An "unresolved external symbol" error in C++ indicates that the linker cannot find the definition of a function or variable that has been declared but not defined, often due to missing object files or incorrect linking of source files.
Here's an example of how this error might occur:
// main.cpp
#include <iostream>
void greet(); // Declaration of the function
int main() {
greet(); // Calling the function
return 0;
}
// Missing definition of the greet function
To resolve this, you would need to provide the definition for the `greet` function, possibly in a separate file that is correctly linked during the build process.
Understanding Unresolved External Symbols in C++
What is an Unresolved External Symbol?
An unresolved external symbol is a type of error that occurs during the linking phase of C++ compilation. Essentially, when a C++ program is compiled, the compiler generates object files containing compiled code. However, these object files may reference functions or variables that are declared but not defined. When the linker attempts to combine these object files into an executable, it encounters references to symbols it cannot resolve, resulting in this error.
Understanding how C++ compilers link code is essential for avoiding these errors. The linking process involves matching function declarations with their corresponding definitions. If the linker cannot find a required definition, it will flag that as an unresolved external symbol.
Causes of Unresolved External Symbols
The causes of unresolved external symbols can often be traced back to a few common issues:
-
Missing function definitions: This is perhaps the most straightforward cause, occurring when a function is declared but lacks an implementation.
-
Incorrect linker settings: Misconfigured or missing project settings can prevent the linker from locating the appropriate files or libraries.
-
Using the wrong library or object files: Utilizing mismatched or outdated libraries can lead to unresolved references.
-
Name mangling issues in C++: C++ compilers often "mangle" names to include type information, which can cause issues when interfacing with C code or using certain libraries.
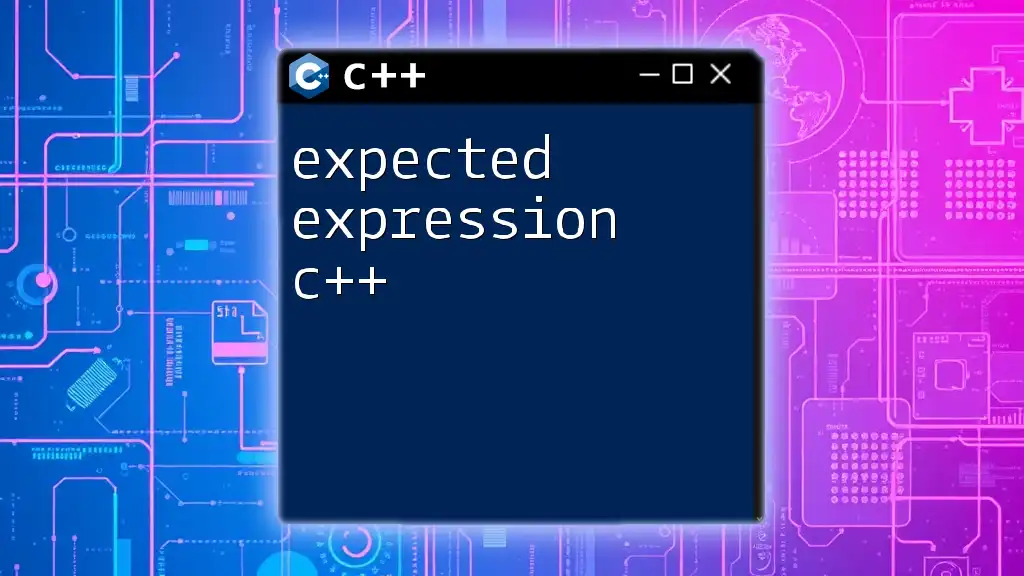
Common Scenarios Leading to Unresolved External Symbols
Scenario 1: Missing Function Definitions
One of the most prevalent causes of unresolved external symbol errors is missing function definitions. For instance, consider the following example:
// Header file: my_functions.h
void myFunction(); // Declaration
// Main file: main.cpp
#include "my_functions.h"
int main() {
myFunction(); // This will cause an unresolved external symbol error
return 0;
}
In this scenario, the header file declares the function `myFunction()`, but there is no corresponding definition provided in any source file. When the linker encounters the call to `myFunction()`, it cannot find its implementation, leading to an unresolved external symbol error.
Resolution: To fix this, you must provide the function definition:
// Implementation in my_functions.cpp
#include "my_functions.h"
void myFunction() {
// Function implementation
}
Scenario 2: Incorrect Linker Settings
Another frequent issue arises from incorrect linker settings. Misconfigured project settings can lead to the linker not being able to find the necessary object files or libraries. This typically happens during project setup, where the paths to required libraries are not correctly specified.
If you are using an IDE like Visual Studio, check the project properties under Linker > General. Ensure that the Additional Library Directories include paths to all relevant libraries. For GCC users, verify that you are linking against the correct library using the `-l` flag in your Makefile or compile command.
Scenario 3: Using the Wrong Library
Using the wrong version of a library can also result in unresolved external symbol errors. For example, if you compiled your code with one version of a library but linked against another incompatible version, the linker may not find the definitions it expects.
To prevent this, ensure that you are consistently using the same library version across your project. Checking the documentation of the libraries you are using, specifically the compatibility notes, can also help prevent these issues.
Scenario 4: Name Mangling Issues
Name mangling is a process by which C++ compilers encode additional information into the names of functions to facilitate function overloading. This can lead to unresolved external symbols, especially when interfacing with C code or using libraries that do not have the same name mangling conventions.
For instance, when using C functions in a C++ program, it is wise to declare those functions with `extern "C"`:
// C code file: my_c_code.c
extern "C" void myFunction(); // Prevent name mangling
// C++ file: main.cpp
#include "my_c_code.h"
int main() {
myFunction();
return 0;
}
Using `extern "C"` tells the C++ compiler that the function should not be name-mangled, allowing it to link correctly with the C function.
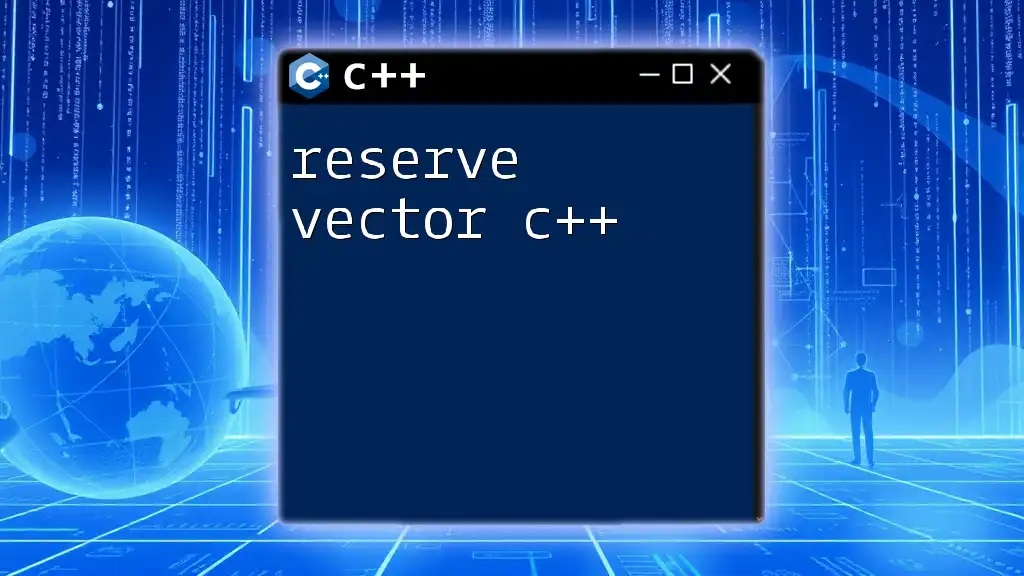
Debugging Unresolved External Symbols
Best Practices for Debugging
Debugging unresolved external symbols often begins with examining the error messages provided by the linker. These messages usually give you a clue about which symbol is unresolved and the file or line of code where the issue arises.
Utilizing compiler flags such as `-Wall` for GCC can also help, as these options provide additional warnings and notifications to aid in identification. Moreover, using debugging tools provided by your IDE can streamline the debugging process by allowing you to trace symbol usage across your project.
Tools and Techniques for Troubleshooting
In addition to simple error messages, several tools can assist in troubleshooting unresolved symbols. For example, the `nm` command on Linux allows you to inspect the symbols contained within compiled object files:
nm my_functions.o
For Windows users, `dumpbin` is a similar tool that can inspect object files and libraries, allowing you to check for the presence of expected function definitions.
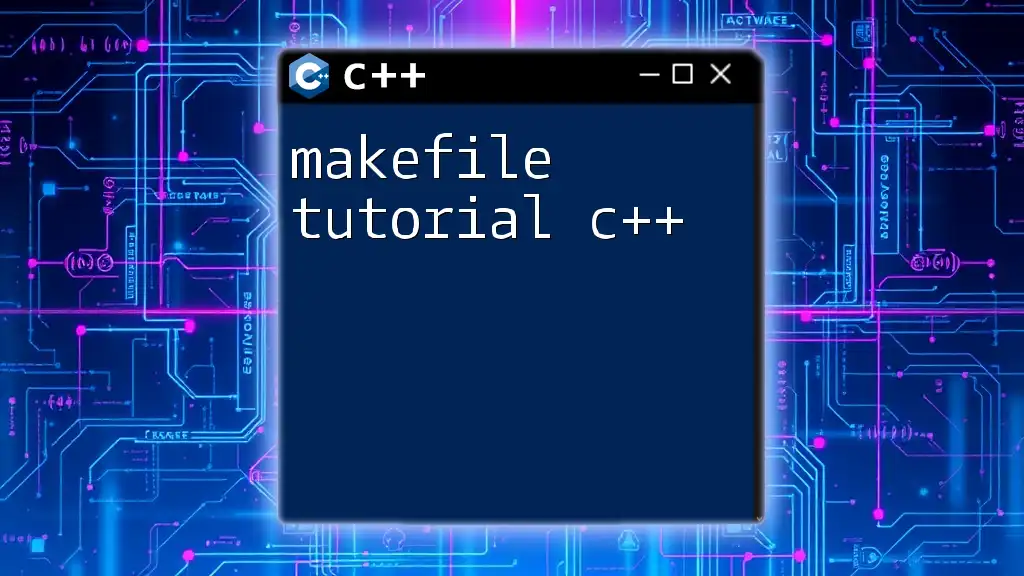
Preventing Unresolved External Symbol Errors
Writing Modular and Organized Code
To simplify your debugging process and avoid unresolved external symbols, it is crucial to maintain a clean and organized codebase. Ensure that function declarations and definitions are correctly separated, ideally in header (`.h`) and source (`.cpp`) files. Proper encapsulation promotes reusability and reduces the chances of running into linking errors.
Proper Project Configuration
It's also essential to ensure consistent settings across different build configurations, such as Debug and Release modes. Carefully check that all necessary files are included and linked correctly, and consider using version control tools to manage and track changes in project configurations.
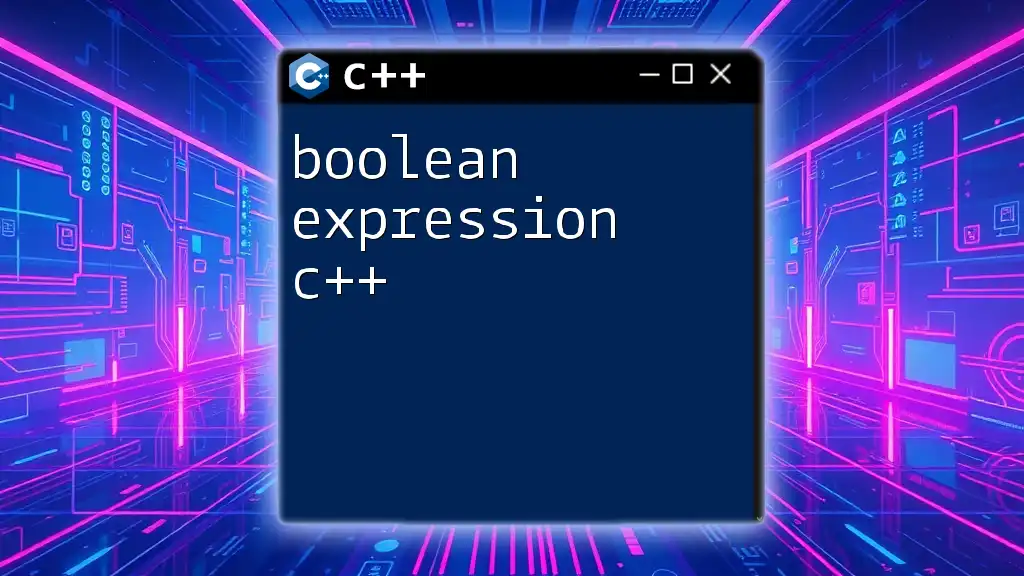
Conclusion
Understanding unresolved external symbols in C++ is vital for any developer working in this language. From recognizing the root causes of these errors to implementing best practices for debugging, your knowledge can significantly decrease the frequency of such occurrences.
Always remember that careful coding, thorough project organization, and consistent library usage go a long way toward avoiding unresolved external symbol errors. Embracing these principles can enhance your proficiency in C++ development and ensure smoother compilation and linking processes.
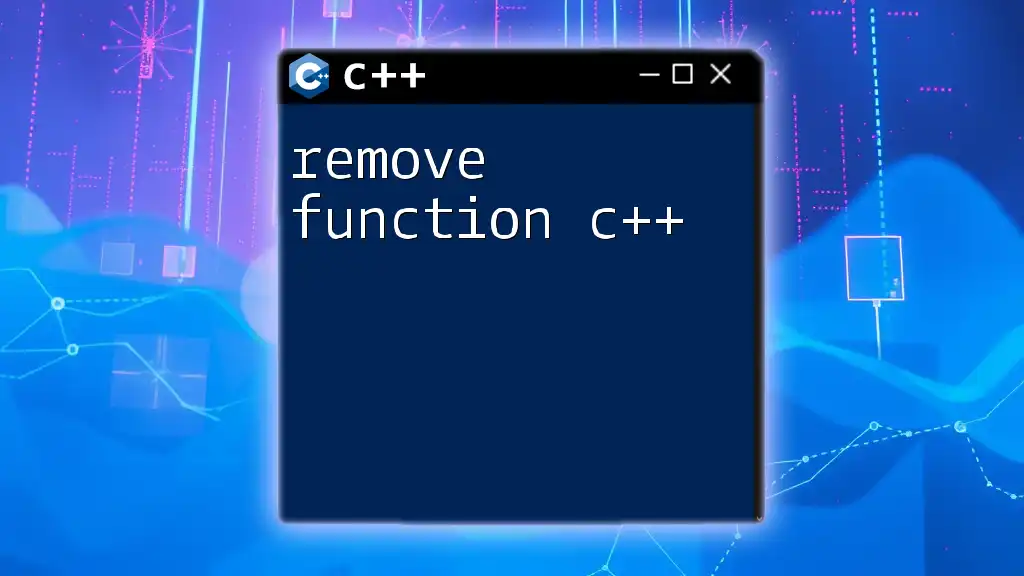
Call to Action
We’d love to hear about your experiences with unresolved external symbols! Share your story in the comments, and for more tips on mastering C++ commands quickly and effectively, check out our resources. Happy coding!
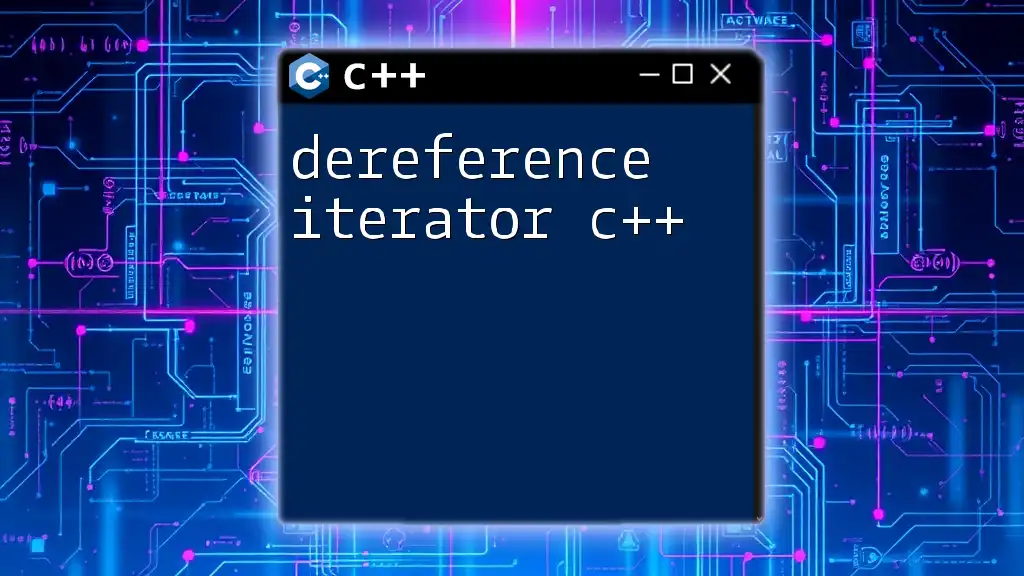
Additional Resources
For further reading on C++ linking and external symbols, consider exploring the official C++ documentation, participating in online forums, or even enrolling in specialized courses to deepen your understanding of these concepts.