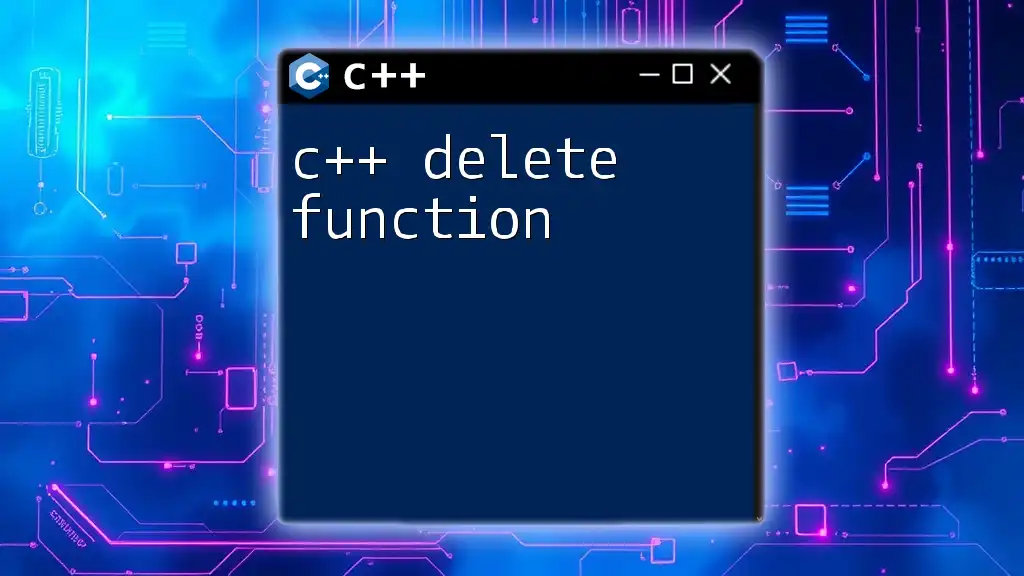
Best Practices for Using New and Delete in C++
Always Pair New with Delete
For every `new` allocation, there should be a corresponding `delete`. This pairing ensures that memory is effectively managed, and resources are released appropriately. Neglecting this principle can result in memory leaks.
Use Smart Pointers
C++11 introduced smart pointers (like `std::unique_ptr` and `std::shared_ptr`) which automatically manage memory for you. By utilizing smart pointers, the burden of manual memory management is lifted, leading to cleaner code:
#include <memory>
std::unique_ptr<MyClass> obj = std::make_unique<MyClass>(); // Automatic cleanup
Smart pointers ensure memory is properly released when they go out of scope, minimizing the chances of memory leaks and enhancing application stability.
Avoid Global New/Delete Overloading
While it’s possible to overload the `new` and `delete` operators, doing so can complicate maintenance and lead to unexpected behavior across your codebase. Stick to the default implementations unless there's a compelling reason.
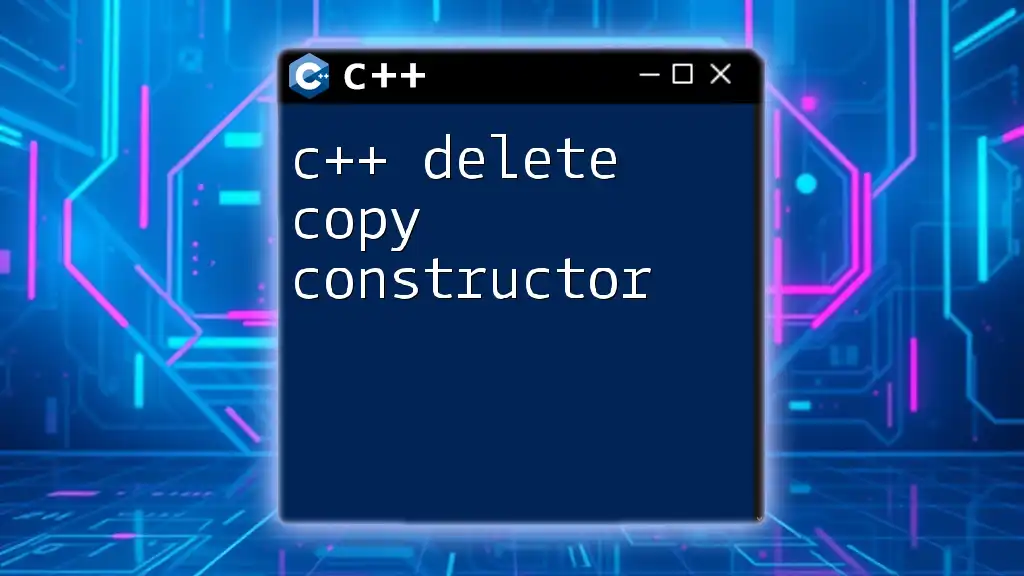
Real-World Examples of C++ New and Delete Usage
Creating and Destroying Objects in Game Development
In game development, dynamic memory management is crucial. Objects representing game entities (like characters or items) can be created at runtime. For instance:
Player* player = new Player();
When the player leaves the game, freeing the associated resources is critical to ensure performance:
delete player;
Dynamic Data Structures
New and delete are also fundamental when using dynamic data structures, such as linked lists. A simple linked list node can be created and deleted as follows:
struct Node {
int data;
Node* next;
};
Node* head = new Node();
head->data = 1;
head->next = nullptr;
// Later on, when we no longer need the node
delete head; // Release memory
This pattern of dynamically creating, manipulating, and deleting nodes underlines the necessity of careful memory management in C++.
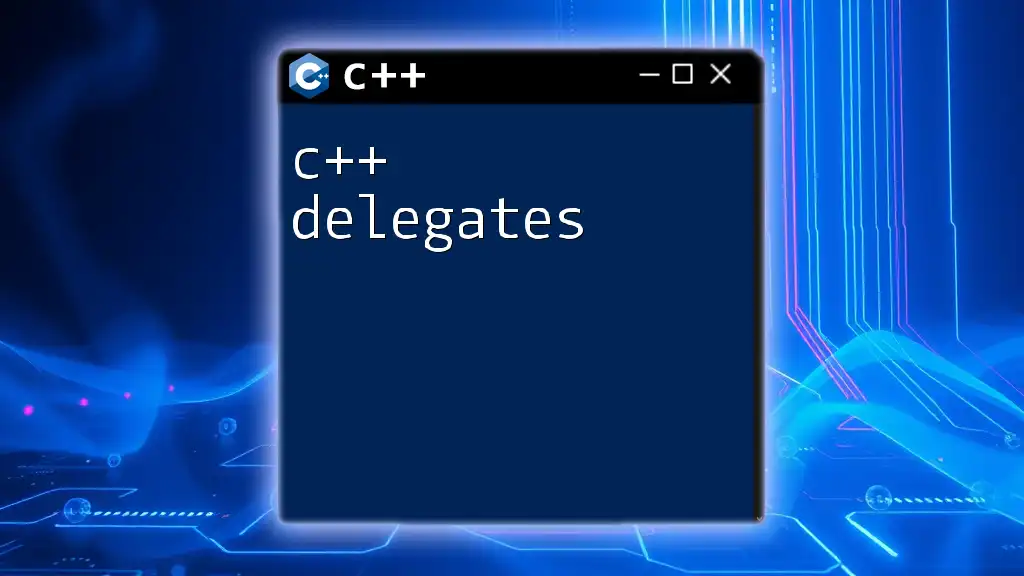
Conclusion
Summary of Key Points
Understanding how to properly use the `new` and `delete` operators in C++ is fundamental to effective memory management. Always ensure to pair these operators correctly, watch for common pitfalls, and leverage smart pointers when possible to maintain clean, efficient code.
Further Learning Resources
Deepening your knowledge in C++ memory management will greatly enhance your programming skills. Consider reading reputable C++ resources or online documentation, and practice through coding exercises to solidify your understanding of dynamic memory concepts.