The `system("pause")` command in C++ is used to halt the program's execution and wait for the user to press a key before it continues or exits. Here's an example of how to use it:
#include <cstdlib> // Include necessary header for system
int main() {
// Your code logic here
system("pause"); // Pauses the console and waits for user input
return 0;
}
What is C++ System Pause?
Definition of System Pause in C++
C++ system pause is a command often utilized in programming to temporarily halt the execution of a program, waiting for user interaction. This command allows users to see output data before the program terminates. Without a pause, the console window may close too quickly for users to read important messages or debug information.
Key Uses and Applications
The system pause command is particularly valuable in contexts such as debugging and user interactions. Debugging requires validating the program's functioning, and a pause is crucial to review outputs. Moreover, in applications with graphical user interfaces (GUIs), console applications may still leverage system pause to maintain user engagement and ensure readability.
Example Use Cases:
- Interactive console applications that need user confirmation.
- Displaying programming results before program termination during testing.

Understanding the Basic Syntax
Syntax of System Pause Command
The typical way to implement system pause in C++ is through the following syntax:
system("pause");
This tells the operating system to execute the pause command, which—on Windows—displays "Press any key to continue..." and waits for user input.
Compatibility with Different Operating Systems
It's important to note that the behavior of the system pause command varies by platform. While `system("pause")` is effective on Windows, it won't work on Unix-based systems like Linux or macOS. Here, developers must explore alternatives, as `system("pause")` is not universally supported.
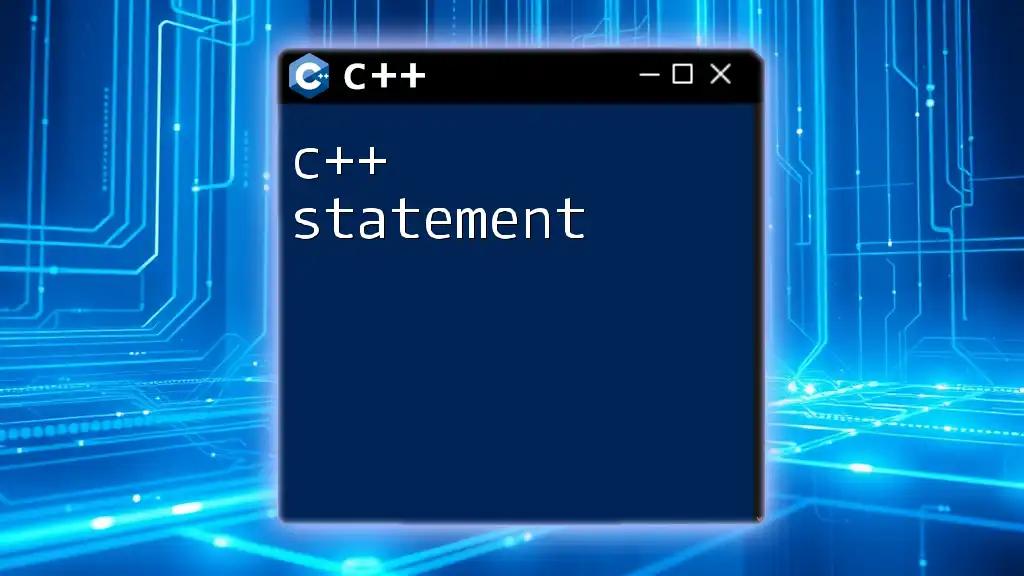
How to Use System Pause in C++
Example Code Snippet
Here's a straightforward example demonstrating how to integrate the system pause command in a C++ program:
#include <iostream>
#include <cstdlib> // For system()
int main() {
std::cout << "Hello, World!" << std::endl;
system("pause"); // This line causes the program to wait for user input
return 0;
}
In this example, after printing "Hello, World!", the program execution halts until the user presses a key. This is particularly useful during the development stage, where immediate feedback on console output can help identify issues quickly.
Step-by-Step Implementation
- Include Necessary Headers: Make sure to include `#include <cstdlib>` to utilize the `system()` function.
- Write the Main Function: Create the `main()` function where your program logic will reside.
- Implement the Pause Command: Strategically place `system("pause");` where you want to halt execution—typically before the program ends.
- Compile and Run: Use an IDE or command line to compile and execute your code, watching as it pauses for user input.

Common Mistakes and Troubleshooting
Mistakes to Avoid When Using System Pause
A common pitfall is overreliance on system pause, which can lead to poor programming practices. Developers might forget to remove this command in production code, leading to unnecessary pauses that frustrate users. Additionally, failing to include necessary headers may prevent the program from compiling.
Troubleshooting Common Errors
If `system("pause")` generates errors:
- Verify the syntax and ensure that `<cstdlib>` is included.
- Check if you're using an incompatible operating system; consider alternative methods for Unix-based systems, like `std::cin.get()`.

Best Practices for Using System Pause
When to Use System Pause
It's essential to use system pause judiciously, primarily in situations requiring user verification or when debugging. Avoid using it excessively, as it can break program flow in practical applications where user experience matters.
Performance and Code Quality Considerations
Using system pause can impact performance slightly due to the additional wait time. Excessive pauses may lead to a jarring experience for users, particularly in applications designed for efficiency. Aim to strike a balance between necessary pauses and smooth program execution.
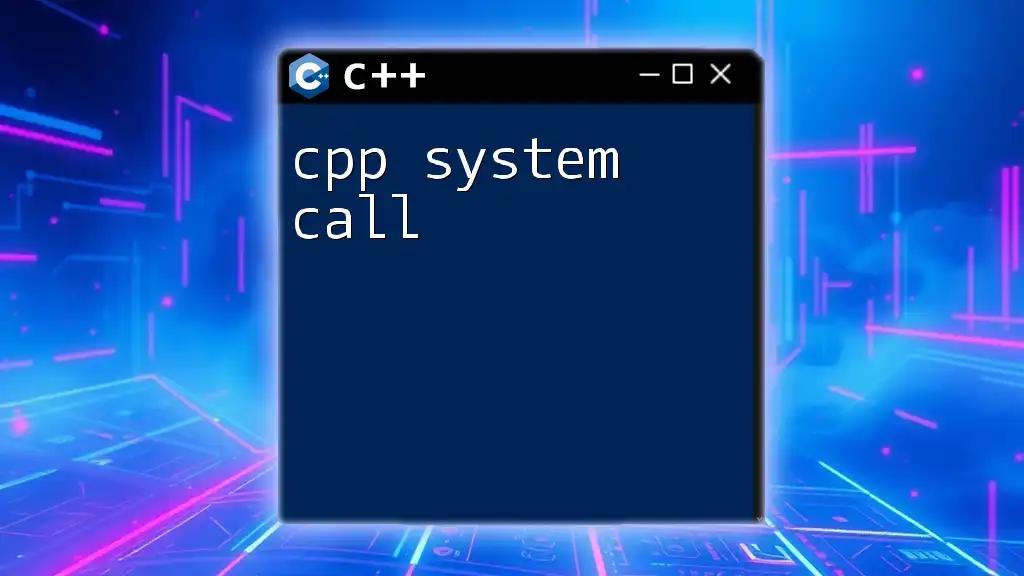
Alternatives to System Pause
Alternative Commands in C++
Instead of `system("pause"),` consider using `std::cin.get()`:
std::cin.get(); // Waits for the user to press Enter
This alternative is more reliable and portable across various systems, enhancing code quality.
Cross-Platform Alternatives
When developing applications intended for multiple platforms, explore platform-independent approaches to implement pauses. In addition to `std::cin.get()`, you could utilize `std::this_thread::sleep_for()` to create timed delays, although this keeps the program running rather than pausing for user input.
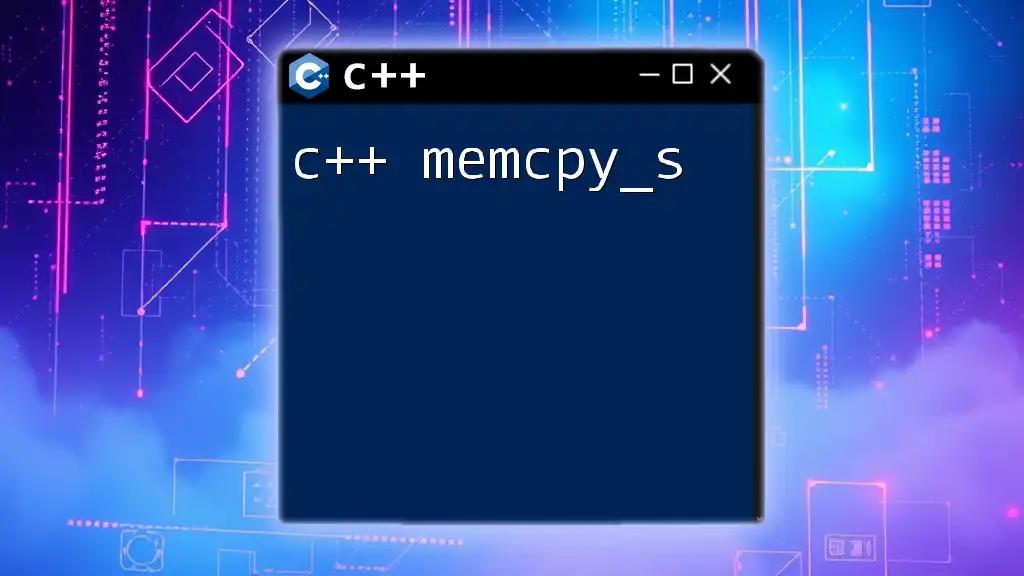
Conclusion
Recap of System Pause in C++
In summary, the C++ system pause command is a powerful tool that can aid developers by providing crucial output visibility during the testing phase. However, it is essential to use this command thoughtfully and recognize its limitations across different operating systems.
Encouragement for Further Learning
As you continue your journey in C++, consider exploring additional topics related to system commands and console control. Mastery of these concepts will not only improve your programming skills but also enhance the overall quality of your code and user experience.