In C++, the `&` symbol is used both as a bitwise AND operator and to denote a reference in variable declarations, enabling you to create aliases to existing variables.
Here’s an example of using the `&` symbol for both cases:
#include <iostream>
int main() {
int a = 5, b = 3;
int c = a & b; // Bitwise AND operation
int& ref = a; // Reference to variable 'a'
ref = 10; // Changing value of 'a' through the reference
std::cout << "Bitwise AND of a and b: " << c << std::endl; // Outputs: 1
std::cout << "Value of a through reference: " << ref << std::endl; // Outputs: 10
return 0;
}
What is the "&" Symbol in C++?
The & symbol in C++ is versatile, offering functionality in various programming contexts. Primarily, it serves as both the address-of operator and a means of creating reference variables. Understanding its usage is crucial for effective C++ programming.
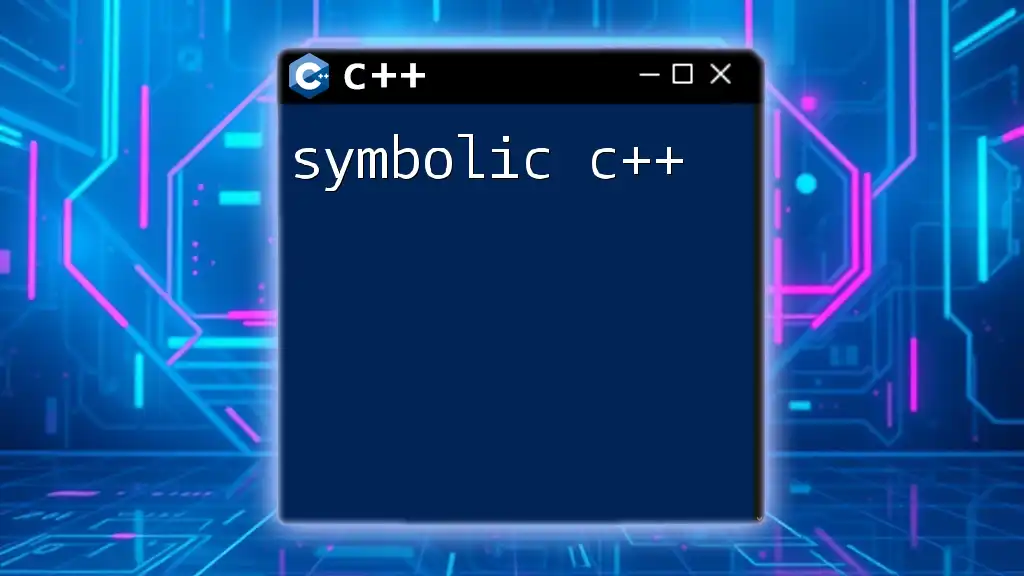
The "&" Symbol as an Address-of Operator
What is the Address-of Operator?
The address-of operator allows you to retrieve the memory address of a variable. When you apply the & symbol before a variable name, it returns the location in memory where that variable is stored.
For example, consider the following code snippet:
int x = 10;
int* ptr = &x; // ptr now holds the address of x
In this code, `ptr` becomes a pointer that stores the address of the variable `x`.
Advantages of Using the Address-of Operator
Using the address-of operator can significantly enhance your program's performance and efficiency in memory management. By directly accessing the memory address, you can manipulate variable values without unnecessary copies, which can be particularly beneficial when working with large data structures.
For instance, passing a large object to a function by its address rather than by value can improve execution speed and reduce memory usage.
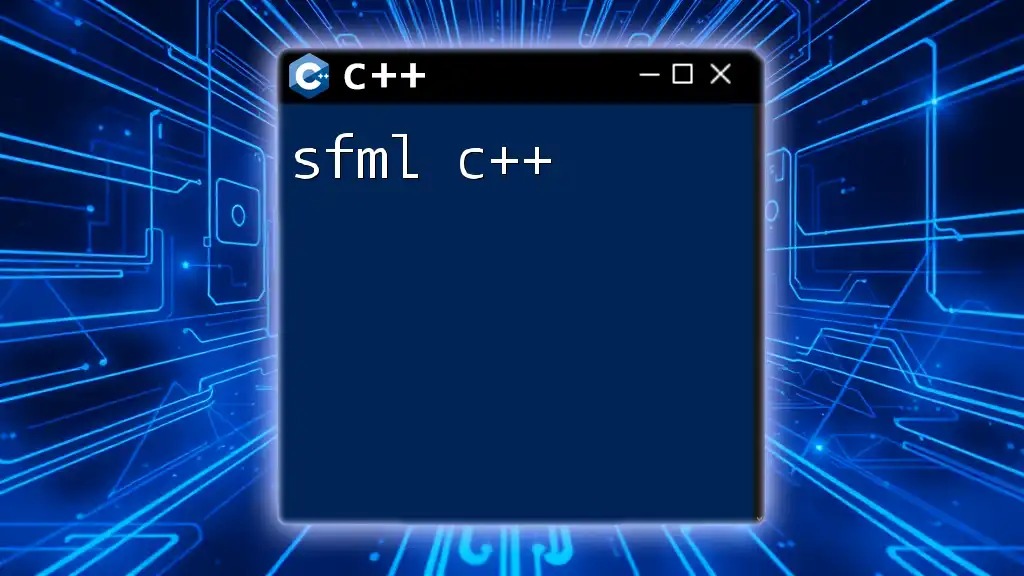
The "&" Symbol in Reference Variables
Understanding Reference Variables
A reference variable is an alias for another variable. Unlike pointers, which can be reassigned to different memory addresses, reference variables are bound to the variable they refer to at initialization and cannot be changed to refer to another variable.
Consider the following code snippet:
int x = 20;
int& ref = x; // ref is now a reference to x
In this scenario, `ref` is an alias for `x`. Any operation on `ref` directly affects `x`.
Use Cases for Reference Variables
Reference variables are especially useful for function parameters and return types, where you might want to modify the original argument rather than a copy.
Here’s an example demonstrating pass-by-reference:
void increment(int& value) {
value++;
}
In this function, any integer passed will be incremented directly, showcasing the performance benefits of passing by reference over pass-by-value, especially for complex data types.
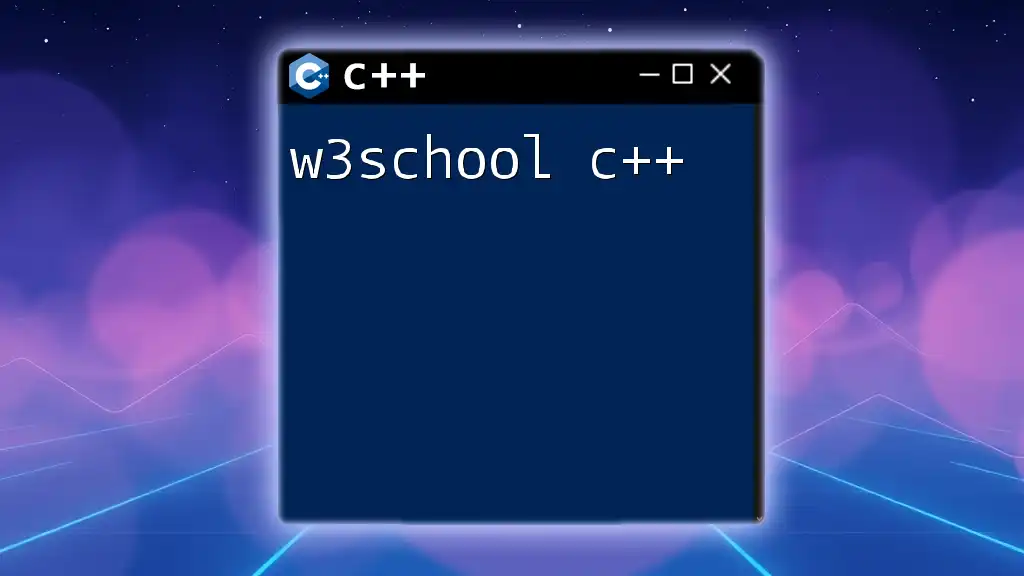
The "&" Symbol in Bitwise AND Operations
What is a Bitwise AND Operation?
In C++, the & symbol also functions as a bitwise AND operator. This operation compares the binary representation of two integers, returning a new integer whose bits are set to 1 only where both operands have a bit set to 1.
Here’s a quick example:
int a = 5; // 0101
int b = 3; // 0011
int result = a & b; // results in 0001, which is 1
In this scenario, the bitwise AND operation results in the integer 1 because only the last bit of both numbers is set.
Practical Applications of Bitwise AND
The bitwise AND operation is prevalent in low-level programming, especially in flag manipulation. For instance, when working with device drivers or embedded systems, you might need to check or modify specific bits within a byte or a word.
Understanding how to effectively use the bitwise AND operator can lead to more efficient algorithms, particularly in bitmasking scenarios.
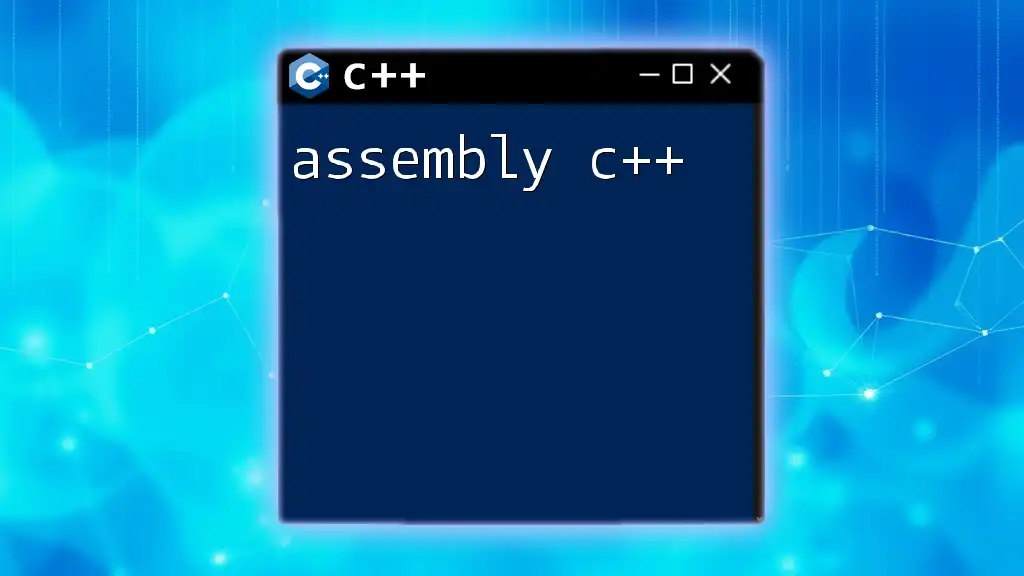
Common Mistakes to Avoid with the "&" Symbol
Misunderstanding and Confusion
One common mistake new programmers make is misunderstanding the context in which the & symbol is used. It's crucial to differentiate between using the & symbol for addressing variables and using it as a bitwise operator.
Additionally, there is often confusion between reference variables and pointers. While both can provide indirect access to a variable's value, their mechanics and use cases differ significantly.
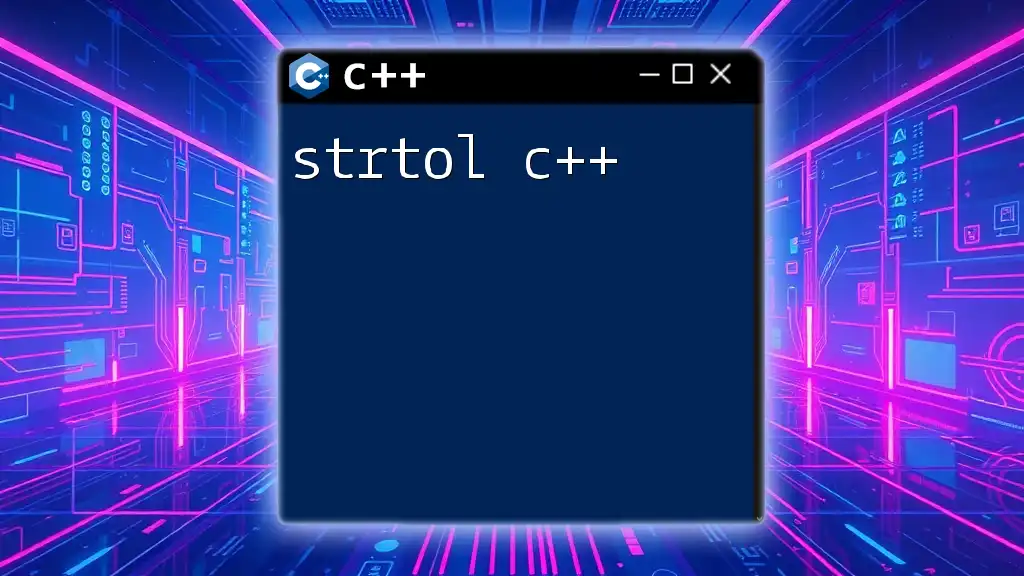
Best Practices for Using the "&" Symbol
To master the & symbol c++, follow these best practices:
- Ensure you understand the context before applying the & symbol to avoid confusion.
- Prefer using reference variables for function parameters when you want to avoid unnecessary copying but need mutable access to the argument.
- Use the address-of operator judiciously, ensuring that you're aware of the lifetime of the referenced variable to avoid dangling pointers.
Implementing these practices will enhance the clarity, maintainability, and performance of your code.
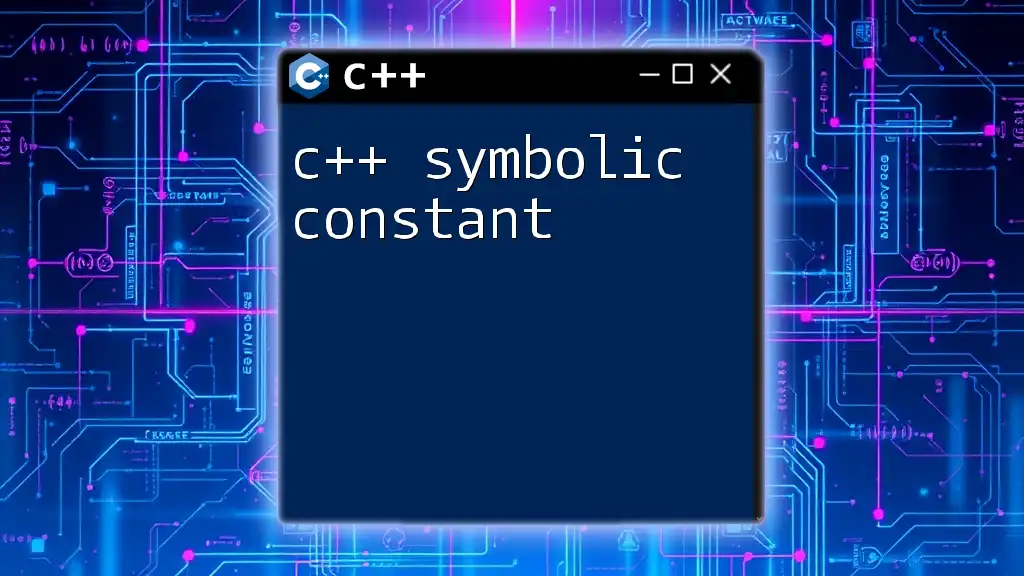
Conclusion
The & symbol c++ is a multifunctional operator that serves crucial roles in memory management, reference variables, and bitwise operations. Understanding its various applications allows for more proficient coding and better performance in your C++ programs. As you practice using the & symbol across different contexts, you'll gain more confidence and expertise in your programming skills.
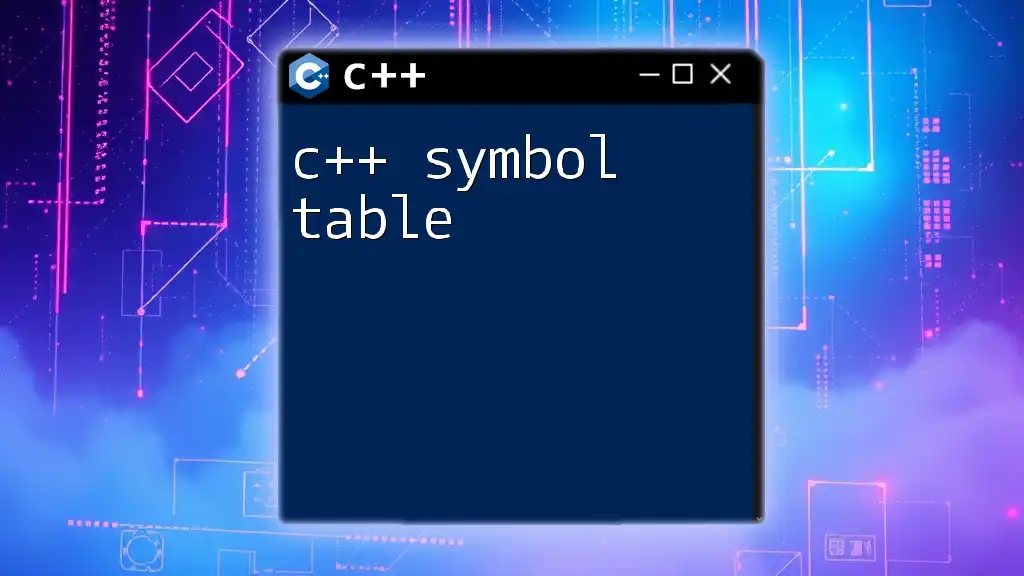
Additional Resources
For further reading, consider exploring materials on advanced C++ topics, focusing on memory management, pointer dereferencing, and bitwise operations to deepen your understanding of the & symbol and its applications.