A Makefile simplifies the build process in C++ by allowing you to automate the compilation of your code with a set of rules, reducing the need for lengthy command line instructions.
Here's a basic example of a Makefile for a C++ project:
# Makefile example
# Compiler
CXX = g++
# Sources and Executable
SRC = main.cpp utils.cpp
EXEC = my_program
# Build rules
all: $(EXEC)
$(EXEC): $(SRC)
$(CXX) -o $(EXEC) $(SRC)
clean:
rm -f $(EXEC)
What is a Makefile?
A Makefile is a specialized file used by the `make` utility, designed to automate the build process of a project. In the context of C++ development, a Makefile serves to compile source code into executable programs efficiently, managing complex tasks with minimal code.
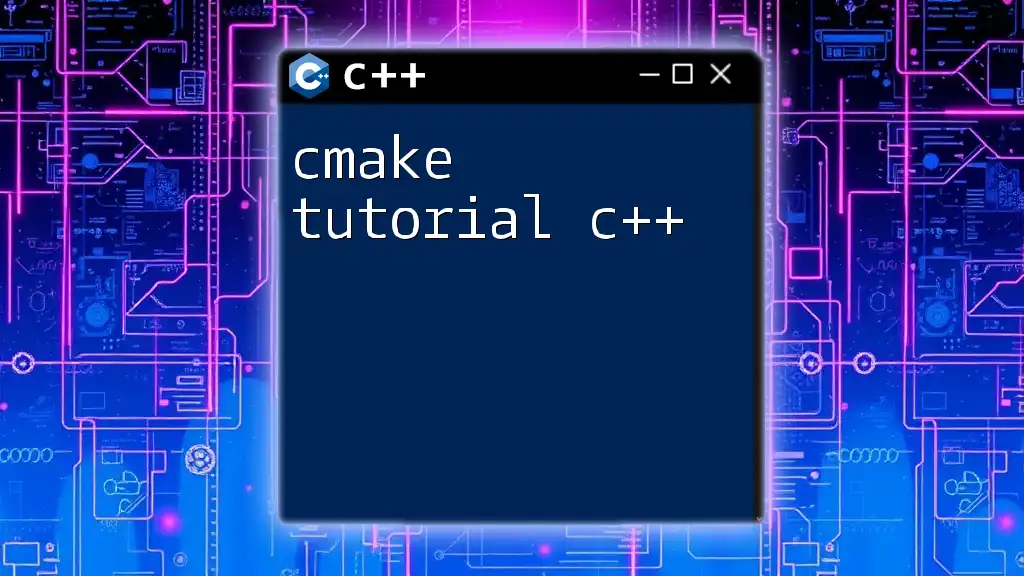
Why Use Makefiles?
Utilizing Makefiles in your C++ projects offers several advantages:
- Efficiency: Makefiles automate the repetitive tasks involved in building your application, saving developers time.
- Error Reduction: By specifying rules precisely, you lessen the chances of manual errors during the compilation process.
- Dependency Management: Makefiles track file dependencies and ensure that only files affected by changes are recompiled.
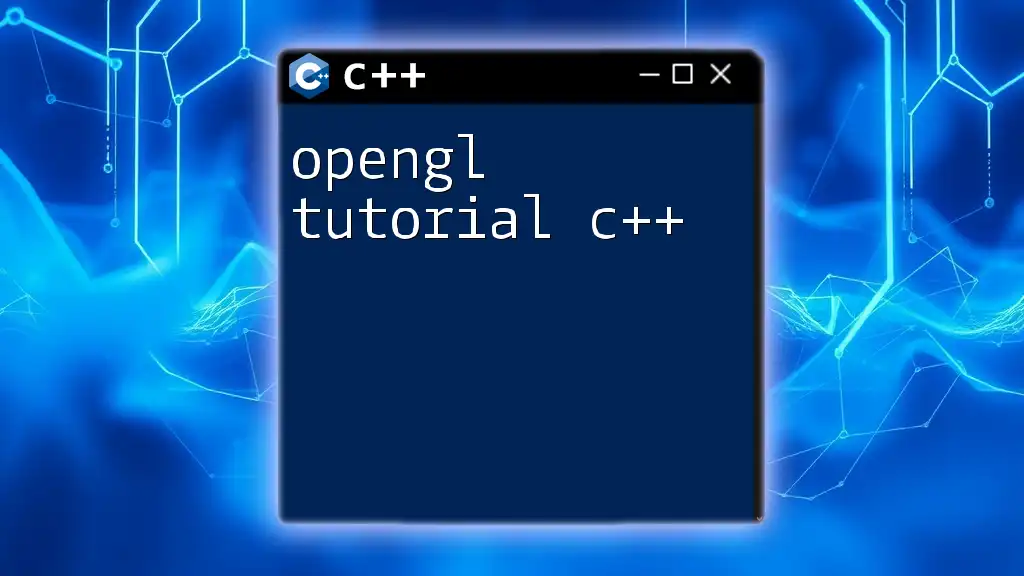
Understanding the Basic Structure of a Makefile
Components of a Makefile
A standard Makefile consists of three key components:
-
Targets: These specify what you want to build. Each target can include an executable, an object file, or even a phony target like `clean`.
-
Dependencies: For any target, dependencies denote which files must be up to date for the target to build successfully. If a dependency has changed, the target will be rebuilt.
-
Commands: Each target and its respective dependencies are followed by commands, which are executed when you build the target.
Basic Syntax of Makefiles
Makefile syntax follows specific formatting rules:
- Start each command with a tab (not spaces).
- Use colons to separate targets from their dependencies.
- Commands must be on a new line and begin with a tab.
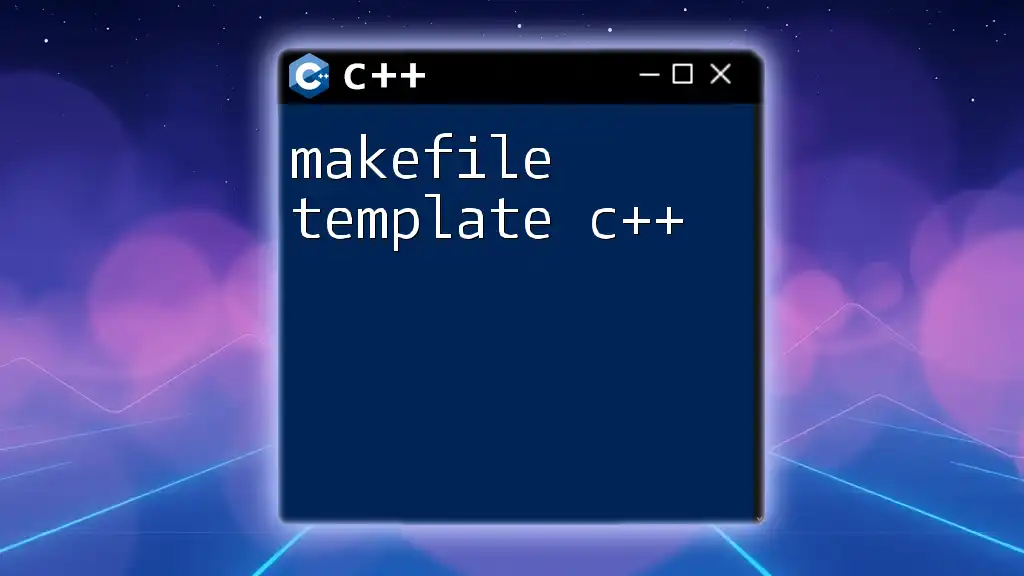
How to Create a Simple Makefile for a C++ Project
Creating Your Project Structure
Before you can write a Makefile, it’s essential to have a logical project structure. Here’s a common layout:
project_directory/
├── src/
│ └── hello.cpp
├── include/
│ └── hello.h
└── Makefile
Writing Your First Makefile
Let’s create a simple Makefile for a C++ program that prints "Hello, World!" to the console. Here’s how it looks:
all: hello
hello: hello.o
g++ -o hello hello.o
hello.o: hello.cpp
g++ -c hello.cpp
clean:
rm -f hello.o hello
In this Makefile:
- The `all` target builds the executable `hello`.
- The `hello` target has a dependency on `hello.o`. The command compiles `hello.o` into the final executable.
- The `hello.o` target indicates how to compile the C++ source file `hello.cpp`.
- The `clean` target removes the generated files, allowing for a fresh build.
Common Variables in Makefiles
You can simplify your Makefile by using variables to manage the compiler, compiler flags, and object files. Here’s an example:
CC = g++
CXXFLAGS = -Wall -g
OBJ = main.o utils.o
all: program
program: $(OBJ)
$(CC) -o program $(OBJ)
Here, `CC` is set to the standard C++ compiler, `CXXFLAGS` includes options to show all warnings and enable debugging, while `OBJ` holds the object files to be linked. This approach adds clarity and flexibility to your Makefile.
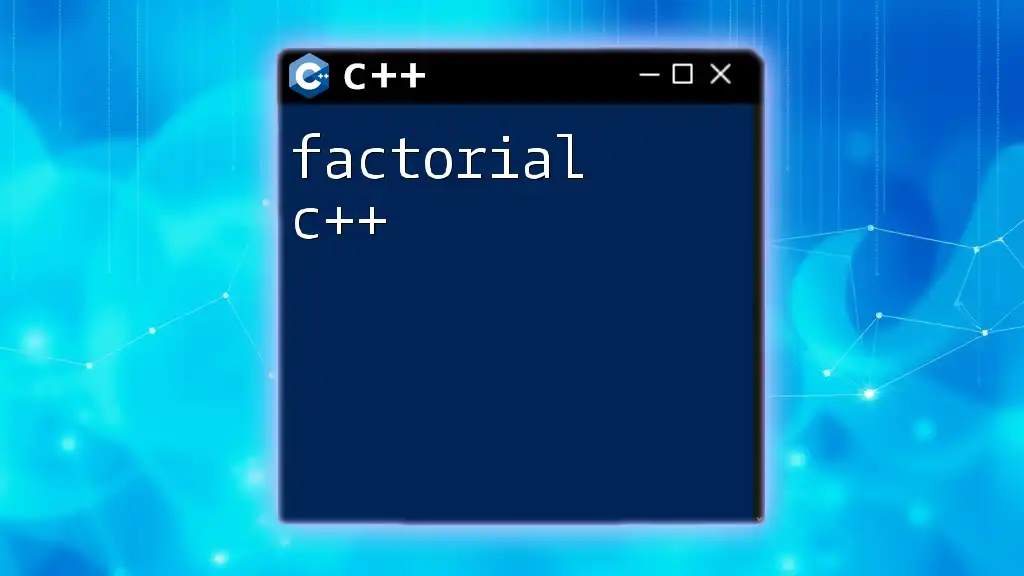
Advanced Makefile Features
Adding Multiple Source Files
As your project grows, you’ll likely have multiple source files. Here's how to handle it efficiently:
SOURCES = main.cpp utils.cpp
OBJECTS = $(SOURCES:.cpp=.o)
all: program
program: $(OBJECTS)
$(CC) -o program $(OBJECTS)
This snippet dynamically creates object files from your source files. The `$(SOURCES:.cpp=.o)` syntax is a powerful feature in Makefiles for pattern substitution, ensuring that all `.cpp` files are compiled into corresponding `.o` files.
Phony Targets
Phony targets are necessary for actions that do not directly correspond to files. Common examples include:
- `all`: Used to denote the default make action.
- `clean`: Used to clean up files.
Marking a target as phony can be done like this:
.PHONY: all clean
clean:
rm -rf *.o program
This ensures that the `clean` action is executed, even if a file named `clean` exists in the directory.
Conditional Statements and Nested Makefiles
Conditional statements allow for more control over your Makefile's behavior. Here’s an example:
ifeq ($(OS), Windows_NT)
RM = del
else
RM = rm -f
endif
This snippet defines the `RM` variable to use the appropriate remove command depending on the operating system. Such flexibility makes your Makefile more robust.
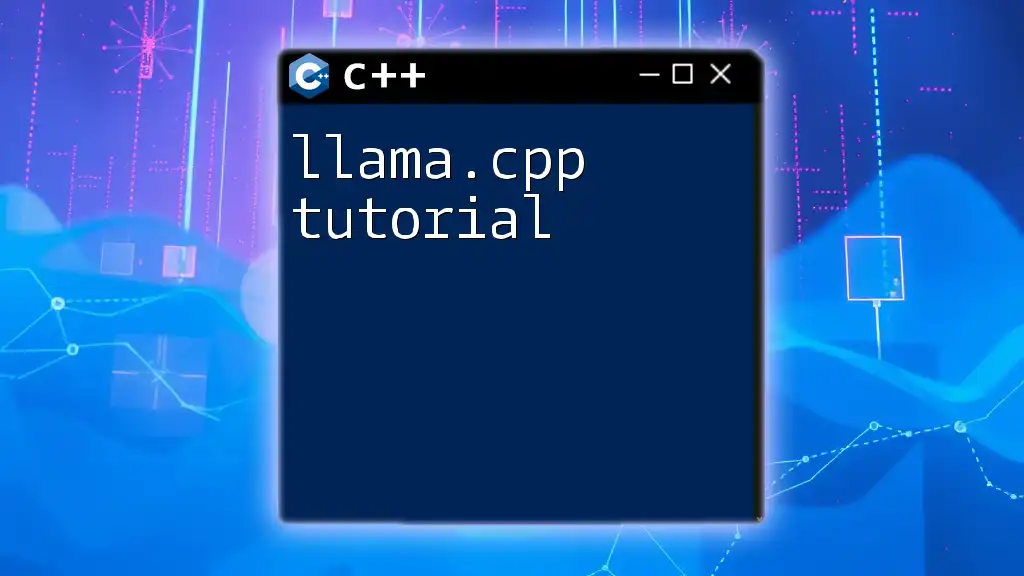
Debugging and Troubleshooting Makefiles
Common Errors in Makefiles
Errors often stem from improper syntax or incorrect dependencies. Common mistakes include:
- Forgetting to start commands with a tab.
- Misnaming files or using incorrect file paths in dependencies.
Debugging Makefile Issues
If something goes wrong, you can gain insights into what `make` is doing by using:
make -d
This command will display detailed information about the make process, helping you trace where things went awry.
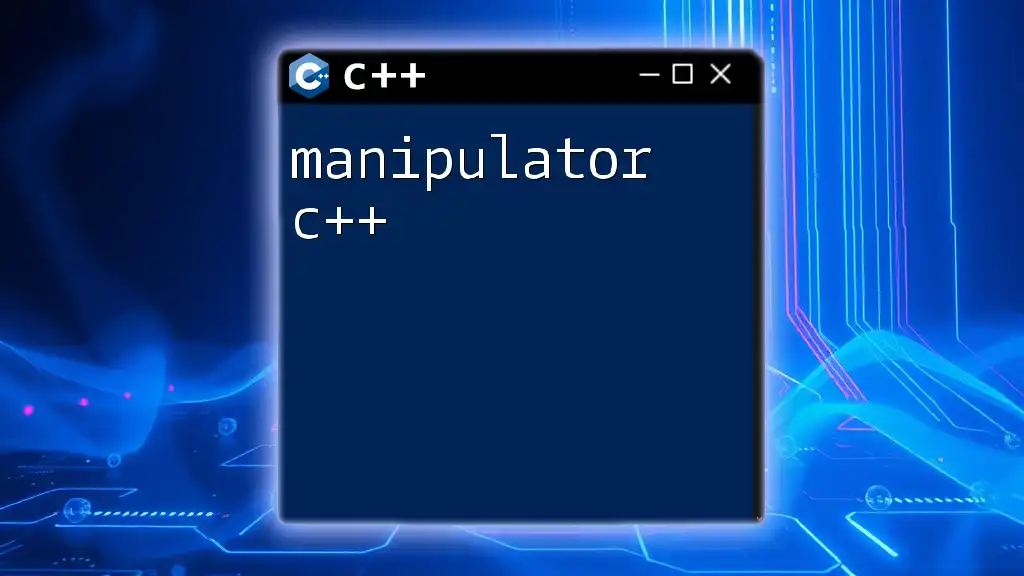
Example C++ Makefile Projects
Simple Calculator Project
Consider building a simple calculator. The project may look like this:
calculator/
├── src/
│ ├── main.cpp
│ └── calculator.cpp
└── Makefile
An example Makefile for this project could be:
SOURCES = main.cpp calculator.cpp
OBJECTS = $(SOURCES:.cpp=.o)
all: calculator
calculator: $(OBJECTS)
$(CC) -o calculator $(OBJECTS)
clean:
$(RM) $(OBJECTS) calculator
File Management Utility
For more complex projects, such as a file management utility, the directory could include libraries and multiple source files. An example Makefile might look like this:
SOURCES = main.cpp file_handler.cpp utility.cpp
OBJECTS = $(SOURCES:.cpp=.o)
all: file_manager
file_manager: $(OBJECTS)
$(CC) -o file_manager $(OBJECTS)
clean:
$(RM) $(OBJECTS) file_manager
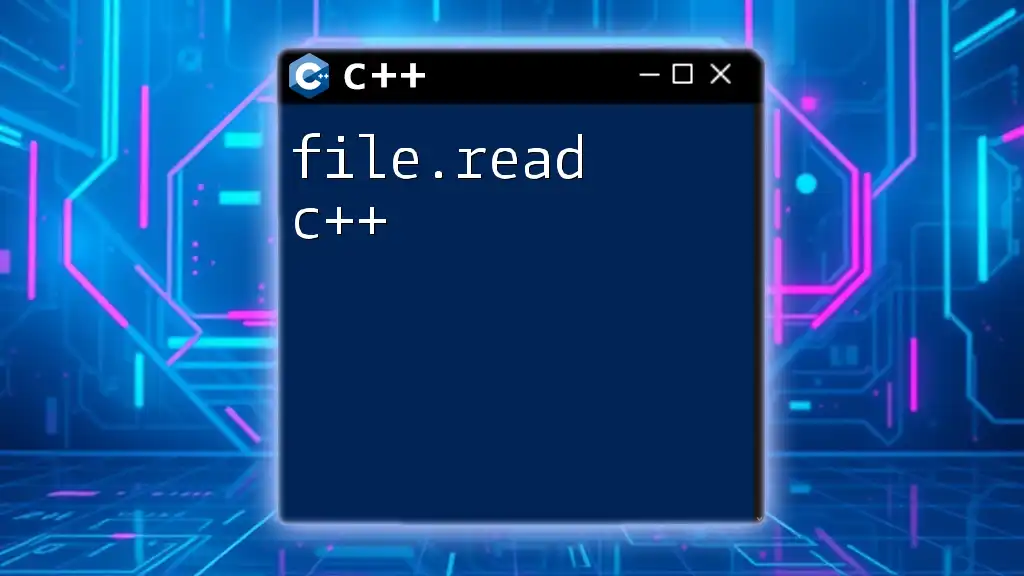
Conclusion
Using a Makefile effectively streamlines your C++ development, minimizing errors and saving time while handling complex builds. By structuring your Makefile and including appropriate dependencies and targets, you pave the way for easier, more efficient project management.
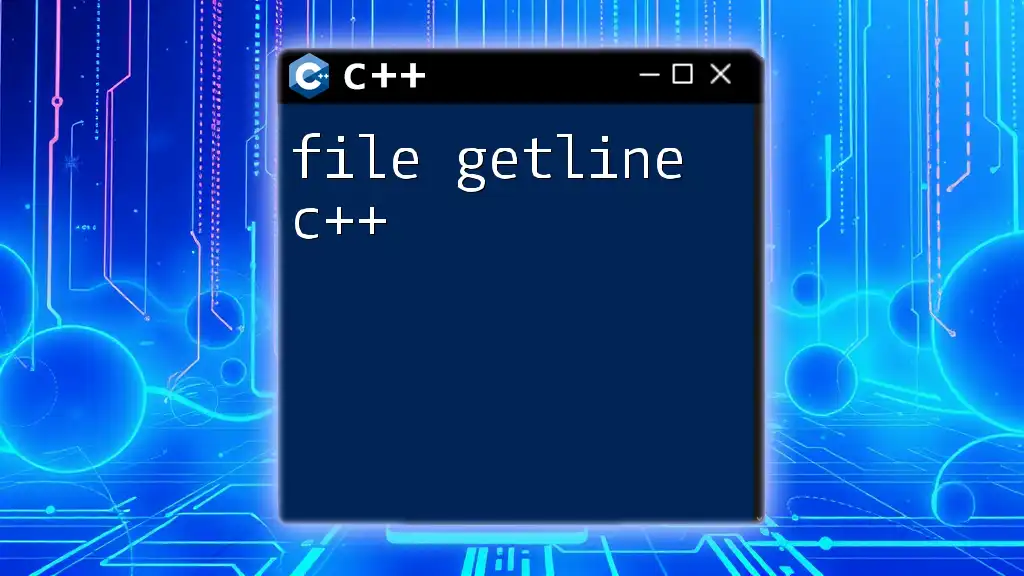
Additional Resources
To deepen your understanding, consider exploring further readings on advanced Makefile features. Online forums, documentation, and community discussions can also provide valuable insights.
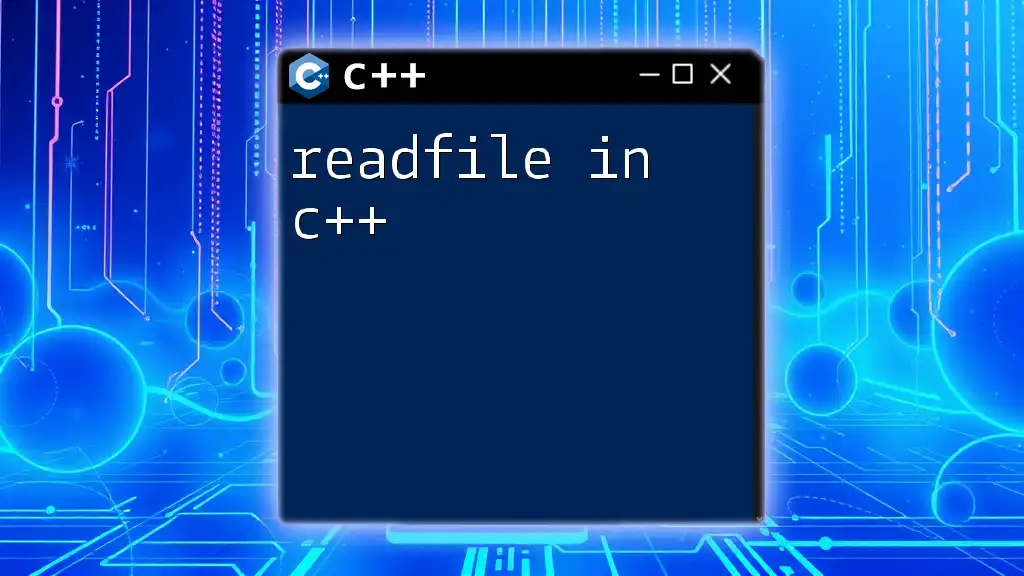
Call to Action
Have you created a Makefile for your project? Share your experiences or questions with us! Stay tuned for more tutorials and resources to enhance your C++ skills.