The error "undefined reference to main" in C++ indicates that the compiler cannot find the main function, which serves as the entry point for the program.
Here's an example code snippet that includes a properly defined main function:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is the 'main' Function in C++?
Definition and Purpose
In C++, the `main` function serves as the entry point of a program. It is where execution begins, and every C++ program must contain a `main` function for it to function properly. The standard format for the `main` function includes a return type of `int`. Its primary purpose is to execute the program's logic and return an integer to the operating system, indicating whether the program completed successfully.
Example of a basic main function:
int main() {
return 0;
}
Implicit and Explicit Declarations
While `main` can be defined with no parameters, it can also accept command-line arguments. The two common forms of the `main` function are:
-
No parameters: In this version, `main` does not take any arguments.
-
With parameters: Here, `main` can accept command-line arguments, which can be useful in many scenarios.
Code Snippet: Different versions of the `main` function
int main() {
return 0; // A simple main function with no parameters
}
int main(int argc, char *argv[]) {
return 0; // A main function that accepts command-line arguments
}

Understanding the Error: Undefined Reference to Main
What Does "Undefined Reference to Main" Mean?
The error "undefined reference to `main`" typically arises during the linking stage of the C++ compilation process. It implies that the linker cannot find the defined `main` function, which is a requirement for executing any C++ program.
Common Scenarios Leading to the Error
There are several common scenarios in which this error might occur:
-
Missing `main` function: If your code lacks a proper implementation of the `main` function, you'll encounter this error.
-
Incorrect filename conventions: Sometimes, the project structure and naming conventions can lead to confusion, especially in larger projects.
Code Snippet: Scenario with missing main
// missing main function
void myFunction() {
// Function logic
}
// This will result in "undefined reference to main" during linking.

Causes of the Undefined Reference to Main Error
Mistakes with the Function Signature
One of the frequent causes of the "undefined reference to `main`" error is providing an incorrect function signature.
- The `main` function must have an int return type.
- It should also not have parameters when no arguments are needed.
Code Snippet: Incorrect signature
void main() { // Incorrect return type
return 0; // This would lead to linking errors
}
Compiling Multiple Files Without Main
When dealing with multiple source files, it is crucial to ensure that one of the files includes a correctly defined `main`. If you compile multiple files without a proper entry point, you'll receive the undefined reference error.
Code Snippet: Multiple files, missing main
// File1.cpp
void example() {
// Function logic
}
// File2.cpp
// Missing the main function will lead to "undefined reference to main"
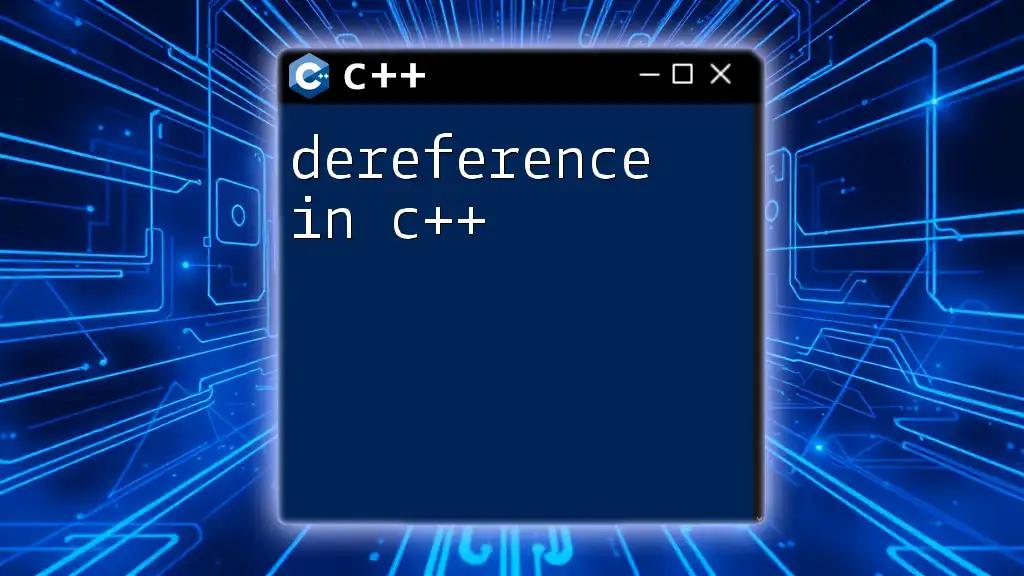
How to Fix the "Undefined Reference to Main" Error
Ensure a Properly Defined Main Function
The first step to resolve this error is to confirm that you have a `main` function defined in your code. It should follow the standard form:
- Return type: `int`
- Name: `main`
- Proper parameters (if applicable)
Example: Correct main function
int main() {
// Your program logic here
return 0; // correctly returns an int
}
Check Compilation and Linking Process
Another crucial factor is verifying how you compile and link your code. If you are working with multiple source files, you need to ensure they're compiled together correctly so that the linker can resolve all references, including the `main` function.
You can compile multiple files using a command such as:
Example: Compilation command in g++
g++ main.cpp otherfile.cpp -o output_program
This command compiles `main.cpp` and `otherfile.cpp` into a single executable called `output_program`. Be sure that at least one of these files contains a valid `main` function.
Verify File Names and Project Structure
A critical aspect to consider is that the naming of your files should adhere to conventional practices. Often, the issue arises from simple mistakes like using incorrect file names or having a misaligned project structure. Ensuring a clear and logical project structure can help avoid these errors altogether.

Best Practices to Avoid "Undefined Reference to Main" Errors
Consistent Coding Standards
Maintaining consistent conventions across your C++ code helps to avoid confusion. Ensuring that every program has a defined `main` function with a recognizable signature can save time and effort in debugging.
Regular Compilation Checks
Integrating frequent compilation checks into your development workflow is essential. Doing so can ensure that any errors are caught early on, preventing frustration during later stages of development. Many IDEs come equipped with features that flag issues in real time, which is beneficial for maintaining a clean codebase.
Utilizing Comments and Documentation
Keeping your code well-documented is not just best practice; it’s essential for understanding your code over time. Commenting on the purpose of the `main` function and describing its logic can help others (or even yourself) understand your code better, which is priceless when troubleshooting errors like the "undefined reference to main".

Conclusion
In summary, the "undefined reference to `main`" error is often a straightforward issue rooted in programming structure or syntax. Understanding the function of `main`, ensuring correct signatures, and adhering to best practices can help you avoid this common stumbling block.
By embracing these principles, you can navigate the nuances of C++ programming more easily and develop robust applications with fewer headaches.

Further Reading and Resources
For those eager to explore more on C++ errors and debugging techniques, consider checking out forums, online communities, and further educational resources dedicated to C++. Being part of these communities can also provide additional support and insights into common programming challenges.

Call to Action
Your experiences matter! Share your run-ins with the "undefined reference to main" error, and let's learn together. Don't forget to subscribe for newsletters or enroll in courses to deepen your understanding of C++ programming and its complexities.