Unreal Engine 5 allows developers to implement gameplay mechanics using C++ for enhanced performance and flexibility; here’s a simple example that demonstrates how to create a basic Actor class:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYPROJECT_API AMyActor : public AActor
{
GENERATED_BODY()
public:
AMyActor();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
};
AMyActor::AMyActor()
{
PrimaryActorTick.bCanEverTick = true;
}
void AMyActor::BeginPlay()
{
Super::BeginPlay();
}
void AMyActor::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
Setting Up Your Development Environment
Installing Unreal Engine 5
To start developing with Unreal Engine 5 C++, you need to install the engine. Visit the official Unreal Engine website and download the Epic Games Launcher. Once downloaded, follow these steps:
- Open the Epic Games Launcher.
- Go to the "Unreal Engine" tab and select "Library."
- Click on the "+" icon to add a version, and choose Unreal Engine 5, then click "Install."
After installation, ensure you have the necessary components, particularly those related to C++ development, included in the installation process.
Setting Up Visual Studio
Unreal Engine 5 highly recommends using Visual Studio for C++ development. Make sure to install the latest version (at least 2019 or newer) with the following components:
- Desktop development with C++
- Game development with C++
- C++ profiling tools
Once installed, open Visual Studio and navigate to the extensions to install any recommended plugins that enhance your workflow with Unreal Engine.
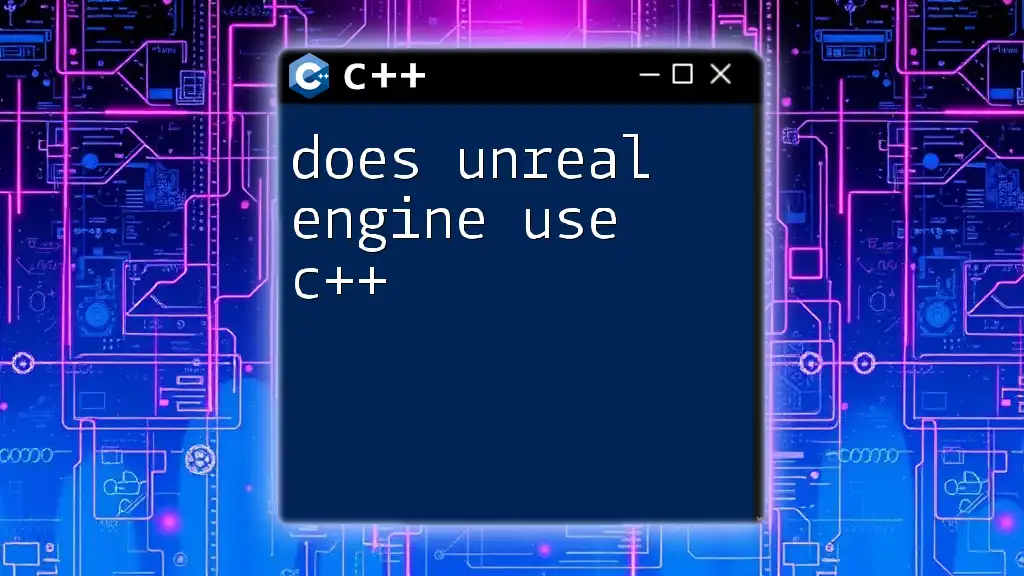
Understanding the Core Concepts of Unreal Engine 5 C++
Game Modes and Game States
A crucial part of any game is managing its rules and flow. Game Modes dictate how the game operates, while Game States track the current status within that game.
- Game Mode is classified as a set of rules dictating how the game operates (e.g., team-based rules or score limits).
- Game State manages relevant game data for all players, like scores and round status.
To implement a simple Game Mode, you can create a new C++ class extending from AGameModeBase. Here’s an example:
#include "GameFramework/GameModeBase.h"
#include "MyGameMode.generated.h"
UCLASS()
class MYPROJECT_API AMyGameMode : public AGameModeBase
{
GENERATED_BODY()
public:
AMyGameMode()
{
// Set default pawn class to our character
DefaultPawnClass = AMyPawn::StaticClass();
}
};
Actors and Components
In Unreal Engine 5, Actors are the base objects that exist in the game world, while Components enable specific functionalities.
Creating custom Actor classes allows you to define unique behaviors and properties. For instance:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYPROJECT_API AMyActor : public AActor
{
GENERATED_BODY()
public:
AMyActor();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
};
Blueprint vs. C++
While Blueprint provides a visually-driven way to script gameplay, using C++ allows for more control and efficiency in programming.
Advantages of C++:
- Better performance, especially for complex calculations.
- Direct access to engine systems that may not be exposed to Blueprint.
- Easier to manage large codebases with traditional programming paradigms.
To illustrate the difference, consider a simple action to make a character jump:
In Blueprint, you would visually connect nodes to trigger the jump functionality.
In C++, you can streamline this with a simple function call:
if (bJump)
{
ACharacter::Jump();
}
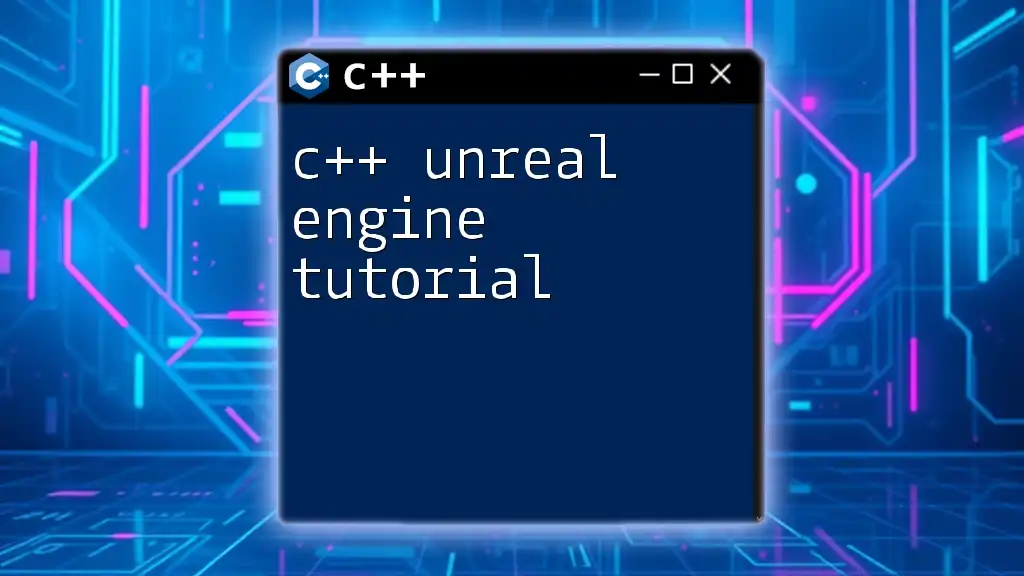
Building Your First C++ Project
Creating a New C++ Project
To create your initial C++ project in Unreal Engine 5:
- Open the Epic Games Launcher and start the Unreal Engine.
- Click on "New Project," select "Games," then choose C++.
- Select a template (e.g., First Person, Third Person) and configure settings for your project.
- Click "Create Project" and let UE5 set everything up.
Writing Your First C++ Game Code
Creating Custom Classes
Once your project is created, it's time to add some custom classes. Here’s how to set up a basic Character class:
#include "GameFramework/Character.h"
#include "MyCharacter.generated.h"
UCLASS()
class MYPROJECT_API AMyCharacter : public ACharacter
{
GENERATED_BODY()
public:
AMyCharacter();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
virtual void SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent) override;
};
Implementing Basic Gameplay Mechanics
Now let's implement player movement controls. In the SetupPlayerInputComponent function, you can bind control values to actions:
void AMyCharacter::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
PlayerInputComponent->BindAxis("MoveForward", this, &AMyCharacter::MoveForward);
PlayerInputComponent->BindAxis("MoveRight", this, &AMyCharacter::MoveRight);
}
void AMyCharacter::MoveForward(float Value)
{
AddMovementInput(GetActorForwardVector(), Value);
}
void AMyCharacter::MoveRight(float Value)
{
AddMovementInput(GetActorRightVector(), Value);
}
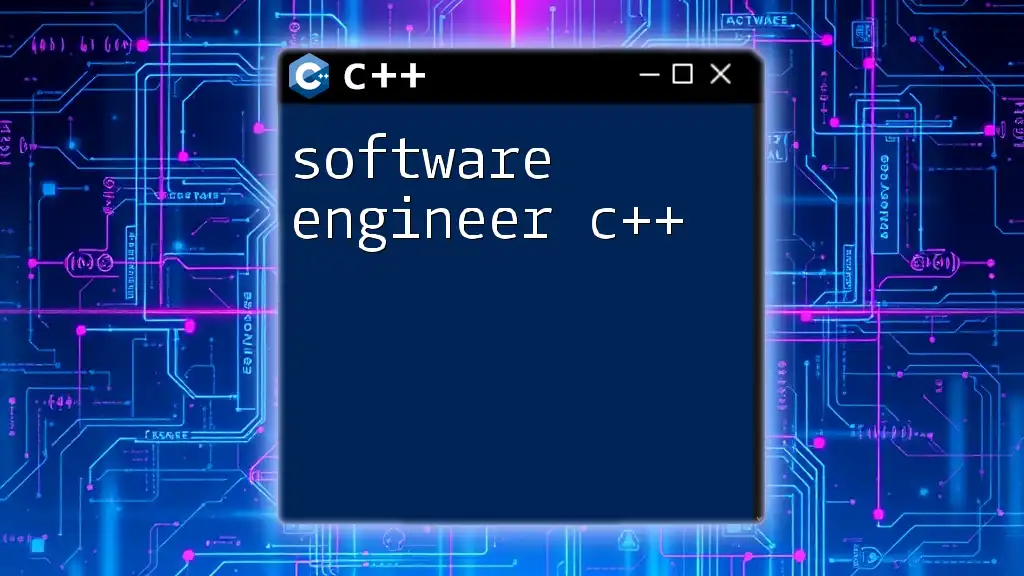
Advanced C++ Features in Unreal Engine 5
Memory Management
Memory management is crucial in C++ to avoid leaks and crashes. Unreal’s Garbage Collection system handles UObjects effectively. Always prefer smart pointers when dealing with non-UObjects to manage memory automatically.
Here’s how you would typically manage memory in C++:
TSharedPtr<MyClass> MySharedPtr = MakeShareable(new MyClass());
Unreal Engine's Reflection System
The Reflection System in Unreal is what allows you to expose C++ properties and functions to Blueprint, allowing for a seamless blend of both coding methods. The use of UFUNCTION and UPROPERTY macros simplifies this process significantly:
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category="Attributes")
float Health;
UFUNCTION(BlueprintCallable, Category="Actions")
void Attack();
Inheritance and Polymorphism
Inheritance is at the core of C++. By extending classes, you can create a hierarchy that facilitates code reuse and greater flexibility. Polymorphism allows for methods in derived classes to have unique implementations. This is particularly handy in game design by creating a base enemy class and various enemy types derived from it:
class ABaseEnemy : public ACharacter
{
public:
virtual void Attack();
};
class AOrcEnemy : public ABaseEnemy
{
public:
void Attack() override
{
// Orc specific attack logic
}
};
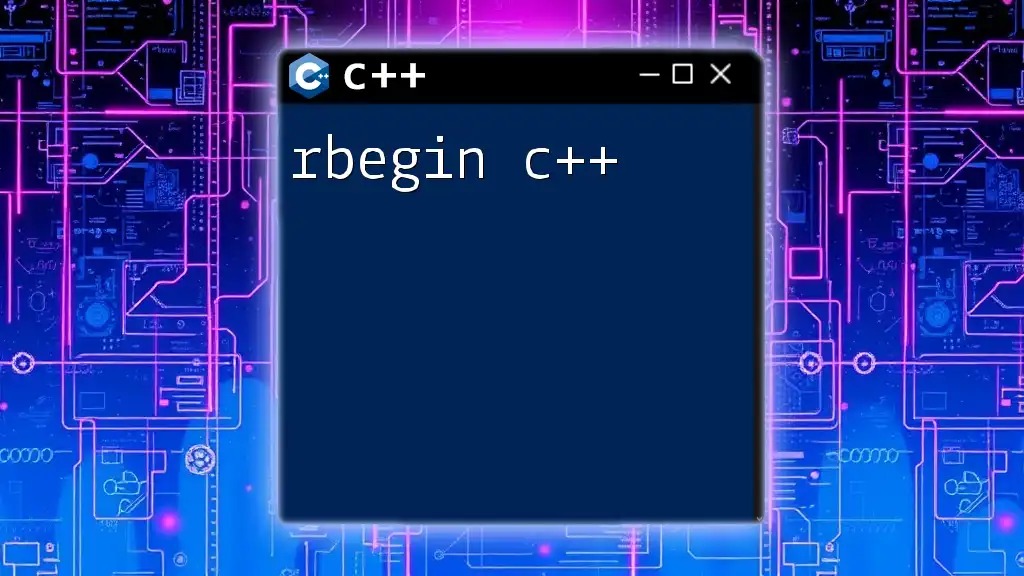
Optimization Techniques
Profiling Your C++ Code
Profiling is essential in optimizing the performance of your application. Use tools like Unreal Insights or Visual Studio Profiler to monitor CPU and memory usage. Learn to identify performance bottlenecks and optimize accordingly, whether by refining algorithms or reducing memory usage.
Improving Performance with C++
Follow best practices for writing efficient C++ code:
- Prefer using structures like TArray and TMap appropriately.
- Avoid frequently using complex loops; instead, opt for algorithms such as ForEach.
- Look out for unnecessary calculations and streamline them.
For instance, when polling input every frame, cache results instead of recalculating positions repeatedly.
Example of optimized looping:
for (const auto& Element : MyArray)
{
// Perform operations on each element
}
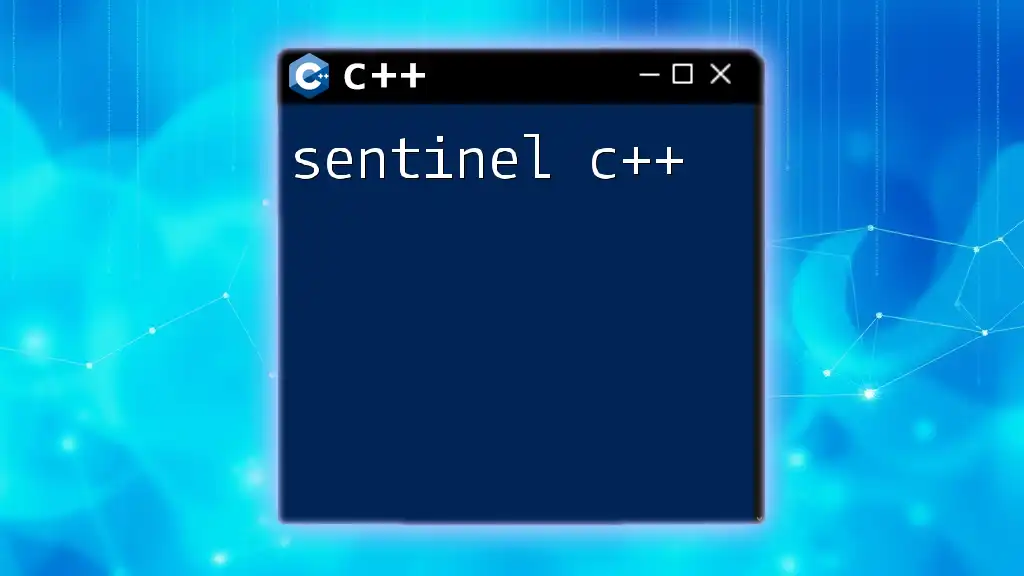
Debugging and Troubleshooting C++ Code
Common Errors in Unreal Engine C++
While working with Unreal Engine 5 C++, you may run into compilation errors. Common issues include syntax errors or missing includes. It's vital to understand Unreal’s build configuration tool, UnrealBuildTool, which handles C# project management.
Debugging Techniques
Debugging is crucial for maintaining a stable game. Use Visual Studio's debugging features, such as setting breakpoints and stepping through code. Making use of logging macros such as:
UE_LOG(LogTemp, Warning, TEXT("My message: %s"), *VariableName);
is invaluable for tracking the flow and identifying problem areas in your code.
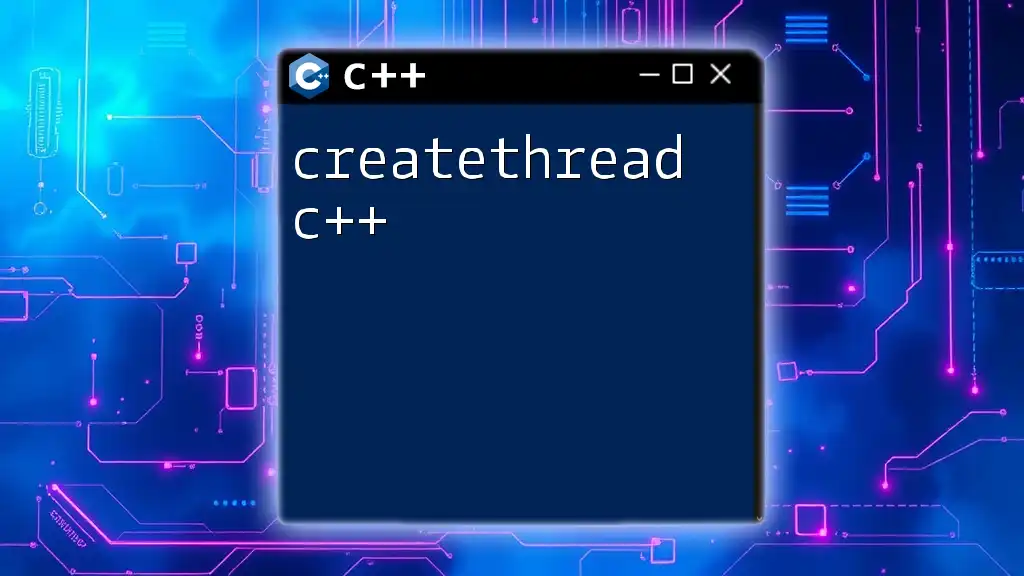
Conclusion
Leveraging Unreal Engine 5 C++ opens up numerous avenues for game development, allowing for more control and depth in gameplay mechanics. As you continue to explore the capabilities of this powerful engine and language, remember to experiment freely and utilize the resources provided by the community.
Embrace the journey of learning, and soon enough, you will be crafting complex games using Unreal Engine 5 C++. Engage with available documentation, forums, and online tutorials to enhance your understanding and skills further. Happy coding!