In C++, a pointer is a variable that stores the memory address of another variable, enabling direct memory access and manipulation.
Here’s a simple code snippet demonstrating the use of pointers:
#include <iostream>
using namespace std;
int main() {
int num = 42;
int *ptr = # // Pointer to num
cout << "Value of num: " << num << endl; // Output: 42
cout << "Value via pointer: " << *ptr << endl; // Output: 42
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. It allows you to directly manipulate memory and access variables in memory using their addresses. To declare a pointer, you use the `*` symbol. Here’s a simple example of declaring and initializing a pointer:
int a = 10;
int *ptr = &a; // Pointer to an integer
In this example, `ptr` is a pointer that holds the address of the variable `a`. The `&` operator is used to obtain the address of a variable.
Why Use Pointers?
Pointers are essential for various reasons:
- Memory Management: Pointers enable dynamic memory allocation, allowing the creation of variables at runtime.
- Pass-by-Reference vs Pass-by-Value: Pointers allow you to modify the original data instead of working with a copy. This is particularly useful in functions where you want changes to persist outside the function's scope. For instance, consider the following function that increments a value:
void increment(int* ptr) {
(*ptr)++;
}
Here, the `increment` function accepts a pointer to `int`, allowing it to modify the value of the variable it points to directly.
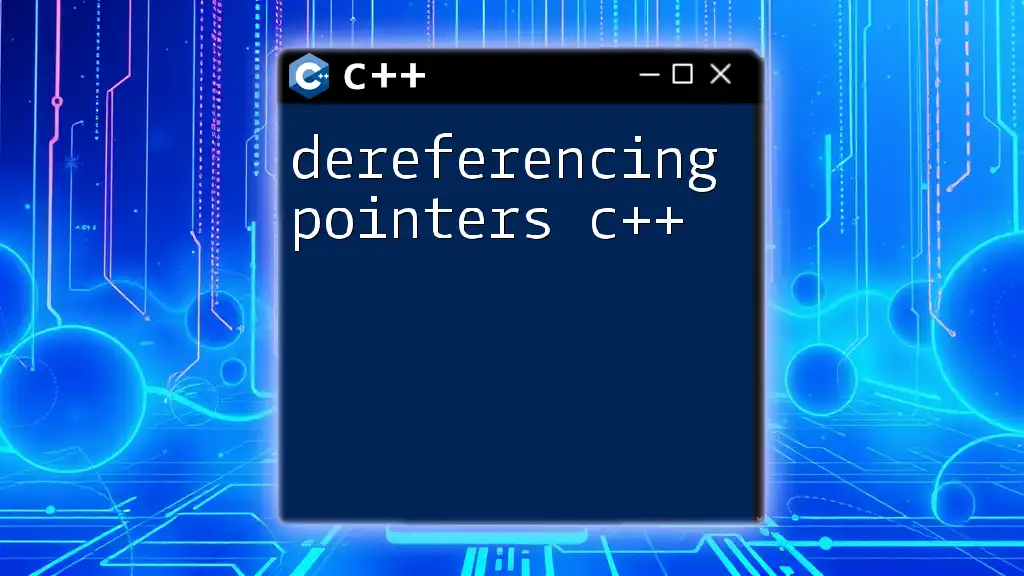
Understanding References in C++
What is a Reference?
In C++, a reference is an alias for another variable. It provides a way to reference a variable without using pointers or needing to dereference them. To declare a reference, you use the `&` symbol:
int b = 20;
int& ref = b; // Reference to an integer
In this case, `ref` acts as an alias for `b`. Any modifications made through `ref` directly affect `b`.
Benefits of Using References
Using references in C++ has several advantages:
- Safer and Easier to Use: Since references cannot be null and must always refer to a valid variable, they eliminate the risk of dereferencing a null pointer.
- Automatic Dereferencing: No need to manually dereference a pointer when accessing the value. This makes the syntax cleaner and easier to read.
For instance, consider the following function, which updates a value using a reference:
void update(int& ref) {
ref += 10;
}
When you call `update(b);`, the value of `b` increases by 10 without needing to handle pointers.
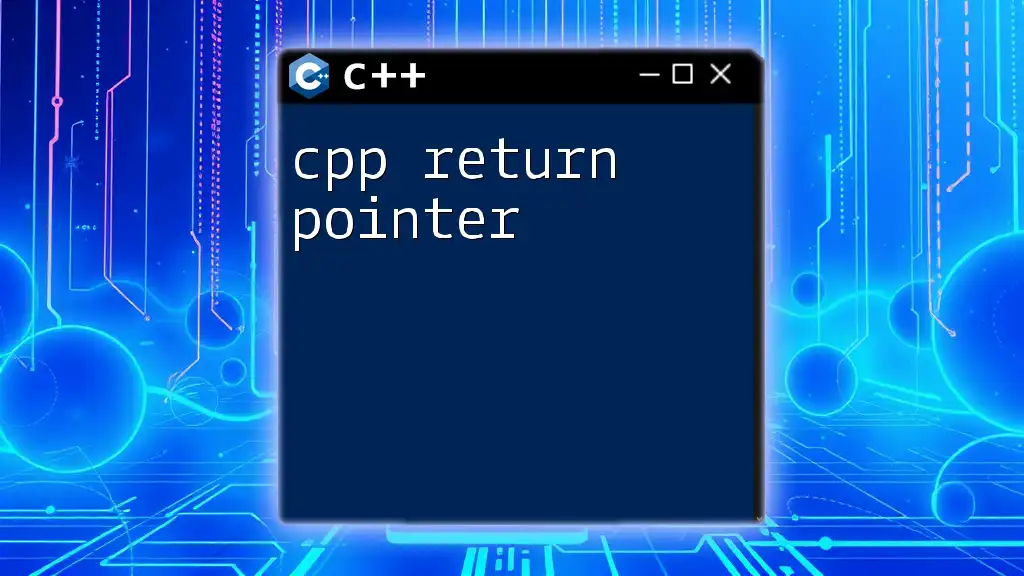
C++ Reference to Pointer
What is a Reference to a Pointer?
A reference to a pointer is a reference that allows direct access and modification of a pointer itself. This is achieved by declaring a reference with the pointer type:
int a = 30;
int* ptr = &a;
int*& refPtr = ptr; // Reference to a pointer
Now, `refPtr` is a reference to the pointer `ptr`. Any changes made through `refPtr` affect the original pointer.
When to Use Reference to Pointer
References to pointers are particularly useful when you need to modify the pointer itself in functions. For example, if you want to swap two pointers, using a reference to a pointer simplifies the syntax and the operation:
void swapPointers(int*& a, int*& b) {
int* temp = a;
a = b;
b = temp;
}
By using references to pointers, you avoid the necessity to return the pointer from the function, making the function call cleaner and more intuitive.
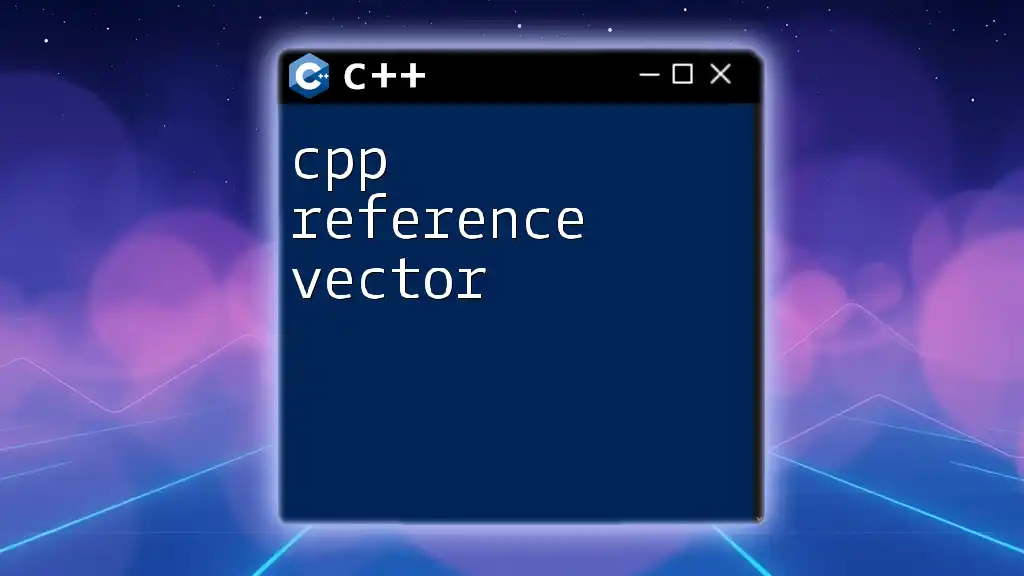
Advantages of Using References to Pointers
Simplifying Pointer Manipulation
Using references to pointers greatly reduces the complexity of pointer manipulation. It allows for cleaner function signatures and minimizes the risk of pointer-related errors. For instance, functions can modify pointers more transparently without the need for additional return values.
Safety and Readability
Code that uses references to pointers is often more readable. Since pointers can be tricky, especially for beginners, references to pointers can reduce cognitive load by providing a clear indication that you're dealing with pointer manipulation. This improves maintainability and can help prevent errors during debugging.
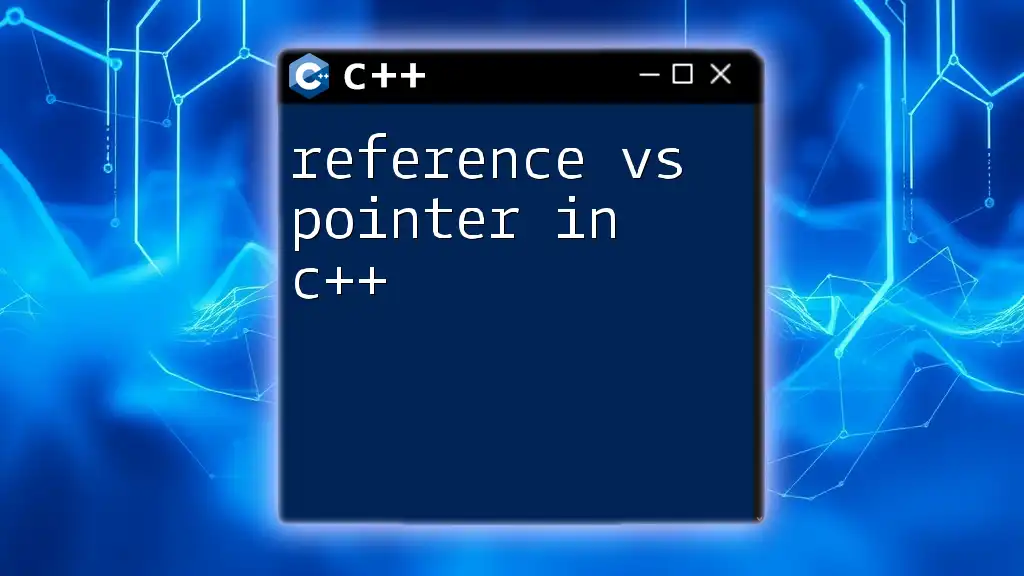
Common Mistakes and Pitfalls
Confusing References with Pointers
One common mistake among programmers is confusing references with pointers. While both are used for similar purposes, references cannot be null and must always be initialized. This misconception can lead to bugs in the code. Understanding the differences between the two concepts is crucial for effective programming in C++.
Forgetting Memory Management When Using Pointers
Memory management is critical in C++. When using pointers, it's essential to ensure that dynamically allocated memory is properly managed. Failure to do so can lead to memory leaks. For example, neglecting to delete a dynamically allocated pointer can result in memory that is never freed:
int* dynamicInt = new int(5); // Dynamically allocated memory
// ... Use dynamicInt ...
delete dynamicInt; // Free the allocated memory
When you’re using pointers, always remember to deallocate memory when it’s no longer needed.
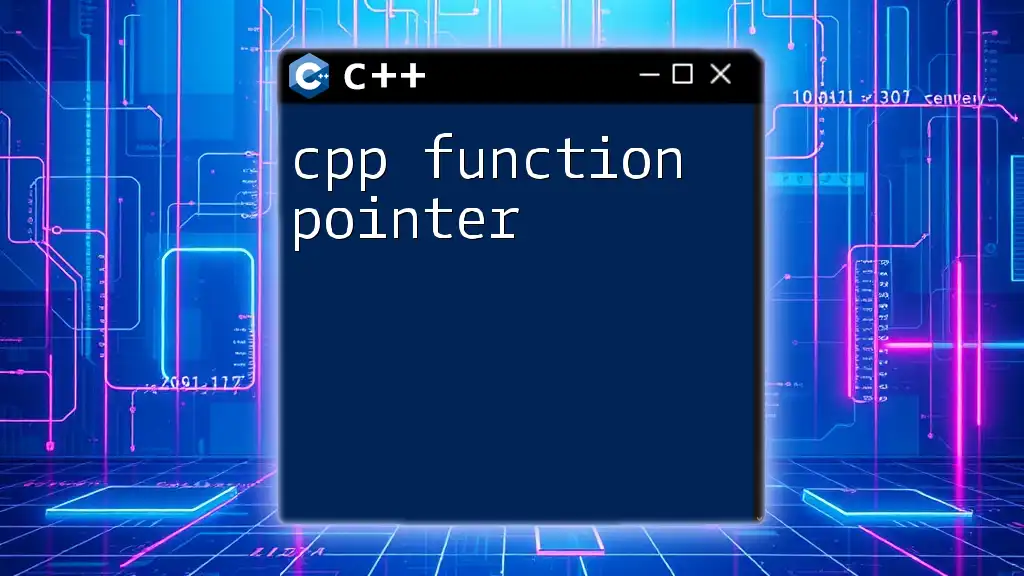
Conclusion
Understanding cpp reference to pointer is fundamental for effective C++ programming. References to pointers provide a comfortable way to manipulate pointers without the associated risks of raw pointer usage. By mastering this concept, you can write safer, more efficient, and easily maintainable code. Remember to practice the examples provided to reinforce your learning, and don't hesitate to explore additional resources to enhance your understanding of pointers and references in C++.